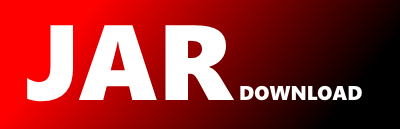
com.kolibrifx.plovercrest.performance.tools.serialization.PriceData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plovercrest-performance-tools Show documentation
Show all versions of plovercrest-performance-tools Show documentation
Performance testing tools for Plovercrest.
The newest version!
/*
* Copyright (c) 2010-2017, KolibriFX AS. Licensed under the Apache License, version 2.0.
*/
package com.kolibrifx.plovercrest.performance.tools.serialization;
import com.kolibrifx.napo.base.Param;
import com.kolibrifx.napo.base.Timestamp;
public class PriceData {
private final long timestamp;
private final double bidPrice;
private final double offerPrice;
private final double bidSize;
private final double offerSize;
public PriceData(@Param("Timestamp") final long timestamp,
@Param("BidPrice") final double bidPrice,
@Param("OfferPrice") final double offerPrice,
@Param("BidSize") final double bidSize,
@Param("OfferSize") final double offerSize) {
this.timestamp = timestamp;
this.bidPrice = bidPrice;
this.offerPrice = offerPrice;
this.bidSize = bidSize;
this.offerSize = offerSize;
}
public double getBidPrice() {
return bidPrice;
}
public double getBidSize() {
return bidSize;
}
public double getOfferPrice() {
return offerPrice;
}
public double getOfferSize() {
return offerSize;
}
@Timestamp
public long getTimestamp() {
return timestamp;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
long temp;
temp = Double.doubleToLongBits(bidPrice);
result = prime * result + (int) (temp ^ (temp >>> 32));
temp = Double.doubleToLongBits(bidSize);
result = prime * result + (int) (temp ^ (temp >>> 32));
temp = Double.doubleToLongBits(offerPrice);
result = prime * result + (int) (temp ^ (temp >>> 32));
temp = Double.doubleToLongBits(offerSize);
result = prime * result + (int) (temp ^ (temp >>> 32));
result = prime * result + (int) (timestamp ^ (timestamp >>> 32));
return result;
}
@Override
public boolean equals(final Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (getClass() != obj.getClass()) {
return false;
}
final PriceData other = (PriceData) obj;
if (Double.doubleToLongBits(bidPrice) != Double.doubleToLongBits(other.bidPrice)) {
return false;
}
if (Double.doubleToLongBits(bidSize) != Double.doubleToLongBits(other.bidSize)) {
return false;
}
if (Double.doubleToLongBits(offerPrice) != Double.doubleToLongBits(other.offerPrice)) {
return false;
}
if (Double.doubleToLongBits(offerSize) != Double.doubleToLongBits(other.offerSize)) {
return false;
}
if (timestamp != other.timestamp) {
return false;
}
return true;
}
@Override
public String toString() {
return "PriceData [timestamp=" + timestamp + ", bidPrice=" + bidPrice + ", offerPrice=" + offerPrice
+ ", bidSize=" + bidSize + ", offerSize=" + offerSize + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy