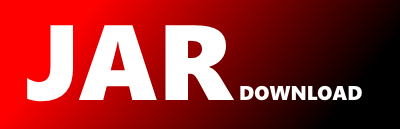
com.kotlinnlp.linguisticconditions.conditions.agreement.TokensAgreement.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of linguisticconditions Show documentation
Show all versions of linguisticconditions Show documentation
A helper module for verifying linguistic conditions on morpho-syntactic tokens.
/* Copyright 2018-present The KotlinNLP Authors. All Rights Reserved.
*
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, you can obtain one at http://mozilla.org/MPL/2.0/.
* ------------------------------------------------------------------*/
package com.kotlinnlp.linguisticconditions.conditions.agreement
import com.beust.klaxon.JsonObject
import com.kotlinnlp.dependencytree.DependencyTree
import com.kotlinnlp.linguisticconditions.conditions.DoubleCondition
import com.kotlinnlp.linguisticdescription.sentence.token.MorphoSynToken
/**
* The condition that verifies the morphological agreement between two tokens.
*
* @param checkContext whether to check the agreement looking at the context morphology
* @param lemma whether to check the agreement of the 'lemma' property of the morphology
* @param pos whether to check the agreement of the 'pos' property of the morphology
* @param gender whether to check the agreement of the 'gender' property of the morphology
* @param number whether to check the agreement of the 'number' property of the morphology
* @param person whether to check the agreement of the 'person' property of the morphology
* @param case whether to check the agreement of the 'grammatical case' property of the morphology
* @param degree whether to check the agreement of the 'degree' property of the morphology
* @param mood whether to check the agreement of the 'mood' property of the morphology
* @param tense whether to check the agreement of the 'tense' property of the morphology
*/
internal class TokensAgreement(
override val checkContext: Boolean = false,
override val lemma: Boolean = false,
override val pos: Boolean = false,
override val gender: Boolean = false,
override val number: Boolean = false,
override val person: Boolean = false,
override val case: Boolean = false,
override val degree: Boolean = false,
override val mood: Boolean = false,
override val tense: Boolean = false
) : MorphoAgreement, DoubleCondition() {
companion object {
/**
* The annotation of the condition.
*/
const val ANNOTATION: String = "tokens-agreement"
}
/**
* Build a [TokensAgreement] condition from a JSON object.
*
* @param jsonObject the JSON object that represents a [TokensAgreement] condition
*
* @return a new condition interpreted from the given [jsonObject]
*/
constructor(jsonObject: JsonObject) : this(
checkContext = jsonObject.boolean("context") ?: false,
lemma = jsonObject.array("properties")!!.contains("lemma"),
pos = jsonObject.array("properties")!!.contains("pos"),
gender = jsonObject.array("properties")!!.contains("gender"),
number = jsonObject.array("properties")!!.contains("number"),
person = jsonObject.array("properties")!!.contains("person"),
case = jsonObject.array("properties")!!.contains("case"),
degree = jsonObject.array("properties")!!.contains("degree"),
mood = jsonObject.array("properties")!!.contains("mood"),
tense = jsonObject.array("properties")!!.contains("tense")
)
/**
* Whether this condition looks at a single token, without requiring to check other tokens properties.
*/
override val isUnary: Boolean = false
/**
* Whether this condition looks at a dependent-governor tokens pair, without requiring to check other tokens
* properties.
*/
override val isBinary: Boolean = true
/**
* Whether this condition needs to look at the morphology.
*/
override val checkMorpho: Boolean = true
/**
* Whether this condition needs to look at the morphological properties.
*/
override val checkMorphoProp: Boolean = sequenceOf(gender, number, person, case, degree, mood, tense).any()
/**
* Check requirements.
*/
init {
require(!this.checkContext || this.checkMorphoProp) {
"The 'checkContext' property cannot be true if the condition does not check morphological properties."
}
}
/**
* @param tokenA a token of the sentence
* @param tokenB a token of the sentence
* @param tokens the list of all the tokens that compose the sentence
* @param dependencyTree the dependency tree of the token sentence
*
* @return a boolean indicating if this condition is verified for the two given tokens
*/
override fun isVerified(tokenA: MorphoSynToken.Single,
tokenB: MorphoSynToken.Single,
tokens: List,
dependencyTree: DependencyTree): Boolean = this.isVerified(tokenA, tokenB)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy