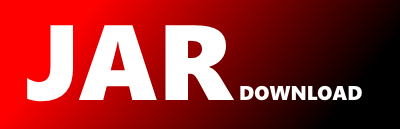
com.kotlinnlp.linguisticdescription.morphology.MorphologyFactory.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of linguisticdescription Show documentation
Show all versions of linguisticdescription Show documentation
LinguisticDescription is a Kotlin library designed to support linguistic annotations over morphological,
syntactic and semantic levels.
/* Copyright 2017-present The KotlinNLP Authors. All Rights Reserved.
*
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/.
* ------------------------------------------------------------------*/
package com.kotlinnlp.linguisticdescription.morphology
import com.kotlinnlp.linguisticdescription.morphology.properties.MorphologyProperty
import com.kotlinnlp.linguisticdescription.MissingMorphologyProperty
import kotlin.reflect.KClass
import kotlin.reflect.KFunction
import kotlin.reflect.KParameter
/**
* The factory of a new [SingleMorphology].
*/
object MorphologyFactory {
/**
* Create a new [SingleMorphology] given its [properties].
*
* @param lemma the lemma of the morphology
* @param type the morphology type
* @param properties the map of property names to their values (optional, unnecessary adding properties are ignored)
* @param allowIncompleteProperties allow to build the morphology even if the [properties] map does not contain all
* the required properties (default = false)
*
* @throws MissingMorphologyProperty when a required property is missing
*
* @return a new morphology
*/
operator fun invoke(lemma: String,
type: MorphologyType,
properties: Map = mapOf(),
allowIncompleteProperties: Boolean = false): SingleMorphology {
require(type != MorphologyType.Num) {
"'NUM' morphologies cannot be created with the factory because they have an adding 'numericForm' property."
}
val kClass: KClass<*> = morphologyClasses[type]!!
val constructor: KFunction = kClass.constructors.last()
val keywordArgs: Map = mapOf(
*constructor.parameters
.mapNotNull {
val propertyName: String = it.name!!
when {
propertyName == "lemma" -> it to lemma
propertyName in properties -> it to properties.getValue(propertyName)
allowIncompleteProperties -> null
else -> throw MissingMorphologyProperty(propertyName = propertyName, morphologyType = type, lemma = lemma)
}
}
.toTypedArray()
)
return constructor.callBy(keywordArgs) as SingleMorphology
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy