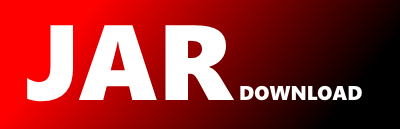
com.kotlinnlp.utils.Parallelization.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of utils Show documentation
Show all versions of utils Show documentation
Utils is a Kotlin package containing utilities for the KotlinNLP library.
The newest version!
/* Copyright 2018-present The KotlinNLP Authors. All Rights Reserved.
*
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/.
* ------------------------------------------------------------------*/
package com.kotlinnlp.utils
import java.util.concurrent.*
import java.util.concurrent.atomic.AtomicInteger
/**
* Map the elements of this iterable executing the [transform] function in parallel threads and collecting the results.
* If given, at max [maxConcurrentThreads] threads are executed in parallel.
*
* @param maxConcurrentThreads the max number of concurrent threads at a time
* @param transform the transform function applied to each element of this collection
*/
fun Iterable.pmap(maxConcurrentThreads: Int? = null, transform: (T) -> R): List {
require(maxConcurrentThreads == null || maxConcurrentThreads > 0) {
"The number of max concurrent threads must be greater than 0."
}
if (maxConcurrentThreads == 1) return this.map(transform)
val sem: Semaphore? = maxConcurrentThreads?.let { Semaphore(it) }
val results = ConcurrentHashMap()
val elements: List = this.toList()
val nextElmIndex = AtomicInteger(0)
List(maxConcurrentThreads ?: elements.size) {
Thread {
var elmIndex: Int = nextElmIndex.getAndIncrement()
while (elmIndex < elements.size) {
sem?.acquire()
results[elmIndex] = transform(elements[elmIndex])
elmIndex = nextElmIndex.getAndIncrement()
sem?.release()
}
}.apply { start() }
}.forEach { it.join() }
return elements.indices.map { elmIndex -> results.getValue(elmIndex) }
}