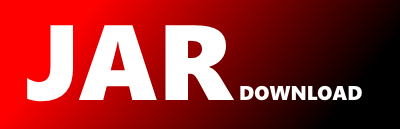
com.dominodatalab.api.model.DominoDatasetrwApiDatasetRwDto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of domino-java-client Show documentation
Show all versions of domino-java-client Show documentation
Domino Data Lab API Client to connect to Domino web services using Java HTTP Client.
/*
* Domino Data Lab API v4
* This API provides access to select Domino functions available in Domino's non-public API. To authenticate your requests, include your API Key (which you can find on your account page) with the header X-Domino-Api-Key.
*
* The version of the OpenAPI document: 4.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.api.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.dominodatalab.api.model.DominoDatasetrwApiDatasetRwSizeStatus;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.dominodatalab.api.invoker.ApiClient;
/**
* DominoDatasetrwApiDatasetRwDto
*/
@JsonPropertyOrder({
DominoDatasetrwApiDatasetRwDto.JSON_PROPERTY_ACTIVE_R_O_SNAPSHOT_COUNT,
DominoDatasetrwApiDatasetRwDto.JSON_PROPERTY_AUTHOR,
DominoDatasetrwApiDatasetRwDto.JSON_PROPERTY_CREATED_TIME,
DominoDatasetrwApiDatasetRwDto.JSON_PROPERTY_DATA_PLANE_IDS,
DominoDatasetrwApiDatasetRwDto.JSON_PROPERTY_DATASET_PATH,
DominoDatasetrwApiDatasetRwDto.JSON_PROPERTY_DESCRIPTION,
DominoDatasetrwApiDatasetRwDto.JSON_PROPERTY_ID,
DominoDatasetrwApiDatasetRwDto.JSON_PROPERTY_LIFECYCLE_STATUS,
DominoDatasetrwApiDatasetRwDto.JSON_PROPERTY_NAME,
DominoDatasetrwApiDatasetRwDto.JSON_PROPERTY_OWNER_USERNAMES,
DominoDatasetrwApiDatasetRwDto.JSON_PROPERTY_PROJECT_ID,
DominoDatasetrwApiDatasetRwDto.JSON_PROPERTY_READ_WRITE_SNAPSHOT_ID,
DominoDatasetrwApiDatasetRwDto.JSON_PROPERTY_SIZE_IN_BYTES,
DominoDatasetrwApiDatasetRwDto.JSON_PROPERTY_SIZE_STATUS,
DominoDatasetrwApiDatasetRwDto.JSON_PROPERTY_SNAPSHOT_IDS,
DominoDatasetrwApiDatasetRwDto.JSON_PROPERTY_STATUS_LAST_UPDATED_BY,
DominoDatasetrwApiDatasetRwDto.JSON_PROPERTY_STATUS_LAST_UPDATED_TIME,
DominoDatasetrwApiDatasetRwDto.JSON_PROPERTY_TAGS
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-10-04T16:37:26.309454-04:00[America/New_York]", comments = "Generator version: 7.8.0")
public class DominoDatasetrwApiDatasetRwDto {
public static final String JSON_PROPERTY_ACTIVE_R_O_SNAPSHOT_COUNT = "activeROSnapshotCount";
private Integer activeROSnapshotCount;
public static final String JSON_PROPERTY_AUTHOR = "author";
private String author;
public static final String JSON_PROPERTY_CREATED_TIME = "createdTime";
private Long createdTime;
public static final String JSON_PROPERTY_DATA_PLANE_IDS = "dataPlaneIds";
private List dataPlaneIds = new ArrayList<>();
public static final String JSON_PROPERTY_DATASET_PATH = "datasetPath";
private String datasetPath;
public static final String JSON_PROPERTY_DESCRIPTION = "description";
private String description;
public static final String JSON_PROPERTY_ID = "id";
private String id;
/**
* Gets or Sets lifecycleStatus
*/
public enum LifecycleStatusEnum {
PENDING("Pending"),
DELETED("Deleted"),
DELETION_IN_PROGRESS("DeletionInProgress"),
FAILED("Failed"),
ACTIVE("Active"),
MARKED_FOR_DELETION("MarkedForDeletion");
private String value;
LifecycleStatusEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static LifecycleStatusEnum fromValue(String value) {
for (LifecycleStatusEnum b : LifecycleStatusEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_LIFECYCLE_STATUS = "lifecycleStatus";
private LifecycleStatusEnum lifecycleStatus;
public static final String JSON_PROPERTY_NAME = "name";
private String name;
public static final String JSON_PROPERTY_OWNER_USERNAMES = "ownerUsernames";
private List ownerUsernames;
public static final String JSON_PROPERTY_PROJECT_ID = "projectId";
private String projectId;
public static final String JSON_PROPERTY_READ_WRITE_SNAPSHOT_ID = "readWriteSnapshotId";
private String readWriteSnapshotId;
public static final String JSON_PROPERTY_SIZE_IN_BYTES = "sizeInBytes";
private Long sizeInBytes;
public static final String JSON_PROPERTY_SIZE_STATUS = "sizeStatus";
private DominoDatasetrwApiDatasetRwSizeStatus sizeStatus;
public static final String JSON_PROPERTY_SNAPSHOT_IDS = "snapshotIds";
private List snapshotIds = new ArrayList<>();
public static final String JSON_PROPERTY_STATUS_LAST_UPDATED_BY = "statusLastUpdatedBy";
private String statusLastUpdatedBy;
public static final String JSON_PROPERTY_STATUS_LAST_UPDATED_TIME = "statusLastUpdatedTime";
private Long statusLastUpdatedTime;
public static final String JSON_PROPERTY_TAGS = "tags";
private Map tags = new HashMap<>();
public DominoDatasetrwApiDatasetRwDto() {
}
public DominoDatasetrwApiDatasetRwDto activeROSnapshotCount(Integer activeROSnapshotCount) {
this.activeROSnapshotCount = activeROSnapshotCount;
return this;
}
/**
* Get activeROSnapshotCount
* @return activeROSnapshotCount
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_ACTIVE_R_O_SNAPSHOT_COUNT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getActiveROSnapshotCount() {
return activeROSnapshotCount;
}
@JsonProperty(JSON_PROPERTY_ACTIVE_R_O_SNAPSHOT_COUNT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setActiveROSnapshotCount(Integer activeROSnapshotCount) {
this.activeROSnapshotCount = activeROSnapshotCount;
}
public DominoDatasetrwApiDatasetRwDto author(String author) {
this.author = author;
return this;
}
/**
* Get author
* @return author
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_AUTHOR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getAuthor() {
return author;
}
@JsonProperty(JSON_PROPERTY_AUTHOR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAuthor(String author) {
this.author = author;
}
public DominoDatasetrwApiDatasetRwDto createdTime(Long createdTime) {
this.createdTime = createdTime;
return this;
}
/**
* Get createdTime
* @return createdTime
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_CREATED_TIME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Long getCreatedTime() {
return createdTime;
}
@JsonProperty(JSON_PROPERTY_CREATED_TIME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setCreatedTime(Long createdTime) {
this.createdTime = createdTime;
}
public DominoDatasetrwApiDatasetRwDto dataPlaneIds(List dataPlaneIds) {
this.dataPlaneIds = dataPlaneIds;
return this;
}
public DominoDatasetrwApiDatasetRwDto addDataPlaneIdsItem(String dataPlaneIdsItem) {
if (this.dataPlaneIds == null) {
this.dataPlaneIds = new ArrayList<>();
}
this.dataPlaneIds.add(dataPlaneIdsItem);
return this;
}
/**
* Get dataPlaneIds
* @return dataPlaneIds
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DATA_PLANE_IDS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getDataPlaneIds() {
return dataPlaneIds;
}
@JsonProperty(JSON_PROPERTY_DATA_PLANE_IDS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDataPlaneIds(List dataPlaneIds) {
this.dataPlaneIds = dataPlaneIds;
}
public DominoDatasetrwApiDatasetRwDto datasetPath(String datasetPath) {
this.datasetPath = datasetPath;
return this;
}
/**
* Get datasetPath
* @return datasetPath
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_DATASET_PATH)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getDatasetPath() {
return datasetPath;
}
@JsonProperty(JSON_PROPERTY_DATASET_PATH)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDatasetPath(String datasetPath) {
this.datasetPath = datasetPath;
}
public DominoDatasetrwApiDatasetRwDto description(String description) {
this.description = description;
return this;
}
/**
* Get description
* @return description
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDescription() {
return description;
}
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDescription(String description) {
this.description = description;
}
public DominoDatasetrwApiDatasetRwDto id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getId() {
return id;
}
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setId(String id) {
this.id = id;
}
public DominoDatasetrwApiDatasetRwDto lifecycleStatus(LifecycleStatusEnum lifecycleStatus) {
this.lifecycleStatus = lifecycleStatus;
return this;
}
/**
* Get lifecycleStatus
* @return lifecycleStatus
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_LIFECYCLE_STATUS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public LifecycleStatusEnum getLifecycleStatus() {
return lifecycleStatus;
}
@JsonProperty(JSON_PROPERTY_LIFECYCLE_STATUS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setLifecycleStatus(LifecycleStatusEnum lifecycleStatus) {
this.lifecycleStatus = lifecycleStatus;
}
public DominoDatasetrwApiDatasetRwDto name(String name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getName() {
return name;
}
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setName(String name) {
this.name = name;
}
public DominoDatasetrwApiDatasetRwDto ownerUsernames(List ownerUsernames) {
this.ownerUsernames = ownerUsernames;
return this;
}
public DominoDatasetrwApiDatasetRwDto addOwnerUsernamesItem(String ownerUsernamesItem) {
if (this.ownerUsernames == null) {
this.ownerUsernames = new ArrayList<>();
}
this.ownerUsernames.add(ownerUsernamesItem);
return this;
}
/**
* Get ownerUsernames
* @return ownerUsernames
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_OWNER_USERNAMES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getOwnerUsernames() {
return ownerUsernames;
}
@JsonProperty(JSON_PROPERTY_OWNER_USERNAMES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setOwnerUsernames(List ownerUsernames) {
this.ownerUsernames = ownerUsernames;
}
public DominoDatasetrwApiDatasetRwDto projectId(String projectId) {
this.projectId = projectId;
return this;
}
/**
* Get projectId
* @return projectId
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_PROJECT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getProjectId() {
return projectId;
}
@JsonProperty(JSON_PROPERTY_PROJECT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setProjectId(String projectId) {
this.projectId = projectId;
}
public DominoDatasetrwApiDatasetRwDto readWriteSnapshotId(String readWriteSnapshotId) {
this.readWriteSnapshotId = readWriteSnapshotId;
return this;
}
/**
* Get readWriteSnapshotId
* @return readWriteSnapshotId
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_READ_WRITE_SNAPSHOT_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getReadWriteSnapshotId() {
return readWriteSnapshotId;
}
@JsonProperty(JSON_PROPERTY_READ_WRITE_SNAPSHOT_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setReadWriteSnapshotId(String readWriteSnapshotId) {
this.readWriteSnapshotId = readWriteSnapshotId;
}
public DominoDatasetrwApiDatasetRwDto sizeInBytes(Long sizeInBytes) {
this.sizeInBytes = sizeInBytes;
return this;
}
/**
* Get sizeInBytes
* @return sizeInBytes
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_SIZE_IN_BYTES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getSizeInBytes() {
return sizeInBytes;
}
@JsonProperty(JSON_PROPERTY_SIZE_IN_BYTES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSizeInBytes(Long sizeInBytes) {
this.sizeInBytes = sizeInBytes;
}
public DominoDatasetrwApiDatasetRwDto sizeStatus(DominoDatasetrwApiDatasetRwSizeStatus sizeStatus) {
this.sizeStatus = sizeStatus;
return this;
}
/**
* Get sizeStatus
* @return sizeStatus
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_SIZE_STATUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DominoDatasetrwApiDatasetRwSizeStatus getSizeStatus() {
return sizeStatus;
}
@JsonProperty(JSON_PROPERTY_SIZE_STATUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSizeStatus(DominoDatasetrwApiDatasetRwSizeStatus sizeStatus) {
this.sizeStatus = sizeStatus;
}
public DominoDatasetrwApiDatasetRwDto snapshotIds(List snapshotIds) {
this.snapshotIds = snapshotIds;
return this;
}
public DominoDatasetrwApiDatasetRwDto addSnapshotIdsItem(String snapshotIdsItem) {
if (this.snapshotIds == null) {
this.snapshotIds = new ArrayList<>();
}
this.snapshotIds.add(snapshotIdsItem);
return this;
}
/**
* Get snapshotIds
* @return snapshotIds
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_SNAPSHOT_IDS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getSnapshotIds() {
return snapshotIds;
}
@JsonProperty(JSON_PROPERTY_SNAPSHOT_IDS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setSnapshotIds(List snapshotIds) {
this.snapshotIds = snapshotIds;
}
public DominoDatasetrwApiDatasetRwDto statusLastUpdatedBy(String statusLastUpdatedBy) {
this.statusLastUpdatedBy = statusLastUpdatedBy;
return this;
}
/**
* Get statusLastUpdatedBy
* @return statusLastUpdatedBy
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_STATUS_LAST_UPDATED_BY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getStatusLastUpdatedBy() {
return statusLastUpdatedBy;
}
@JsonProperty(JSON_PROPERTY_STATUS_LAST_UPDATED_BY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setStatusLastUpdatedBy(String statusLastUpdatedBy) {
this.statusLastUpdatedBy = statusLastUpdatedBy;
}
public DominoDatasetrwApiDatasetRwDto statusLastUpdatedTime(Long statusLastUpdatedTime) {
this.statusLastUpdatedTime = statusLastUpdatedTime;
return this;
}
/**
* Get statusLastUpdatedTime
* @return statusLastUpdatedTime
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_STATUS_LAST_UPDATED_TIME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Long getStatusLastUpdatedTime() {
return statusLastUpdatedTime;
}
@JsonProperty(JSON_PROPERTY_STATUS_LAST_UPDATED_TIME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setStatusLastUpdatedTime(Long statusLastUpdatedTime) {
this.statusLastUpdatedTime = statusLastUpdatedTime;
}
public DominoDatasetrwApiDatasetRwDto tags(Map tags) {
this.tags = tags;
return this;
}
public DominoDatasetrwApiDatasetRwDto putTagsItem(String key, String tagsItem) {
if (this.tags == null) {
this.tags = new HashMap<>();
}
this.tags.put(key, tagsItem);
return this;
}
/**
* Get tags
* @return tags
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_TAGS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Map getTags() {
return tags;
}
@JsonProperty(JSON_PROPERTY_TAGS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setTags(Map tags) {
this.tags = tags;
}
/**
* Return true if this domino.datasetrw.api.DatasetRwDto object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DominoDatasetrwApiDatasetRwDto dominoDatasetrwApiDatasetRwDto = (DominoDatasetrwApiDatasetRwDto) o;
return Objects.equals(this.activeROSnapshotCount, dominoDatasetrwApiDatasetRwDto.activeROSnapshotCount) &&
Objects.equals(this.author, dominoDatasetrwApiDatasetRwDto.author) &&
Objects.equals(this.createdTime, dominoDatasetrwApiDatasetRwDto.createdTime) &&
Objects.equals(this.dataPlaneIds, dominoDatasetrwApiDatasetRwDto.dataPlaneIds) &&
Objects.equals(this.datasetPath, dominoDatasetrwApiDatasetRwDto.datasetPath) &&
Objects.equals(this.description, dominoDatasetrwApiDatasetRwDto.description) &&
Objects.equals(this.id, dominoDatasetrwApiDatasetRwDto.id) &&
Objects.equals(this.lifecycleStatus, dominoDatasetrwApiDatasetRwDto.lifecycleStatus) &&
Objects.equals(this.name, dominoDatasetrwApiDatasetRwDto.name) &&
Objects.equals(this.ownerUsernames, dominoDatasetrwApiDatasetRwDto.ownerUsernames) &&
Objects.equals(this.projectId, dominoDatasetrwApiDatasetRwDto.projectId) &&
Objects.equals(this.readWriteSnapshotId, dominoDatasetrwApiDatasetRwDto.readWriteSnapshotId) &&
Objects.equals(this.sizeInBytes, dominoDatasetrwApiDatasetRwDto.sizeInBytes) &&
Objects.equals(this.sizeStatus, dominoDatasetrwApiDatasetRwDto.sizeStatus) &&
Objects.equals(this.snapshotIds, dominoDatasetrwApiDatasetRwDto.snapshotIds) &&
Objects.equals(this.statusLastUpdatedBy, dominoDatasetrwApiDatasetRwDto.statusLastUpdatedBy) &&
Objects.equals(this.statusLastUpdatedTime, dominoDatasetrwApiDatasetRwDto.statusLastUpdatedTime) &&
Objects.equals(this.tags, dominoDatasetrwApiDatasetRwDto.tags);
}
@Override
public int hashCode() {
return Objects.hash(activeROSnapshotCount, author, createdTime, dataPlaneIds, datasetPath, description, id, lifecycleStatus, name, ownerUsernames, projectId, readWriteSnapshotId, sizeInBytes, sizeStatus, snapshotIds, statusLastUpdatedBy, statusLastUpdatedTime, tags);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DominoDatasetrwApiDatasetRwDto {\n");
sb.append(" activeROSnapshotCount: ").append(toIndentedString(activeROSnapshotCount)).append("\n");
sb.append(" author: ").append(toIndentedString(author)).append("\n");
sb.append(" createdTime: ").append(toIndentedString(createdTime)).append("\n");
sb.append(" dataPlaneIds: ").append(toIndentedString(dataPlaneIds)).append("\n");
sb.append(" datasetPath: ").append(toIndentedString(datasetPath)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" lifecycleStatus: ").append(toIndentedString(lifecycleStatus)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" ownerUsernames: ").append(toIndentedString(ownerUsernames)).append("\n");
sb.append(" projectId: ").append(toIndentedString(projectId)).append("\n");
sb.append(" readWriteSnapshotId: ").append(toIndentedString(readWriteSnapshotId)).append("\n");
sb.append(" sizeInBytes: ").append(toIndentedString(sizeInBytes)).append("\n");
sb.append(" sizeStatus: ").append(toIndentedString(sizeStatus)).append("\n");
sb.append(" snapshotIds: ").append(toIndentedString(snapshotIds)).append("\n");
sb.append(" statusLastUpdatedBy: ").append(toIndentedString(statusLastUpdatedBy)).append("\n");
sb.append(" statusLastUpdatedTime: ").append(toIndentedString(statusLastUpdatedTime)).append("\n");
sb.append(" tags: ").append(toIndentedString(tags)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `activeROSnapshotCount` to the URL query string
if (getActiveROSnapshotCount() != null) {
joiner.add(String.format("%sactiveROSnapshotCount%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getActiveROSnapshotCount()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `author` to the URL query string
if (getAuthor() != null) {
joiner.add(String.format("%sauthor%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getAuthor()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `createdTime` to the URL query string
if (getCreatedTime() != null) {
joiner.add(String.format("%screatedTime%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getCreatedTime()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `dataPlaneIds` to the URL query string
if (getDataPlaneIds() != null) {
for (int i = 0; i < getDataPlaneIds().size(); i++) {
joiner.add(String.format("%sdataPlaneIds%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(ApiClient.valueToString(getDataPlaneIds().get(i)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
// add `datasetPath` to the URL query string
if (getDatasetPath() != null) {
joiner.add(String.format("%sdatasetPath%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getDatasetPath()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `description` to the URL query string
if (getDescription() != null) {
joiner.add(String.format("%sdescription%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getDescription()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `id` to the URL query string
if (getId() != null) {
joiner.add(String.format("%sid%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `lifecycleStatus` to the URL query string
if (getLifecycleStatus() != null) {
joiner.add(String.format("%slifecycleStatus%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getLifecycleStatus()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `name` to the URL query string
if (getName() != null) {
joiner.add(String.format("%sname%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getName()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `ownerUsernames` to the URL query string
if (getOwnerUsernames() != null) {
for (int i = 0; i < getOwnerUsernames().size(); i++) {
joiner.add(String.format("%sownerUsernames%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(ApiClient.valueToString(getOwnerUsernames().get(i)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
// add `projectId` to the URL query string
if (getProjectId() != null) {
joiner.add(String.format("%sprojectId%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getProjectId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `readWriteSnapshotId` to the URL query string
if (getReadWriteSnapshotId() != null) {
joiner.add(String.format("%sreadWriteSnapshotId%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getReadWriteSnapshotId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `sizeInBytes` to the URL query string
if (getSizeInBytes() != null) {
joiner.add(String.format("%ssizeInBytes%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getSizeInBytes()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `sizeStatus` to the URL query string
if (getSizeStatus() != null) {
joiner.add(String.format("%ssizeStatus%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getSizeStatus()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `snapshotIds` to the URL query string
if (getSnapshotIds() != null) {
for (int i = 0; i < getSnapshotIds().size(); i++) {
joiner.add(String.format("%ssnapshotIds%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(ApiClient.valueToString(getSnapshotIds().get(i)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
// add `statusLastUpdatedBy` to the URL query string
if (getStatusLastUpdatedBy() != null) {
joiner.add(String.format("%sstatusLastUpdatedBy%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getStatusLastUpdatedBy()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `statusLastUpdatedTime` to the URL query string
if (getStatusLastUpdatedTime() != null) {
joiner.add(String.format("%sstatusLastUpdatedTime%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getStatusLastUpdatedTime()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `tags` to the URL query string
if (getTags() != null) {
for (String _key : getTags().keySet()) {
joiner.add(String.format("%stags%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, _key, containerSuffix),
getTags().get(_key), URLEncoder.encode(ApiClient.valueToString(getTags().get(_key)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy