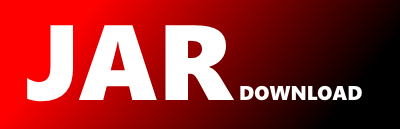
com.dominodatalab.api.model.DominoDatasetrwApiDatasetRwProjectMountDto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of domino-java-client Show documentation
Show all versions of domino-java-client Show documentation
Domino Data Lab API Client to connect to Domino web services using Java HTTP Client.
/*
* Domino Data Lab API v4
* This API provides access to select Domino functions available in Domino's non-public API. To authenticate your requests, include your API Key (which you can find on your account page) with the header X-Domino-Api-Key.
*
* The version of the OpenAPI document: 4.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.api.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.dominodatalab.api.model.DominoDataplaneDataPlaneDto;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.dominodatalab.api.invoker.ApiClient;
/**
* DominoDatasetrwApiDatasetRwProjectMountDto
*/
@JsonPropertyOrder({
DominoDatasetrwApiDatasetRwProjectMountDto.JSON_PROPERTY_AVAILABLE_VERSIONS,
DominoDatasetrwApiDatasetRwProjectMountDto.JSON_PROPERTY_DATA_PLANES,
DominoDatasetrwApiDatasetRwProjectMountDto.JSON_PROPERTY_DATASET_ID,
DominoDatasetrwApiDatasetRwProjectMountDto.JSON_PROPERTY_DESCRIPTION,
DominoDatasetrwApiDatasetRwProjectMountDto.JSON_PROPERTY_IS_PARTIAL_SIZE,
DominoDatasetrwApiDatasetRwProjectMountDto.JSON_PROPERTY_MOUNT_PATHS_FOR_PROJECT,
DominoDatasetrwApiDatasetRwProjectMountDto.JSON_PROPERTY_NAME,
DominoDatasetrwApiDatasetRwProjectMountDto.JSON_PROPERTY_OWNER_PROJECT_ID,
DominoDatasetrwApiDatasetRwProjectMountDto.JSON_PROPERTY_OWNER_PROJECT_NAME,
DominoDatasetrwApiDatasetRwProjectMountDto.JSON_PROPERTY_OWNER_PROJECT_OWNER_USERNAME,
DominoDatasetrwApiDatasetRwProjectMountDto.JSON_PROPERTY_OWNER_USERNAMES,
DominoDatasetrwApiDatasetRwProjectMountDto.JSON_PROPERTY_SIZE_IN_BYTES,
DominoDatasetrwApiDatasetRwProjectMountDto.JSON_PROPERTY_SIZE_STATUS,
DominoDatasetrwApiDatasetRwProjectMountDto.JSON_PROPERTY_SNAPSHOT_ID,
DominoDatasetrwApiDatasetRwProjectMountDto.JSON_PROPERTY_STORAGE_SIZE,
DominoDatasetrwApiDatasetRwProjectMountDto.JSON_PROPERTY_VERSION_NUMBER
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-10-04T16:37:26.309454-04:00[America/New_York]", comments = "Generator version: 7.8.0")
public class DominoDatasetrwApiDatasetRwProjectMountDto {
public static final String JSON_PROPERTY_AVAILABLE_VERSIONS = "availableVersions";
private Integer availableVersions;
public static final String JSON_PROPERTY_DATA_PLANES = "dataPlanes";
private List dataPlanes = new ArrayList<>();
public static final String JSON_PROPERTY_DATASET_ID = "datasetId";
private String datasetId;
public static final String JSON_PROPERTY_DESCRIPTION = "description";
private String description;
public static final String JSON_PROPERTY_IS_PARTIAL_SIZE = "isPartialSize";
private Boolean isPartialSize;
public static final String JSON_PROPERTY_MOUNT_PATHS_FOR_PROJECT = "mountPathsForProject";
private List mountPathsForProject = new ArrayList<>();
public static final String JSON_PROPERTY_NAME = "name";
private String name;
public static final String JSON_PROPERTY_OWNER_PROJECT_ID = "ownerProjectId";
private String ownerProjectId;
public static final String JSON_PROPERTY_OWNER_PROJECT_NAME = "ownerProjectName";
private String ownerProjectName;
public static final String JSON_PROPERTY_OWNER_PROJECT_OWNER_USERNAME = "ownerProjectOwnerUsername";
private String ownerProjectOwnerUsername;
public static final String JSON_PROPERTY_OWNER_USERNAMES = "ownerUsernames";
private List ownerUsernames;
public static final String JSON_PROPERTY_SIZE_IN_BYTES = "sizeInBytes";
private Long sizeInBytes;
/**
* Gets or Sets sizeStatus
*/
public enum SizeStatusEnum {
ACTIVE("Active"),
PENDING("Pending");
private String value;
SizeStatusEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static SizeStatusEnum fromValue(String value) {
for (SizeStatusEnum b : SizeStatusEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return null;
}
}
public static final String JSON_PROPERTY_SIZE_STATUS = "sizeStatus";
private SizeStatusEnum sizeStatus;
public static final String JSON_PROPERTY_SNAPSHOT_ID = "snapshotId";
private String snapshotId;
public static final String JSON_PROPERTY_STORAGE_SIZE = "storageSize";
private Long storageSize;
public static final String JSON_PROPERTY_VERSION_NUMBER = "versionNumber";
private Integer versionNumber;
public DominoDatasetrwApiDatasetRwProjectMountDto() {
}
public DominoDatasetrwApiDatasetRwProjectMountDto availableVersions(Integer availableVersions) {
this.availableVersions = availableVersions;
return this;
}
/**
* Get availableVersions
* @return availableVersions
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_AVAILABLE_VERSIONS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Integer getAvailableVersions() {
return availableVersions;
}
@JsonProperty(JSON_PROPERTY_AVAILABLE_VERSIONS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setAvailableVersions(Integer availableVersions) {
this.availableVersions = availableVersions;
}
public DominoDatasetrwApiDatasetRwProjectMountDto dataPlanes(List dataPlanes) {
this.dataPlanes = dataPlanes;
return this;
}
public DominoDatasetrwApiDatasetRwProjectMountDto addDataPlanesItem(DominoDataplaneDataPlaneDto dataPlanesItem) {
if (this.dataPlanes == null) {
this.dataPlanes = new ArrayList<>();
}
this.dataPlanes.add(dataPlanesItem);
return this;
}
/**
* Get dataPlanes
* @return dataPlanes
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_DATA_PLANES)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getDataPlanes() {
return dataPlanes;
}
@JsonProperty(JSON_PROPERTY_DATA_PLANES)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDataPlanes(List dataPlanes) {
this.dataPlanes = dataPlanes;
}
public DominoDatasetrwApiDatasetRwProjectMountDto datasetId(String datasetId) {
this.datasetId = datasetId;
return this;
}
/**
* Get datasetId
* @return datasetId
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_DATASET_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getDatasetId() {
return datasetId;
}
@JsonProperty(JSON_PROPERTY_DATASET_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDatasetId(String datasetId) {
this.datasetId = datasetId;
}
public DominoDatasetrwApiDatasetRwProjectMountDto description(String description) {
this.description = description;
return this;
}
/**
* Get description
* @return description
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDescription() {
return description;
}
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDescription(String description) {
this.description = description;
}
public DominoDatasetrwApiDatasetRwProjectMountDto isPartialSize(Boolean isPartialSize) {
this.isPartialSize = isPartialSize;
return this;
}
/**
* Get isPartialSize
* @return isPartialSize
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_IS_PARTIAL_SIZE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getIsPartialSize() {
return isPartialSize;
}
@JsonProperty(JSON_PROPERTY_IS_PARTIAL_SIZE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setIsPartialSize(Boolean isPartialSize) {
this.isPartialSize = isPartialSize;
}
public DominoDatasetrwApiDatasetRwProjectMountDto mountPathsForProject(List mountPathsForProject) {
this.mountPathsForProject = mountPathsForProject;
return this;
}
public DominoDatasetrwApiDatasetRwProjectMountDto addMountPathsForProjectItem(String mountPathsForProjectItem) {
if (this.mountPathsForProject == null) {
this.mountPathsForProject = new ArrayList<>();
}
this.mountPathsForProject.add(mountPathsForProjectItem);
return this;
}
/**
* Get mountPathsForProject
* @return mountPathsForProject
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_MOUNT_PATHS_FOR_PROJECT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getMountPathsForProject() {
return mountPathsForProject;
}
@JsonProperty(JSON_PROPERTY_MOUNT_PATHS_FOR_PROJECT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setMountPathsForProject(List mountPathsForProject) {
this.mountPathsForProject = mountPathsForProject;
}
public DominoDatasetrwApiDatasetRwProjectMountDto name(String name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getName() {
return name;
}
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setName(String name) {
this.name = name;
}
public DominoDatasetrwApiDatasetRwProjectMountDto ownerProjectId(String ownerProjectId) {
this.ownerProjectId = ownerProjectId;
return this;
}
/**
* Get ownerProjectId
* @return ownerProjectId
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_OWNER_PROJECT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getOwnerProjectId() {
return ownerProjectId;
}
@JsonProperty(JSON_PROPERTY_OWNER_PROJECT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setOwnerProjectId(String ownerProjectId) {
this.ownerProjectId = ownerProjectId;
}
public DominoDatasetrwApiDatasetRwProjectMountDto ownerProjectName(String ownerProjectName) {
this.ownerProjectName = ownerProjectName;
return this;
}
/**
* Get ownerProjectName
* @return ownerProjectName
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_OWNER_PROJECT_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getOwnerProjectName() {
return ownerProjectName;
}
@JsonProperty(JSON_PROPERTY_OWNER_PROJECT_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setOwnerProjectName(String ownerProjectName) {
this.ownerProjectName = ownerProjectName;
}
public DominoDatasetrwApiDatasetRwProjectMountDto ownerProjectOwnerUsername(String ownerProjectOwnerUsername) {
this.ownerProjectOwnerUsername = ownerProjectOwnerUsername;
return this;
}
/**
* Get ownerProjectOwnerUsername
* @return ownerProjectOwnerUsername
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_OWNER_PROJECT_OWNER_USERNAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getOwnerProjectOwnerUsername() {
return ownerProjectOwnerUsername;
}
@JsonProperty(JSON_PROPERTY_OWNER_PROJECT_OWNER_USERNAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setOwnerProjectOwnerUsername(String ownerProjectOwnerUsername) {
this.ownerProjectOwnerUsername = ownerProjectOwnerUsername;
}
public DominoDatasetrwApiDatasetRwProjectMountDto ownerUsernames(List ownerUsernames) {
this.ownerUsernames = ownerUsernames;
return this;
}
public DominoDatasetrwApiDatasetRwProjectMountDto addOwnerUsernamesItem(String ownerUsernamesItem) {
if (this.ownerUsernames == null) {
this.ownerUsernames = new ArrayList<>();
}
this.ownerUsernames.add(ownerUsernamesItem);
return this;
}
/**
* Get ownerUsernames
* @return ownerUsernames
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_OWNER_USERNAMES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getOwnerUsernames() {
return ownerUsernames;
}
@JsonProperty(JSON_PROPERTY_OWNER_USERNAMES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setOwnerUsernames(List ownerUsernames) {
this.ownerUsernames = ownerUsernames;
}
public DominoDatasetrwApiDatasetRwProjectMountDto sizeInBytes(Long sizeInBytes) {
this.sizeInBytes = sizeInBytes;
return this;
}
/**
* Get sizeInBytes
* @return sizeInBytes
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_SIZE_IN_BYTES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getSizeInBytes() {
return sizeInBytes;
}
@JsonProperty(JSON_PROPERTY_SIZE_IN_BYTES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSizeInBytes(Long sizeInBytes) {
this.sizeInBytes = sizeInBytes;
}
public DominoDatasetrwApiDatasetRwProjectMountDto sizeStatus(SizeStatusEnum sizeStatus) {
this.sizeStatus = sizeStatus;
return this;
}
/**
* Get sizeStatus
* @return sizeStatus
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_SIZE_STATUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public SizeStatusEnum getSizeStatus() {
return sizeStatus;
}
@JsonProperty(JSON_PROPERTY_SIZE_STATUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSizeStatus(SizeStatusEnum sizeStatus) {
this.sizeStatus = sizeStatus;
}
public DominoDatasetrwApiDatasetRwProjectMountDto snapshotId(String snapshotId) {
this.snapshotId = snapshotId;
return this;
}
/**
* Get snapshotId
* @return snapshotId
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_SNAPSHOT_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getSnapshotId() {
return snapshotId;
}
@JsonProperty(JSON_PROPERTY_SNAPSHOT_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setSnapshotId(String snapshotId) {
this.snapshotId = snapshotId;
}
public DominoDatasetrwApiDatasetRwProjectMountDto storageSize(Long storageSize) {
this.storageSize = storageSize;
return this;
}
/**
* Get storageSize
* @return storageSize
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_STORAGE_SIZE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getStorageSize() {
return storageSize;
}
@JsonProperty(JSON_PROPERTY_STORAGE_SIZE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setStorageSize(Long storageSize) {
this.storageSize = storageSize;
}
public DominoDatasetrwApiDatasetRwProjectMountDto versionNumber(Integer versionNumber) {
this.versionNumber = versionNumber;
return this;
}
/**
* Get versionNumber
* @return versionNumber
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_VERSION_NUMBER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getVersionNumber() {
return versionNumber;
}
@JsonProperty(JSON_PROPERTY_VERSION_NUMBER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setVersionNumber(Integer versionNumber) {
this.versionNumber = versionNumber;
}
/**
* Return true if this domino.datasetrw.api.DatasetRwProjectMountDto object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DominoDatasetrwApiDatasetRwProjectMountDto dominoDatasetrwApiDatasetRwProjectMountDto = (DominoDatasetrwApiDatasetRwProjectMountDto) o;
return Objects.equals(this.availableVersions, dominoDatasetrwApiDatasetRwProjectMountDto.availableVersions) &&
Objects.equals(this.dataPlanes, dominoDatasetrwApiDatasetRwProjectMountDto.dataPlanes) &&
Objects.equals(this.datasetId, dominoDatasetrwApiDatasetRwProjectMountDto.datasetId) &&
Objects.equals(this.description, dominoDatasetrwApiDatasetRwProjectMountDto.description) &&
Objects.equals(this.isPartialSize, dominoDatasetrwApiDatasetRwProjectMountDto.isPartialSize) &&
Objects.equals(this.mountPathsForProject, dominoDatasetrwApiDatasetRwProjectMountDto.mountPathsForProject) &&
Objects.equals(this.name, dominoDatasetrwApiDatasetRwProjectMountDto.name) &&
Objects.equals(this.ownerProjectId, dominoDatasetrwApiDatasetRwProjectMountDto.ownerProjectId) &&
Objects.equals(this.ownerProjectName, dominoDatasetrwApiDatasetRwProjectMountDto.ownerProjectName) &&
Objects.equals(this.ownerProjectOwnerUsername, dominoDatasetrwApiDatasetRwProjectMountDto.ownerProjectOwnerUsername) &&
Objects.equals(this.ownerUsernames, dominoDatasetrwApiDatasetRwProjectMountDto.ownerUsernames) &&
Objects.equals(this.sizeInBytes, dominoDatasetrwApiDatasetRwProjectMountDto.sizeInBytes) &&
Objects.equals(this.sizeStatus, dominoDatasetrwApiDatasetRwProjectMountDto.sizeStatus) &&
Objects.equals(this.snapshotId, dominoDatasetrwApiDatasetRwProjectMountDto.snapshotId) &&
Objects.equals(this.storageSize, dominoDatasetrwApiDatasetRwProjectMountDto.storageSize) &&
Objects.equals(this.versionNumber, dominoDatasetrwApiDatasetRwProjectMountDto.versionNumber);
}
@Override
public int hashCode() {
return Objects.hash(availableVersions, dataPlanes, datasetId, description, isPartialSize, mountPathsForProject, name, ownerProjectId, ownerProjectName, ownerProjectOwnerUsername, ownerUsernames, sizeInBytes, sizeStatus, snapshotId, storageSize, versionNumber);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DominoDatasetrwApiDatasetRwProjectMountDto {\n");
sb.append(" availableVersions: ").append(toIndentedString(availableVersions)).append("\n");
sb.append(" dataPlanes: ").append(toIndentedString(dataPlanes)).append("\n");
sb.append(" datasetId: ").append(toIndentedString(datasetId)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" isPartialSize: ").append(toIndentedString(isPartialSize)).append("\n");
sb.append(" mountPathsForProject: ").append(toIndentedString(mountPathsForProject)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" ownerProjectId: ").append(toIndentedString(ownerProjectId)).append("\n");
sb.append(" ownerProjectName: ").append(toIndentedString(ownerProjectName)).append("\n");
sb.append(" ownerProjectOwnerUsername: ").append(toIndentedString(ownerProjectOwnerUsername)).append("\n");
sb.append(" ownerUsernames: ").append(toIndentedString(ownerUsernames)).append("\n");
sb.append(" sizeInBytes: ").append(toIndentedString(sizeInBytes)).append("\n");
sb.append(" sizeStatus: ").append(toIndentedString(sizeStatus)).append("\n");
sb.append(" snapshotId: ").append(toIndentedString(snapshotId)).append("\n");
sb.append(" storageSize: ").append(toIndentedString(storageSize)).append("\n");
sb.append(" versionNumber: ").append(toIndentedString(versionNumber)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `availableVersions` to the URL query string
if (getAvailableVersions() != null) {
joiner.add(String.format("%savailableVersions%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getAvailableVersions()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `dataPlanes` to the URL query string
if (getDataPlanes() != null) {
for (int i = 0; i < getDataPlanes().size(); i++) {
if (getDataPlanes().get(i) != null) {
joiner.add(getDataPlanes().get(i).toUrlQueryString(String.format("%sdataPlanes%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `datasetId` to the URL query string
if (getDatasetId() != null) {
joiner.add(String.format("%sdatasetId%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getDatasetId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `description` to the URL query string
if (getDescription() != null) {
joiner.add(String.format("%sdescription%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getDescription()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `isPartialSize` to the URL query string
if (getIsPartialSize() != null) {
joiner.add(String.format("%sisPartialSize%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getIsPartialSize()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `mountPathsForProject` to the URL query string
if (getMountPathsForProject() != null) {
for (int i = 0; i < getMountPathsForProject().size(); i++) {
joiner.add(String.format("%smountPathsForProject%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(ApiClient.valueToString(getMountPathsForProject().get(i)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
// add `name` to the URL query string
if (getName() != null) {
joiner.add(String.format("%sname%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getName()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `ownerProjectId` to the URL query string
if (getOwnerProjectId() != null) {
joiner.add(String.format("%sownerProjectId%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getOwnerProjectId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `ownerProjectName` to the URL query string
if (getOwnerProjectName() != null) {
joiner.add(String.format("%sownerProjectName%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getOwnerProjectName()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `ownerProjectOwnerUsername` to the URL query string
if (getOwnerProjectOwnerUsername() != null) {
joiner.add(String.format("%sownerProjectOwnerUsername%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getOwnerProjectOwnerUsername()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `ownerUsernames` to the URL query string
if (getOwnerUsernames() != null) {
for (int i = 0; i < getOwnerUsernames().size(); i++) {
joiner.add(String.format("%sownerUsernames%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(ApiClient.valueToString(getOwnerUsernames().get(i)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
// add `sizeInBytes` to the URL query string
if (getSizeInBytes() != null) {
joiner.add(String.format("%ssizeInBytes%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getSizeInBytes()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `sizeStatus` to the URL query string
if (getSizeStatus() != null) {
joiner.add(String.format("%ssizeStatus%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getSizeStatus()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `snapshotId` to the URL query string
if (getSnapshotId() != null) {
joiner.add(String.format("%ssnapshotId%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getSnapshotId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `storageSize` to the URL query string
if (getStorageSize() != null) {
joiner.add(String.format("%sstorageSize%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getStorageSize()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `versionNumber` to the URL query string
if (getVersionNumber() != null) {
joiner.add(String.format("%sversionNumber%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getVersionNumber()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy