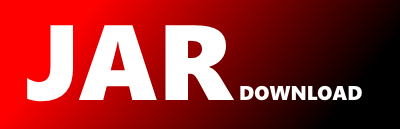
com.dominodatalab.api.model.DominoDatasourceApiDataSourceDto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of domino-java-client Show documentation
Show all versions of domino-java-client Show documentation
Domino Data Lab API Client to connect to Domino web services using Java HTTP Client.
/*
* Domino Data Lab API v4
* This API provides access to select Domino functions available in Domino's non-public API. To authenticate your requests, include your API Key (which you can find on your account page) with the header X-Domino-Api-Key.
*
* The version of the OpenAPI document: 4.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.api.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.dominodatalab.api.model.DominoDatasourceApiDataSourceAdminInfoDto;
import com.dominodatalab.api.model.DominoDatasourceApiDataSourceDataPlaneInfo;
import com.dominodatalab.api.model.DominoDatasourceApiDataSourceOwnerInfoDto;
import com.dominodatalab.api.model.DominoDatasourceApiDataSourcePermissionsDto;
import com.dominodatalab.api.model.DominoDatasourceApiEngineInfoDto;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.dominodatalab.api.invoker.ApiClient;
/**
* DominoDatasourceApiDataSourceDto
*/
@JsonPropertyOrder({
DominoDatasourceApiDataSourceDto.JSON_PROPERTY_ADDED_BY,
DominoDatasourceApiDataSourceDto.JSON_PROPERTY_ADDED_TO_PROJECT_TIME_MAP,
DominoDatasourceApiDataSourceDto.JSON_PROPERTY_ADMIN_INFO,
DominoDatasourceApiDataSourceDto.JSON_PROPERTY_AUTH_TYPE,
DominoDatasourceApiDataSourceDto.JSON_PROPERTY_CONFIG,
DominoDatasourceApiDataSourceDto.JSON_PROPERTY_DATA_PLANES,
DominoDatasourceApiDataSourceDto.JSON_PROPERTY_DATA_SOURCE_PERMISSIONS,
DominoDatasourceApiDataSourceDto.JSON_PROPERTY_DATA_SOURCE_TYPE,
DominoDatasourceApiDataSourceDto.JSON_PROPERTY_DESCRIPTION,
DominoDatasourceApiDataSourceDto.JSON_PROPERTY_DISPLAY_NAME,
DominoDatasourceApiDataSourceDto.JSON_PROPERTY_ENGINE_INFO,
DominoDatasourceApiDataSourceDto.JSON_PROPERTY_ID,
DominoDatasourceApiDataSourceDto.JSON_PROPERTY_LAST_ACCESSED,
DominoDatasourceApiDataSourceDto.JSON_PROPERTY_LAST_UPDATED,
DominoDatasourceApiDataSourceDto.JSON_PROPERTY_LAST_UPDATED_BY,
DominoDatasourceApiDataSourceDto.JSON_PROPERTY_NAME,
DominoDatasourceApiDataSourceDto.JSON_PROPERTY_OWNER_ID,
DominoDatasourceApiDataSourceDto.JSON_PROPERTY_OWNER_INFO,
DominoDatasourceApiDataSourceDto.JSON_PROPERTY_PROJECT_IDS,
DominoDatasourceApiDataSourceDto.JSON_PROPERTY_STATUS,
DominoDatasourceApiDataSourceDto.JSON_PROPERTY_USE_ALL_DATA_PLANES
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-10-04T16:37:26.309454-04:00[America/New_York]", comments = "Generator version: 7.8.0")
public class DominoDatasourceApiDataSourceDto {
public static final String JSON_PROPERTY_ADDED_BY = "addedBy";
private Map addedBy = new HashMap<>();
public static final String JSON_PROPERTY_ADDED_TO_PROJECT_TIME_MAP = "addedToProjectTimeMap";
private Map addedToProjectTimeMap = new HashMap<>();
public static final String JSON_PROPERTY_ADMIN_INFO = "adminInfo";
private DominoDatasourceApiDataSourceAdminInfoDto adminInfo;
/**
* Gets or Sets authType
*/
public enum AuthTypeEnum {
AZURE_BASIC("AzureBasic"),
BASIC("Basic"),
GCP_BASIC("GCPBasic"),
AWSIAM_BASIC("AWSIAMBasic"),
AWSIAM_BASIC_NO_OVERRIDE("AWSIAMBasicNoOverride"),
AWSIAM_ROLE("AWSIAMRole"),
AWSIAM_ROLE_WITH_USERNAME("AWSIAMRoleWithUsername"),
O_AUTH("OAuth"),
PERSONAL_TOKEN("PersonalToken"),
USER_ONLY("UserOnly"),
BASIC_OPTIONAL("BasicOptional"),
NO_AUTH("NoAuth"),
CLIENT_ID_SECRET("ClientIdSecret"),
O_AUTH_TOKEN("OAuthToken"),
API_KEY("APIKey");
private String value;
AuthTypeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static AuthTypeEnum fromValue(String value) {
for (AuthTypeEnum b : AuthTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_AUTH_TYPE = "authType";
private AuthTypeEnum authType;
public static final String JSON_PROPERTY_CONFIG = "config";
private Map config = new HashMap<>();
public static final String JSON_PROPERTY_DATA_PLANES = "dataPlanes";
private List dataPlanes = new ArrayList<>();
public static final String JSON_PROPERTY_DATA_SOURCE_PERMISSIONS = "dataSourcePermissions";
private DominoDatasourceApiDataSourcePermissionsDto dataSourcePermissions;
/**
* Gets or Sets dataSourceType
*/
public enum DataSourceTypeEnum {
ADLS_CONFIG("ADLSConfig"),
AZURE_BLOB_STORAGE_CONFIG("AzureBlobStorageConfig"),
BIG_QUERY_CONFIG("BigQueryConfig"),
CLICK_HOUSE_CONFIG("ClickHouseConfig"),
DATABRICKS_CONFIG("DatabricksConfig"),
DB2_CONFIG("DB2Config"),
DRUID_CONFIG("DruidConfig"),
GCS_CONFIG("GCSConfig"),
GENERIC_JDBC_CONFIG("GenericJDBCConfig"),
GENERIC_S3_CONFIG("GenericS3Config"),
GREENPLUM_CONFIG("GreenplumConfig"),
IGNITE_CONFIG("IgniteConfig"),
MARIA_DB_CONFIG("MariaDBConfig"),
MONGO_DB_CONFIG("MongoDBConfig"),
MY_SQL_CONFIG("MySQLConfig"),
NETEZZA_CONFIG("NetezzaConfig"),
ORACLE_CONFIG("OracleConfig"),
PALANTIR_CONFIG("PalantirConfig"),
PINECONE_CONFIG("PineconeConfig"),
POSTGRE_SQL_CONFIG("PostgreSQLConfig"),
QDRANT_CONFIG("QdrantConfig"),
REDSHIFT_CONFIG("RedshiftConfig"),
S3_CONFIG("S3Config"),
SAP_HANA_CONFIG("SAPHanaConfig"),
SINGLE_STORE_CONFIG("SingleStoreConfig"),
SQL_SERVER_CONFIG("SQLServerConfig"),
SNOWFLAKE_CONFIG("SnowflakeConfig"),
SYNAPSE_CONFIG("SynapseConfig"),
TABULAR_S3_GLUE_CONFIG("TabularS3GlueConfig"),
TERADATA_CONFIG("TeradataConfig"),
TRINO_CONFIG("TrinoConfig"),
VERTICA_CONFIG("VerticaConfig");
private String value;
DataSourceTypeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static DataSourceTypeEnum fromValue(String value) {
for (DataSourceTypeEnum b : DataSourceTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_DATA_SOURCE_TYPE = "dataSourceType";
private DataSourceTypeEnum dataSourceType;
public static final String JSON_PROPERTY_DESCRIPTION = "description";
private String description;
public static final String JSON_PROPERTY_DISPLAY_NAME = "displayName";
private String displayName;
public static final String JSON_PROPERTY_ENGINE_INFO = "engineInfo";
private DominoDatasourceApiEngineInfoDto engineInfo;
public static final String JSON_PROPERTY_ID = "id";
private String id;
public static final String JSON_PROPERTY_LAST_ACCESSED = "lastAccessed";
private Map lastAccessed = new HashMap<>();
public static final String JSON_PROPERTY_LAST_UPDATED = "lastUpdated";
private Long lastUpdated;
public static final String JSON_PROPERTY_LAST_UPDATED_BY = "lastUpdatedBy";
private String lastUpdatedBy;
public static final String JSON_PROPERTY_NAME = "name";
private String name;
public static final String JSON_PROPERTY_OWNER_ID = "ownerId";
private String ownerId;
public static final String JSON_PROPERTY_OWNER_INFO = "ownerInfo";
private DominoDatasourceApiDataSourceOwnerInfoDto ownerInfo;
public static final String JSON_PROPERTY_PROJECT_IDS = "projectIds";
private List projectIds = new ArrayList<>();
/**
* Gets or Sets status
*/
public enum StatusEnum {
PENDING("Pending"),
ACTIVE("Active"),
DELETED("Deleted");
private String value;
StatusEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static StatusEnum fromValue(String value) {
for (StatusEnum b : StatusEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_STATUS = "status";
private StatusEnum status;
public static final String JSON_PROPERTY_USE_ALL_DATA_PLANES = "useAllDataPlanes";
private Boolean useAllDataPlanes;
public DominoDatasourceApiDataSourceDto() {
}
public DominoDatasourceApiDataSourceDto addedBy(Map addedBy) {
this.addedBy = addedBy;
return this;
}
public DominoDatasourceApiDataSourceDto putAddedByItem(String key, String addedByItem) {
if (this.addedBy == null) {
this.addedBy = new HashMap<>();
}
this.addedBy.put(key, addedByItem);
return this;
}
/**
* Get addedBy
* @return addedBy
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ADDED_BY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Map getAddedBy() {
return addedBy;
}
@JsonProperty(JSON_PROPERTY_ADDED_BY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setAddedBy(Map addedBy) {
this.addedBy = addedBy;
}
public DominoDatasourceApiDataSourceDto addedToProjectTimeMap(Map addedToProjectTimeMap) {
this.addedToProjectTimeMap = addedToProjectTimeMap;
return this;
}
public DominoDatasourceApiDataSourceDto putAddedToProjectTimeMapItem(String key, Long addedToProjectTimeMapItem) {
if (this.addedToProjectTimeMap == null) {
this.addedToProjectTimeMap = new HashMap<>();
}
this.addedToProjectTimeMap.put(key, addedToProjectTimeMapItem);
return this;
}
/**
* Get addedToProjectTimeMap
* @return addedToProjectTimeMap
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ADDED_TO_PROJECT_TIME_MAP)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Map getAddedToProjectTimeMap() {
return addedToProjectTimeMap;
}
@JsonProperty(JSON_PROPERTY_ADDED_TO_PROJECT_TIME_MAP)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setAddedToProjectTimeMap(Map addedToProjectTimeMap) {
this.addedToProjectTimeMap = addedToProjectTimeMap;
}
public DominoDatasourceApiDataSourceDto adminInfo(DominoDatasourceApiDataSourceAdminInfoDto adminInfo) {
this.adminInfo = adminInfo;
return this;
}
/**
* Get adminInfo
* @return adminInfo
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ADMIN_INFO)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public DominoDatasourceApiDataSourceAdminInfoDto getAdminInfo() {
return adminInfo;
}
@JsonProperty(JSON_PROPERTY_ADMIN_INFO)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setAdminInfo(DominoDatasourceApiDataSourceAdminInfoDto adminInfo) {
this.adminInfo = adminInfo;
}
public DominoDatasourceApiDataSourceDto authType(AuthTypeEnum authType) {
this.authType = authType;
return this;
}
/**
* Get authType
* @return authType
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_AUTH_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public AuthTypeEnum getAuthType() {
return authType;
}
@JsonProperty(JSON_PROPERTY_AUTH_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAuthType(AuthTypeEnum authType) {
this.authType = authType;
}
public DominoDatasourceApiDataSourceDto config(Map config) {
this.config = config;
return this;
}
public DominoDatasourceApiDataSourceDto putConfigItem(String key, String configItem) {
if (this.config == null) {
this.config = new HashMap<>();
}
this.config.put(key, configItem);
return this;
}
/**
* Get config
* @return config
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_CONFIG)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Map getConfig() {
return config;
}
@JsonProperty(JSON_PROPERTY_CONFIG)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setConfig(Map config) {
this.config = config;
}
public DominoDatasourceApiDataSourceDto dataPlanes(List dataPlanes) {
this.dataPlanes = dataPlanes;
return this;
}
public DominoDatasourceApiDataSourceDto addDataPlanesItem(DominoDatasourceApiDataSourceDataPlaneInfo dataPlanesItem) {
if (this.dataPlanes == null) {
this.dataPlanes = new ArrayList<>();
}
this.dataPlanes.add(dataPlanesItem);
return this;
}
/**
* Get dataPlanes
* @return dataPlanes
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DATA_PLANES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getDataPlanes() {
return dataPlanes;
}
@JsonProperty(JSON_PROPERTY_DATA_PLANES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDataPlanes(List dataPlanes) {
this.dataPlanes = dataPlanes;
}
public DominoDatasourceApiDataSourceDto dataSourcePermissions(DominoDatasourceApiDataSourcePermissionsDto dataSourcePermissions) {
this.dataSourcePermissions = dataSourcePermissions;
return this;
}
/**
* Get dataSourcePermissions
* @return dataSourcePermissions
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_DATA_SOURCE_PERMISSIONS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public DominoDatasourceApiDataSourcePermissionsDto getDataSourcePermissions() {
return dataSourcePermissions;
}
@JsonProperty(JSON_PROPERTY_DATA_SOURCE_PERMISSIONS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDataSourcePermissions(DominoDatasourceApiDataSourcePermissionsDto dataSourcePermissions) {
this.dataSourcePermissions = dataSourcePermissions;
}
public DominoDatasourceApiDataSourceDto dataSourceType(DataSourceTypeEnum dataSourceType) {
this.dataSourceType = dataSourceType;
return this;
}
/**
* Get dataSourceType
* @return dataSourceType
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_DATA_SOURCE_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public DataSourceTypeEnum getDataSourceType() {
return dataSourceType;
}
@JsonProperty(JSON_PROPERTY_DATA_SOURCE_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDataSourceType(DataSourceTypeEnum dataSourceType) {
this.dataSourceType = dataSourceType;
}
public DominoDatasourceApiDataSourceDto description(String description) {
this.description = description;
return this;
}
/**
* Get description
* @return description
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDescription() {
return description;
}
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDescription(String description) {
this.description = description;
}
public DominoDatasourceApiDataSourceDto displayName(String displayName) {
this.displayName = displayName;
return this;
}
/**
* Get displayName
* @return displayName
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DISPLAY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDisplayName() {
return displayName;
}
@JsonProperty(JSON_PROPERTY_DISPLAY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
public DominoDatasourceApiDataSourceDto engineInfo(DominoDatasourceApiEngineInfoDto engineInfo) {
this.engineInfo = engineInfo;
return this;
}
/**
* Get engineInfo
* @return engineInfo
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_ENGINE_INFO)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DominoDatasourceApiEngineInfoDto getEngineInfo() {
return engineInfo;
}
@JsonProperty(JSON_PROPERTY_ENGINE_INFO)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEngineInfo(DominoDatasourceApiEngineInfoDto engineInfo) {
this.engineInfo = engineInfo;
}
public DominoDatasourceApiDataSourceDto id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getId() {
return id;
}
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setId(String id) {
this.id = id;
}
public DominoDatasourceApiDataSourceDto lastAccessed(Map lastAccessed) {
this.lastAccessed = lastAccessed;
return this;
}
public DominoDatasourceApiDataSourceDto putLastAccessedItem(String key, Long lastAccessedItem) {
if (this.lastAccessed == null) {
this.lastAccessed = new HashMap<>();
}
this.lastAccessed.put(key, lastAccessedItem);
return this;
}
/**
* Get lastAccessed
* @return lastAccessed
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_LAST_ACCESSED)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Map getLastAccessed() {
return lastAccessed;
}
@JsonProperty(JSON_PROPERTY_LAST_ACCESSED)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setLastAccessed(Map lastAccessed) {
this.lastAccessed = lastAccessed;
}
public DominoDatasourceApiDataSourceDto lastUpdated(Long lastUpdated) {
this.lastUpdated = lastUpdated;
return this;
}
/**
* Get lastUpdated
* @return lastUpdated
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_LAST_UPDATED)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Long getLastUpdated() {
return lastUpdated;
}
@JsonProperty(JSON_PROPERTY_LAST_UPDATED)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setLastUpdated(Long lastUpdated) {
this.lastUpdated = lastUpdated;
}
public DominoDatasourceApiDataSourceDto lastUpdatedBy(String lastUpdatedBy) {
this.lastUpdatedBy = lastUpdatedBy;
return this;
}
/**
* Get lastUpdatedBy
* @return lastUpdatedBy
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_LAST_UPDATED_BY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getLastUpdatedBy() {
return lastUpdatedBy;
}
@JsonProperty(JSON_PROPERTY_LAST_UPDATED_BY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setLastUpdatedBy(String lastUpdatedBy) {
this.lastUpdatedBy = lastUpdatedBy;
}
public DominoDatasourceApiDataSourceDto name(String name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getName() {
return name;
}
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setName(String name) {
this.name = name;
}
public DominoDatasourceApiDataSourceDto ownerId(String ownerId) {
this.ownerId = ownerId;
return this;
}
/**
* Get ownerId
* @return ownerId
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_OWNER_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getOwnerId() {
return ownerId;
}
@JsonProperty(JSON_PROPERTY_OWNER_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setOwnerId(String ownerId) {
this.ownerId = ownerId;
}
public DominoDatasourceApiDataSourceDto ownerInfo(DominoDatasourceApiDataSourceOwnerInfoDto ownerInfo) {
this.ownerInfo = ownerInfo;
return this;
}
/**
* Get ownerInfo
* @return ownerInfo
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_OWNER_INFO)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public DominoDatasourceApiDataSourceOwnerInfoDto getOwnerInfo() {
return ownerInfo;
}
@JsonProperty(JSON_PROPERTY_OWNER_INFO)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setOwnerInfo(DominoDatasourceApiDataSourceOwnerInfoDto ownerInfo) {
this.ownerInfo = ownerInfo;
}
public DominoDatasourceApiDataSourceDto projectIds(List projectIds) {
this.projectIds = projectIds;
return this;
}
public DominoDatasourceApiDataSourceDto addProjectIdsItem(String projectIdsItem) {
if (this.projectIds == null) {
this.projectIds = new ArrayList<>();
}
this.projectIds.add(projectIdsItem);
return this;
}
/**
* Get projectIds
* @return projectIds
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_PROJECT_IDS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getProjectIds() {
return projectIds;
}
@JsonProperty(JSON_PROPERTY_PROJECT_IDS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setProjectIds(List projectIds) {
this.projectIds = projectIds;
}
public DominoDatasourceApiDataSourceDto status(StatusEnum status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public StatusEnum getStatus() {
return status;
}
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setStatus(StatusEnum status) {
this.status = status;
}
public DominoDatasourceApiDataSourceDto useAllDataPlanes(Boolean useAllDataPlanes) {
this.useAllDataPlanes = useAllDataPlanes;
return this;
}
/**
* Get useAllDataPlanes
* @return useAllDataPlanes
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_USE_ALL_DATA_PLANES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getUseAllDataPlanes() {
return useAllDataPlanes;
}
@JsonProperty(JSON_PROPERTY_USE_ALL_DATA_PLANES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUseAllDataPlanes(Boolean useAllDataPlanes) {
this.useAllDataPlanes = useAllDataPlanes;
}
/**
* Return true if this domino.datasource.api.DataSourceDto object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DominoDatasourceApiDataSourceDto dominoDatasourceApiDataSourceDto = (DominoDatasourceApiDataSourceDto) o;
return Objects.equals(this.addedBy, dominoDatasourceApiDataSourceDto.addedBy) &&
Objects.equals(this.addedToProjectTimeMap, dominoDatasourceApiDataSourceDto.addedToProjectTimeMap) &&
Objects.equals(this.adminInfo, dominoDatasourceApiDataSourceDto.adminInfo) &&
Objects.equals(this.authType, dominoDatasourceApiDataSourceDto.authType) &&
Objects.equals(this.config, dominoDatasourceApiDataSourceDto.config) &&
Objects.equals(this.dataPlanes, dominoDatasourceApiDataSourceDto.dataPlanes) &&
Objects.equals(this.dataSourcePermissions, dominoDatasourceApiDataSourceDto.dataSourcePermissions) &&
Objects.equals(this.dataSourceType, dominoDatasourceApiDataSourceDto.dataSourceType) &&
Objects.equals(this.description, dominoDatasourceApiDataSourceDto.description) &&
Objects.equals(this.displayName, dominoDatasourceApiDataSourceDto.displayName) &&
Objects.equals(this.engineInfo, dominoDatasourceApiDataSourceDto.engineInfo) &&
Objects.equals(this.id, dominoDatasourceApiDataSourceDto.id) &&
Objects.equals(this.lastAccessed, dominoDatasourceApiDataSourceDto.lastAccessed) &&
Objects.equals(this.lastUpdated, dominoDatasourceApiDataSourceDto.lastUpdated) &&
Objects.equals(this.lastUpdatedBy, dominoDatasourceApiDataSourceDto.lastUpdatedBy) &&
Objects.equals(this.name, dominoDatasourceApiDataSourceDto.name) &&
Objects.equals(this.ownerId, dominoDatasourceApiDataSourceDto.ownerId) &&
Objects.equals(this.ownerInfo, dominoDatasourceApiDataSourceDto.ownerInfo) &&
Objects.equals(this.projectIds, dominoDatasourceApiDataSourceDto.projectIds) &&
Objects.equals(this.status, dominoDatasourceApiDataSourceDto.status) &&
Objects.equals(this.useAllDataPlanes, dominoDatasourceApiDataSourceDto.useAllDataPlanes);
}
@Override
public int hashCode() {
return Objects.hash(addedBy, addedToProjectTimeMap, adminInfo, authType, config, dataPlanes, dataSourcePermissions, dataSourceType, description, displayName, engineInfo, id, lastAccessed, lastUpdated, lastUpdatedBy, name, ownerId, ownerInfo, projectIds, status, useAllDataPlanes);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DominoDatasourceApiDataSourceDto {\n");
sb.append(" addedBy: ").append(toIndentedString(addedBy)).append("\n");
sb.append(" addedToProjectTimeMap: ").append(toIndentedString(addedToProjectTimeMap)).append("\n");
sb.append(" adminInfo: ").append(toIndentedString(adminInfo)).append("\n");
sb.append(" authType: ").append(toIndentedString(authType)).append("\n");
sb.append(" config: ").append(toIndentedString(config)).append("\n");
sb.append(" dataPlanes: ").append(toIndentedString(dataPlanes)).append("\n");
sb.append(" dataSourcePermissions: ").append(toIndentedString(dataSourcePermissions)).append("\n");
sb.append(" dataSourceType: ").append(toIndentedString(dataSourceType)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" displayName: ").append(toIndentedString(displayName)).append("\n");
sb.append(" engineInfo: ").append(toIndentedString(engineInfo)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" lastAccessed: ").append(toIndentedString(lastAccessed)).append("\n");
sb.append(" lastUpdated: ").append(toIndentedString(lastUpdated)).append("\n");
sb.append(" lastUpdatedBy: ").append(toIndentedString(lastUpdatedBy)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" ownerId: ").append(toIndentedString(ownerId)).append("\n");
sb.append(" ownerInfo: ").append(toIndentedString(ownerInfo)).append("\n");
sb.append(" projectIds: ").append(toIndentedString(projectIds)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" useAllDataPlanes: ").append(toIndentedString(useAllDataPlanes)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `addedBy` to the URL query string
if (getAddedBy() != null) {
for (String _key : getAddedBy().keySet()) {
joiner.add(String.format("%saddedBy%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, _key, containerSuffix),
getAddedBy().get(_key), URLEncoder.encode(ApiClient.valueToString(getAddedBy().get(_key)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
// add `addedToProjectTimeMap` to the URL query string
if (getAddedToProjectTimeMap() != null) {
for (String _key : getAddedToProjectTimeMap().keySet()) {
joiner.add(String.format("%saddedToProjectTimeMap%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, _key, containerSuffix),
getAddedToProjectTimeMap().get(_key), URLEncoder.encode(ApiClient.valueToString(getAddedToProjectTimeMap().get(_key)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
// add `adminInfo` to the URL query string
if (getAdminInfo() != null) {
joiner.add(getAdminInfo().toUrlQueryString(prefix + "adminInfo" + suffix));
}
// add `authType` to the URL query string
if (getAuthType() != null) {
joiner.add(String.format("%sauthType%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getAuthType()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `config` to the URL query string
if (getConfig() != null) {
for (String _key : getConfig().keySet()) {
joiner.add(String.format("%sconfig%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, _key, containerSuffix),
getConfig().get(_key), URLEncoder.encode(ApiClient.valueToString(getConfig().get(_key)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
// add `dataPlanes` to the URL query string
if (getDataPlanes() != null) {
for (int i = 0; i < getDataPlanes().size(); i++) {
if (getDataPlanes().get(i) != null) {
joiner.add(getDataPlanes().get(i).toUrlQueryString(String.format("%sdataPlanes%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `dataSourcePermissions` to the URL query string
if (getDataSourcePermissions() != null) {
joiner.add(getDataSourcePermissions().toUrlQueryString(prefix + "dataSourcePermissions" + suffix));
}
// add `dataSourceType` to the URL query string
if (getDataSourceType() != null) {
joiner.add(String.format("%sdataSourceType%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getDataSourceType()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `description` to the URL query string
if (getDescription() != null) {
joiner.add(String.format("%sdescription%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getDescription()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `displayName` to the URL query string
if (getDisplayName() != null) {
joiner.add(String.format("%sdisplayName%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getDisplayName()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `engineInfo` to the URL query string
if (getEngineInfo() != null) {
joiner.add(getEngineInfo().toUrlQueryString(prefix + "engineInfo" + suffix));
}
// add `id` to the URL query string
if (getId() != null) {
joiner.add(String.format("%sid%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `lastAccessed` to the URL query string
if (getLastAccessed() != null) {
for (String _key : getLastAccessed().keySet()) {
joiner.add(String.format("%slastAccessed%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, _key, containerSuffix),
getLastAccessed().get(_key), URLEncoder.encode(ApiClient.valueToString(getLastAccessed().get(_key)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
// add `lastUpdated` to the URL query string
if (getLastUpdated() != null) {
joiner.add(String.format("%slastUpdated%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getLastUpdated()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `lastUpdatedBy` to the URL query string
if (getLastUpdatedBy() != null) {
joiner.add(String.format("%slastUpdatedBy%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getLastUpdatedBy()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `name` to the URL query string
if (getName() != null) {
joiner.add(String.format("%sname%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getName()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `ownerId` to the URL query string
if (getOwnerId() != null) {
joiner.add(String.format("%sownerId%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getOwnerId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `ownerInfo` to the URL query string
if (getOwnerInfo() != null) {
joiner.add(getOwnerInfo().toUrlQueryString(prefix + "ownerInfo" + suffix));
}
// add `projectIds` to the URL query string
if (getProjectIds() != null) {
for (int i = 0; i < getProjectIds().size(); i++) {
joiner.add(String.format("%sprojectIds%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(ApiClient.valueToString(getProjectIds().get(i)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
// add `status` to the URL query string
if (getStatus() != null) {
joiner.add(String.format("%sstatus%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getStatus()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `useAllDataPlanes` to the URL query string
if (getUseAllDataPlanes() != null) {
joiner.add(String.format("%suseAllDataPlanes%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getUseAllDataPlanes()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy