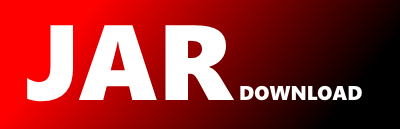
com.dominodatalab.api.model.DominoJobsWebStartJobRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of domino-java-client Show documentation
Show all versions of domino-java-client Show documentation
Domino Data Lab API Client to connect to Domino web services using Java HTTP Client.
/*
* Domino Data Lab API v4
* This API provides access to select Domino functions available in Domino's non-public API. To authenticate your requests, include your API Key (which you can find on your account page) with the header X-Domino-Api-Key.
*
* The version of the OpenAPI document: 4.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.api.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.dominodatalab.api.model.DominoJobsInterfaceComputeClusterConfigSpecDto;
import com.dominodatalab.api.model.DominoJobsInterfaceComputeClusterConfigSpecDtoComputeEnvironmentRevisionSpec;
import com.dominodatalab.api.model.DominoProjectsApiOnDemandSparkClusterPropertiesSpec;
import com.dominodatalab.api.model.DominoProjectsApiRepositoriesReferenceDTO;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.dominodatalab.api.invoker.ApiClient;
/**
* DominoJobsWebStartJobRequest
*/
@JsonPropertyOrder({
DominoJobsWebStartJobRequest.JSON_PROPERTY_COMMAND_TO_RUN,
DominoJobsWebStartJobRequest.JSON_PROPERTY_COMMIT_ID,
DominoJobsWebStartJobRequest.JSON_PROPERTY_COMPUTE_CLUSTER_PROPERTIES,
DominoJobsWebStartJobRequest.JSON_PROPERTY_ENVIRONMENT_ID,
DominoJobsWebStartJobRequest.JSON_PROPERTY_ENVIRONMENT_REVISION_SPEC,
DominoJobsWebStartJobRequest.JSON_PROPERTY_EXTERNAL_VOLUME_MOUNTS,
DominoJobsWebStartJobRequest.JSON_PROPERTY_MAIN_REPO_GIT_REF,
DominoJobsWebStartJobRequest.JSON_PROPERTY_ON_DEMAND_SPARK_CLUSTER_PROPERTIES,
DominoJobsWebStartJobRequest.JSON_PROPERTY_OVERRIDE_HARDWARE_TIER_ID,
DominoJobsWebStartJobRequest.JSON_PROPERTY_OVERRIDE_VOLUME_SIZE_GI_B,
DominoJobsWebStartJobRequest.JSON_PROPERTY_PROJECT_ID,
DominoJobsWebStartJobRequest.JSON_PROPERTY_SNAPSHOT_DATASETS_ON_COMPLETION,
DominoJobsWebStartJobRequest.JSON_PROPERTY_TITLE
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-10-04T16:37:26.309454-04:00[America/New_York]", comments = "Generator version: 7.8.0")
public class DominoJobsWebStartJobRequest {
public static final String JSON_PROPERTY_COMMAND_TO_RUN = "commandToRun";
private String commandToRun;
public static final String JSON_PROPERTY_COMMIT_ID = "commitId";
private String commitId;
public static final String JSON_PROPERTY_COMPUTE_CLUSTER_PROPERTIES = "computeClusterProperties";
private DominoJobsInterfaceComputeClusterConfigSpecDto computeClusterProperties;
public static final String JSON_PROPERTY_ENVIRONMENT_ID = "environmentId";
private String environmentId;
public static final String JSON_PROPERTY_ENVIRONMENT_REVISION_SPEC = "environmentRevisionSpec";
private DominoJobsInterfaceComputeClusterConfigSpecDtoComputeEnvironmentRevisionSpec environmentRevisionSpec;
public static final String JSON_PROPERTY_EXTERNAL_VOLUME_MOUNTS = "externalVolumeMounts";
private List externalVolumeMounts;
public static final String JSON_PROPERTY_MAIN_REPO_GIT_REF = "mainRepoGitRef";
private DominoProjectsApiRepositoriesReferenceDTO mainRepoGitRef;
public static final String JSON_PROPERTY_ON_DEMAND_SPARK_CLUSTER_PROPERTIES = "onDemandSparkClusterProperties";
private DominoProjectsApiOnDemandSparkClusterPropertiesSpec onDemandSparkClusterProperties;
public static final String JSON_PROPERTY_OVERRIDE_HARDWARE_TIER_ID = "overrideHardwareTierId";
private String overrideHardwareTierId;
public static final String JSON_PROPERTY_OVERRIDE_VOLUME_SIZE_GI_B = "overrideVolumeSizeGiB";
private Double overrideVolumeSizeGiB;
public static final String JSON_PROPERTY_PROJECT_ID = "projectId";
private String projectId;
public static final String JSON_PROPERTY_SNAPSHOT_DATASETS_ON_COMPLETION = "snapshotDatasetsOnCompletion";
private Boolean snapshotDatasetsOnCompletion;
public static final String JSON_PROPERTY_TITLE = "title";
private String title;
public DominoJobsWebStartJobRequest() {
}
public DominoJobsWebStartJobRequest commandToRun(String commandToRun) {
this.commandToRun = commandToRun;
return this;
}
/**
* Get commandToRun
* @return commandToRun
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_COMMAND_TO_RUN)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getCommandToRun() {
return commandToRun;
}
@JsonProperty(JSON_PROPERTY_COMMAND_TO_RUN)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setCommandToRun(String commandToRun) {
this.commandToRun = commandToRun;
}
public DominoJobsWebStartJobRequest commitId(String commitId) {
this.commitId = commitId;
return this;
}
/**
* Get commitId
* @return commitId
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_COMMIT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getCommitId() {
return commitId;
}
@JsonProperty(JSON_PROPERTY_COMMIT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCommitId(String commitId) {
this.commitId = commitId;
}
public DominoJobsWebStartJobRequest computeClusterProperties(DominoJobsInterfaceComputeClusterConfigSpecDto computeClusterProperties) {
this.computeClusterProperties = computeClusterProperties;
return this;
}
/**
* Get computeClusterProperties
* @return computeClusterProperties
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_COMPUTE_CLUSTER_PROPERTIES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DominoJobsInterfaceComputeClusterConfigSpecDto getComputeClusterProperties() {
return computeClusterProperties;
}
@JsonProperty(JSON_PROPERTY_COMPUTE_CLUSTER_PROPERTIES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setComputeClusterProperties(DominoJobsInterfaceComputeClusterConfigSpecDto computeClusterProperties) {
this.computeClusterProperties = computeClusterProperties;
}
public DominoJobsWebStartJobRequest environmentId(String environmentId) {
this.environmentId = environmentId;
return this;
}
/**
* Get environmentId
* @return environmentId
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_ENVIRONMENT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getEnvironmentId() {
return environmentId;
}
@JsonProperty(JSON_PROPERTY_ENVIRONMENT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEnvironmentId(String environmentId) {
this.environmentId = environmentId;
}
public DominoJobsWebStartJobRequest environmentRevisionSpec(DominoJobsInterfaceComputeClusterConfigSpecDtoComputeEnvironmentRevisionSpec environmentRevisionSpec) {
this.environmentRevisionSpec = environmentRevisionSpec;
return this;
}
/**
* Get environmentRevisionSpec
* @return environmentRevisionSpec
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_ENVIRONMENT_REVISION_SPEC)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DominoJobsInterfaceComputeClusterConfigSpecDtoComputeEnvironmentRevisionSpec getEnvironmentRevisionSpec() {
return environmentRevisionSpec;
}
@JsonProperty(JSON_PROPERTY_ENVIRONMENT_REVISION_SPEC)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEnvironmentRevisionSpec(DominoJobsInterfaceComputeClusterConfigSpecDtoComputeEnvironmentRevisionSpec environmentRevisionSpec) {
this.environmentRevisionSpec = environmentRevisionSpec;
}
public DominoJobsWebStartJobRequest externalVolumeMounts(List externalVolumeMounts) {
this.externalVolumeMounts = externalVolumeMounts;
return this;
}
public DominoJobsWebStartJobRequest addExternalVolumeMountsItem(String externalVolumeMountsItem) {
if (this.externalVolumeMounts == null) {
this.externalVolumeMounts = new ArrayList<>();
}
this.externalVolumeMounts.add(externalVolumeMountsItem);
return this;
}
/**
* Get externalVolumeMounts
* @return externalVolumeMounts
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_EXTERNAL_VOLUME_MOUNTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getExternalVolumeMounts() {
return externalVolumeMounts;
}
@JsonProperty(JSON_PROPERTY_EXTERNAL_VOLUME_MOUNTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setExternalVolumeMounts(List externalVolumeMounts) {
this.externalVolumeMounts = externalVolumeMounts;
}
public DominoJobsWebStartJobRequest mainRepoGitRef(DominoProjectsApiRepositoriesReferenceDTO mainRepoGitRef) {
this.mainRepoGitRef = mainRepoGitRef;
return this;
}
/**
* Get mainRepoGitRef
* @return mainRepoGitRef
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_MAIN_REPO_GIT_REF)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DominoProjectsApiRepositoriesReferenceDTO getMainRepoGitRef() {
return mainRepoGitRef;
}
@JsonProperty(JSON_PROPERTY_MAIN_REPO_GIT_REF)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMainRepoGitRef(DominoProjectsApiRepositoriesReferenceDTO mainRepoGitRef) {
this.mainRepoGitRef = mainRepoGitRef;
}
public DominoJobsWebStartJobRequest onDemandSparkClusterProperties(DominoProjectsApiOnDemandSparkClusterPropertiesSpec onDemandSparkClusterProperties) {
this.onDemandSparkClusterProperties = onDemandSparkClusterProperties;
return this;
}
/**
* Get onDemandSparkClusterProperties
* @return onDemandSparkClusterProperties
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_ON_DEMAND_SPARK_CLUSTER_PROPERTIES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DominoProjectsApiOnDemandSparkClusterPropertiesSpec getOnDemandSparkClusterProperties() {
return onDemandSparkClusterProperties;
}
@JsonProperty(JSON_PROPERTY_ON_DEMAND_SPARK_CLUSTER_PROPERTIES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setOnDemandSparkClusterProperties(DominoProjectsApiOnDemandSparkClusterPropertiesSpec onDemandSparkClusterProperties) {
this.onDemandSparkClusterProperties = onDemandSparkClusterProperties;
}
public DominoJobsWebStartJobRequest overrideHardwareTierId(String overrideHardwareTierId) {
this.overrideHardwareTierId = overrideHardwareTierId;
return this;
}
/**
* Get overrideHardwareTierId
* @return overrideHardwareTierId
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_OVERRIDE_HARDWARE_TIER_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getOverrideHardwareTierId() {
return overrideHardwareTierId;
}
@JsonProperty(JSON_PROPERTY_OVERRIDE_HARDWARE_TIER_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setOverrideHardwareTierId(String overrideHardwareTierId) {
this.overrideHardwareTierId = overrideHardwareTierId;
}
public DominoJobsWebStartJobRequest overrideVolumeSizeGiB(Double overrideVolumeSizeGiB) {
this.overrideVolumeSizeGiB = overrideVolumeSizeGiB;
return this;
}
/**
* Get overrideVolumeSizeGiB
* @return overrideVolumeSizeGiB
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_OVERRIDE_VOLUME_SIZE_GI_B)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getOverrideVolumeSizeGiB() {
return overrideVolumeSizeGiB;
}
@JsonProperty(JSON_PROPERTY_OVERRIDE_VOLUME_SIZE_GI_B)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setOverrideVolumeSizeGiB(Double overrideVolumeSizeGiB) {
this.overrideVolumeSizeGiB = overrideVolumeSizeGiB;
}
public DominoJobsWebStartJobRequest projectId(String projectId) {
this.projectId = projectId;
return this;
}
/**
* Get projectId
* @return projectId
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_PROJECT_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getProjectId() {
return projectId;
}
@JsonProperty(JSON_PROPERTY_PROJECT_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setProjectId(String projectId) {
this.projectId = projectId;
}
public DominoJobsWebStartJobRequest snapshotDatasetsOnCompletion(Boolean snapshotDatasetsOnCompletion) {
this.snapshotDatasetsOnCompletion = snapshotDatasetsOnCompletion;
return this;
}
/**
* Get snapshotDatasetsOnCompletion
* @return snapshotDatasetsOnCompletion
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_SNAPSHOT_DATASETS_ON_COMPLETION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getSnapshotDatasetsOnCompletion() {
return snapshotDatasetsOnCompletion;
}
@JsonProperty(JSON_PROPERTY_SNAPSHOT_DATASETS_ON_COMPLETION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSnapshotDatasetsOnCompletion(Boolean snapshotDatasetsOnCompletion) {
this.snapshotDatasetsOnCompletion = snapshotDatasetsOnCompletion;
}
public DominoJobsWebStartJobRequest title(String title) {
this.title = title;
return this;
}
/**
* Get title
* @return title
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_TITLE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getTitle() {
return title;
}
@JsonProperty(JSON_PROPERTY_TITLE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTitle(String title) {
this.title = title;
}
/**
* Return true if this domino.jobs.web.StartJobRequest object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DominoJobsWebStartJobRequest dominoJobsWebStartJobRequest = (DominoJobsWebStartJobRequest) o;
return Objects.equals(this.commandToRun, dominoJobsWebStartJobRequest.commandToRun) &&
Objects.equals(this.commitId, dominoJobsWebStartJobRequest.commitId) &&
Objects.equals(this.computeClusterProperties, dominoJobsWebStartJobRequest.computeClusterProperties) &&
Objects.equals(this.environmentId, dominoJobsWebStartJobRequest.environmentId) &&
Objects.equals(this.environmentRevisionSpec, dominoJobsWebStartJobRequest.environmentRevisionSpec) &&
Objects.equals(this.externalVolumeMounts, dominoJobsWebStartJobRequest.externalVolumeMounts) &&
Objects.equals(this.mainRepoGitRef, dominoJobsWebStartJobRequest.mainRepoGitRef) &&
Objects.equals(this.onDemandSparkClusterProperties, dominoJobsWebStartJobRequest.onDemandSparkClusterProperties) &&
Objects.equals(this.overrideHardwareTierId, dominoJobsWebStartJobRequest.overrideHardwareTierId) &&
Objects.equals(this.overrideVolumeSizeGiB, dominoJobsWebStartJobRequest.overrideVolumeSizeGiB) &&
Objects.equals(this.projectId, dominoJobsWebStartJobRequest.projectId) &&
Objects.equals(this.snapshotDatasetsOnCompletion, dominoJobsWebStartJobRequest.snapshotDatasetsOnCompletion) &&
Objects.equals(this.title, dominoJobsWebStartJobRequest.title);
}
@Override
public int hashCode() {
return Objects.hash(commandToRun, commitId, computeClusterProperties, environmentId, environmentRevisionSpec, externalVolumeMounts, mainRepoGitRef, onDemandSparkClusterProperties, overrideHardwareTierId, overrideVolumeSizeGiB, projectId, snapshotDatasetsOnCompletion, title);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DominoJobsWebStartJobRequest {\n");
sb.append(" commandToRun: ").append(toIndentedString(commandToRun)).append("\n");
sb.append(" commitId: ").append(toIndentedString(commitId)).append("\n");
sb.append(" computeClusterProperties: ").append(toIndentedString(computeClusterProperties)).append("\n");
sb.append(" environmentId: ").append(toIndentedString(environmentId)).append("\n");
sb.append(" environmentRevisionSpec: ").append(toIndentedString(environmentRevisionSpec)).append("\n");
sb.append(" externalVolumeMounts: ").append(toIndentedString(externalVolumeMounts)).append("\n");
sb.append(" mainRepoGitRef: ").append(toIndentedString(mainRepoGitRef)).append("\n");
sb.append(" onDemandSparkClusterProperties: ").append(toIndentedString(onDemandSparkClusterProperties)).append("\n");
sb.append(" overrideHardwareTierId: ").append(toIndentedString(overrideHardwareTierId)).append("\n");
sb.append(" overrideVolumeSizeGiB: ").append(toIndentedString(overrideVolumeSizeGiB)).append("\n");
sb.append(" projectId: ").append(toIndentedString(projectId)).append("\n");
sb.append(" snapshotDatasetsOnCompletion: ").append(toIndentedString(snapshotDatasetsOnCompletion)).append("\n");
sb.append(" title: ").append(toIndentedString(title)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `commandToRun` to the URL query string
if (getCommandToRun() != null) {
joiner.add(String.format("%scommandToRun%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getCommandToRun()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `commitId` to the URL query string
if (getCommitId() != null) {
joiner.add(String.format("%scommitId%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getCommitId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `computeClusterProperties` to the URL query string
if (getComputeClusterProperties() != null) {
joiner.add(getComputeClusterProperties().toUrlQueryString(prefix + "computeClusterProperties" + suffix));
}
// add `environmentId` to the URL query string
if (getEnvironmentId() != null) {
joiner.add(String.format("%senvironmentId%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getEnvironmentId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `environmentRevisionSpec` to the URL query string
if (getEnvironmentRevisionSpec() != null) {
joiner.add(getEnvironmentRevisionSpec().toUrlQueryString(prefix + "environmentRevisionSpec" + suffix));
}
// add `externalVolumeMounts` to the URL query string
if (getExternalVolumeMounts() != null) {
for (int i = 0; i < getExternalVolumeMounts().size(); i++) {
joiner.add(String.format("%sexternalVolumeMounts%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(ApiClient.valueToString(getExternalVolumeMounts().get(i)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
// add `mainRepoGitRef` to the URL query string
if (getMainRepoGitRef() != null) {
joiner.add(getMainRepoGitRef().toUrlQueryString(prefix + "mainRepoGitRef" + suffix));
}
// add `onDemandSparkClusterProperties` to the URL query string
if (getOnDemandSparkClusterProperties() != null) {
joiner.add(getOnDemandSparkClusterProperties().toUrlQueryString(prefix + "onDemandSparkClusterProperties" + suffix));
}
// add `overrideHardwareTierId` to the URL query string
if (getOverrideHardwareTierId() != null) {
joiner.add(String.format("%soverrideHardwareTierId%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getOverrideHardwareTierId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `overrideVolumeSizeGiB` to the URL query string
if (getOverrideVolumeSizeGiB() != null) {
joiner.add(String.format("%soverrideVolumeSizeGiB%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getOverrideVolumeSizeGiB()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `projectId` to the URL query string
if (getProjectId() != null) {
joiner.add(String.format("%sprojectId%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getProjectId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `snapshotDatasetsOnCompletion` to the URL query string
if (getSnapshotDatasetsOnCompletion() != null) {
joiner.add(String.format("%ssnapshotDatasetsOnCompletion%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getSnapshotDatasetsOnCompletion()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `title` to the URL query string
if (getTitle() != null) {
joiner.add(String.format("%stitle%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getTitle()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy