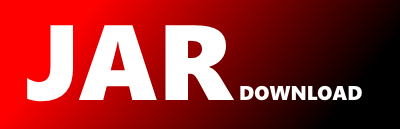
com.dominodatalab.api.model.DominoProjectsApiProjectSummary Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of domino-java-client Show documentation
Show all versions of domino-java-client Show documentation
Domino Data Lab API Client to connect to Domino web services using Java HTTP Client.
/*
* Domino Data Lab API v4
* This API provides access to select Domino functions available in Domino's non-public API. To authenticate your requests, include your API Key (which you can find on your account page) with the header X-Domino-Api-Key.
*
* The version of the OpenAPI document: 4.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.api.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.dominodatalab.api.model.DominoProjectsApiBillingTag;
import com.dominodatalab.api.model.DominoProjectsApiCollaboratorDTO;
import com.dominodatalab.api.model.DominoProjectsApiProjectGitRepositoryTemp;
import com.dominodatalab.api.model.DominoProjectsApiProjectStatus;
import com.dominodatalab.api.model.DominoProjectsApiProjectTagDTO;
import com.dominodatalab.api.model.DominoProjectsApiProjectTemplateDetails;
import com.dominodatalab.api.model.DominoProjectsApiRepositoriesGitRepositoryDTO;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.dominodatalab.api.invoker.ApiClient;
/**
* DominoProjectsApiProjectSummary
*/
@JsonPropertyOrder({
DominoProjectsApiProjectSummary.JSON_PROPERTY_BILLING_TAG,
DominoProjectsApiProjectSummary.JSON_PROPERTY_COLLABORATOR_IDS,
DominoProjectsApiProjectSummary.JSON_PROPERTY_COLLABORATORS,
DominoProjectsApiProjectSummary.JSON_PROPERTY_CREATED,
DominoProjectsApiProjectSummary.JSON_PROPERTY_DESCRIPTION,
DominoProjectsApiProjectSummary.JSON_PROPERTY_ID,
DominoProjectsApiProjectSummary.JSON_PROPERTY_IMPORTED_GIT_REPOSITORIES,
DominoProjectsApiProjectSummary.JSON_PROPERTY_INTERNAL_TAGS,
DominoProjectsApiProjectSummary.JSON_PROPERTY_MAIN_REPOSITORY,
DominoProjectsApiProjectSummary.JSON_PROPERTY_NAME,
DominoProjectsApiProjectSummary.JSON_PROPERTY_OWNER_ID,
DominoProjectsApiProjectSummary.JSON_PROPERTY_OWNER_USERNAME,
DominoProjectsApiProjectSummary.JSON_PROPERTY_STAGE_ID,
DominoProjectsApiProjectSummary.JSON_PROPERTY_STATUS,
DominoProjectsApiProjectSummary.JSON_PROPERTY_TAGS,
DominoProjectsApiProjectSummary.JSON_PROPERTY_TEMPLATE_DETAILS,
DominoProjectsApiProjectSummary.JSON_PROPERTY_VISIBILITY
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-10-04T16:37:26.309454-04:00[America/New_York]", comments = "Generator version: 7.8.0")
public class DominoProjectsApiProjectSummary {
public static final String JSON_PROPERTY_BILLING_TAG = "billingTag";
private DominoProjectsApiBillingTag billingTag;
public static final String JSON_PROPERTY_COLLABORATOR_IDS = "collaboratorIds";
private List collaboratorIds = new ArrayList<>();
public static final String JSON_PROPERTY_COLLABORATORS = "collaborators";
private List collaborators = new ArrayList<>();
public static final String JSON_PROPERTY_CREATED = "created";
private OffsetDateTime created;
public static final String JSON_PROPERTY_DESCRIPTION = "description";
private String description;
public static final String JSON_PROPERTY_ID = "id";
private String id;
public static final String JSON_PROPERTY_IMPORTED_GIT_REPOSITORIES = "importedGitRepositories";
private List importedGitRepositories = new ArrayList<>();
public static final String JSON_PROPERTY_INTERNAL_TAGS = "internalTags";
private List internalTags;
public static final String JSON_PROPERTY_MAIN_REPOSITORY = "mainRepository";
private DominoProjectsApiProjectGitRepositoryTemp mainRepository;
public static final String JSON_PROPERTY_NAME = "name";
private String name;
public static final String JSON_PROPERTY_OWNER_ID = "ownerId";
private String ownerId;
public static final String JSON_PROPERTY_OWNER_USERNAME = "ownerUsername";
private String ownerUsername;
public static final String JSON_PROPERTY_STAGE_ID = "stageId";
private String stageId;
public static final String JSON_PROPERTY_STATUS = "status";
private DominoProjectsApiProjectStatus status;
public static final String JSON_PROPERTY_TAGS = "tags";
private List tags = new ArrayList<>();
public static final String JSON_PROPERTY_TEMPLATE_DETAILS = "templateDetails";
private DominoProjectsApiProjectTemplateDetails templateDetails;
public static final String JSON_PROPERTY_VISIBILITY = "visibility";
private String visibility;
public DominoProjectsApiProjectSummary() {
}
public DominoProjectsApiProjectSummary billingTag(DominoProjectsApiBillingTag billingTag) {
this.billingTag = billingTag;
return this;
}
/**
* Get billingTag
* @return billingTag
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_BILLING_TAG)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DominoProjectsApiBillingTag getBillingTag() {
return billingTag;
}
@JsonProperty(JSON_PROPERTY_BILLING_TAG)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setBillingTag(DominoProjectsApiBillingTag billingTag) {
this.billingTag = billingTag;
}
public DominoProjectsApiProjectSummary collaboratorIds(List collaboratorIds) {
this.collaboratorIds = collaboratorIds;
return this;
}
public DominoProjectsApiProjectSummary addCollaboratorIdsItem(String collaboratorIdsItem) {
if (this.collaboratorIds == null) {
this.collaboratorIds = new ArrayList<>();
}
this.collaboratorIds.add(collaboratorIdsItem);
return this;
}
/**
* Get collaboratorIds
* @return collaboratorIds
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_COLLABORATOR_IDS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getCollaboratorIds() {
return collaboratorIds;
}
@JsonProperty(JSON_PROPERTY_COLLABORATOR_IDS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setCollaboratorIds(List collaboratorIds) {
this.collaboratorIds = collaboratorIds;
}
public DominoProjectsApiProjectSummary collaborators(List collaborators) {
this.collaborators = collaborators;
return this;
}
public DominoProjectsApiProjectSummary addCollaboratorsItem(DominoProjectsApiCollaboratorDTO collaboratorsItem) {
if (this.collaborators == null) {
this.collaborators = new ArrayList<>();
}
this.collaborators.add(collaboratorsItem);
return this;
}
/**
* Get collaborators
* @return collaborators
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_COLLABORATORS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getCollaborators() {
return collaborators;
}
@JsonProperty(JSON_PROPERTY_COLLABORATORS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setCollaborators(List collaborators) {
this.collaborators = collaborators;
}
public DominoProjectsApiProjectSummary created(OffsetDateTime created) {
this.created = created;
return this;
}
/**
* Get created
* @return created
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_CREATED)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public OffsetDateTime getCreated() {
return created;
}
@JsonProperty(JSON_PROPERTY_CREATED)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setCreated(OffsetDateTime created) {
this.created = created;
}
public DominoProjectsApiProjectSummary description(String description) {
this.description = description;
return this;
}
/**
* Get description
* @return description
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getDescription() {
return description;
}
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDescription(String description) {
this.description = description;
}
public DominoProjectsApiProjectSummary id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getId() {
return id;
}
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setId(String id) {
this.id = id;
}
public DominoProjectsApiProjectSummary importedGitRepositories(List importedGitRepositories) {
this.importedGitRepositories = importedGitRepositories;
return this;
}
public DominoProjectsApiProjectSummary addImportedGitRepositoriesItem(DominoProjectsApiRepositoriesGitRepositoryDTO importedGitRepositoriesItem) {
if (this.importedGitRepositories == null) {
this.importedGitRepositories = new ArrayList<>();
}
this.importedGitRepositories.add(importedGitRepositoriesItem);
return this;
}
/**
* Get importedGitRepositories
* @return importedGitRepositories
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_IMPORTED_GIT_REPOSITORIES)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getImportedGitRepositories() {
return importedGitRepositories;
}
@JsonProperty(JSON_PROPERTY_IMPORTED_GIT_REPOSITORIES)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setImportedGitRepositories(List importedGitRepositories) {
this.importedGitRepositories = importedGitRepositories;
}
public DominoProjectsApiProjectSummary internalTags(List internalTags) {
this.internalTags = internalTags;
return this;
}
public DominoProjectsApiProjectSummary addInternalTagsItem(String internalTagsItem) {
if (this.internalTags == null) {
this.internalTags = new ArrayList<>();
}
this.internalTags.add(internalTagsItem);
return this;
}
/**
* Get internalTags
* @return internalTags
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_INTERNAL_TAGS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getInternalTags() {
return internalTags;
}
@JsonProperty(JSON_PROPERTY_INTERNAL_TAGS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setInternalTags(List internalTags) {
this.internalTags = internalTags;
}
public DominoProjectsApiProjectSummary mainRepository(DominoProjectsApiProjectGitRepositoryTemp mainRepository) {
this.mainRepository = mainRepository;
return this;
}
/**
* Get mainRepository
* @return mainRepository
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_MAIN_REPOSITORY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DominoProjectsApiProjectGitRepositoryTemp getMainRepository() {
return mainRepository;
}
@JsonProperty(JSON_PROPERTY_MAIN_REPOSITORY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMainRepository(DominoProjectsApiProjectGitRepositoryTemp mainRepository) {
this.mainRepository = mainRepository;
}
public DominoProjectsApiProjectSummary name(String name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getName() {
return name;
}
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setName(String name) {
this.name = name;
}
public DominoProjectsApiProjectSummary ownerId(String ownerId) {
this.ownerId = ownerId;
return this;
}
/**
* Get ownerId
* @return ownerId
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_OWNER_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getOwnerId() {
return ownerId;
}
@JsonProperty(JSON_PROPERTY_OWNER_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setOwnerId(String ownerId) {
this.ownerId = ownerId;
}
public DominoProjectsApiProjectSummary ownerUsername(String ownerUsername) {
this.ownerUsername = ownerUsername;
return this;
}
/**
* Get ownerUsername
* @return ownerUsername
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_OWNER_USERNAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getOwnerUsername() {
return ownerUsername;
}
@JsonProperty(JSON_PROPERTY_OWNER_USERNAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setOwnerUsername(String ownerUsername) {
this.ownerUsername = ownerUsername;
}
public DominoProjectsApiProjectSummary stageId(String stageId) {
this.stageId = stageId;
return this;
}
/**
* Get stageId
* @return stageId
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_STAGE_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getStageId() {
return stageId;
}
@JsonProperty(JSON_PROPERTY_STAGE_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setStageId(String stageId) {
this.stageId = stageId;
}
public DominoProjectsApiProjectSummary status(DominoProjectsApiProjectStatus status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public DominoProjectsApiProjectStatus getStatus() {
return status;
}
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setStatus(DominoProjectsApiProjectStatus status) {
this.status = status;
}
public DominoProjectsApiProjectSummary tags(List tags) {
this.tags = tags;
return this;
}
public DominoProjectsApiProjectSummary addTagsItem(DominoProjectsApiProjectTagDTO tagsItem) {
if (this.tags == null) {
this.tags = new ArrayList<>();
}
this.tags.add(tagsItem);
return this;
}
/**
* Get tags
* @return tags
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_TAGS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getTags() {
return tags;
}
@JsonProperty(JSON_PROPERTY_TAGS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setTags(List tags) {
this.tags = tags;
}
public DominoProjectsApiProjectSummary templateDetails(DominoProjectsApiProjectTemplateDetails templateDetails) {
this.templateDetails = templateDetails;
return this;
}
/**
* Get templateDetails
* @return templateDetails
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_TEMPLATE_DETAILS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DominoProjectsApiProjectTemplateDetails getTemplateDetails() {
return templateDetails;
}
@JsonProperty(JSON_PROPERTY_TEMPLATE_DETAILS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTemplateDetails(DominoProjectsApiProjectTemplateDetails templateDetails) {
this.templateDetails = templateDetails;
}
public DominoProjectsApiProjectSummary visibility(String visibility) {
this.visibility = visibility;
return this;
}
/**
* Get visibility
* @return visibility
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_VISIBILITY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getVisibility() {
return visibility;
}
@JsonProperty(JSON_PROPERTY_VISIBILITY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setVisibility(String visibility) {
this.visibility = visibility;
}
/**
* Return true if this domino.projects.api.ProjectSummary object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DominoProjectsApiProjectSummary dominoProjectsApiProjectSummary = (DominoProjectsApiProjectSummary) o;
return Objects.equals(this.billingTag, dominoProjectsApiProjectSummary.billingTag) &&
Objects.equals(this.collaboratorIds, dominoProjectsApiProjectSummary.collaboratorIds) &&
Objects.equals(this.collaborators, dominoProjectsApiProjectSummary.collaborators) &&
Objects.equals(this.created, dominoProjectsApiProjectSummary.created) &&
Objects.equals(this.description, dominoProjectsApiProjectSummary.description) &&
Objects.equals(this.id, dominoProjectsApiProjectSummary.id) &&
Objects.equals(this.importedGitRepositories, dominoProjectsApiProjectSummary.importedGitRepositories) &&
Objects.equals(this.internalTags, dominoProjectsApiProjectSummary.internalTags) &&
Objects.equals(this.mainRepository, dominoProjectsApiProjectSummary.mainRepository) &&
Objects.equals(this.name, dominoProjectsApiProjectSummary.name) &&
Objects.equals(this.ownerId, dominoProjectsApiProjectSummary.ownerId) &&
Objects.equals(this.ownerUsername, dominoProjectsApiProjectSummary.ownerUsername) &&
Objects.equals(this.stageId, dominoProjectsApiProjectSummary.stageId) &&
Objects.equals(this.status, dominoProjectsApiProjectSummary.status) &&
Objects.equals(this.tags, dominoProjectsApiProjectSummary.tags) &&
Objects.equals(this.templateDetails, dominoProjectsApiProjectSummary.templateDetails) &&
Objects.equals(this.visibility, dominoProjectsApiProjectSummary.visibility);
}
@Override
public int hashCode() {
return Objects.hash(billingTag, collaboratorIds, collaborators, created, description, id, importedGitRepositories, internalTags, mainRepository, name, ownerId, ownerUsername, stageId, status, tags, templateDetails, visibility);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DominoProjectsApiProjectSummary {\n");
sb.append(" billingTag: ").append(toIndentedString(billingTag)).append("\n");
sb.append(" collaboratorIds: ").append(toIndentedString(collaboratorIds)).append("\n");
sb.append(" collaborators: ").append(toIndentedString(collaborators)).append("\n");
sb.append(" created: ").append(toIndentedString(created)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" importedGitRepositories: ").append(toIndentedString(importedGitRepositories)).append("\n");
sb.append(" internalTags: ").append(toIndentedString(internalTags)).append("\n");
sb.append(" mainRepository: ").append(toIndentedString(mainRepository)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" ownerId: ").append(toIndentedString(ownerId)).append("\n");
sb.append(" ownerUsername: ").append(toIndentedString(ownerUsername)).append("\n");
sb.append(" stageId: ").append(toIndentedString(stageId)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" tags: ").append(toIndentedString(tags)).append("\n");
sb.append(" templateDetails: ").append(toIndentedString(templateDetails)).append("\n");
sb.append(" visibility: ").append(toIndentedString(visibility)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `billingTag` to the URL query string
if (getBillingTag() != null) {
joiner.add(getBillingTag().toUrlQueryString(prefix + "billingTag" + suffix));
}
// add `collaboratorIds` to the URL query string
if (getCollaboratorIds() != null) {
for (int i = 0; i < getCollaboratorIds().size(); i++) {
joiner.add(String.format("%scollaboratorIds%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(ApiClient.valueToString(getCollaboratorIds().get(i)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
// add `collaborators` to the URL query string
if (getCollaborators() != null) {
for (int i = 0; i < getCollaborators().size(); i++) {
if (getCollaborators().get(i) != null) {
joiner.add(getCollaborators().get(i).toUrlQueryString(String.format("%scollaborators%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `created` to the URL query string
if (getCreated() != null) {
joiner.add(String.format("%screated%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getCreated()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `description` to the URL query string
if (getDescription() != null) {
joiner.add(String.format("%sdescription%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getDescription()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `id` to the URL query string
if (getId() != null) {
joiner.add(String.format("%sid%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `importedGitRepositories` to the URL query string
if (getImportedGitRepositories() != null) {
for (int i = 0; i < getImportedGitRepositories().size(); i++) {
if (getImportedGitRepositories().get(i) != null) {
joiner.add(getImportedGitRepositories().get(i).toUrlQueryString(String.format("%simportedGitRepositories%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `internalTags` to the URL query string
if (getInternalTags() != null) {
for (int i = 0; i < getInternalTags().size(); i++) {
joiner.add(String.format("%sinternalTags%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(ApiClient.valueToString(getInternalTags().get(i)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
// add `mainRepository` to the URL query string
if (getMainRepository() != null) {
joiner.add(getMainRepository().toUrlQueryString(prefix + "mainRepository" + suffix));
}
// add `name` to the URL query string
if (getName() != null) {
joiner.add(String.format("%sname%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getName()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `ownerId` to the URL query string
if (getOwnerId() != null) {
joiner.add(String.format("%sownerId%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getOwnerId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `ownerUsername` to the URL query string
if (getOwnerUsername() != null) {
joiner.add(String.format("%sownerUsername%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getOwnerUsername()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `stageId` to the URL query string
if (getStageId() != null) {
joiner.add(String.format("%sstageId%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getStageId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `status` to the URL query string
if (getStatus() != null) {
joiner.add(getStatus().toUrlQueryString(prefix + "status" + suffix));
}
// add `tags` to the URL query string
if (getTags() != null) {
for (int i = 0; i < getTags().size(); i++) {
if (getTags().get(i) != null) {
joiner.add(getTags().get(i).toUrlQueryString(String.format("%stags%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `templateDetails` to the URL query string
if (getTemplateDetails() != null) {
joiner.add(getTemplateDetails().toUrlQueryString(prefix + "templateDetails" + suffix));
}
// add `visibility` to the URL query string
if (getVisibility() != null) {
joiner.add(String.format("%svisibility%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getVisibility()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy