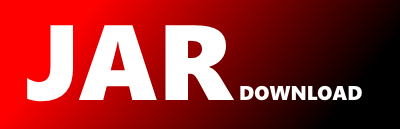
com.dominodatalab.api.model.DominoServerProjectsApiProjectGatewayOverview Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of domino-java-client Show documentation
Show all versions of domino-java-client Show documentation
Domino Data Lab API Client to connect to Domino web services using Java HTTP Client.
/*
* Domino Data Lab API v4
* This API provides access to select Domino functions available in Domino's non-public API. To authenticate your requests, include your API Key (which you can find on your account page) with the header X-Domino-Api-Key.
*
* The version of the OpenAPI document: 4.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.api.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.dominodatalab.api.model.DominoProjectsApiProjectTemplateDetails;
import com.dominodatalab.api.model.DominoRepomanDomainGitRepository;
import com.dominodatalab.api.model.DominoServerProjectsApiMainRepositoryDetails;
import com.dominodatalab.api.model.DominoServerProjectsApiOwner;
import com.dominodatalab.api.model.DominoServerProjectsApiProjectGatewayExecutingRunsByType;
import com.dominodatalab.api.model.DominoServerProjectsApiProjectGatewayOverviewDetails;
import com.dominodatalab.api.model.DominoServerProjectsApiProjectGatewayTag;
import com.dominodatalab.api.model.DominoServerProjectsApiProjectStatus;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.dominodatalab.api.invoker.ApiClient;
/**
* Project Overview entity returned by the API gateway
*/
@JsonPropertyOrder({
DominoServerProjectsApiProjectGatewayOverview.JSON_PROPERTY_ALLOWED_OPERATIONS,
DominoServerProjectsApiProjectGatewayOverview.JSON_PROPERTY_DESCRIPTION,
DominoServerProjectsApiProjectGatewayOverview.JSON_PROPERTY_DETAILS,
DominoServerProjectsApiProjectGatewayOverview.JSON_PROPERTY_ENABLED_GIT_REPOSITORIES,
DominoServerProjectsApiProjectGatewayOverview.JSON_PROPERTY_ENVIRONMENT_NAME,
DominoServerProjectsApiProjectGatewayOverview.JSON_PROPERTY_HARDWARE_TIER_ID,
DominoServerProjectsApiProjectGatewayOverview.JSON_PROPERTY_HARDWARE_TIER_NAME,
DominoServerProjectsApiProjectGatewayOverview.JSON_PROPERTY_ID,
DominoServerProjectsApiProjectGatewayOverview.JSON_PROPERTY_LAST_STAGE_CHANGE_IN_MILLIS,
DominoServerProjectsApiProjectGatewayOverview.JSON_PROPERTY_LAST_STATUS_CHANGE_IN_MILLIS,
DominoServerProjectsApiProjectGatewayOverview.JSON_PROPERTY_MAIN_REPOSITORY,
DominoServerProjectsApiProjectGatewayOverview.JSON_PROPERTY_NAME,
DominoServerProjectsApiProjectGatewayOverview.JSON_PROPERTY_NUM_COMMENTS,
DominoServerProjectsApiProjectGatewayOverview.JSON_PROPERTY_OWNER,
DominoServerProjectsApiProjectGatewayOverview.JSON_PROPERTY_REQUESTING_USER_ROLE,
DominoServerProjectsApiProjectGatewayOverview.JSON_PROPERTY_RUNS_COUNT_BY_TYPE,
DominoServerProjectsApiProjectGatewayOverview.JSON_PROPERTY_STAGE_ID,
DominoServerProjectsApiProjectGatewayOverview.JSON_PROPERTY_STATUS,
DominoServerProjectsApiProjectGatewayOverview.JSON_PROPERTY_TAGS,
DominoServerProjectsApiProjectGatewayOverview.JSON_PROPERTY_TEMPLATE_DETAILS,
DominoServerProjectsApiProjectGatewayOverview.JSON_PROPERTY_TOTAL_RUN_TIME,
DominoServerProjectsApiProjectGatewayOverview.JSON_PROPERTY_UPDATED_AT,
DominoServerProjectsApiProjectGatewayOverview.JSON_PROPERTY_VISIBILITY
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-10-04T16:37:26.309454-04:00[America/New_York]", comments = "Generator version: 7.8.0")
public class DominoServerProjectsApiProjectGatewayOverview {
/**
* Gets or Sets allowedOperations
*/
public enum AllowedOperationsEnum {
CHANGE_PROJECT_SETTINGS("ChangeProjectSettings"),
RUN("Run"),
EDIT("Edit"),
VIEW_WORKSPACES("ViewWorkspaces"),
UPDATE_PROJECT_DESCRIPTION("UpdateProjectDescription"),
PROJECT_SEARCH_PREVIEW("ProjectSearchPreview"),
BROWSE_READ_FILES("BrowseReadFiles"),
EDIT_TAGS("EditTags"),
RUN_LAUNCHER("RunLauncher"),
RUN_LAUNCHERS("RunLaunchers"),
VIEW_RUNS("ViewRuns");
private String value;
AllowedOperationsEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static AllowedOperationsEnum fromValue(String value) {
for (AllowedOperationsEnum b : AllowedOperationsEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_ALLOWED_OPERATIONS = "allowedOperations";
private List allowedOperations = new ArrayList<>();
public static final String JSON_PROPERTY_DESCRIPTION = "description";
private String description;
public static final String JSON_PROPERTY_DETAILS = "details";
private DominoServerProjectsApiProjectGatewayOverviewDetails details;
public static final String JSON_PROPERTY_ENABLED_GIT_REPOSITORIES = "enabledGitRepositories";
private List enabledGitRepositories = new ArrayList<>();
public static final String JSON_PROPERTY_ENVIRONMENT_NAME = "environmentName";
private String environmentName;
public static final String JSON_PROPERTY_HARDWARE_TIER_ID = "hardwareTierId";
private String hardwareTierId;
public static final String JSON_PROPERTY_HARDWARE_TIER_NAME = "hardwareTierName";
private String hardwareTierName;
public static final String JSON_PROPERTY_ID = "id";
private String id;
public static final String JSON_PROPERTY_LAST_STAGE_CHANGE_IN_MILLIS = "lastStageChangeInMillis";
private Long lastStageChangeInMillis;
public static final String JSON_PROPERTY_LAST_STATUS_CHANGE_IN_MILLIS = "lastStatusChangeInMillis";
private Long lastStatusChangeInMillis;
public static final String JSON_PROPERTY_MAIN_REPOSITORY = "mainRepository";
private DominoServerProjectsApiMainRepositoryDetails mainRepository;
public static final String JSON_PROPERTY_NAME = "name";
private String name;
public static final String JSON_PROPERTY_NUM_COMMENTS = "numComments";
private Long numComments;
public static final String JSON_PROPERTY_OWNER = "owner";
private DominoServerProjectsApiOwner owner;
/**
* Gets or Sets requestingUserRole
*/
public enum RequestingUserRoleEnum {
ADMIN("Admin"),
PROJECT_IMPORTER("ProjectImporter"),
CONTRIBUTOR("Contributor"),
VIEWER("Viewer"),
RESULTS_CONSUMER("ResultsConsumer"),
LAUNCHER_USER("LauncherUser"),
OWNER("Owner");
private String value;
RequestingUserRoleEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static RequestingUserRoleEnum fromValue(String value) {
for (RequestingUserRoleEnum b : RequestingUserRoleEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_REQUESTING_USER_ROLE = "requestingUserRole";
private RequestingUserRoleEnum requestingUserRole;
public static final String JSON_PROPERTY_RUNS_COUNT_BY_TYPE = "runsCountByType";
private List runsCountByType = new ArrayList<>();
public static final String JSON_PROPERTY_STAGE_ID = "stageId";
private String stageId;
public static final String JSON_PROPERTY_STATUS = "status";
private DominoServerProjectsApiProjectStatus status;
public static final String JSON_PROPERTY_TAGS = "tags";
private List tags = new ArrayList<>();
public static final String JSON_PROPERTY_TEMPLATE_DETAILS = "templateDetails";
private DominoProjectsApiProjectTemplateDetails templateDetails;
public static final String JSON_PROPERTY_TOTAL_RUN_TIME = "totalRunTime";
private String totalRunTime;
public static final String JSON_PROPERTY_UPDATED_AT = "updatedAt";
private OffsetDateTime updatedAt;
/**
* Gets or Sets visibility
*/
public enum VisibilityEnum {
PUBLIC("Public"),
SEARCHABLE("Searchable"),
PRIVATE("Private");
private String value;
VisibilityEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static VisibilityEnum fromValue(String value) {
for (VisibilityEnum b : VisibilityEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_VISIBILITY = "visibility";
private VisibilityEnum visibility;
public DominoServerProjectsApiProjectGatewayOverview() {
}
public DominoServerProjectsApiProjectGatewayOverview allowedOperations(List allowedOperations) {
this.allowedOperations = allowedOperations;
return this;
}
public DominoServerProjectsApiProjectGatewayOverview addAllowedOperationsItem(AllowedOperationsEnum allowedOperationsItem) {
if (this.allowedOperations == null) {
this.allowedOperations = new ArrayList<>();
}
this.allowedOperations.add(allowedOperationsItem);
return this;
}
/**
* Get allowedOperations
* @return allowedOperations
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ALLOWED_OPERATIONS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getAllowedOperations() {
return allowedOperations;
}
@JsonProperty(JSON_PROPERTY_ALLOWED_OPERATIONS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setAllowedOperations(List allowedOperations) {
this.allowedOperations = allowedOperations;
}
public DominoServerProjectsApiProjectGatewayOverview description(String description) {
this.description = description;
return this;
}
/**
* Get description
* @return description
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getDescription() {
return description;
}
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDescription(String description) {
this.description = description;
}
public DominoServerProjectsApiProjectGatewayOverview details(DominoServerProjectsApiProjectGatewayOverviewDetails details) {
this.details = details;
return this;
}
/**
* Get details
* @return details
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DETAILS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DominoServerProjectsApiProjectGatewayOverviewDetails getDetails() {
return details;
}
@JsonProperty(JSON_PROPERTY_DETAILS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDetails(DominoServerProjectsApiProjectGatewayOverviewDetails details) {
this.details = details;
}
public DominoServerProjectsApiProjectGatewayOverview enabledGitRepositories(List enabledGitRepositories) {
this.enabledGitRepositories = enabledGitRepositories;
return this;
}
public DominoServerProjectsApiProjectGatewayOverview addEnabledGitRepositoriesItem(DominoRepomanDomainGitRepository enabledGitRepositoriesItem) {
if (this.enabledGitRepositories == null) {
this.enabledGitRepositories = new ArrayList<>();
}
this.enabledGitRepositories.add(enabledGitRepositoriesItem);
return this;
}
/**
* Get enabledGitRepositories
* @return enabledGitRepositories
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_ENABLED_GIT_REPOSITORIES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getEnabledGitRepositories() {
return enabledGitRepositories;
}
@JsonProperty(JSON_PROPERTY_ENABLED_GIT_REPOSITORIES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEnabledGitRepositories(List enabledGitRepositories) {
this.enabledGitRepositories = enabledGitRepositories;
}
public DominoServerProjectsApiProjectGatewayOverview environmentName(String environmentName) {
this.environmentName = environmentName;
return this;
}
/**
* Get environmentName
* @return environmentName
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ENVIRONMENT_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getEnvironmentName() {
return environmentName;
}
@JsonProperty(JSON_PROPERTY_ENVIRONMENT_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setEnvironmentName(String environmentName) {
this.environmentName = environmentName;
}
public DominoServerProjectsApiProjectGatewayOverview hardwareTierId(String hardwareTierId) {
this.hardwareTierId = hardwareTierId;
return this;
}
/**
* Get hardwareTierId
* @return hardwareTierId
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_HARDWARE_TIER_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getHardwareTierId() {
return hardwareTierId;
}
@JsonProperty(JSON_PROPERTY_HARDWARE_TIER_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setHardwareTierId(String hardwareTierId) {
this.hardwareTierId = hardwareTierId;
}
public DominoServerProjectsApiProjectGatewayOverview hardwareTierName(String hardwareTierName) {
this.hardwareTierName = hardwareTierName;
return this;
}
/**
* Get hardwareTierName
* @return hardwareTierName
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_HARDWARE_TIER_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getHardwareTierName() {
return hardwareTierName;
}
@JsonProperty(JSON_PROPERTY_HARDWARE_TIER_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setHardwareTierName(String hardwareTierName) {
this.hardwareTierName = hardwareTierName;
}
public DominoServerProjectsApiProjectGatewayOverview id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getId() {
return id;
}
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setId(String id) {
this.id = id;
}
public DominoServerProjectsApiProjectGatewayOverview lastStageChangeInMillis(Long lastStageChangeInMillis) {
this.lastStageChangeInMillis = lastStageChangeInMillis;
return this;
}
/**
* Get lastStageChangeInMillis
* @return lastStageChangeInMillis
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_LAST_STAGE_CHANGE_IN_MILLIS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getLastStageChangeInMillis() {
return lastStageChangeInMillis;
}
@JsonProperty(JSON_PROPERTY_LAST_STAGE_CHANGE_IN_MILLIS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLastStageChangeInMillis(Long lastStageChangeInMillis) {
this.lastStageChangeInMillis = lastStageChangeInMillis;
}
public DominoServerProjectsApiProjectGatewayOverview lastStatusChangeInMillis(Long lastStatusChangeInMillis) {
this.lastStatusChangeInMillis = lastStatusChangeInMillis;
return this;
}
/**
* Get lastStatusChangeInMillis
* @return lastStatusChangeInMillis
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_LAST_STATUS_CHANGE_IN_MILLIS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getLastStatusChangeInMillis() {
return lastStatusChangeInMillis;
}
@JsonProperty(JSON_PROPERTY_LAST_STATUS_CHANGE_IN_MILLIS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLastStatusChangeInMillis(Long lastStatusChangeInMillis) {
this.lastStatusChangeInMillis = lastStatusChangeInMillis;
}
public DominoServerProjectsApiProjectGatewayOverview mainRepository(DominoServerProjectsApiMainRepositoryDetails mainRepository) {
this.mainRepository = mainRepository;
return this;
}
/**
* Get mainRepository
* @return mainRepository
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_MAIN_REPOSITORY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DominoServerProjectsApiMainRepositoryDetails getMainRepository() {
return mainRepository;
}
@JsonProperty(JSON_PROPERTY_MAIN_REPOSITORY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMainRepository(DominoServerProjectsApiMainRepositoryDetails mainRepository) {
this.mainRepository = mainRepository;
}
public DominoServerProjectsApiProjectGatewayOverview name(String name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getName() {
return name;
}
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setName(String name) {
this.name = name;
}
public DominoServerProjectsApiProjectGatewayOverview numComments(Long numComments) {
this.numComments = numComments;
return this;
}
/**
* Get numComments
* @return numComments
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_NUM_COMMENTS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Long getNumComments() {
return numComments;
}
@JsonProperty(JSON_PROPERTY_NUM_COMMENTS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setNumComments(Long numComments) {
this.numComments = numComments;
}
public DominoServerProjectsApiProjectGatewayOverview owner(DominoServerProjectsApiOwner owner) {
this.owner = owner;
return this;
}
/**
* Get owner
* @return owner
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_OWNER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public DominoServerProjectsApiOwner getOwner() {
return owner;
}
@JsonProperty(JSON_PROPERTY_OWNER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setOwner(DominoServerProjectsApiOwner owner) {
this.owner = owner;
}
public DominoServerProjectsApiProjectGatewayOverview requestingUserRole(RequestingUserRoleEnum requestingUserRole) {
this.requestingUserRole = requestingUserRole;
return this;
}
/**
* Get requestingUserRole
* @return requestingUserRole
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_REQUESTING_USER_ROLE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public RequestingUserRoleEnum getRequestingUserRole() {
return requestingUserRole;
}
@JsonProperty(JSON_PROPERTY_REQUESTING_USER_ROLE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setRequestingUserRole(RequestingUserRoleEnum requestingUserRole) {
this.requestingUserRole = requestingUserRole;
}
public DominoServerProjectsApiProjectGatewayOverview runsCountByType(List runsCountByType) {
this.runsCountByType = runsCountByType;
return this;
}
public DominoServerProjectsApiProjectGatewayOverview addRunsCountByTypeItem(DominoServerProjectsApiProjectGatewayExecutingRunsByType runsCountByTypeItem) {
if (this.runsCountByType == null) {
this.runsCountByType = new ArrayList<>();
}
this.runsCountByType.add(runsCountByTypeItem);
return this;
}
/**
* Get runsCountByType
* @return runsCountByType
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_RUNS_COUNT_BY_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getRunsCountByType() {
return runsCountByType;
}
@JsonProperty(JSON_PROPERTY_RUNS_COUNT_BY_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setRunsCountByType(List runsCountByType) {
this.runsCountByType = runsCountByType;
}
public DominoServerProjectsApiProjectGatewayOverview stageId(String stageId) {
this.stageId = stageId;
return this;
}
/**
* Get stageId
* @return stageId
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_STAGE_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getStageId() {
return stageId;
}
@JsonProperty(JSON_PROPERTY_STAGE_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setStageId(String stageId) {
this.stageId = stageId;
}
public DominoServerProjectsApiProjectGatewayOverview status(DominoServerProjectsApiProjectStatus status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public DominoServerProjectsApiProjectStatus getStatus() {
return status;
}
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setStatus(DominoServerProjectsApiProjectStatus status) {
this.status = status;
}
public DominoServerProjectsApiProjectGatewayOverview tags(List tags) {
this.tags = tags;
return this;
}
public DominoServerProjectsApiProjectGatewayOverview addTagsItem(DominoServerProjectsApiProjectGatewayTag tagsItem) {
if (this.tags == null) {
this.tags = new ArrayList<>();
}
this.tags.add(tagsItem);
return this;
}
/**
* Get tags
* @return tags
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_TAGS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getTags() {
return tags;
}
@JsonProperty(JSON_PROPERTY_TAGS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setTags(List tags) {
this.tags = tags;
}
public DominoServerProjectsApiProjectGatewayOverview templateDetails(DominoProjectsApiProjectTemplateDetails templateDetails) {
this.templateDetails = templateDetails;
return this;
}
/**
* Get templateDetails
* @return templateDetails
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_TEMPLATE_DETAILS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DominoProjectsApiProjectTemplateDetails getTemplateDetails() {
return templateDetails;
}
@JsonProperty(JSON_PROPERTY_TEMPLATE_DETAILS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTemplateDetails(DominoProjectsApiProjectTemplateDetails templateDetails) {
this.templateDetails = templateDetails;
}
public DominoServerProjectsApiProjectGatewayOverview totalRunTime(String totalRunTime) {
this.totalRunTime = totalRunTime;
return this;
}
/**
* sum of run times of all executions in the project, in ISO8601 duration format including only seconds and milliseconds
* @return totalRunTime
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_TOTAL_RUN_TIME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getTotalRunTime() {
return totalRunTime;
}
@JsonProperty(JSON_PROPERTY_TOTAL_RUN_TIME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setTotalRunTime(String totalRunTime) {
this.totalRunTime = totalRunTime;
}
public DominoServerProjectsApiProjectGatewayOverview updatedAt(OffsetDateTime updatedAt) {
this.updatedAt = updatedAt;
return this;
}
/**
* Get updatedAt
* @return updatedAt
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_UPDATED_AT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public OffsetDateTime getUpdatedAt() {
return updatedAt;
}
@JsonProperty(JSON_PROPERTY_UPDATED_AT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setUpdatedAt(OffsetDateTime updatedAt) {
this.updatedAt = updatedAt;
}
public DominoServerProjectsApiProjectGatewayOverview visibility(VisibilityEnum visibility) {
this.visibility = visibility;
return this;
}
/**
* Get visibility
* @return visibility
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_VISIBILITY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public VisibilityEnum getVisibility() {
return visibility;
}
@JsonProperty(JSON_PROPERTY_VISIBILITY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setVisibility(VisibilityEnum visibility) {
this.visibility = visibility;
}
/**
* Return true if this domino.server.projects.api.ProjectGatewayOverview object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DominoServerProjectsApiProjectGatewayOverview dominoServerProjectsApiProjectGatewayOverview = (DominoServerProjectsApiProjectGatewayOverview) o;
return Objects.equals(this.allowedOperations, dominoServerProjectsApiProjectGatewayOverview.allowedOperations) &&
Objects.equals(this.description, dominoServerProjectsApiProjectGatewayOverview.description) &&
Objects.equals(this.details, dominoServerProjectsApiProjectGatewayOverview.details) &&
Objects.equals(this.enabledGitRepositories, dominoServerProjectsApiProjectGatewayOverview.enabledGitRepositories) &&
Objects.equals(this.environmentName, dominoServerProjectsApiProjectGatewayOverview.environmentName) &&
Objects.equals(this.hardwareTierId, dominoServerProjectsApiProjectGatewayOverview.hardwareTierId) &&
Objects.equals(this.hardwareTierName, dominoServerProjectsApiProjectGatewayOverview.hardwareTierName) &&
Objects.equals(this.id, dominoServerProjectsApiProjectGatewayOverview.id) &&
Objects.equals(this.lastStageChangeInMillis, dominoServerProjectsApiProjectGatewayOverview.lastStageChangeInMillis) &&
Objects.equals(this.lastStatusChangeInMillis, dominoServerProjectsApiProjectGatewayOverview.lastStatusChangeInMillis) &&
Objects.equals(this.mainRepository, dominoServerProjectsApiProjectGatewayOverview.mainRepository) &&
Objects.equals(this.name, dominoServerProjectsApiProjectGatewayOverview.name) &&
Objects.equals(this.numComments, dominoServerProjectsApiProjectGatewayOverview.numComments) &&
Objects.equals(this.owner, dominoServerProjectsApiProjectGatewayOverview.owner) &&
Objects.equals(this.requestingUserRole, dominoServerProjectsApiProjectGatewayOverview.requestingUserRole) &&
Objects.equals(this.runsCountByType, dominoServerProjectsApiProjectGatewayOverview.runsCountByType) &&
Objects.equals(this.stageId, dominoServerProjectsApiProjectGatewayOverview.stageId) &&
Objects.equals(this.status, dominoServerProjectsApiProjectGatewayOverview.status) &&
Objects.equals(this.tags, dominoServerProjectsApiProjectGatewayOverview.tags) &&
Objects.equals(this.templateDetails, dominoServerProjectsApiProjectGatewayOverview.templateDetails) &&
Objects.equals(this.totalRunTime, dominoServerProjectsApiProjectGatewayOverview.totalRunTime) &&
Objects.equals(this.updatedAt, dominoServerProjectsApiProjectGatewayOverview.updatedAt) &&
Objects.equals(this.visibility, dominoServerProjectsApiProjectGatewayOverview.visibility);
}
@Override
public int hashCode() {
return Objects.hash(allowedOperations, description, details, enabledGitRepositories, environmentName, hardwareTierId, hardwareTierName, id, lastStageChangeInMillis, lastStatusChangeInMillis, mainRepository, name, numComments, owner, requestingUserRole, runsCountByType, stageId, status, tags, templateDetails, totalRunTime, updatedAt, visibility);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DominoServerProjectsApiProjectGatewayOverview {\n");
sb.append(" allowedOperations: ").append(toIndentedString(allowedOperations)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" details: ").append(toIndentedString(details)).append("\n");
sb.append(" enabledGitRepositories: ").append(toIndentedString(enabledGitRepositories)).append("\n");
sb.append(" environmentName: ").append(toIndentedString(environmentName)).append("\n");
sb.append(" hardwareTierId: ").append(toIndentedString(hardwareTierId)).append("\n");
sb.append(" hardwareTierName: ").append(toIndentedString(hardwareTierName)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" lastStageChangeInMillis: ").append(toIndentedString(lastStageChangeInMillis)).append("\n");
sb.append(" lastStatusChangeInMillis: ").append(toIndentedString(lastStatusChangeInMillis)).append("\n");
sb.append(" mainRepository: ").append(toIndentedString(mainRepository)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" numComments: ").append(toIndentedString(numComments)).append("\n");
sb.append(" owner: ").append(toIndentedString(owner)).append("\n");
sb.append(" requestingUserRole: ").append(toIndentedString(requestingUserRole)).append("\n");
sb.append(" runsCountByType: ").append(toIndentedString(runsCountByType)).append("\n");
sb.append(" stageId: ").append(toIndentedString(stageId)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" tags: ").append(toIndentedString(tags)).append("\n");
sb.append(" templateDetails: ").append(toIndentedString(templateDetails)).append("\n");
sb.append(" totalRunTime: ").append(toIndentedString(totalRunTime)).append("\n");
sb.append(" updatedAt: ").append(toIndentedString(updatedAt)).append("\n");
sb.append(" visibility: ").append(toIndentedString(visibility)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `allowedOperations` to the URL query string
if (getAllowedOperations() != null) {
for (int i = 0; i < getAllowedOperations().size(); i++) {
joiner.add(String.format("%sallowedOperations%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(ApiClient.valueToString(getAllowedOperations().get(i)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
// add `description` to the URL query string
if (getDescription() != null) {
joiner.add(String.format("%sdescription%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getDescription()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `details` to the URL query string
if (getDetails() != null) {
joiner.add(getDetails().toUrlQueryString(prefix + "details" + suffix));
}
// add `enabledGitRepositories` to the URL query string
if (getEnabledGitRepositories() != null) {
for (int i = 0; i < getEnabledGitRepositories().size(); i++) {
if (getEnabledGitRepositories().get(i) != null) {
joiner.add(getEnabledGitRepositories().get(i).toUrlQueryString(String.format("%senabledGitRepositories%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `environmentName` to the URL query string
if (getEnvironmentName() != null) {
joiner.add(String.format("%senvironmentName%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getEnvironmentName()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `hardwareTierId` to the URL query string
if (getHardwareTierId() != null) {
joiner.add(String.format("%shardwareTierId%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getHardwareTierId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `hardwareTierName` to the URL query string
if (getHardwareTierName() != null) {
joiner.add(String.format("%shardwareTierName%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getHardwareTierName()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `id` to the URL query string
if (getId() != null) {
joiner.add(String.format("%sid%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `lastStageChangeInMillis` to the URL query string
if (getLastStageChangeInMillis() != null) {
joiner.add(String.format("%slastStageChangeInMillis%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getLastStageChangeInMillis()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `lastStatusChangeInMillis` to the URL query string
if (getLastStatusChangeInMillis() != null) {
joiner.add(String.format("%slastStatusChangeInMillis%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getLastStatusChangeInMillis()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `mainRepository` to the URL query string
if (getMainRepository() != null) {
joiner.add(getMainRepository().toUrlQueryString(prefix + "mainRepository" + suffix));
}
// add `name` to the URL query string
if (getName() != null) {
joiner.add(String.format("%sname%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getName()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `numComments` to the URL query string
if (getNumComments() != null) {
joiner.add(String.format("%snumComments%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getNumComments()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `owner` to the URL query string
if (getOwner() != null) {
joiner.add(getOwner().toUrlQueryString(prefix + "owner" + suffix));
}
// add `requestingUserRole` to the URL query string
if (getRequestingUserRole() != null) {
joiner.add(String.format("%srequestingUserRole%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getRequestingUserRole()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `runsCountByType` to the URL query string
if (getRunsCountByType() != null) {
for (int i = 0; i < getRunsCountByType().size(); i++) {
if (getRunsCountByType().get(i) != null) {
joiner.add(getRunsCountByType().get(i).toUrlQueryString(String.format("%srunsCountByType%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `stageId` to the URL query string
if (getStageId() != null) {
joiner.add(String.format("%sstageId%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getStageId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `status` to the URL query string
if (getStatus() != null) {
joiner.add(getStatus().toUrlQueryString(prefix + "status" + suffix));
}
// add `tags` to the URL query string
if (getTags() != null) {
for (int i = 0; i < getTags().size(); i++) {
if (getTags().get(i) != null) {
joiner.add(getTags().get(i).toUrlQueryString(String.format("%stags%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `templateDetails` to the URL query string
if (getTemplateDetails() != null) {
joiner.add(getTemplateDetails().toUrlQueryString(prefix + "templateDetails" + suffix));
}
// add `totalRunTime` to the URL query string
if (getTotalRunTime() != null) {
joiner.add(String.format("%stotalRunTime%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getTotalRunTime()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `updatedAt` to the URL query string
if (getUpdatedAt() != null) {
joiner.add(String.format("%supdatedAt%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getUpdatedAt()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `visibility` to the URL query string
if (getVisibility() != null) {
joiner.add(String.format("%svisibility%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getVisibility()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy