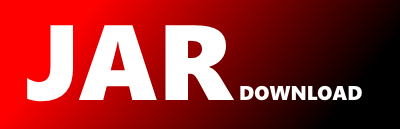
com.dominodatalab.api.model.DominoServerProjectsApiProjectGatewaySummary Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of domino-java-client Show documentation
Show all versions of domino-java-client Show documentation
Domino Data Lab API Client to connect to Domino web services using Java HTTP Client.
/*
* Domino Data Lab API v4
* This API provides access to select Domino functions available in Domino's non-public API. To authenticate your requests, include your API Key (which you can find on your account page) with the header X-Domino-Api-Key.
*
* The version of the OpenAPI document: 4.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.api.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.dominodatalab.api.model.DominoServerProjectsApiProjectGatewayOverviewDetails;
import com.dominodatalab.api.model.DominoServerProjectsApiProjectGatewayRunCountForType;
import com.dominodatalab.api.model.DominoServerProjectsApiProjectGatewayTag;
import com.dominodatalab.api.model.DominoServerProjectsApiProjectStatus;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.dominodatalab.api.invoker.ApiClient;
/**
* DominoServerProjectsApiProjectGatewaySummary
*/
@JsonPropertyOrder({
DominoServerProjectsApiProjectGatewaySummary.JSON_PROPERTY_DESCRIPTION,
DominoServerProjectsApiProjectGatewaySummary.JSON_PROPERTY_DETAILS,
DominoServerProjectsApiProjectGatewaySummary.JSON_PROPERTY_ID,
DominoServerProjectsApiProjectGatewaySummary.JSON_PROPERTY_IS_PINNED,
DominoServerProjectsApiProjectGatewaySummary.JSON_PROPERTY_LATEST_RESULT_RUN_ID,
DominoServerProjectsApiProjectGatewaySummary.JSON_PROPERTY_LATEST_RESULT_TIMESTAMP,
DominoServerProjectsApiProjectGatewaySummary.JSON_PROPERTY_MAIN_GIT_REPOSITORY_URI,
DominoServerProjectsApiProjectGatewaySummary.JSON_PROPERTY_NAME,
DominoServerProjectsApiProjectGatewaySummary.JSON_PROPERTY_OWNER_ID,
DominoServerProjectsApiProjectGatewaySummary.JSON_PROPERTY_OWNER_NAME,
DominoServerProjectsApiProjectGatewaySummary.JSON_PROPERTY_PROJECT_TYPE,
DominoServerProjectsApiProjectGatewaySummary.JSON_PROPERTY_RELATIONSHIP,
DominoServerProjectsApiProjectGatewaySummary.JSON_PROPERTY_RUN_COUNTS,
DominoServerProjectsApiProjectGatewaySummary.JSON_PROPERTY_SERVICE_PROVIDER,
DominoServerProjectsApiProjectGatewaySummary.JSON_PROPERTY_STAGE_ID,
DominoServerProjectsApiProjectGatewaySummary.JSON_PROPERTY_STATUS,
DominoServerProjectsApiProjectGatewaySummary.JSON_PROPERTY_TAGS,
DominoServerProjectsApiProjectGatewaySummary.JSON_PROPERTY_VISIBILITY
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-10-04T16:37:26.309454-04:00[America/New_York]", comments = "Generator version: 7.8.0")
public class DominoServerProjectsApiProjectGatewaySummary {
public static final String JSON_PROPERTY_DESCRIPTION = "description";
private String description;
public static final String JSON_PROPERTY_DETAILS = "details";
private DominoServerProjectsApiProjectGatewayOverviewDetails details;
public static final String JSON_PROPERTY_ID = "id";
private String id;
public static final String JSON_PROPERTY_IS_PINNED = "isPinned";
private Boolean isPinned;
public static final String JSON_PROPERTY_LATEST_RESULT_RUN_ID = "latestResultRunId";
private String latestResultRunId;
public static final String JSON_PROPERTY_LATEST_RESULT_TIMESTAMP = "latestResultTimestamp";
private OffsetDateTime latestResultTimestamp;
public static final String JSON_PROPERTY_MAIN_GIT_REPOSITORY_URI = "mainGitRepositoryUri";
private String mainGitRepositoryUri;
public static final String JSON_PROPERTY_NAME = "name";
private String name;
public static final String JSON_PROPERTY_OWNER_ID = "ownerId";
private String ownerId;
public static final String JSON_PROPERTY_OWNER_NAME = "ownerName";
private String ownerName;
/**
* Gets or Sets projectType
*/
public enum ProjectTypeEnum {
ANALYTIC("Analytic"),
DATA_SET("DataSet"),
TEMPLATE("Template");
private String value;
ProjectTypeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static ProjectTypeEnum fromValue(String value) {
for (ProjectTypeEnum b : ProjectTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_PROJECT_TYPE = "projectType";
private ProjectTypeEnum projectType;
/**
* Gets or Sets relationship
*/
public enum RelationshipEnum {
COLLABORATING("Collaborating"),
POPULAR("Popular"),
OWNED_AND_COLLABORATING("OwnedAndCollaborating"),
SUGGESTED("Suggested"),
OWNED("Owned");
private String value;
RelationshipEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static RelationshipEnum fromValue(String value) {
for (RelationshipEnum b : RelationshipEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_RELATIONSHIP = "relationship";
private RelationshipEnum relationship;
public static final String JSON_PROPERTY_RUN_COUNTS = "runCounts";
private List runCounts = new ArrayList<>();
/**
* Gets or Sets serviceProvider
*/
public enum ServiceProviderEnum {
GITHUB("github"),
GITLAB("gitlab"),
GITHUB_ENTERPRISE("githubEnterprise"),
UNKNOWN("unknown"),
GITLAB_ENTERPRISE("gitlabEnterprise"),
BITBUCKET("bitbucket"),
BITBUCKET_SERVER("bitbucketServer");
private String value;
ServiceProviderEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static ServiceProviderEnum fromValue(String value) {
for (ServiceProviderEnum b : ServiceProviderEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return null;
}
}
public static final String JSON_PROPERTY_SERVICE_PROVIDER = "serviceProvider";
private ServiceProviderEnum serviceProvider;
public static final String JSON_PROPERTY_STAGE_ID = "stageId";
private String stageId;
public static final String JSON_PROPERTY_STATUS = "status";
private DominoServerProjectsApiProjectStatus status;
public static final String JSON_PROPERTY_TAGS = "tags";
private List tags = new ArrayList<>();
/**
* Gets or Sets visibility
*/
public enum VisibilityEnum {
PUBLIC("Public"),
SEARCHABLE("Searchable"),
PRIVATE("Private");
private String value;
VisibilityEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static VisibilityEnum fromValue(String value) {
for (VisibilityEnum b : VisibilityEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_VISIBILITY = "visibility";
private VisibilityEnum visibility;
public DominoServerProjectsApiProjectGatewaySummary() {
}
public DominoServerProjectsApiProjectGatewaySummary description(String description) {
this.description = description;
return this;
}
/**
* Get description
* @return description
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getDescription() {
return description;
}
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDescription(String description) {
this.description = description;
}
public DominoServerProjectsApiProjectGatewaySummary details(DominoServerProjectsApiProjectGatewayOverviewDetails details) {
this.details = details;
return this;
}
/**
* Get details
* @return details
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_DETAILS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public DominoServerProjectsApiProjectGatewayOverviewDetails getDetails() {
return details;
}
@JsonProperty(JSON_PROPERTY_DETAILS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDetails(DominoServerProjectsApiProjectGatewayOverviewDetails details) {
this.details = details;
}
public DominoServerProjectsApiProjectGatewaySummary id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getId() {
return id;
}
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setId(String id) {
this.id = id;
}
public DominoServerProjectsApiProjectGatewaySummary isPinned(Boolean isPinned) {
this.isPinned = isPinned;
return this;
}
/**
* Get isPinned
* @return isPinned
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_IS_PINNED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getIsPinned() {
return isPinned;
}
@JsonProperty(JSON_PROPERTY_IS_PINNED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIsPinned(Boolean isPinned) {
this.isPinned = isPinned;
}
public DominoServerProjectsApiProjectGatewaySummary latestResultRunId(String latestResultRunId) {
this.latestResultRunId = latestResultRunId;
return this;
}
/**
* Get latestResultRunId
* @return latestResultRunId
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_LATEST_RESULT_RUN_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getLatestResultRunId() {
return latestResultRunId;
}
@JsonProperty(JSON_PROPERTY_LATEST_RESULT_RUN_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLatestResultRunId(String latestResultRunId) {
this.latestResultRunId = latestResultRunId;
}
public DominoServerProjectsApiProjectGatewaySummary latestResultTimestamp(OffsetDateTime latestResultTimestamp) {
this.latestResultTimestamp = latestResultTimestamp;
return this;
}
/**
* Get latestResultTimestamp
* @return latestResultTimestamp
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_LATEST_RESULT_TIMESTAMP)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getLatestResultTimestamp() {
return latestResultTimestamp;
}
@JsonProperty(JSON_PROPERTY_LATEST_RESULT_TIMESTAMP)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLatestResultTimestamp(OffsetDateTime latestResultTimestamp) {
this.latestResultTimestamp = latestResultTimestamp;
}
public DominoServerProjectsApiProjectGatewaySummary mainGitRepositoryUri(String mainGitRepositoryUri) {
this.mainGitRepositoryUri = mainGitRepositoryUri;
return this;
}
/**
* Get mainGitRepositoryUri
* @return mainGitRepositoryUri
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_MAIN_GIT_REPOSITORY_URI)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getMainGitRepositoryUri() {
return mainGitRepositoryUri;
}
@JsonProperty(JSON_PROPERTY_MAIN_GIT_REPOSITORY_URI)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMainGitRepositoryUri(String mainGitRepositoryUri) {
this.mainGitRepositoryUri = mainGitRepositoryUri;
}
public DominoServerProjectsApiProjectGatewaySummary name(String name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getName() {
return name;
}
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setName(String name) {
this.name = name;
}
public DominoServerProjectsApiProjectGatewaySummary ownerId(String ownerId) {
this.ownerId = ownerId;
return this;
}
/**
* Get ownerId
* @return ownerId
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_OWNER_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getOwnerId() {
return ownerId;
}
@JsonProperty(JSON_PROPERTY_OWNER_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setOwnerId(String ownerId) {
this.ownerId = ownerId;
}
public DominoServerProjectsApiProjectGatewaySummary ownerName(String ownerName) {
this.ownerName = ownerName;
return this;
}
/**
* Get ownerName
* @return ownerName
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_OWNER_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getOwnerName() {
return ownerName;
}
@JsonProperty(JSON_PROPERTY_OWNER_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setOwnerName(String ownerName) {
this.ownerName = ownerName;
}
public DominoServerProjectsApiProjectGatewaySummary projectType(ProjectTypeEnum projectType) {
this.projectType = projectType;
return this;
}
/**
* Get projectType
* @return projectType
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_PROJECT_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public ProjectTypeEnum getProjectType() {
return projectType;
}
@JsonProperty(JSON_PROPERTY_PROJECT_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setProjectType(ProjectTypeEnum projectType) {
this.projectType = projectType;
}
public DominoServerProjectsApiProjectGatewaySummary relationship(RelationshipEnum relationship) {
this.relationship = relationship;
return this;
}
/**
* Get relationship
* @return relationship
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_RELATIONSHIP)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public RelationshipEnum getRelationship() {
return relationship;
}
@JsonProperty(JSON_PROPERTY_RELATIONSHIP)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setRelationship(RelationshipEnum relationship) {
this.relationship = relationship;
}
public DominoServerProjectsApiProjectGatewaySummary runCounts(List runCounts) {
this.runCounts = runCounts;
return this;
}
public DominoServerProjectsApiProjectGatewaySummary addRunCountsItem(DominoServerProjectsApiProjectGatewayRunCountForType runCountsItem) {
if (this.runCounts == null) {
this.runCounts = new ArrayList<>();
}
this.runCounts.add(runCountsItem);
return this;
}
/**
* Get runCounts
* @return runCounts
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_RUN_COUNTS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getRunCounts() {
return runCounts;
}
@JsonProperty(JSON_PROPERTY_RUN_COUNTS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setRunCounts(List runCounts) {
this.runCounts = runCounts;
}
public DominoServerProjectsApiProjectGatewaySummary serviceProvider(ServiceProviderEnum serviceProvider) {
this.serviceProvider = serviceProvider;
return this;
}
/**
* Get serviceProvider
* @return serviceProvider
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_SERVICE_PROVIDER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ServiceProviderEnum getServiceProvider() {
return serviceProvider;
}
@JsonProperty(JSON_PROPERTY_SERVICE_PROVIDER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setServiceProvider(ServiceProviderEnum serviceProvider) {
this.serviceProvider = serviceProvider;
}
public DominoServerProjectsApiProjectGatewaySummary stageId(String stageId) {
this.stageId = stageId;
return this;
}
/**
* Get stageId
* @return stageId
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_STAGE_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getStageId() {
return stageId;
}
@JsonProperty(JSON_PROPERTY_STAGE_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setStageId(String stageId) {
this.stageId = stageId;
}
public DominoServerProjectsApiProjectGatewaySummary status(DominoServerProjectsApiProjectStatus status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public DominoServerProjectsApiProjectStatus getStatus() {
return status;
}
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setStatus(DominoServerProjectsApiProjectStatus status) {
this.status = status;
}
public DominoServerProjectsApiProjectGatewaySummary tags(List tags) {
this.tags = tags;
return this;
}
public DominoServerProjectsApiProjectGatewaySummary addTagsItem(DominoServerProjectsApiProjectGatewayTag tagsItem) {
if (this.tags == null) {
this.tags = new ArrayList<>();
}
this.tags.add(tagsItem);
return this;
}
/**
* Get tags
* @return tags
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_TAGS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getTags() {
return tags;
}
@JsonProperty(JSON_PROPERTY_TAGS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setTags(List tags) {
this.tags = tags;
}
public DominoServerProjectsApiProjectGatewaySummary visibility(VisibilityEnum visibility) {
this.visibility = visibility;
return this;
}
/**
* Get visibility
* @return visibility
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_VISIBILITY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public VisibilityEnum getVisibility() {
return visibility;
}
@JsonProperty(JSON_PROPERTY_VISIBILITY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setVisibility(VisibilityEnum visibility) {
this.visibility = visibility;
}
/**
* Return true if this domino.server.projects.api.ProjectGatewaySummary object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DominoServerProjectsApiProjectGatewaySummary dominoServerProjectsApiProjectGatewaySummary = (DominoServerProjectsApiProjectGatewaySummary) o;
return Objects.equals(this.description, dominoServerProjectsApiProjectGatewaySummary.description) &&
Objects.equals(this.details, dominoServerProjectsApiProjectGatewaySummary.details) &&
Objects.equals(this.id, dominoServerProjectsApiProjectGatewaySummary.id) &&
Objects.equals(this.isPinned, dominoServerProjectsApiProjectGatewaySummary.isPinned) &&
Objects.equals(this.latestResultRunId, dominoServerProjectsApiProjectGatewaySummary.latestResultRunId) &&
Objects.equals(this.latestResultTimestamp, dominoServerProjectsApiProjectGatewaySummary.latestResultTimestamp) &&
Objects.equals(this.mainGitRepositoryUri, dominoServerProjectsApiProjectGatewaySummary.mainGitRepositoryUri) &&
Objects.equals(this.name, dominoServerProjectsApiProjectGatewaySummary.name) &&
Objects.equals(this.ownerId, dominoServerProjectsApiProjectGatewaySummary.ownerId) &&
Objects.equals(this.ownerName, dominoServerProjectsApiProjectGatewaySummary.ownerName) &&
Objects.equals(this.projectType, dominoServerProjectsApiProjectGatewaySummary.projectType) &&
Objects.equals(this.relationship, dominoServerProjectsApiProjectGatewaySummary.relationship) &&
Objects.equals(this.runCounts, dominoServerProjectsApiProjectGatewaySummary.runCounts) &&
Objects.equals(this.serviceProvider, dominoServerProjectsApiProjectGatewaySummary.serviceProvider) &&
Objects.equals(this.stageId, dominoServerProjectsApiProjectGatewaySummary.stageId) &&
Objects.equals(this.status, dominoServerProjectsApiProjectGatewaySummary.status) &&
Objects.equals(this.tags, dominoServerProjectsApiProjectGatewaySummary.tags) &&
Objects.equals(this.visibility, dominoServerProjectsApiProjectGatewaySummary.visibility);
}
@Override
public int hashCode() {
return Objects.hash(description, details, id, isPinned, latestResultRunId, latestResultTimestamp, mainGitRepositoryUri, name, ownerId, ownerName, projectType, relationship, runCounts, serviceProvider, stageId, status, tags, visibility);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DominoServerProjectsApiProjectGatewaySummary {\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" details: ").append(toIndentedString(details)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" isPinned: ").append(toIndentedString(isPinned)).append("\n");
sb.append(" latestResultRunId: ").append(toIndentedString(latestResultRunId)).append("\n");
sb.append(" latestResultTimestamp: ").append(toIndentedString(latestResultTimestamp)).append("\n");
sb.append(" mainGitRepositoryUri: ").append(toIndentedString(mainGitRepositoryUri)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" ownerId: ").append(toIndentedString(ownerId)).append("\n");
sb.append(" ownerName: ").append(toIndentedString(ownerName)).append("\n");
sb.append(" projectType: ").append(toIndentedString(projectType)).append("\n");
sb.append(" relationship: ").append(toIndentedString(relationship)).append("\n");
sb.append(" runCounts: ").append(toIndentedString(runCounts)).append("\n");
sb.append(" serviceProvider: ").append(toIndentedString(serviceProvider)).append("\n");
sb.append(" stageId: ").append(toIndentedString(stageId)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" tags: ").append(toIndentedString(tags)).append("\n");
sb.append(" visibility: ").append(toIndentedString(visibility)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `description` to the URL query string
if (getDescription() != null) {
joiner.add(String.format("%sdescription%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getDescription()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `details` to the URL query string
if (getDetails() != null) {
joiner.add(getDetails().toUrlQueryString(prefix + "details" + suffix));
}
// add `id` to the URL query string
if (getId() != null) {
joiner.add(String.format("%sid%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `isPinned` to the URL query string
if (getIsPinned() != null) {
joiner.add(String.format("%sisPinned%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getIsPinned()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `latestResultRunId` to the URL query string
if (getLatestResultRunId() != null) {
joiner.add(String.format("%slatestResultRunId%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getLatestResultRunId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `latestResultTimestamp` to the URL query string
if (getLatestResultTimestamp() != null) {
joiner.add(String.format("%slatestResultTimestamp%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getLatestResultTimestamp()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `mainGitRepositoryUri` to the URL query string
if (getMainGitRepositoryUri() != null) {
joiner.add(String.format("%smainGitRepositoryUri%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getMainGitRepositoryUri()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `name` to the URL query string
if (getName() != null) {
joiner.add(String.format("%sname%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getName()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `ownerId` to the URL query string
if (getOwnerId() != null) {
joiner.add(String.format("%sownerId%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getOwnerId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `ownerName` to the URL query string
if (getOwnerName() != null) {
joiner.add(String.format("%sownerName%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getOwnerName()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `projectType` to the URL query string
if (getProjectType() != null) {
joiner.add(String.format("%sprojectType%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getProjectType()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `relationship` to the URL query string
if (getRelationship() != null) {
joiner.add(String.format("%srelationship%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getRelationship()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `runCounts` to the URL query string
if (getRunCounts() != null) {
for (int i = 0; i < getRunCounts().size(); i++) {
if (getRunCounts().get(i) != null) {
joiner.add(getRunCounts().get(i).toUrlQueryString(String.format("%srunCounts%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `serviceProvider` to the URL query string
if (getServiceProvider() != null) {
joiner.add(String.format("%sserviceProvider%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getServiceProvider()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `stageId` to the URL query string
if (getStageId() != null) {
joiner.add(String.format("%sstageId%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getStageId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `status` to the URL query string
if (getStatus() != null) {
joiner.add(getStatus().toUrlQueryString(prefix + "status" + suffix));
}
// add `tags` to the URL query string
if (getTags() != null) {
for (int i = 0; i < getTags().size(); i++) {
if (getTags().get(i) != null) {
joiner.add(getTags().get(i).toUrlQueryString(String.format("%stags%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `visibility` to the URL query string
if (getVisibility() != null) {
joiner.add(String.format("%svisibility%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getVisibility()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy