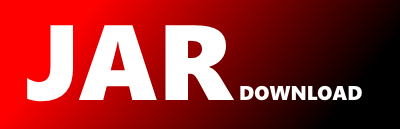
com.dominodatalab.api.rest.LogsWithProblemSuggestionApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of domino-java-client Show documentation
Show all versions of domino-java-client Show documentation
Domino Data Lab API Client to connect to Domino web services using Java HTTP Client.
/*
* Domino Data Lab API v4
* This API provides access to select Domino functions available in Domino's non-public API. To authenticate your requests, include your API Key (which you can find on your account page) with the header X-Domino-Api-Key.
*
* The version of the OpenAPI document: 4.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.api.rest;
import com.dominodatalab.api.invoker.ApiClient;
import com.dominodatalab.api.invoker.ApiException;
import com.dominodatalab.api.invoker.ApiResponse;
import com.dominodatalab.api.invoker.Pair;
import java.math.BigDecimal;
import com.dominodatalab.api.model.DominoJobsInterfaceLogsWithProblemSuggestion;
import com.dominodatalab.api.model.Error;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.InputStream;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.IOException;
import java.io.OutputStream;
import java.net.http.HttpRequest;
import java.nio.channels.Channels;
import java.nio.channels.Pipe;
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.time.Duration;
import java.util.ArrayList;
import java.util.StringJoiner;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.function.Consumer;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-10-04T16:37:26.309454-04:00[America/New_York]", comments = "Generator version: 7.8.0")
public class LogsWithProblemSuggestionApi {
protected final HttpClient memberVarHttpClient;
protected final ObjectMapper memberVarObjectMapper;
protected final String memberVarBaseUri;
protected final Consumer memberVarInterceptor;
protected final Duration memberVarReadTimeout;
protected final Consumer> memberVarResponseInterceptor;
protected final Consumer> memberVarAsyncResponseInterceptor;
public LogsWithProblemSuggestionApi() {
this(new ApiClient());
}
public LogsWithProblemSuggestionApi(ApiClient apiClient) {
memberVarHttpClient = apiClient.getHttpClient();
memberVarObjectMapper = apiClient.getObjectMapper();
memberVarBaseUri = apiClient.getBaseUri();
memberVarInterceptor = apiClient.getRequestInterceptor();
memberVarReadTimeout = apiClient.getReadTimeout();
memberVarResponseInterceptor = apiClient.getResponseInterceptor();
memberVarAsyncResponseInterceptor = apiClient.getAsyncResponseInterceptor();
}
protected ApiException getApiException(String operationId, HttpResponse response) throws IOException {
String body = response.body() == null ? null : new String(response.body().readAllBytes());
String message = formatExceptionMessage(operationId, response.statusCode(), body);
return new ApiException(response.statusCode(), message, response.headers(), body);
}
protected String formatExceptionMessage(String operationId, int statusCode, String body) {
if (body == null || body.isEmpty()) {
body = "[no body]";
}
return operationId + " call failed with: " + statusCode + " - " + body;
}
/**
* Get the suggestion when problem occurs in a job along with the logs
*
* @param jobId (required)
* @param logType Types of logs: * `console` - This is the default if the value is not provided. All logs lines displayed in the job's runtime environment. * `stdout` - Log lines displayed in the stderr of the job's runtime environment. * `stderr` - Log lines displayed in the stderr of the job's runtime environment. * `stdoutstderr` - Interleaved stdout and stderr. * `prepareoutput` - Log lines generated by the environment preparing the job. (optional, default to console)
* @param limit Max number of log lines to fetch. Will be overridden by the configuration's limit if this value exceeds the configuration's limit, or if this value is not provided. (optional, default to 10000)
* @param offset The index of the current body of logs to start fetching from. 0 by default and typically won't be used for a timestamp-based offset log fetching strategy. (optional, default to 0)
* @param latestTimeNano The epoch time in nanoseconds to start fetching from, such as \"1543538813745986325\". \"0\" by default and will typically be used for a timestamp-based offset log fetching strategy. (optional, default to 0)
* @return DominoJobsInterfaceLogsWithProblemSuggestion
* @throws ApiException if fails to make API call
*/
public DominoJobsInterfaceLogsWithProblemSuggestion getLogsWithProblemSuggestions(String jobId, String logType, BigDecimal limit, BigDecimal offset, String latestTimeNano) throws ApiException {
ApiResponse localVarResponse = getLogsWithProblemSuggestionsWithHttpInfo(jobId, logType, limit, offset, latestTimeNano);
return localVarResponse.getData();
}
/**
* Get the suggestion when problem occurs in a job along with the logs
*
* @param jobId (required)
* @param logType Types of logs: * `console` - This is the default if the value is not provided. All logs lines displayed in the job's runtime environment. * `stdout` - Log lines displayed in the stderr of the job's runtime environment. * `stderr` - Log lines displayed in the stderr of the job's runtime environment. * `stdoutstderr` - Interleaved stdout and stderr. * `prepareoutput` - Log lines generated by the environment preparing the job. (optional, default to console)
* @param limit Max number of log lines to fetch. Will be overridden by the configuration's limit if this value exceeds the configuration's limit, or if this value is not provided. (optional, default to 10000)
* @param offset The index of the current body of logs to start fetching from. 0 by default and typically won't be used for a timestamp-based offset log fetching strategy. (optional, default to 0)
* @param latestTimeNano The epoch time in nanoseconds to start fetching from, such as \"1543538813745986325\". \"0\" by default and will typically be used for a timestamp-based offset log fetching strategy. (optional, default to 0)
* @return ApiResponse<DominoJobsInterfaceLogsWithProblemSuggestion>
* @throws ApiException if fails to make API call
*/
public ApiResponse getLogsWithProblemSuggestionsWithHttpInfo(String jobId, String logType, BigDecimal limit, BigDecimal offset, String latestTimeNano) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getLogsWithProblemSuggestionsRequestBuilder(jobId, logType, limit, offset, latestTimeNano);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getLogsWithProblemSuggestions", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder getLogsWithProblemSuggestionsRequestBuilder(String jobId, String logType, BigDecimal limit, BigDecimal offset, String latestTimeNano) throws ApiException {
// verify the required parameter 'jobId' is set
if (jobId == null) {
throw new ApiException(400, "Missing the required parameter 'jobId' when calling getLogsWithProblemSuggestions");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/jobs/{jobId}/logsWithProblemSuggestions"
.replace("{jobId}", ApiClient.urlEncode(jobId.toString()));
List localVarQueryParams = new ArrayList<>();
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
localVarQueryParameterBaseName = "logType";
localVarQueryParams.addAll(ApiClient.parameterToPairs("logType", logType));
localVarQueryParameterBaseName = "limit";
localVarQueryParams.addAll(ApiClient.parameterToPairs("limit", limit));
localVarQueryParameterBaseName = "offset";
localVarQueryParams.addAll(ApiClient.parameterToPairs("offset", offset));
localVarQueryParameterBaseName = "latestTimeNano";
localVarQueryParams.addAll(ApiClient.parameterToPairs("latestTimeNano", latestTimeNano));
if (!localVarQueryParams.isEmpty() || localVarQueryStringJoiner.length() != 0) {
StringJoiner queryJoiner = new StringJoiner("&");
localVarQueryParams.forEach(p -> queryJoiner.add(p.getName() + '=' + p.getValue()));
if (localVarQueryStringJoiner.length() != 0) {
queryJoiner.add(localVarQueryStringJoiner.toString());
}
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath + '?' + queryJoiner.toString()));
} else {
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
}
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy