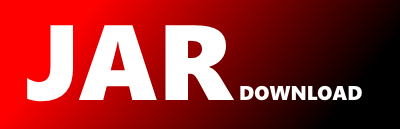
com.dominodatalab.pub.model.AthenaBillingConfigsV1 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of domino-java-client Show documentation
Show all versions of domino-java-client Show documentation
Domino Data Lab API Client to connect to Domino web services using Java HTTP Client.
/*
* Domino Public API
* Domino Public API Endpoints
*
* The version of the OpenAPI document: 0.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.pub.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.dominodatalab.pub.invoker.ApiClient;
/**
* AthenaBillingConfigsV1
*/
@JsonPropertyOrder({
AthenaBillingConfigsV1.JSON_PROPERTY_ATHENA_BUCKET_NAME,
AthenaBillingConfigsV1.JSON_PROPERTY_ATHENA_DATABASE,
AthenaBillingConfigsV1.JSON_PROPERTY_ATHENA_REGION,
AthenaBillingConfigsV1.JSON_PROPERTY_ATHENA_TABLE,
AthenaBillingConfigsV1.JSON_PROPERTY_PROJECT_I_D,
AthenaBillingConfigsV1.JSON_PROPERTY_SERVICE_KEY_NAME,
AthenaBillingConfigsV1.JSON_PROPERTY_SERVICE_KEY_SECRET
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-10-04T16:37:28.765500600-04:00[America/New_York]", comments = "Generator version: 7.8.0")
public class AthenaBillingConfigsV1 {
public static final String JSON_PROPERTY_ATHENA_BUCKET_NAME = "athenaBucketName";
private String athenaBucketName;
public static final String JSON_PROPERTY_ATHENA_DATABASE = "athenaDatabase";
private String athenaDatabase;
public static final String JSON_PROPERTY_ATHENA_REGION = "athenaRegion";
private String athenaRegion;
public static final String JSON_PROPERTY_ATHENA_TABLE = "athenaTable";
private String athenaTable;
public static final String JSON_PROPERTY_PROJECT_I_D = "projectID";
private String projectID;
public static final String JSON_PROPERTY_SERVICE_KEY_NAME = "serviceKeyName";
private String serviceKeyName;
public static final String JSON_PROPERTY_SERVICE_KEY_SECRET = "serviceKeySecret";
private String serviceKeySecret;
public AthenaBillingConfigsV1() {
}
public AthenaBillingConfigsV1 athenaBucketName(String athenaBucketName) {
this.athenaBucketName = athenaBucketName;
return this;
}
/**
* Get athenaBucketName
* @return athenaBucketName
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ATHENA_BUCKET_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getAthenaBucketName() {
return athenaBucketName;
}
@JsonProperty(JSON_PROPERTY_ATHENA_BUCKET_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setAthenaBucketName(String athenaBucketName) {
this.athenaBucketName = athenaBucketName;
}
public AthenaBillingConfigsV1 athenaDatabase(String athenaDatabase) {
this.athenaDatabase = athenaDatabase;
return this;
}
/**
* Get athenaDatabase
* @return athenaDatabase
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ATHENA_DATABASE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getAthenaDatabase() {
return athenaDatabase;
}
@JsonProperty(JSON_PROPERTY_ATHENA_DATABASE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setAthenaDatabase(String athenaDatabase) {
this.athenaDatabase = athenaDatabase;
}
public AthenaBillingConfigsV1 athenaRegion(String athenaRegion) {
this.athenaRegion = athenaRegion;
return this;
}
/**
* Get athenaRegion
* @return athenaRegion
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ATHENA_REGION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getAthenaRegion() {
return athenaRegion;
}
@JsonProperty(JSON_PROPERTY_ATHENA_REGION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setAthenaRegion(String athenaRegion) {
this.athenaRegion = athenaRegion;
}
public AthenaBillingConfigsV1 athenaTable(String athenaTable) {
this.athenaTable = athenaTable;
return this;
}
/**
* Get athenaTable
* @return athenaTable
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ATHENA_TABLE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getAthenaTable() {
return athenaTable;
}
@JsonProperty(JSON_PROPERTY_ATHENA_TABLE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setAthenaTable(String athenaTable) {
this.athenaTable = athenaTable;
}
public AthenaBillingConfigsV1 projectID(String projectID) {
this.projectID = projectID;
return this;
}
/**
* Get projectID
* @return projectID
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_PROJECT_I_D)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getProjectID() {
return projectID;
}
@JsonProperty(JSON_PROPERTY_PROJECT_I_D)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setProjectID(String projectID) {
this.projectID = projectID;
}
public AthenaBillingConfigsV1 serviceKeyName(String serviceKeyName) {
this.serviceKeyName = serviceKeyName;
return this;
}
/**
* Get serviceKeyName
* @return serviceKeyName
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_SERVICE_KEY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getServiceKeyName() {
return serviceKeyName;
}
@JsonProperty(JSON_PROPERTY_SERVICE_KEY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setServiceKeyName(String serviceKeyName) {
this.serviceKeyName = serviceKeyName;
}
public AthenaBillingConfigsV1 serviceKeySecret(String serviceKeySecret) {
this.serviceKeySecret = serviceKeySecret;
return this;
}
/**
* Get serviceKeySecret
* @return serviceKeySecret
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_SERVICE_KEY_SECRET)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getServiceKeySecret() {
return serviceKeySecret;
}
@JsonProperty(JSON_PROPERTY_SERVICE_KEY_SECRET)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setServiceKeySecret(String serviceKeySecret) {
this.serviceKeySecret = serviceKeySecret;
}
/**
* Return true if this AthenaBillingConfigsV1 object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AthenaBillingConfigsV1 athenaBillingConfigsV1 = (AthenaBillingConfigsV1) o;
return Objects.equals(this.athenaBucketName, athenaBillingConfigsV1.athenaBucketName) &&
Objects.equals(this.athenaDatabase, athenaBillingConfigsV1.athenaDatabase) &&
Objects.equals(this.athenaRegion, athenaBillingConfigsV1.athenaRegion) &&
Objects.equals(this.athenaTable, athenaBillingConfigsV1.athenaTable) &&
Objects.equals(this.projectID, athenaBillingConfigsV1.projectID) &&
Objects.equals(this.serviceKeyName, athenaBillingConfigsV1.serviceKeyName) &&
Objects.equals(this.serviceKeySecret, athenaBillingConfigsV1.serviceKeySecret);
}
@Override
public int hashCode() {
return Objects.hash(athenaBucketName, athenaDatabase, athenaRegion, athenaTable, projectID, serviceKeyName, serviceKeySecret);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AthenaBillingConfigsV1 {\n");
sb.append(" athenaBucketName: ").append(toIndentedString(athenaBucketName)).append("\n");
sb.append(" athenaDatabase: ").append(toIndentedString(athenaDatabase)).append("\n");
sb.append(" athenaRegion: ").append(toIndentedString(athenaRegion)).append("\n");
sb.append(" athenaTable: ").append(toIndentedString(athenaTable)).append("\n");
sb.append(" projectID: ").append(toIndentedString(projectID)).append("\n");
sb.append(" serviceKeyName: ").append(toIndentedString(serviceKeyName)).append("\n");
sb.append(" serviceKeySecret: ").append(toIndentedString(serviceKeySecret)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `athenaBucketName` to the URL query string
if (getAthenaBucketName() != null) {
joiner.add(String.format("%sathenaBucketName%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getAthenaBucketName()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `athenaDatabase` to the URL query string
if (getAthenaDatabase() != null) {
joiner.add(String.format("%sathenaDatabase%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getAthenaDatabase()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `athenaRegion` to the URL query string
if (getAthenaRegion() != null) {
joiner.add(String.format("%sathenaRegion%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getAthenaRegion()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `athenaTable` to the URL query string
if (getAthenaTable() != null) {
joiner.add(String.format("%sathenaTable%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getAthenaTable()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `projectID` to the URL query string
if (getProjectID() != null) {
joiner.add(String.format("%sprojectID%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getProjectID()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `serviceKeyName` to the URL query string
if (getServiceKeyName() != null) {
joiner.add(String.format("%sserviceKeyName%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getServiceKeyName()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `serviceKeySecret` to the URL query string
if (getServiceKeySecret() != null) {
joiner.add(String.format("%sserviceKeySecret%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getServiceKeySecret()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy