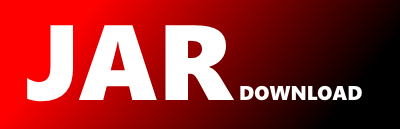
com.dominodatalab.pub.model.ComputeTierFullConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of domino-java-client Show documentation
Show all versions of domino-java-client Show documentation
Domino Data Lab API Client to connect to Domino web services using Java HTTP Client.
/*
* Domino Public API
* Domino Public API Endpoints
*
* The version of the OpenAPI document: 0.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.pub.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.dominodatalab.pub.model.ComputeProviderTypeModelDeploymentTypes;
import com.dominodatalab.pub.model.DefinedSchemaValue;
import com.dominodatalab.pub.model.SchemaDefinitionItem;
import com.dominodatalab.pub.model.SupportedSource;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.dominodatalab.pub.invoker.ApiClient;
/**
* ComputeTierFullConfiguration
*/
@JsonPropertyOrder({
ComputeTierFullConfiguration.JSON_PROPERTY_COMPUTE_PROVIDER_CONFIGURATION,
ComputeTierFullConfiguration.JSON_PROPERTY_COMPUTE_PROVIDER_ID,
ComputeTierFullConfiguration.JSON_PROPERTY_COMPUTE_PROVIDER_NAME,
ComputeTierFullConfiguration.JSON_PROPERTY_COMPUTE_PROVIDER_TYPE_ID,
ComputeTierFullConfiguration.JSON_PROPERTY_COMPUTE_TIER_CONFIGURATION,
ComputeTierFullConfiguration.JSON_PROPERTY_DATA_PLANE_ID,
ComputeTierFullConfiguration.JSON_PROPERTY_DISPLAY_NAME,
ComputeTierFullConfiguration.JSON_PROPERTY_ID,
ComputeTierFullConfiguration.JSON_PROPERTY_MODEL_DEPLOYMENT_CONFIGURATION_SCHEMA,
ComputeTierFullConfiguration.JSON_PROPERTY_MODEL_DEPLOYMENT_STATE_SCHEMA,
ComputeTierFullConfiguration.JSON_PROPERTY_MODEL_DEPLOYMENT_SUPPORTED_MODEL_SOURCES,
ComputeTierFullConfiguration.JSON_PROPERTY_MODEL_DEPLOYMENT_TYPES,
ComputeTierFullConfiguration.JSON_PROPERTY_MODEL_DETAILS_CONFIGURATION_SCHEMA,
ComputeTierFullConfiguration.JSON_PROPERTY_MODEL_DETAILS_STATE_SCHEMA
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-10-04T16:37:28.765500600-04:00[America/New_York]", comments = "Generator version: 7.8.0")
public class ComputeTierFullConfiguration {
public static final String JSON_PROPERTY_COMPUTE_PROVIDER_CONFIGURATION = "computeProviderConfiguration";
private List computeProviderConfiguration = new ArrayList<>();
public static final String JSON_PROPERTY_COMPUTE_PROVIDER_ID = "computeProviderId";
private String computeProviderId;
public static final String JSON_PROPERTY_COMPUTE_PROVIDER_NAME = "computeProviderName";
private String computeProviderName;
public static final String JSON_PROPERTY_COMPUTE_PROVIDER_TYPE_ID = "computeProviderTypeId";
private String computeProviderTypeId;
public static final String JSON_PROPERTY_COMPUTE_TIER_CONFIGURATION = "computeTierConfiguration";
private List computeTierConfiguration = new ArrayList<>();
public static final String JSON_PROPERTY_DATA_PLANE_ID = "dataPlaneId";
private String dataPlaneId;
public static final String JSON_PROPERTY_DISPLAY_NAME = "displayName";
private String displayName;
public static final String JSON_PROPERTY_ID = "id";
private String id;
public static final String JSON_PROPERTY_MODEL_DEPLOYMENT_CONFIGURATION_SCHEMA = "modelDeploymentConfigurationSchema";
private List modelDeploymentConfigurationSchema = new ArrayList<>();
public static final String JSON_PROPERTY_MODEL_DEPLOYMENT_STATE_SCHEMA = "modelDeploymentStateSchema";
private List modelDeploymentStateSchema = new ArrayList<>();
public static final String JSON_PROPERTY_MODEL_DEPLOYMENT_SUPPORTED_MODEL_SOURCES = "modelDeploymentSupportedModelSources";
private List modelDeploymentSupportedModelSources = new ArrayList<>();
public static final String JSON_PROPERTY_MODEL_DEPLOYMENT_TYPES = "modelDeploymentTypes";
private ComputeProviderTypeModelDeploymentTypes modelDeploymentTypes;
public static final String JSON_PROPERTY_MODEL_DETAILS_CONFIGURATION_SCHEMA = "modelDetailsConfigurationSchema";
private List modelDetailsConfigurationSchema = new ArrayList<>();
public static final String JSON_PROPERTY_MODEL_DETAILS_STATE_SCHEMA = "modelDetailsStateSchema";
private List modelDetailsStateSchema = new ArrayList<>();
public ComputeTierFullConfiguration() {
}
public ComputeTierFullConfiguration computeProviderConfiguration(List computeProviderConfiguration) {
this.computeProviderConfiguration = computeProviderConfiguration;
return this;
}
public ComputeTierFullConfiguration addComputeProviderConfigurationItem(DefinedSchemaValue computeProviderConfigurationItem) {
if (this.computeProviderConfiguration == null) {
this.computeProviderConfiguration = new ArrayList<>();
}
this.computeProviderConfiguration.add(computeProviderConfigurationItem);
return this;
}
/**
* Get computeProviderConfiguration
* @return computeProviderConfiguration
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_COMPUTE_PROVIDER_CONFIGURATION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getComputeProviderConfiguration() {
return computeProviderConfiguration;
}
@JsonProperty(JSON_PROPERTY_COMPUTE_PROVIDER_CONFIGURATION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setComputeProviderConfiguration(List computeProviderConfiguration) {
this.computeProviderConfiguration = computeProviderConfiguration;
}
public ComputeTierFullConfiguration computeProviderId(String computeProviderId) {
this.computeProviderId = computeProviderId;
return this;
}
/**
* UUID for the Compute Provider
* @return computeProviderId
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_COMPUTE_PROVIDER_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getComputeProviderId() {
return computeProviderId;
}
@JsonProperty(JSON_PROPERTY_COMPUTE_PROVIDER_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setComputeProviderId(String computeProviderId) {
this.computeProviderId = computeProviderId;
}
public ComputeTierFullConfiguration computeProviderName(String computeProviderName) {
this.computeProviderName = computeProviderName;
return this;
}
/**
* Display name of the Compute Provider this Compute Tier belongs to
* @return computeProviderName
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_COMPUTE_PROVIDER_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getComputeProviderName() {
return computeProviderName;
}
@JsonProperty(JSON_PROPERTY_COMPUTE_PROVIDER_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setComputeProviderName(String computeProviderName) {
this.computeProviderName = computeProviderName;
}
public ComputeTierFullConfiguration computeProviderTypeId(String computeProviderTypeId) {
this.computeProviderTypeId = computeProviderTypeId;
return this;
}
/**
* UUID for the Compute Provider Type
* @return computeProviderTypeId
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_COMPUTE_PROVIDER_TYPE_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getComputeProviderTypeId() {
return computeProviderTypeId;
}
@JsonProperty(JSON_PROPERTY_COMPUTE_PROVIDER_TYPE_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setComputeProviderTypeId(String computeProviderTypeId) {
this.computeProviderTypeId = computeProviderTypeId;
}
public ComputeTierFullConfiguration computeTierConfiguration(List computeTierConfiguration) {
this.computeTierConfiguration = computeTierConfiguration;
return this;
}
public ComputeTierFullConfiguration addComputeTierConfigurationItem(DefinedSchemaValue computeTierConfigurationItem) {
if (this.computeTierConfiguration == null) {
this.computeTierConfiguration = new ArrayList<>();
}
this.computeTierConfiguration.add(computeTierConfigurationItem);
return this;
}
/**
* Get computeTierConfiguration
* @return computeTierConfiguration
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_COMPUTE_TIER_CONFIGURATION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getComputeTierConfiguration() {
return computeTierConfiguration;
}
@JsonProperty(JSON_PROPERTY_COMPUTE_TIER_CONFIGURATION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setComputeTierConfiguration(List computeTierConfiguration) {
this.computeTierConfiguration = computeTierConfiguration;
}
public ComputeTierFullConfiguration dataPlaneId(String dataPlaneId) {
this.dataPlaneId = dataPlaneId;
return this;
}
/**
* ID of the data plane where the operator is deployed
* @return dataPlaneId
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_DATA_PLANE_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getDataPlaneId() {
return dataPlaneId;
}
@JsonProperty(JSON_PROPERTY_DATA_PLANE_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDataPlaneId(String dataPlaneId) {
this.dataPlaneId = dataPlaneId;
}
public ComputeTierFullConfiguration displayName(String displayName) {
this.displayName = displayName;
return this;
}
/**
* Display name for the Compute Tier
* @return displayName
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_DISPLAY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getDisplayName() {
return displayName;
}
@JsonProperty(JSON_PROPERTY_DISPLAY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
public ComputeTierFullConfiguration id(String id) {
this.id = id;
return this;
}
/**
* UUID for the Compute Tier
* @return id
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getId() {
return id;
}
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setId(String id) {
this.id = id;
}
public ComputeTierFullConfiguration modelDeploymentConfigurationSchema(List modelDeploymentConfigurationSchema) {
this.modelDeploymentConfigurationSchema = modelDeploymentConfigurationSchema;
return this;
}
public ComputeTierFullConfiguration addModelDeploymentConfigurationSchemaItem(SchemaDefinitionItem modelDeploymentConfigurationSchemaItem) {
if (this.modelDeploymentConfigurationSchema == null) {
this.modelDeploymentConfigurationSchema = new ArrayList<>();
}
this.modelDeploymentConfigurationSchema.add(modelDeploymentConfigurationSchemaItem);
return this;
}
/**
* Get modelDeploymentConfigurationSchema
* @return modelDeploymentConfigurationSchema
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_MODEL_DEPLOYMENT_CONFIGURATION_SCHEMA)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getModelDeploymentConfigurationSchema() {
return modelDeploymentConfigurationSchema;
}
@JsonProperty(JSON_PROPERTY_MODEL_DEPLOYMENT_CONFIGURATION_SCHEMA)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setModelDeploymentConfigurationSchema(List modelDeploymentConfigurationSchema) {
this.modelDeploymentConfigurationSchema = modelDeploymentConfigurationSchema;
}
public ComputeTierFullConfiguration modelDeploymentStateSchema(List modelDeploymentStateSchema) {
this.modelDeploymentStateSchema = modelDeploymentStateSchema;
return this;
}
public ComputeTierFullConfiguration addModelDeploymentStateSchemaItem(SchemaDefinitionItem modelDeploymentStateSchemaItem) {
if (this.modelDeploymentStateSchema == null) {
this.modelDeploymentStateSchema = new ArrayList<>();
}
this.modelDeploymentStateSchema.add(modelDeploymentStateSchemaItem);
return this;
}
/**
* Get modelDeploymentStateSchema
* @return modelDeploymentStateSchema
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_MODEL_DEPLOYMENT_STATE_SCHEMA)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getModelDeploymentStateSchema() {
return modelDeploymentStateSchema;
}
@JsonProperty(JSON_PROPERTY_MODEL_DEPLOYMENT_STATE_SCHEMA)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setModelDeploymentStateSchema(List modelDeploymentStateSchema) {
this.modelDeploymentStateSchema = modelDeploymentStateSchema;
}
public ComputeTierFullConfiguration modelDeploymentSupportedModelSources(List modelDeploymentSupportedModelSources) {
this.modelDeploymentSupportedModelSources = modelDeploymentSupportedModelSources;
return this;
}
public ComputeTierFullConfiguration addModelDeploymentSupportedModelSourcesItem(SupportedSource modelDeploymentSupportedModelSourcesItem) {
if (this.modelDeploymentSupportedModelSources == null) {
this.modelDeploymentSupportedModelSources = new ArrayList<>();
}
this.modelDeploymentSupportedModelSources.add(modelDeploymentSupportedModelSourcesItem);
return this;
}
/**
* Model sources supported by Domino
* @return modelDeploymentSupportedModelSources
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_MODEL_DEPLOYMENT_SUPPORTED_MODEL_SOURCES)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getModelDeploymentSupportedModelSources() {
return modelDeploymentSupportedModelSources;
}
@JsonProperty(JSON_PROPERTY_MODEL_DEPLOYMENT_SUPPORTED_MODEL_SOURCES)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setModelDeploymentSupportedModelSources(List modelDeploymentSupportedModelSources) {
this.modelDeploymentSupportedModelSources = modelDeploymentSupportedModelSources;
}
public ComputeTierFullConfiguration modelDeploymentTypes(ComputeProviderTypeModelDeploymentTypes modelDeploymentTypes) {
this.modelDeploymentTypes = modelDeploymentTypes;
return this;
}
/**
* Get modelDeploymentTypes
* @return modelDeploymentTypes
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_MODEL_DEPLOYMENT_TYPES)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public ComputeProviderTypeModelDeploymentTypes getModelDeploymentTypes() {
return modelDeploymentTypes;
}
@JsonProperty(JSON_PROPERTY_MODEL_DEPLOYMENT_TYPES)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setModelDeploymentTypes(ComputeProviderTypeModelDeploymentTypes modelDeploymentTypes) {
this.modelDeploymentTypes = modelDeploymentTypes;
}
public ComputeTierFullConfiguration modelDetailsConfigurationSchema(List modelDetailsConfigurationSchema) {
this.modelDetailsConfigurationSchema = modelDetailsConfigurationSchema;
return this;
}
public ComputeTierFullConfiguration addModelDetailsConfigurationSchemaItem(SchemaDefinitionItem modelDetailsConfigurationSchemaItem) {
if (this.modelDetailsConfigurationSchema == null) {
this.modelDetailsConfigurationSchema = new ArrayList<>();
}
this.modelDetailsConfigurationSchema.add(modelDetailsConfigurationSchemaItem);
return this;
}
/**
* Get modelDetailsConfigurationSchema
* @return modelDetailsConfigurationSchema
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_MODEL_DETAILS_CONFIGURATION_SCHEMA)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getModelDetailsConfigurationSchema() {
return modelDetailsConfigurationSchema;
}
@JsonProperty(JSON_PROPERTY_MODEL_DETAILS_CONFIGURATION_SCHEMA)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setModelDetailsConfigurationSchema(List modelDetailsConfigurationSchema) {
this.modelDetailsConfigurationSchema = modelDetailsConfigurationSchema;
}
public ComputeTierFullConfiguration modelDetailsStateSchema(List modelDetailsStateSchema) {
this.modelDetailsStateSchema = modelDetailsStateSchema;
return this;
}
public ComputeTierFullConfiguration addModelDetailsStateSchemaItem(SchemaDefinitionItem modelDetailsStateSchemaItem) {
if (this.modelDetailsStateSchema == null) {
this.modelDetailsStateSchema = new ArrayList<>();
}
this.modelDetailsStateSchema.add(modelDetailsStateSchemaItem);
return this;
}
/**
* Get modelDetailsStateSchema
* @return modelDetailsStateSchema
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_MODEL_DETAILS_STATE_SCHEMA)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getModelDetailsStateSchema() {
return modelDetailsStateSchema;
}
@JsonProperty(JSON_PROPERTY_MODEL_DETAILS_STATE_SCHEMA)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setModelDetailsStateSchema(List modelDetailsStateSchema) {
this.modelDetailsStateSchema = modelDetailsStateSchema;
}
/**
* Return true if this ComputeTierFullConfiguration object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ComputeTierFullConfiguration computeTierFullConfiguration = (ComputeTierFullConfiguration) o;
return Objects.equals(this.computeProviderConfiguration, computeTierFullConfiguration.computeProviderConfiguration) &&
Objects.equals(this.computeProviderId, computeTierFullConfiguration.computeProviderId) &&
Objects.equals(this.computeProviderName, computeTierFullConfiguration.computeProviderName) &&
Objects.equals(this.computeProviderTypeId, computeTierFullConfiguration.computeProviderTypeId) &&
Objects.equals(this.computeTierConfiguration, computeTierFullConfiguration.computeTierConfiguration) &&
Objects.equals(this.dataPlaneId, computeTierFullConfiguration.dataPlaneId) &&
Objects.equals(this.displayName, computeTierFullConfiguration.displayName) &&
Objects.equals(this.id, computeTierFullConfiguration.id) &&
Objects.equals(this.modelDeploymentConfigurationSchema, computeTierFullConfiguration.modelDeploymentConfigurationSchema) &&
Objects.equals(this.modelDeploymentStateSchema, computeTierFullConfiguration.modelDeploymentStateSchema) &&
Objects.equals(this.modelDeploymentSupportedModelSources, computeTierFullConfiguration.modelDeploymentSupportedModelSources) &&
Objects.equals(this.modelDeploymentTypes, computeTierFullConfiguration.modelDeploymentTypes) &&
Objects.equals(this.modelDetailsConfigurationSchema, computeTierFullConfiguration.modelDetailsConfigurationSchema) &&
Objects.equals(this.modelDetailsStateSchema, computeTierFullConfiguration.modelDetailsStateSchema);
}
@Override
public int hashCode() {
return Objects.hash(computeProviderConfiguration, computeProviderId, computeProviderName, computeProviderTypeId, computeTierConfiguration, dataPlaneId, displayName, id, modelDeploymentConfigurationSchema, modelDeploymentStateSchema, modelDeploymentSupportedModelSources, modelDeploymentTypes, modelDetailsConfigurationSchema, modelDetailsStateSchema);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ComputeTierFullConfiguration {\n");
sb.append(" computeProviderConfiguration: ").append(toIndentedString(computeProviderConfiguration)).append("\n");
sb.append(" computeProviderId: ").append(toIndentedString(computeProviderId)).append("\n");
sb.append(" computeProviderName: ").append(toIndentedString(computeProviderName)).append("\n");
sb.append(" computeProviderTypeId: ").append(toIndentedString(computeProviderTypeId)).append("\n");
sb.append(" computeTierConfiguration: ").append(toIndentedString(computeTierConfiguration)).append("\n");
sb.append(" dataPlaneId: ").append(toIndentedString(dataPlaneId)).append("\n");
sb.append(" displayName: ").append(toIndentedString(displayName)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" modelDeploymentConfigurationSchema: ").append(toIndentedString(modelDeploymentConfigurationSchema)).append("\n");
sb.append(" modelDeploymentStateSchema: ").append(toIndentedString(modelDeploymentStateSchema)).append("\n");
sb.append(" modelDeploymentSupportedModelSources: ").append(toIndentedString(modelDeploymentSupportedModelSources)).append("\n");
sb.append(" modelDeploymentTypes: ").append(toIndentedString(modelDeploymentTypes)).append("\n");
sb.append(" modelDetailsConfigurationSchema: ").append(toIndentedString(modelDetailsConfigurationSchema)).append("\n");
sb.append(" modelDetailsStateSchema: ").append(toIndentedString(modelDetailsStateSchema)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `computeProviderConfiguration` to the URL query string
if (getComputeProviderConfiguration() != null) {
for (int i = 0; i < getComputeProviderConfiguration().size(); i++) {
if (getComputeProviderConfiguration().get(i) != null) {
joiner.add(getComputeProviderConfiguration().get(i).toUrlQueryString(String.format("%scomputeProviderConfiguration%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `computeProviderId` to the URL query string
if (getComputeProviderId() != null) {
joiner.add(String.format("%scomputeProviderId%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getComputeProviderId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `computeProviderName` to the URL query string
if (getComputeProviderName() != null) {
joiner.add(String.format("%scomputeProviderName%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getComputeProviderName()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `computeProviderTypeId` to the URL query string
if (getComputeProviderTypeId() != null) {
joiner.add(String.format("%scomputeProviderTypeId%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getComputeProviderTypeId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `computeTierConfiguration` to the URL query string
if (getComputeTierConfiguration() != null) {
for (int i = 0; i < getComputeTierConfiguration().size(); i++) {
if (getComputeTierConfiguration().get(i) != null) {
joiner.add(getComputeTierConfiguration().get(i).toUrlQueryString(String.format("%scomputeTierConfiguration%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `dataPlaneId` to the URL query string
if (getDataPlaneId() != null) {
joiner.add(String.format("%sdataPlaneId%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getDataPlaneId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `displayName` to the URL query string
if (getDisplayName() != null) {
joiner.add(String.format("%sdisplayName%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getDisplayName()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `id` to the URL query string
if (getId() != null) {
joiner.add(String.format("%sid%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `modelDeploymentConfigurationSchema` to the URL query string
if (getModelDeploymentConfigurationSchema() != null) {
for (int i = 0; i < getModelDeploymentConfigurationSchema().size(); i++) {
if (getModelDeploymentConfigurationSchema().get(i) != null) {
joiner.add(getModelDeploymentConfigurationSchema().get(i).toUrlQueryString(String.format("%smodelDeploymentConfigurationSchema%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `modelDeploymentStateSchema` to the URL query string
if (getModelDeploymentStateSchema() != null) {
for (int i = 0; i < getModelDeploymentStateSchema().size(); i++) {
if (getModelDeploymentStateSchema().get(i) != null) {
joiner.add(getModelDeploymentStateSchema().get(i).toUrlQueryString(String.format("%smodelDeploymentStateSchema%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `modelDeploymentSupportedModelSources` to the URL query string
if (getModelDeploymentSupportedModelSources() != null) {
for (int i = 0; i < getModelDeploymentSupportedModelSources().size(); i++) {
if (getModelDeploymentSupportedModelSources().get(i) != null) {
joiner.add(String.format("%smodelDeploymentSupportedModelSources%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(ApiClient.valueToString(getModelDeploymentSupportedModelSources().get(i)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
}
// add `modelDeploymentTypes` to the URL query string
if (getModelDeploymentTypes() != null) {
joiner.add(getModelDeploymentTypes().toUrlQueryString(prefix + "modelDeploymentTypes" + suffix));
}
// add `modelDetailsConfigurationSchema` to the URL query string
if (getModelDetailsConfigurationSchema() != null) {
for (int i = 0; i < getModelDetailsConfigurationSchema().size(); i++) {
if (getModelDetailsConfigurationSchema().get(i) != null) {
joiner.add(getModelDetailsConfigurationSchema().get(i).toUrlQueryString(String.format("%smodelDetailsConfigurationSchema%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `modelDetailsStateSchema` to the URL query string
if (getModelDetailsStateSchema() != null) {
for (int i = 0; i < getModelDetailsStateSchema().size(); i++) {
if (getModelDetailsStateSchema().get(i) != null) {
joiner.add(getModelDetailsStateSchema().get(i).toUrlQueryString(String.format("%smodelDetailsStateSchema%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy