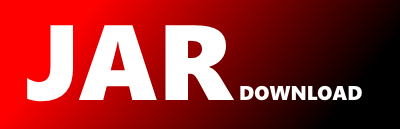
com.dominodatalab.pub.model.CostAllocationV1 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of domino-java-client Show documentation
Show all versions of domino-java-client Show documentation
Domino Data Lab API Client to connect to Domino web services using Java HTTP Client.
/*
* Domino Public API
* Domino Public API Endpoints
*
* The version of the OpenAPI document: 0.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.pub.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.dominodatalab.pub.model.CostAllocationV1Labels;
import com.dominodatalab.pub.model.CostAllocationV1Pv;
import com.dominodatalab.pub.model.CostAllocationV1Window;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.dominodatalab.pub.invoker.ApiClient;
/**
* CostAllocationV1
*/
@JsonPropertyOrder({
CostAllocationV1.JSON_PROPERTY_CPU_CORE_HOURS,
CostAllocationV1.JSON_PROPERTY_CPU_CORE_REQUEST_AVERAGE,
CostAllocationV1.JSON_PROPERTY_CPU_CORE_USAGE_AVERAGE,
CostAllocationV1.JSON_PROPERTY_CPU_CORES,
CostAllocationV1.JSON_PROPERTY_CPU_COST,
CostAllocationV1.JSON_PROPERTY_CPU_COST_ADJUSTMENT,
CostAllocationV1.JSON_PROPERTY_CPU_EFFICINCY,
CostAllocationV1.JSON_PROPERTY_DISCOUNT,
CostAllocationV1.JSON_PROPERTY_GPU_COST,
CostAllocationV1.JSON_PROPERTY_GPU_COST_ADJUSTMENT,
CostAllocationV1.JSON_PROPERTY_GPU_COUNT,
CostAllocationV1.JSON_PROPERTY_GPU_HOURS,
CostAllocationV1.JSON_PROPERTY_LABELS,
CostAllocationV1.JSON_PROPERTY_LOAD_BALANCER_COST,
CostAllocationV1.JSON_PROPERTY_LOAD_BALANCER_COST_ADJUSTMENT,
CostAllocationV1.JSON_PROPERTY_NAME,
CostAllocationV1.JSON_PROPERTY_NODE_TYPE,
CostAllocationV1.JSON_PROPERTY_PV,
CostAllocationV1.JSON_PROPERTY_RAM_COST,
CostAllocationV1.JSON_PROPERTY_RAM_COST_ADJUSTMENT,
CostAllocationV1.JSON_PROPERTY_TOTAL_COST,
CostAllocationV1.JSON_PROPERTY_WINDOW
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-10-04T16:37:28.765500600-04:00[America/New_York]", comments = "Generator version: 7.8.0")
public class CostAllocationV1 {
public static final String JSON_PROPERTY_CPU_CORE_HOURS = "cpuCoreHours";
private Double cpuCoreHours;
public static final String JSON_PROPERTY_CPU_CORE_REQUEST_AVERAGE = "cpuCoreRequestAverage";
private Double cpuCoreRequestAverage;
public static final String JSON_PROPERTY_CPU_CORE_USAGE_AVERAGE = "cpuCoreUsageAverage";
private Double cpuCoreUsageAverage;
public static final String JSON_PROPERTY_CPU_CORES = "cpuCores";
private Double cpuCores;
public static final String JSON_PROPERTY_CPU_COST = "cpuCost";
private Double cpuCost;
public static final String JSON_PROPERTY_CPU_COST_ADJUSTMENT = "cpuCostAdjustment";
private Double cpuCostAdjustment;
public static final String JSON_PROPERTY_CPU_EFFICINCY = "cpuEfficincy";
private Double cpuEfficincy;
public static final String JSON_PROPERTY_DISCOUNT = "discount";
private Double discount;
public static final String JSON_PROPERTY_GPU_COST = "gpuCost";
private Double gpuCost;
public static final String JSON_PROPERTY_GPU_COST_ADJUSTMENT = "gpuCostAdjustment";
private Double gpuCostAdjustment;
public static final String JSON_PROPERTY_GPU_COUNT = "gpuCount";
private Double gpuCount;
public static final String JSON_PROPERTY_GPU_HOURS = "gpuHours";
private Double gpuHours;
public static final String JSON_PROPERTY_LABELS = "labels";
private CostAllocationV1Labels labels;
public static final String JSON_PROPERTY_LOAD_BALANCER_COST = "loadBalancerCost";
private Double loadBalancerCost;
public static final String JSON_PROPERTY_LOAD_BALANCER_COST_ADJUSTMENT = "loadBalancerCostAdjustment";
private Double loadBalancerCostAdjustment;
public static final String JSON_PROPERTY_NAME = "name";
private String name;
public static final String JSON_PROPERTY_NODE_TYPE = "nodeType";
private String nodeType;
public static final String JSON_PROPERTY_PV = "pv";
private CostAllocationV1Pv pv;
public static final String JSON_PROPERTY_RAM_COST = "ramCost";
private Double ramCost;
public static final String JSON_PROPERTY_RAM_COST_ADJUSTMENT = "ramCostAdjustment";
private Double ramCostAdjustment;
public static final String JSON_PROPERTY_TOTAL_COST = "totalCost";
private Double totalCost;
public static final String JSON_PROPERTY_WINDOW = "window";
private CostAllocationV1Window window;
public CostAllocationV1() {
}
public CostAllocationV1 cpuCoreHours(Double cpuCoreHours) {
this.cpuCoreHours = cpuCoreHours;
return this;
}
/**
* Get cpuCoreHours
* @return cpuCoreHours
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_CPU_CORE_HOURS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getCpuCoreHours() {
return cpuCoreHours;
}
@JsonProperty(JSON_PROPERTY_CPU_CORE_HOURS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCpuCoreHours(Double cpuCoreHours) {
this.cpuCoreHours = cpuCoreHours;
}
public CostAllocationV1 cpuCoreRequestAverage(Double cpuCoreRequestAverage) {
this.cpuCoreRequestAverage = cpuCoreRequestAverage;
return this;
}
/**
* Get cpuCoreRequestAverage
* @return cpuCoreRequestAverage
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_CPU_CORE_REQUEST_AVERAGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getCpuCoreRequestAverage() {
return cpuCoreRequestAverage;
}
@JsonProperty(JSON_PROPERTY_CPU_CORE_REQUEST_AVERAGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCpuCoreRequestAverage(Double cpuCoreRequestAverage) {
this.cpuCoreRequestAverage = cpuCoreRequestAverage;
}
public CostAllocationV1 cpuCoreUsageAverage(Double cpuCoreUsageAverage) {
this.cpuCoreUsageAverage = cpuCoreUsageAverage;
return this;
}
/**
* Get cpuCoreUsageAverage
* @return cpuCoreUsageAverage
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_CPU_CORE_USAGE_AVERAGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getCpuCoreUsageAverage() {
return cpuCoreUsageAverage;
}
@JsonProperty(JSON_PROPERTY_CPU_CORE_USAGE_AVERAGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCpuCoreUsageAverage(Double cpuCoreUsageAverage) {
this.cpuCoreUsageAverage = cpuCoreUsageAverage;
}
public CostAllocationV1 cpuCores(Double cpuCores) {
this.cpuCores = cpuCores;
return this;
}
/**
* Get cpuCores
* @return cpuCores
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_CPU_CORES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getCpuCores() {
return cpuCores;
}
@JsonProperty(JSON_PROPERTY_CPU_CORES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCpuCores(Double cpuCores) {
this.cpuCores = cpuCores;
}
public CostAllocationV1 cpuCost(Double cpuCost) {
this.cpuCost = cpuCost;
return this;
}
/**
* Get cpuCost
* @return cpuCost
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_CPU_COST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getCpuCost() {
return cpuCost;
}
@JsonProperty(JSON_PROPERTY_CPU_COST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCpuCost(Double cpuCost) {
this.cpuCost = cpuCost;
}
public CostAllocationV1 cpuCostAdjustment(Double cpuCostAdjustment) {
this.cpuCostAdjustment = cpuCostAdjustment;
return this;
}
/**
* Get cpuCostAdjustment
* @return cpuCostAdjustment
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_CPU_COST_ADJUSTMENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getCpuCostAdjustment() {
return cpuCostAdjustment;
}
@JsonProperty(JSON_PROPERTY_CPU_COST_ADJUSTMENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCpuCostAdjustment(Double cpuCostAdjustment) {
this.cpuCostAdjustment = cpuCostAdjustment;
}
public CostAllocationV1 cpuEfficincy(Double cpuEfficincy) {
this.cpuEfficincy = cpuEfficincy;
return this;
}
/**
* Get cpuEfficincy
* @return cpuEfficincy
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_CPU_EFFICINCY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getCpuEfficincy() {
return cpuEfficincy;
}
@JsonProperty(JSON_PROPERTY_CPU_EFFICINCY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCpuEfficincy(Double cpuEfficincy) {
this.cpuEfficincy = cpuEfficincy;
}
public CostAllocationV1 discount(Double discount) {
this.discount = discount;
return this;
}
/**
* Get discount
* @return discount
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DISCOUNT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getDiscount() {
return discount;
}
@JsonProperty(JSON_PROPERTY_DISCOUNT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDiscount(Double discount) {
this.discount = discount;
}
public CostAllocationV1 gpuCost(Double gpuCost) {
this.gpuCost = gpuCost;
return this;
}
/**
* Get gpuCost
* @return gpuCost
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_GPU_COST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getGpuCost() {
return gpuCost;
}
@JsonProperty(JSON_PROPERTY_GPU_COST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setGpuCost(Double gpuCost) {
this.gpuCost = gpuCost;
}
public CostAllocationV1 gpuCostAdjustment(Double gpuCostAdjustment) {
this.gpuCostAdjustment = gpuCostAdjustment;
return this;
}
/**
* Get gpuCostAdjustment
* @return gpuCostAdjustment
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_GPU_COST_ADJUSTMENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getGpuCostAdjustment() {
return gpuCostAdjustment;
}
@JsonProperty(JSON_PROPERTY_GPU_COST_ADJUSTMENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setGpuCostAdjustment(Double gpuCostAdjustment) {
this.gpuCostAdjustment = gpuCostAdjustment;
}
public CostAllocationV1 gpuCount(Double gpuCount) {
this.gpuCount = gpuCount;
return this;
}
/**
* Get gpuCount
* @return gpuCount
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_GPU_COUNT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getGpuCount() {
return gpuCount;
}
@JsonProperty(JSON_PROPERTY_GPU_COUNT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setGpuCount(Double gpuCount) {
this.gpuCount = gpuCount;
}
public CostAllocationV1 gpuHours(Double gpuHours) {
this.gpuHours = gpuHours;
return this;
}
/**
* Get gpuHours
* @return gpuHours
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_GPU_HOURS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getGpuHours() {
return gpuHours;
}
@JsonProperty(JSON_PROPERTY_GPU_HOURS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setGpuHours(Double gpuHours) {
this.gpuHours = gpuHours;
}
public CostAllocationV1 labels(CostAllocationV1Labels labels) {
this.labels = labels;
return this;
}
/**
* Get labels
* @return labels
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_LABELS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public CostAllocationV1Labels getLabels() {
return labels;
}
@JsonProperty(JSON_PROPERTY_LABELS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLabels(CostAllocationV1Labels labels) {
this.labels = labels;
}
public CostAllocationV1 loadBalancerCost(Double loadBalancerCost) {
this.loadBalancerCost = loadBalancerCost;
return this;
}
/**
* Get loadBalancerCost
* @return loadBalancerCost
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_LOAD_BALANCER_COST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getLoadBalancerCost() {
return loadBalancerCost;
}
@JsonProperty(JSON_PROPERTY_LOAD_BALANCER_COST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLoadBalancerCost(Double loadBalancerCost) {
this.loadBalancerCost = loadBalancerCost;
}
public CostAllocationV1 loadBalancerCostAdjustment(Double loadBalancerCostAdjustment) {
this.loadBalancerCostAdjustment = loadBalancerCostAdjustment;
return this;
}
/**
* Get loadBalancerCostAdjustment
* @return loadBalancerCostAdjustment
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_LOAD_BALANCER_COST_ADJUSTMENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getLoadBalancerCostAdjustment() {
return loadBalancerCostAdjustment;
}
@JsonProperty(JSON_PROPERTY_LOAD_BALANCER_COST_ADJUSTMENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLoadBalancerCostAdjustment(Double loadBalancerCostAdjustment) {
this.loadBalancerCostAdjustment = loadBalancerCostAdjustment;
}
public CostAllocationV1 name(String name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getName() {
return name;
}
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setName(String name) {
this.name = name;
}
public CostAllocationV1 nodeType(String nodeType) {
this.nodeType = nodeType;
return this;
}
/**
* Get nodeType
* @return nodeType
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_NODE_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getNodeType() {
return nodeType;
}
@JsonProperty(JSON_PROPERTY_NODE_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setNodeType(String nodeType) {
this.nodeType = nodeType;
}
public CostAllocationV1 pv(CostAllocationV1Pv pv) {
this.pv = pv;
return this;
}
/**
* Get pv
* @return pv
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_PV)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public CostAllocationV1Pv getPv() {
return pv;
}
@JsonProperty(JSON_PROPERTY_PV)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPv(CostAllocationV1Pv pv) {
this.pv = pv;
}
public CostAllocationV1 ramCost(Double ramCost) {
this.ramCost = ramCost;
return this;
}
/**
* Get ramCost
* @return ramCost
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_RAM_COST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getRamCost() {
return ramCost;
}
@JsonProperty(JSON_PROPERTY_RAM_COST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRamCost(Double ramCost) {
this.ramCost = ramCost;
}
public CostAllocationV1 ramCostAdjustment(Double ramCostAdjustment) {
this.ramCostAdjustment = ramCostAdjustment;
return this;
}
/**
* Get ramCostAdjustment
* @return ramCostAdjustment
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_RAM_COST_ADJUSTMENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getRamCostAdjustment() {
return ramCostAdjustment;
}
@JsonProperty(JSON_PROPERTY_RAM_COST_ADJUSTMENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRamCostAdjustment(Double ramCostAdjustment) {
this.ramCostAdjustment = ramCostAdjustment;
}
public CostAllocationV1 totalCost(Double totalCost) {
this.totalCost = totalCost;
return this;
}
/**
* Get totalCost
* @return totalCost
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_TOTAL_COST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getTotalCost() {
return totalCost;
}
@JsonProperty(JSON_PROPERTY_TOTAL_COST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTotalCost(Double totalCost) {
this.totalCost = totalCost;
}
public CostAllocationV1 window(CostAllocationV1Window window) {
this.window = window;
return this;
}
/**
* Get window
* @return window
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_WINDOW)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public CostAllocationV1Window getWindow() {
return window;
}
@JsonProperty(JSON_PROPERTY_WINDOW)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setWindow(CostAllocationV1Window window) {
this.window = window;
}
/**
* Return true if this CostAllocationV1 object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CostAllocationV1 costAllocationV1 = (CostAllocationV1) o;
return Objects.equals(this.cpuCoreHours, costAllocationV1.cpuCoreHours) &&
Objects.equals(this.cpuCoreRequestAverage, costAllocationV1.cpuCoreRequestAverage) &&
Objects.equals(this.cpuCoreUsageAverage, costAllocationV1.cpuCoreUsageAverage) &&
Objects.equals(this.cpuCores, costAllocationV1.cpuCores) &&
Objects.equals(this.cpuCost, costAllocationV1.cpuCost) &&
Objects.equals(this.cpuCostAdjustment, costAllocationV1.cpuCostAdjustment) &&
Objects.equals(this.cpuEfficincy, costAllocationV1.cpuEfficincy) &&
Objects.equals(this.discount, costAllocationV1.discount) &&
Objects.equals(this.gpuCost, costAllocationV1.gpuCost) &&
Objects.equals(this.gpuCostAdjustment, costAllocationV1.gpuCostAdjustment) &&
Objects.equals(this.gpuCount, costAllocationV1.gpuCount) &&
Objects.equals(this.gpuHours, costAllocationV1.gpuHours) &&
Objects.equals(this.labels, costAllocationV1.labels) &&
Objects.equals(this.loadBalancerCost, costAllocationV1.loadBalancerCost) &&
Objects.equals(this.loadBalancerCostAdjustment, costAllocationV1.loadBalancerCostAdjustment) &&
Objects.equals(this.name, costAllocationV1.name) &&
Objects.equals(this.nodeType, costAllocationV1.nodeType) &&
Objects.equals(this.pv, costAllocationV1.pv) &&
Objects.equals(this.ramCost, costAllocationV1.ramCost) &&
Objects.equals(this.ramCostAdjustment, costAllocationV1.ramCostAdjustment) &&
Objects.equals(this.totalCost, costAllocationV1.totalCost) &&
Objects.equals(this.window, costAllocationV1.window);
}
@Override
public int hashCode() {
return Objects.hash(cpuCoreHours, cpuCoreRequestAverage, cpuCoreUsageAverage, cpuCores, cpuCost, cpuCostAdjustment, cpuEfficincy, discount, gpuCost, gpuCostAdjustment, gpuCount, gpuHours, labels, loadBalancerCost, loadBalancerCostAdjustment, name, nodeType, pv, ramCost, ramCostAdjustment, totalCost, window);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CostAllocationV1 {\n");
sb.append(" cpuCoreHours: ").append(toIndentedString(cpuCoreHours)).append("\n");
sb.append(" cpuCoreRequestAverage: ").append(toIndentedString(cpuCoreRequestAverage)).append("\n");
sb.append(" cpuCoreUsageAverage: ").append(toIndentedString(cpuCoreUsageAverage)).append("\n");
sb.append(" cpuCores: ").append(toIndentedString(cpuCores)).append("\n");
sb.append(" cpuCost: ").append(toIndentedString(cpuCost)).append("\n");
sb.append(" cpuCostAdjustment: ").append(toIndentedString(cpuCostAdjustment)).append("\n");
sb.append(" cpuEfficincy: ").append(toIndentedString(cpuEfficincy)).append("\n");
sb.append(" discount: ").append(toIndentedString(discount)).append("\n");
sb.append(" gpuCost: ").append(toIndentedString(gpuCost)).append("\n");
sb.append(" gpuCostAdjustment: ").append(toIndentedString(gpuCostAdjustment)).append("\n");
sb.append(" gpuCount: ").append(toIndentedString(gpuCount)).append("\n");
sb.append(" gpuHours: ").append(toIndentedString(gpuHours)).append("\n");
sb.append(" labels: ").append(toIndentedString(labels)).append("\n");
sb.append(" loadBalancerCost: ").append(toIndentedString(loadBalancerCost)).append("\n");
sb.append(" loadBalancerCostAdjustment: ").append(toIndentedString(loadBalancerCostAdjustment)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" nodeType: ").append(toIndentedString(nodeType)).append("\n");
sb.append(" pv: ").append(toIndentedString(pv)).append("\n");
sb.append(" ramCost: ").append(toIndentedString(ramCost)).append("\n");
sb.append(" ramCostAdjustment: ").append(toIndentedString(ramCostAdjustment)).append("\n");
sb.append(" totalCost: ").append(toIndentedString(totalCost)).append("\n");
sb.append(" window: ").append(toIndentedString(window)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `cpuCoreHours` to the URL query string
if (getCpuCoreHours() != null) {
joiner.add(String.format("%scpuCoreHours%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getCpuCoreHours()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `cpuCoreRequestAverage` to the URL query string
if (getCpuCoreRequestAverage() != null) {
joiner.add(String.format("%scpuCoreRequestAverage%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getCpuCoreRequestAverage()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `cpuCoreUsageAverage` to the URL query string
if (getCpuCoreUsageAverage() != null) {
joiner.add(String.format("%scpuCoreUsageAverage%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getCpuCoreUsageAverage()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `cpuCores` to the URL query string
if (getCpuCores() != null) {
joiner.add(String.format("%scpuCores%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getCpuCores()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `cpuCost` to the URL query string
if (getCpuCost() != null) {
joiner.add(String.format("%scpuCost%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getCpuCost()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `cpuCostAdjustment` to the URL query string
if (getCpuCostAdjustment() != null) {
joiner.add(String.format("%scpuCostAdjustment%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getCpuCostAdjustment()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `cpuEfficincy` to the URL query string
if (getCpuEfficincy() != null) {
joiner.add(String.format("%scpuEfficincy%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getCpuEfficincy()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `discount` to the URL query string
if (getDiscount() != null) {
joiner.add(String.format("%sdiscount%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getDiscount()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `gpuCost` to the URL query string
if (getGpuCost() != null) {
joiner.add(String.format("%sgpuCost%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getGpuCost()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `gpuCostAdjustment` to the URL query string
if (getGpuCostAdjustment() != null) {
joiner.add(String.format("%sgpuCostAdjustment%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getGpuCostAdjustment()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `gpuCount` to the URL query string
if (getGpuCount() != null) {
joiner.add(String.format("%sgpuCount%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getGpuCount()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `gpuHours` to the URL query string
if (getGpuHours() != null) {
joiner.add(String.format("%sgpuHours%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getGpuHours()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `labels` to the URL query string
if (getLabels() != null) {
joiner.add(getLabels().toUrlQueryString(prefix + "labels" + suffix));
}
// add `loadBalancerCost` to the URL query string
if (getLoadBalancerCost() != null) {
joiner.add(String.format("%sloadBalancerCost%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getLoadBalancerCost()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `loadBalancerCostAdjustment` to the URL query string
if (getLoadBalancerCostAdjustment() != null) {
joiner.add(String.format("%sloadBalancerCostAdjustment%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getLoadBalancerCostAdjustment()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `name` to the URL query string
if (getName() != null) {
joiner.add(String.format("%sname%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getName()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `nodeType` to the URL query string
if (getNodeType() != null) {
joiner.add(String.format("%snodeType%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getNodeType()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `pv` to the URL query string
if (getPv() != null) {
joiner.add(getPv().toUrlQueryString(prefix + "pv" + suffix));
}
// add `ramCost` to the URL query string
if (getRamCost() != null) {
joiner.add(String.format("%sramCost%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getRamCost()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `ramCostAdjustment` to the URL query string
if (getRamCostAdjustment() != null) {
joiner.add(String.format("%sramCostAdjustment%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getRamCostAdjustment()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `totalCost` to the URL query string
if (getTotalCost() != null) {
joiner.add(String.format("%stotalCost%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getTotalCost()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `window` to the URL query string
if (getWindow() != null) {
joiner.add(getWindow().toUrlQueryString(prefix + "window" + suffix));
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy