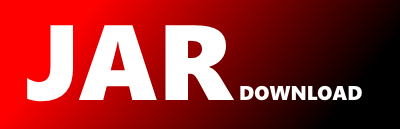
com.dominodatalab.pub.model.CostAllocationV1Pv Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of domino-java-client Show documentation
Show all versions of domino-java-client Show documentation
Domino Data Lab API Client to connect to Domino web services using Java HTTP Client.
/*
* Domino Public API
* Domino Public API Endpoints
*
* The version of the OpenAPI document: 0.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.pub.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.dominodatalab.pub.model.CostAllocationV1PvPvsValue;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.Arrays;
import java.util.HashMap;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.dominodatalab.pub.invoker.ApiClient;
/**
* CostAllocationV1Pv
*/
@JsonPropertyOrder({
CostAllocationV1Pv.JSON_PROPERTY_PV_BYTE_HOURS,
CostAllocationV1Pv.JSON_PROPERTY_PV_BYTES,
CostAllocationV1Pv.JSON_PROPERTY_PV_COST,
CostAllocationV1Pv.JSON_PROPERTY_PV_COST_ADJUSTMENT,
CostAllocationV1Pv.JSON_PROPERTY_PVS
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-10-04T16:37:28.765500600-04:00[America/New_York]", comments = "Generator version: 7.8.0")
public class CostAllocationV1Pv {
public static final String JSON_PROPERTY_PV_BYTE_HOURS = "pvByteHours";
private Double pvByteHours;
public static final String JSON_PROPERTY_PV_BYTES = "pvBytes";
private Double pvBytes;
public static final String JSON_PROPERTY_PV_COST = "pvCost";
private Double pvCost;
public static final String JSON_PROPERTY_PV_COST_ADJUSTMENT = "pvCostAdjustment";
private Double pvCostAdjustment;
public static final String JSON_PROPERTY_PVS = "pvs";
private Map pvs = new HashMap<>();
public CostAllocationV1Pv() {
}
public CostAllocationV1Pv pvByteHours(Double pvByteHours) {
this.pvByteHours = pvByteHours;
return this;
}
/**
* Get pvByteHours
* @return pvByteHours
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_PV_BYTE_HOURS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getPvByteHours() {
return pvByteHours;
}
@JsonProperty(JSON_PROPERTY_PV_BYTE_HOURS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPvByteHours(Double pvByteHours) {
this.pvByteHours = pvByteHours;
}
public CostAllocationV1Pv pvBytes(Double pvBytes) {
this.pvBytes = pvBytes;
return this;
}
/**
* Get pvBytes
* @return pvBytes
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_PV_BYTES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getPvBytes() {
return pvBytes;
}
@JsonProperty(JSON_PROPERTY_PV_BYTES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPvBytes(Double pvBytes) {
this.pvBytes = pvBytes;
}
public CostAllocationV1Pv pvCost(Double pvCost) {
this.pvCost = pvCost;
return this;
}
/**
* Get pvCost
* @return pvCost
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_PV_COST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getPvCost() {
return pvCost;
}
@JsonProperty(JSON_PROPERTY_PV_COST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPvCost(Double pvCost) {
this.pvCost = pvCost;
}
public CostAllocationV1Pv pvCostAdjustment(Double pvCostAdjustment) {
this.pvCostAdjustment = pvCostAdjustment;
return this;
}
/**
* Get pvCostAdjustment
* @return pvCostAdjustment
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_PV_COST_ADJUSTMENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getPvCostAdjustment() {
return pvCostAdjustment;
}
@JsonProperty(JSON_PROPERTY_PV_COST_ADJUSTMENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPvCostAdjustment(Double pvCostAdjustment) {
this.pvCostAdjustment = pvCostAdjustment;
}
public CostAllocationV1Pv pvs(Map pvs) {
this.pvs = pvs;
return this;
}
public CostAllocationV1Pv putPvsItem(String key, CostAllocationV1PvPvsValue pvsItem) {
if (this.pvs == null) {
this.pvs = new HashMap<>();
}
this.pvs.put(key, pvsItem);
return this;
}
/**
* Get pvs
* @return pvs
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_PVS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Map getPvs() {
return pvs;
}
@JsonProperty(JSON_PROPERTY_PVS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPvs(Map pvs) {
this.pvs = pvs;
}
/**
* Return true if this CostAllocationV1_pv object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CostAllocationV1Pv costAllocationV1Pv = (CostAllocationV1Pv) o;
return Objects.equals(this.pvByteHours, costAllocationV1Pv.pvByteHours) &&
Objects.equals(this.pvBytes, costAllocationV1Pv.pvBytes) &&
Objects.equals(this.pvCost, costAllocationV1Pv.pvCost) &&
Objects.equals(this.pvCostAdjustment, costAllocationV1Pv.pvCostAdjustment) &&
Objects.equals(this.pvs, costAllocationV1Pv.pvs);
}
@Override
public int hashCode() {
return Objects.hash(pvByteHours, pvBytes, pvCost, pvCostAdjustment, pvs);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CostAllocationV1Pv {\n");
sb.append(" pvByteHours: ").append(toIndentedString(pvByteHours)).append("\n");
sb.append(" pvBytes: ").append(toIndentedString(pvBytes)).append("\n");
sb.append(" pvCost: ").append(toIndentedString(pvCost)).append("\n");
sb.append(" pvCostAdjustment: ").append(toIndentedString(pvCostAdjustment)).append("\n");
sb.append(" pvs: ").append(toIndentedString(pvs)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `pvByteHours` to the URL query string
if (getPvByteHours() != null) {
joiner.add(String.format("%spvByteHours%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getPvByteHours()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `pvBytes` to the URL query string
if (getPvBytes() != null) {
joiner.add(String.format("%spvBytes%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getPvBytes()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `pvCost` to the URL query string
if (getPvCost() != null) {
joiner.add(String.format("%spvCost%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getPvCost()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `pvCostAdjustment` to the URL query string
if (getPvCostAdjustment() != null) {
joiner.add(String.format("%spvCostAdjustment%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getPvCostAdjustment()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `pvs` to the URL query string
if (getPvs() != null) {
for (String _key : getPvs().keySet()) {
if (getPvs().get(_key) != null) {
joiner.add(getPvs().get(_key).toUrlQueryString(String.format("%spvs%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, _key, containerSuffix))));
}
}
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy