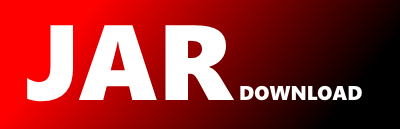
com.dominodatalab.pub.model.CostAssetsV1 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of domino-java-client Show documentation
Show all versions of domino-java-client Show documentation
Domino Data Lab API Client to connect to Domino web services using Java HTTP Client.
/*
* Domino Public API
* Domino Public API Endpoints
*
* The version of the OpenAPI document: 0.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.pub.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.dominodatalab.pub.model.CostAssetsV1Labels;
import com.dominodatalab.pub.model.CostAssetsV1Properties;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.dominodatalab.pub.invoker.ApiClient;
/**
* CostAssetsV1
*/
@JsonPropertyOrder({
CostAssetsV1.JSON_PROPERTY_CPU_COST,
CostAssetsV1.JSON_PROPERTY_GPU_COST,
CostAssetsV1.JSON_PROPERTY_LABELS,
CostAssetsV1.JSON_PROPERTY_NAME,
CostAssetsV1.JSON_PROPERTY_PROPERTIES,
CostAssetsV1.JSON_PROPERTY_RAM_COST,
CostAssetsV1.JSON_PROPERTY_TOTAL_COST,
CostAssetsV1.JSON_PROPERTY_TYPE,
CostAssetsV1.JSON_PROPERTY_WINDOW
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-10-04T16:37:28.765500600-04:00[America/New_York]", comments = "Generator version: 7.8.0")
public class CostAssetsV1 {
public static final String JSON_PROPERTY_CPU_COST = "cpuCost";
private Double cpuCost;
public static final String JSON_PROPERTY_GPU_COST = "gpuCost";
private Double gpuCost;
public static final String JSON_PROPERTY_LABELS = "labels";
private CostAssetsV1Labels labels;
public static final String JSON_PROPERTY_NAME = "name";
private String name;
public static final String JSON_PROPERTY_PROPERTIES = "properties";
private CostAssetsV1Properties properties;
public static final String JSON_PROPERTY_RAM_COST = "ramCost";
private Double ramCost;
public static final String JSON_PROPERTY_TOTAL_COST = "totalCost";
private Double totalCost;
public static final String JSON_PROPERTY_TYPE = "type";
private String type;
public static final String JSON_PROPERTY_WINDOW = "window";
private Object window;
public CostAssetsV1() {
}
public CostAssetsV1 cpuCost(Double cpuCost) {
this.cpuCost = cpuCost;
return this;
}
/**
* Get cpuCost
* @return cpuCost
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_CPU_COST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getCpuCost() {
return cpuCost;
}
@JsonProperty(JSON_PROPERTY_CPU_COST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCpuCost(Double cpuCost) {
this.cpuCost = cpuCost;
}
public CostAssetsV1 gpuCost(Double gpuCost) {
this.gpuCost = gpuCost;
return this;
}
/**
* Get gpuCost
* @return gpuCost
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_GPU_COST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getGpuCost() {
return gpuCost;
}
@JsonProperty(JSON_PROPERTY_GPU_COST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setGpuCost(Double gpuCost) {
this.gpuCost = gpuCost;
}
public CostAssetsV1 labels(CostAssetsV1Labels labels) {
this.labels = labels;
return this;
}
/**
* Get labels
* @return labels
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_LABELS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public CostAssetsV1Labels getLabels() {
return labels;
}
@JsonProperty(JSON_PROPERTY_LABELS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLabels(CostAssetsV1Labels labels) {
this.labels = labels;
}
public CostAssetsV1 name(String name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getName() {
return name;
}
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setName(String name) {
this.name = name;
}
public CostAssetsV1 properties(CostAssetsV1Properties properties) {
this.properties = properties;
return this;
}
/**
* Get properties
* @return properties
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_PROPERTIES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public CostAssetsV1Properties getProperties() {
return properties;
}
@JsonProperty(JSON_PROPERTY_PROPERTIES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setProperties(CostAssetsV1Properties properties) {
this.properties = properties;
}
public CostAssetsV1 ramCost(Double ramCost) {
this.ramCost = ramCost;
return this;
}
/**
* Get ramCost
* @return ramCost
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_RAM_COST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getRamCost() {
return ramCost;
}
@JsonProperty(JSON_PROPERTY_RAM_COST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRamCost(Double ramCost) {
this.ramCost = ramCost;
}
public CostAssetsV1 totalCost(Double totalCost) {
this.totalCost = totalCost;
return this;
}
/**
* Get totalCost
* @return totalCost
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_TOTAL_COST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getTotalCost() {
return totalCost;
}
@JsonProperty(JSON_PROPERTY_TOTAL_COST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTotalCost(Double totalCost) {
this.totalCost = totalCost;
}
public CostAssetsV1 type(String type) {
this.type = type;
return this;
}
/**
* Get type
* @return type
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getType() {
return type;
}
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setType(String type) {
this.type = type;
}
public CostAssetsV1 window(Object window) {
this.window = window;
return this;
}
/**
* Get window
* @return window
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_WINDOW)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Object getWindow() {
return window;
}
@JsonProperty(JSON_PROPERTY_WINDOW)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setWindow(Object window) {
this.window = window;
}
/**
* Return true if this CostAssetsV1 object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CostAssetsV1 costAssetsV1 = (CostAssetsV1) o;
return Objects.equals(this.cpuCost, costAssetsV1.cpuCost) &&
Objects.equals(this.gpuCost, costAssetsV1.gpuCost) &&
Objects.equals(this.labels, costAssetsV1.labels) &&
Objects.equals(this.name, costAssetsV1.name) &&
Objects.equals(this.properties, costAssetsV1.properties) &&
Objects.equals(this.ramCost, costAssetsV1.ramCost) &&
Objects.equals(this.totalCost, costAssetsV1.totalCost) &&
Objects.equals(this.type, costAssetsV1.type) &&
Objects.equals(this.window, costAssetsV1.window);
}
@Override
public int hashCode() {
return Objects.hash(cpuCost, gpuCost, labels, name, properties, ramCost, totalCost, type, window);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CostAssetsV1 {\n");
sb.append(" cpuCost: ").append(toIndentedString(cpuCost)).append("\n");
sb.append(" gpuCost: ").append(toIndentedString(gpuCost)).append("\n");
sb.append(" labels: ").append(toIndentedString(labels)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" properties: ").append(toIndentedString(properties)).append("\n");
sb.append(" ramCost: ").append(toIndentedString(ramCost)).append("\n");
sb.append(" totalCost: ").append(toIndentedString(totalCost)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" window: ").append(toIndentedString(window)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `cpuCost` to the URL query string
if (getCpuCost() != null) {
joiner.add(String.format("%scpuCost%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getCpuCost()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `gpuCost` to the URL query string
if (getGpuCost() != null) {
joiner.add(String.format("%sgpuCost%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getGpuCost()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `labels` to the URL query string
if (getLabels() != null) {
joiner.add(getLabels().toUrlQueryString(prefix + "labels" + suffix));
}
// add `name` to the URL query string
if (getName() != null) {
joiner.add(String.format("%sname%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getName()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `properties` to the URL query string
if (getProperties() != null) {
joiner.add(getProperties().toUrlQueryString(prefix + "properties" + suffix));
}
// add `ramCost` to the URL query string
if (getRamCost() != null) {
joiner.add(String.format("%sramCost%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getRamCost()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `totalCost` to the URL query string
if (getTotalCost() != null) {
joiner.add(String.format("%stotalCost%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getTotalCost()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `type` to the URL query string
if (getType() != null) {
joiner.add(String.format("%stype%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getType()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `window` to the URL query string
if (getWindow() != null) {
joiner.add(String.format("%swindow%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getWindow()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy