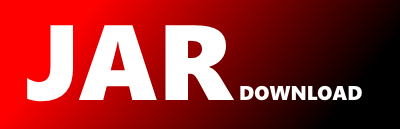
com.dominodatalab.pub.model.DatasetMountV1 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of domino-java-client Show documentation
Show all versions of domino-java-client Show documentation
Domino Data Lab API Client to connect to Domino web services using Java HTTP Client.
/*
* Domino Public API
* Domino Public API Endpoints
*
* The version of the OpenAPI document: 0.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.pub.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.dominodatalab.pub.invoker.ApiClient;
/**
* DatasetMountV1
*/
@JsonPropertyOrder({
DatasetMountV1.JSON_PROPERTY_CONTAINER_PATH,
DatasetMountV1.JSON_PROPERTY_DATASET_NAME,
DatasetMountV1.JSON_PROPERTY_ID,
DatasetMountV1.JSON_PROPERTY_IS_INPUT,
DatasetMountV1.JSON_PROPERTY_PROJECT_ID,
DatasetMountV1.JSON_PROPERTY_SNAPSHOT_ID,
DatasetMountV1.JSON_PROPERTY_SNAPSHOT_VERSION
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-10-04T16:37:28.765500600-04:00[America/New_York]", comments = "Generator version: 7.8.0")
public class DatasetMountV1 {
public static final String JSON_PROPERTY_CONTAINER_PATH = "containerPath";
private String containerPath;
public static final String JSON_PROPERTY_DATASET_NAME = "datasetName";
private String datasetName;
public static final String JSON_PROPERTY_ID = "id";
private String id;
public static final String JSON_PROPERTY_IS_INPUT = "isInput";
private Boolean isInput;
public static final String JSON_PROPERTY_PROJECT_ID = "projectId";
private String projectId;
public static final String JSON_PROPERTY_SNAPSHOT_ID = "snapshotId";
private String snapshotId;
public static final String JSON_PROPERTY_SNAPSHOT_VERSION = "snapshotVersion";
private Integer snapshotVersion;
public DatasetMountV1() {
}
public DatasetMountV1 containerPath(String containerPath) {
this.containerPath = containerPath;
return this;
}
/**
* Location dataset is mounted at in the Job.
* @return containerPath
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_CONTAINER_PATH)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getContainerPath() {
return containerPath;
}
@JsonProperty(JSON_PROPERTY_CONTAINER_PATH)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setContainerPath(String containerPath) {
this.containerPath = containerPath;
}
public DatasetMountV1 datasetName(String datasetName) {
this.datasetName = datasetName;
return this;
}
/**
* Name of dataset to be mounted.
* @return datasetName
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_DATASET_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getDatasetName() {
return datasetName;
}
@JsonProperty(JSON_PROPERTY_DATASET_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDatasetName(String datasetName) {
this.datasetName = datasetName;
}
public DatasetMountV1 id(String id) {
this.id = id;
return this;
}
/**
* Id of dataset to be mounted.
* @return id
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getId() {
return id;
}
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setId(String id) {
this.id = id;
}
public DatasetMountV1 isInput(Boolean isInput) {
this.isInput = isInput;
return this;
}
/**
* Whether a dataset was an input to be used in the execution, or an output created by the execution.
* @return isInput
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_IS_INPUT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getIsInput() {
return isInput;
}
@JsonProperty(JSON_PROPERTY_IS_INPUT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setIsInput(Boolean isInput) {
this.isInput = isInput;
}
public DatasetMountV1 projectId(String projectId) {
this.projectId = projectId;
return this;
}
/**
* Id of project the dataset belongs to.
* @return projectId
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_PROJECT_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getProjectId() {
return projectId;
}
@JsonProperty(JSON_PROPERTY_PROJECT_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setProjectId(String projectId) {
this.projectId = projectId;
}
public DatasetMountV1 snapshotId(String snapshotId) {
this.snapshotId = snapshotId;
return this;
}
/**
* Id of snapshot to mount for this dataset.
* @return snapshotId
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_SNAPSHOT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getSnapshotId() {
return snapshotId;
}
@JsonProperty(JSON_PROPERTY_SNAPSHOT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSnapshotId(String snapshotId) {
this.snapshotId = snapshotId;
}
public DatasetMountV1 snapshotVersion(Integer snapshotVersion) {
this.snapshotVersion = snapshotVersion;
return this;
}
/**
* Version of dataset snapshot to mound.
* @return snapshotVersion
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_SNAPSHOT_VERSION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getSnapshotVersion() {
return snapshotVersion;
}
@JsonProperty(JSON_PROPERTY_SNAPSHOT_VERSION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSnapshotVersion(Integer snapshotVersion) {
this.snapshotVersion = snapshotVersion;
}
/**
* Return true if this DatasetMountV1 object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DatasetMountV1 datasetMountV1 = (DatasetMountV1) o;
return Objects.equals(this.containerPath, datasetMountV1.containerPath) &&
Objects.equals(this.datasetName, datasetMountV1.datasetName) &&
Objects.equals(this.id, datasetMountV1.id) &&
Objects.equals(this.isInput, datasetMountV1.isInput) &&
Objects.equals(this.projectId, datasetMountV1.projectId) &&
Objects.equals(this.snapshotId, datasetMountV1.snapshotId) &&
Objects.equals(this.snapshotVersion, datasetMountV1.snapshotVersion);
}
@Override
public int hashCode() {
return Objects.hash(containerPath, datasetName, id, isInput, projectId, snapshotId, snapshotVersion);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DatasetMountV1 {\n");
sb.append(" containerPath: ").append(toIndentedString(containerPath)).append("\n");
sb.append(" datasetName: ").append(toIndentedString(datasetName)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" isInput: ").append(toIndentedString(isInput)).append("\n");
sb.append(" projectId: ").append(toIndentedString(projectId)).append("\n");
sb.append(" snapshotId: ").append(toIndentedString(snapshotId)).append("\n");
sb.append(" snapshotVersion: ").append(toIndentedString(snapshotVersion)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `containerPath` to the URL query string
if (getContainerPath() != null) {
joiner.add(String.format("%scontainerPath%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getContainerPath()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `datasetName` to the URL query string
if (getDatasetName() != null) {
joiner.add(String.format("%sdatasetName%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getDatasetName()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `id` to the URL query string
if (getId() != null) {
joiner.add(String.format("%sid%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `isInput` to the URL query string
if (getIsInput() != null) {
joiner.add(String.format("%sisInput%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getIsInput()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `projectId` to the URL query string
if (getProjectId() != null) {
joiner.add(String.format("%sprojectId%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getProjectId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `snapshotId` to the URL query string
if (getSnapshotId() != null) {
joiner.add(String.format("%ssnapshotId%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getSnapshotId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `snapshotVersion` to the URL query string
if (getSnapshotVersion() != null) {
joiner.add(String.format("%ssnapshotVersion%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getSnapshotVersion()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy