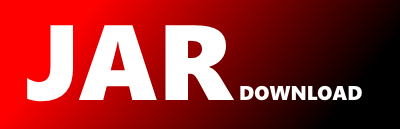
com.dominodatalab.pub.model.EnvironmentV1 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of domino-java-client Show documentation
Show all versions of domino-java-client Show documentation
Domino Data Lab API Client to connect to Domino web services using Java HTTP Client.
/*
* Domino Public API
* Domino Public API Endpoints
*
* The version of the OpenAPI document: 0.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.pub.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.dominodatalab.pub.model.ClusterTypeV1;
import com.dominodatalab.pub.model.EnvironmentOwnerV1;
import com.dominodatalab.pub.model.EnvironmentRevisionV1;
import com.dominodatalab.pub.model.EnvironmentVisibilityV1;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.dominodatalab.pub.invoker.ApiClient;
/**
* EnvironmentV1
*/
@JsonPropertyOrder({
EnvironmentV1.JSON_PROPERTY_ACTIVE_REVISION_TAGS,
EnvironmentV1.JSON_PROPERTY_ARCHIVED,
EnvironmentV1.JSON_PROPERTY_ID,
EnvironmentV1.JSON_PROPERTY_INTERNAL_TAGS,
EnvironmentV1.JSON_PROPERTY_IS_CURATED,
EnvironmentV1.JSON_PROPERTY_LATEST_REVISION,
EnvironmentV1.JSON_PROPERTY_NAME,
EnvironmentV1.JSON_PROPERTY_OWNER,
EnvironmentV1.JSON_PROPERTY_RESTRICTED_REVISION,
EnvironmentV1.JSON_PROPERTY_SELECTED_REVISION,
EnvironmentV1.JSON_PROPERTY_SUPPORTED_CLUSTERS,
EnvironmentV1.JSON_PROPERTY_VISIBILITY
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-10-04T16:37:28.765500600-04:00[America/New_York]", comments = "Generator version: 7.8.0")
public class EnvironmentV1 {
public static final String JSON_PROPERTY_ACTIVE_REVISION_TAGS = "activeRevisionTags";
private List activeRevisionTags = new ArrayList<>();
public static final String JSON_PROPERTY_ARCHIVED = "archived";
private Boolean archived;
public static final String JSON_PROPERTY_ID = "id";
private String id;
public static final String JSON_PROPERTY_INTERNAL_TAGS = "internalTags";
private List internalTags = new ArrayList<>();
public static final String JSON_PROPERTY_IS_CURATED = "isCurated";
private Boolean isCurated;
public static final String JSON_PROPERTY_LATEST_REVISION = "latestRevision";
private EnvironmentRevisionV1 latestRevision;
public static final String JSON_PROPERTY_NAME = "name";
private String name;
public static final String JSON_PROPERTY_OWNER = "owner";
private EnvironmentOwnerV1 owner;
public static final String JSON_PROPERTY_RESTRICTED_REVISION = "restrictedRevision";
private EnvironmentRevisionV1 restrictedRevision;
public static final String JSON_PROPERTY_SELECTED_REVISION = "selectedRevision";
private EnvironmentRevisionV1 selectedRevision;
public static final String JSON_PROPERTY_SUPPORTED_CLUSTERS = "supportedClusters";
private List supportedClusters = new ArrayList<>();
public static final String JSON_PROPERTY_VISIBILITY = "visibility";
private EnvironmentVisibilityV1 visibility;
public EnvironmentV1() {
}
public EnvironmentV1 activeRevisionTags(List activeRevisionTags) {
this.activeRevisionTags = activeRevisionTags;
return this;
}
public EnvironmentV1 addActiveRevisionTagsItem(String activeRevisionTagsItem) {
if (this.activeRevisionTags == null) {
this.activeRevisionTags = new ArrayList<>();
}
this.activeRevisionTags.add(activeRevisionTagsItem);
return this;
}
/**
* The tags on the active revision for this environment
* @return activeRevisionTags
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_ACTIVE_REVISION_TAGS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getActiveRevisionTags() {
return activeRevisionTags;
}
@JsonProperty(JSON_PROPERTY_ACTIVE_REVISION_TAGS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setActiveRevisionTags(List activeRevisionTags) {
this.activeRevisionTags = activeRevisionTags;
}
public EnvironmentV1 archived(Boolean archived) {
this.archived = archived;
return this;
}
/**
* Whether the environment is archived
* @return archived
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ARCHIVED)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getArchived() {
return archived;
}
@JsonProperty(JSON_PROPERTY_ARCHIVED)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setArchived(Boolean archived) {
this.archived = archived;
}
public EnvironmentV1 id(String id) {
this.id = id;
return this;
}
/**
* Id of environment
* @return id
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getId() {
return id;
}
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setId(String id) {
this.id = id;
}
public EnvironmentV1 internalTags(List internalTags) {
this.internalTags = internalTags;
return this;
}
public EnvironmentV1 addInternalTagsItem(String internalTagsItem) {
if (this.internalTags == null) {
this.internalTags = new ArrayList<>();
}
this.internalTags.add(internalTagsItem);
return this;
}
/**
* The internal tags specifying if this environment is restricted
* @return internalTags
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_INTERNAL_TAGS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getInternalTags() {
return internalTags;
}
@JsonProperty(JSON_PROPERTY_INTERNAL_TAGS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setInternalTags(List internalTags) {
this.internalTags = internalTags;
}
public EnvironmentV1 isCurated(Boolean isCurated) {
this.isCurated = isCurated;
return this;
}
/**
* Whether or not the environment is curated for a deployment
* @return isCurated
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_IS_CURATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getIsCurated() {
return isCurated;
}
@JsonProperty(JSON_PROPERTY_IS_CURATED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIsCurated(Boolean isCurated) {
this.isCurated = isCurated;
}
public EnvironmentV1 latestRevision(EnvironmentRevisionV1 latestRevision) {
this.latestRevision = latestRevision;
return this;
}
/**
* Get latestRevision
* @return latestRevision
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_LATEST_REVISION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public EnvironmentRevisionV1 getLatestRevision() {
return latestRevision;
}
@JsonProperty(JSON_PROPERTY_LATEST_REVISION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLatestRevision(EnvironmentRevisionV1 latestRevision) {
this.latestRevision = latestRevision;
}
public EnvironmentV1 name(String name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getName() {
return name;
}
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setName(String name) {
this.name = name;
}
public EnvironmentV1 owner(EnvironmentOwnerV1 owner) {
this.owner = owner;
return this;
}
/**
* Get owner
* @return owner
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_OWNER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public EnvironmentOwnerV1 getOwner() {
return owner;
}
@JsonProperty(JSON_PROPERTY_OWNER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setOwner(EnvironmentOwnerV1 owner) {
this.owner = owner;
}
public EnvironmentV1 restrictedRevision(EnvironmentRevisionV1 restrictedRevision) {
this.restrictedRevision = restrictedRevision;
return this;
}
/**
* Get restrictedRevision
* @return restrictedRevision
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_RESTRICTED_REVISION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public EnvironmentRevisionV1 getRestrictedRevision() {
return restrictedRevision;
}
@JsonProperty(JSON_PROPERTY_RESTRICTED_REVISION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRestrictedRevision(EnvironmentRevisionV1 restrictedRevision) {
this.restrictedRevision = restrictedRevision;
}
public EnvironmentV1 selectedRevision(EnvironmentRevisionV1 selectedRevision) {
this.selectedRevision = selectedRevision;
return this;
}
/**
* Get selectedRevision
* @return selectedRevision
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_SELECTED_REVISION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public EnvironmentRevisionV1 getSelectedRevision() {
return selectedRevision;
}
@JsonProperty(JSON_PROPERTY_SELECTED_REVISION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSelectedRevision(EnvironmentRevisionV1 selectedRevision) {
this.selectedRevision = selectedRevision;
}
public EnvironmentV1 supportedClusters(List supportedClusters) {
this.supportedClusters = supportedClusters;
return this;
}
public EnvironmentV1 addSupportedClustersItem(ClusterTypeV1 supportedClustersItem) {
if (this.supportedClusters == null) {
this.supportedClusters = new ArrayList<>();
}
this.supportedClusters.add(supportedClustersItem);
return this;
}
/**
* Get supportedClusters
* @return supportedClusters
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_SUPPORTED_CLUSTERS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getSupportedClusters() {
return supportedClusters;
}
@JsonProperty(JSON_PROPERTY_SUPPORTED_CLUSTERS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setSupportedClusters(List supportedClusters) {
this.supportedClusters = supportedClusters;
}
public EnvironmentV1 visibility(EnvironmentVisibilityV1 visibility) {
this.visibility = visibility;
return this;
}
/**
* Get visibility
* @return visibility
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_VISIBILITY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public EnvironmentVisibilityV1 getVisibility() {
return visibility;
}
@JsonProperty(JSON_PROPERTY_VISIBILITY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setVisibility(EnvironmentVisibilityV1 visibility) {
this.visibility = visibility;
}
/**
* Return true if this EnvironmentV1 object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
EnvironmentV1 environmentV1 = (EnvironmentV1) o;
return Objects.equals(this.activeRevisionTags, environmentV1.activeRevisionTags) &&
Objects.equals(this.archived, environmentV1.archived) &&
Objects.equals(this.id, environmentV1.id) &&
Objects.equals(this.internalTags, environmentV1.internalTags) &&
Objects.equals(this.isCurated, environmentV1.isCurated) &&
Objects.equals(this.latestRevision, environmentV1.latestRevision) &&
Objects.equals(this.name, environmentV1.name) &&
Objects.equals(this.owner, environmentV1.owner) &&
Objects.equals(this.restrictedRevision, environmentV1.restrictedRevision) &&
Objects.equals(this.selectedRevision, environmentV1.selectedRevision) &&
Objects.equals(this.supportedClusters, environmentV1.supportedClusters) &&
Objects.equals(this.visibility, environmentV1.visibility);
}
@Override
public int hashCode() {
return Objects.hash(activeRevisionTags, archived, id, internalTags, isCurated, latestRevision, name, owner, restrictedRevision, selectedRevision, supportedClusters, visibility);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class EnvironmentV1 {\n");
sb.append(" activeRevisionTags: ").append(toIndentedString(activeRevisionTags)).append("\n");
sb.append(" archived: ").append(toIndentedString(archived)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" internalTags: ").append(toIndentedString(internalTags)).append("\n");
sb.append(" isCurated: ").append(toIndentedString(isCurated)).append("\n");
sb.append(" latestRevision: ").append(toIndentedString(latestRevision)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" owner: ").append(toIndentedString(owner)).append("\n");
sb.append(" restrictedRevision: ").append(toIndentedString(restrictedRevision)).append("\n");
sb.append(" selectedRevision: ").append(toIndentedString(selectedRevision)).append("\n");
sb.append(" supportedClusters: ").append(toIndentedString(supportedClusters)).append("\n");
sb.append(" visibility: ").append(toIndentedString(visibility)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `activeRevisionTags` to the URL query string
if (getActiveRevisionTags() != null) {
for (int i = 0; i < getActiveRevisionTags().size(); i++) {
joiner.add(String.format("%sactiveRevisionTags%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(ApiClient.valueToString(getActiveRevisionTags().get(i)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
// add `archived` to the URL query string
if (getArchived() != null) {
joiner.add(String.format("%sarchived%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getArchived()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `id` to the URL query string
if (getId() != null) {
joiner.add(String.format("%sid%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `internalTags` to the URL query string
if (getInternalTags() != null) {
for (int i = 0; i < getInternalTags().size(); i++) {
joiner.add(String.format("%sinternalTags%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(ApiClient.valueToString(getInternalTags().get(i)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
// add `isCurated` to the URL query string
if (getIsCurated() != null) {
joiner.add(String.format("%sisCurated%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getIsCurated()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `latestRevision` to the URL query string
if (getLatestRevision() != null) {
joiner.add(getLatestRevision().toUrlQueryString(prefix + "latestRevision" + suffix));
}
// add `name` to the URL query string
if (getName() != null) {
joiner.add(String.format("%sname%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getName()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `owner` to the URL query string
if (getOwner() != null) {
joiner.add(getOwner().toUrlQueryString(prefix + "owner" + suffix));
}
// add `restrictedRevision` to the URL query string
if (getRestrictedRevision() != null) {
joiner.add(getRestrictedRevision().toUrlQueryString(prefix + "restrictedRevision" + suffix));
}
// add `selectedRevision` to the URL query string
if (getSelectedRevision() != null) {
joiner.add(getSelectedRevision().toUrlQueryString(prefix + "selectedRevision" + suffix));
}
// add `supportedClusters` to the URL query string
if (getSupportedClusters() != null) {
for (int i = 0; i < getSupportedClusters().size(); i++) {
if (getSupportedClusters().get(i) != null) {
joiner.add(String.format("%ssupportedClusters%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(ApiClient.valueToString(getSupportedClusters().get(i)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
}
// add `visibility` to the URL query string
if (getVisibility() != null) {
joiner.add(String.format("%svisibility%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getVisibility()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy