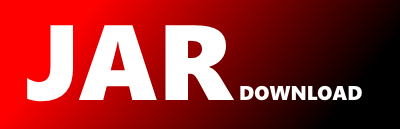
com.dominodatalab.pub.model.HardwareTierCapacityV1 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of domino-java-client Show documentation
Show all versions of domino-java-client Show documentation
Domino Data Lab API Client to connect to Domino web services using Java HTTP Client.
/*
* Domino Public API
* Domino Public API Endpoints
*
* The version of the OpenAPI document: 0.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.pub.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.dominodatalab.pub.invoker.ApiClient;
/**
* Current capacity information for a hardware tier. Note: Not necessary on requests to update a hardware tier.
*/
@JsonPropertyOrder({
HardwareTierCapacityV1.JSON_PROPERTY_AVAILABLE_CAPACITY_WITHOUT_LAUNCHING,
HardwareTierCapacityV1.JSON_PROPERTY_CAPACITY_LEVEL,
HardwareTierCapacityV1.JSON_PROPERTY_EXECUTING_RUNS,
HardwareTierCapacityV1.JSON_PROPERTY_MAX_AVAILABLE_CAPACITY,
HardwareTierCapacityV1.JSON_PROPERTY_MAX_CONCURRENT_RUNS,
HardwareTierCapacityV1.JSON_PROPERTY_MAX_NUMBER_OF_EXECUTORS,
HardwareTierCapacityV1.JSON_PROPERTY_NUMBER_OF_EXECUTORS,
HardwareTierCapacityV1.JSON_PROPERTY_QUEUED_RUNS
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-10-04T16:37:28.765500600-04:00[America/New_York]", comments = "Generator version: 7.8.0")
public class HardwareTierCapacityV1 {
public static final String JSON_PROPERTY_AVAILABLE_CAPACITY_WITHOUT_LAUNCHING = "availableCapacityWithoutLaunching";
private Integer availableCapacityWithoutLaunching;
/**
* Gets or Sets capacityLevel
*/
public enum CapacityLevelEnum {
CAN_EXECUTE_WITH_CURRENT_INSTANCES("CanExecuteWithCurrentInstances"),
REQUIRES_LAUNCHING_INSTANCE("RequiresLaunchingInstance"),
FULL("Full"),
UNKNOWN("Unknown");
private String value;
CapacityLevelEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static CapacityLevelEnum fromValue(String value) {
for (CapacityLevelEnum b : CapacityLevelEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_CAPACITY_LEVEL = "capacityLevel";
private CapacityLevelEnum capacityLevel;
public static final String JSON_PROPERTY_EXECUTING_RUNS = "executingRuns";
private Integer executingRuns;
public static final String JSON_PROPERTY_MAX_AVAILABLE_CAPACITY = "maxAvailableCapacity";
private Integer maxAvailableCapacity;
public static final String JSON_PROPERTY_MAX_CONCURRENT_RUNS = "maxConcurrentRuns";
private Integer maxConcurrentRuns;
public static final String JSON_PROPERTY_MAX_NUMBER_OF_EXECUTORS = "maxNumberOfExecutors";
private Integer maxNumberOfExecutors;
public static final String JSON_PROPERTY_NUMBER_OF_EXECUTORS = "numberOfExecutors";
private Integer numberOfExecutors;
public static final String JSON_PROPERTY_QUEUED_RUNS = "queuedRuns";
private Integer queuedRuns;
public HardwareTierCapacityV1() {
}
public HardwareTierCapacityV1 availableCapacityWithoutLaunching(Integer availableCapacityWithoutLaunching) {
this.availableCapacityWithoutLaunching = availableCapacityWithoutLaunching;
return this;
}
/**
* Get availableCapacityWithoutLaunching
* @return availableCapacityWithoutLaunching
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_AVAILABLE_CAPACITY_WITHOUT_LAUNCHING)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Integer getAvailableCapacityWithoutLaunching() {
return availableCapacityWithoutLaunching;
}
@JsonProperty(JSON_PROPERTY_AVAILABLE_CAPACITY_WITHOUT_LAUNCHING)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setAvailableCapacityWithoutLaunching(Integer availableCapacityWithoutLaunching) {
this.availableCapacityWithoutLaunching = availableCapacityWithoutLaunching;
}
public HardwareTierCapacityV1 capacityLevel(CapacityLevelEnum capacityLevel) {
this.capacityLevel = capacityLevel;
return this;
}
/**
* Get capacityLevel
* @return capacityLevel
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_CAPACITY_LEVEL)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public CapacityLevelEnum getCapacityLevel() {
return capacityLevel;
}
@JsonProperty(JSON_PROPERTY_CAPACITY_LEVEL)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setCapacityLevel(CapacityLevelEnum capacityLevel) {
this.capacityLevel = capacityLevel;
}
public HardwareTierCapacityV1 executingRuns(Integer executingRuns) {
this.executingRuns = executingRuns;
return this;
}
/**
* Get executingRuns
* @return executingRuns
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_EXECUTING_RUNS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Integer getExecutingRuns() {
return executingRuns;
}
@JsonProperty(JSON_PROPERTY_EXECUTING_RUNS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setExecutingRuns(Integer executingRuns) {
this.executingRuns = executingRuns;
}
public HardwareTierCapacityV1 maxAvailableCapacity(Integer maxAvailableCapacity) {
this.maxAvailableCapacity = maxAvailableCapacity;
return this;
}
/**
* Get maxAvailableCapacity
* @return maxAvailableCapacity
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_MAX_AVAILABLE_CAPACITY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Integer getMaxAvailableCapacity() {
return maxAvailableCapacity;
}
@JsonProperty(JSON_PROPERTY_MAX_AVAILABLE_CAPACITY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setMaxAvailableCapacity(Integer maxAvailableCapacity) {
this.maxAvailableCapacity = maxAvailableCapacity;
}
public HardwareTierCapacityV1 maxConcurrentRuns(Integer maxConcurrentRuns) {
this.maxConcurrentRuns = maxConcurrentRuns;
return this;
}
/**
* Get maxConcurrentRuns
* @return maxConcurrentRuns
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_MAX_CONCURRENT_RUNS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Integer getMaxConcurrentRuns() {
return maxConcurrentRuns;
}
@JsonProperty(JSON_PROPERTY_MAX_CONCURRENT_RUNS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setMaxConcurrentRuns(Integer maxConcurrentRuns) {
this.maxConcurrentRuns = maxConcurrentRuns;
}
public HardwareTierCapacityV1 maxNumberOfExecutors(Integer maxNumberOfExecutors) {
this.maxNumberOfExecutors = maxNumberOfExecutors;
return this;
}
/**
* Get maxNumberOfExecutors
* @return maxNumberOfExecutors
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_MAX_NUMBER_OF_EXECUTORS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Integer getMaxNumberOfExecutors() {
return maxNumberOfExecutors;
}
@JsonProperty(JSON_PROPERTY_MAX_NUMBER_OF_EXECUTORS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setMaxNumberOfExecutors(Integer maxNumberOfExecutors) {
this.maxNumberOfExecutors = maxNumberOfExecutors;
}
public HardwareTierCapacityV1 numberOfExecutors(Integer numberOfExecutors) {
this.numberOfExecutors = numberOfExecutors;
return this;
}
/**
* Get numberOfExecutors
* @return numberOfExecutors
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_NUMBER_OF_EXECUTORS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Integer getNumberOfExecutors() {
return numberOfExecutors;
}
@JsonProperty(JSON_PROPERTY_NUMBER_OF_EXECUTORS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setNumberOfExecutors(Integer numberOfExecutors) {
this.numberOfExecutors = numberOfExecutors;
}
public HardwareTierCapacityV1 queuedRuns(Integer queuedRuns) {
this.queuedRuns = queuedRuns;
return this;
}
/**
* Get queuedRuns
* @return queuedRuns
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_QUEUED_RUNS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Integer getQueuedRuns() {
return queuedRuns;
}
@JsonProperty(JSON_PROPERTY_QUEUED_RUNS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setQueuedRuns(Integer queuedRuns) {
this.queuedRuns = queuedRuns;
}
/**
* Return true if this HardwareTierCapacityV1 object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
HardwareTierCapacityV1 hardwareTierCapacityV1 = (HardwareTierCapacityV1) o;
return Objects.equals(this.availableCapacityWithoutLaunching, hardwareTierCapacityV1.availableCapacityWithoutLaunching) &&
Objects.equals(this.capacityLevel, hardwareTierCapacityV1.capacityLevel) &&
Objects.equals(this.executingRuns, hardwareTierCapacityV1.executingRuns) &&
Objects.equals(this.maxAvailableCapacity, hardwareTierCapacityV1.maxAvailableCapacity) &&
Objects.equals(this.maxConcurrentRuns, hardwareTierCapacityV1.maxConcurrentRuns) &&
Objects.equals(this.maxNumberOfExecutors, hardwareTierCapacityV1.maxNumberOfExecutors) &&
Objects.equals(this.numberOfExecutors, hardwareTierCapacityV1.numberOfExecutors) &&
Objects.equals(this.queuedRuns, hardwareTierCapacityV1.queuedRuns);
}
@Override
public int hashCode() {
return Objects.hash(availableCapacityWithoutLaunching, capacityLevel, executingRuns, maxAvailableCapacity, maxConcurrentRuns, maxNumberOfExecutors, numberOfExecutors, queuedRuns);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class HardwareTierCapacityV1 {\n");
sb.append(" availableCapacityWithoutLaunching: ").append(toIndentedString(availableCapacityWithoutLaunching)).append("\n");
sb.append(" capacityLevel: ").append(toIndentedString(capacityLevel)).append("\n");
sb.append(" executingRuns: ").append(toIndentedString(executingRuns)).append("\n");
sb.append(" maxAvailableCapacity: ").append(toIndentedString(maxAvailableCapacity)).append("\n");
sb.append(" maxConcurrentRuns: ").append(toIndentedString(maxConcurrentRuns)).append("\n");
sb.append(" maxNumberOfExecutors: ").append(toIndentedString(maxNumberOfExecutors)).append("\n");
sb.append(" numberOfExecutors: ").append(toIndentedString(numberOfExecutors)).append("\n");
sb.append(" queuedRuns: ").append(toIndentedString(queuedRuns)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `availableCapacityWithoutLaunching` to the URL query string
if (getAvailableCapacityWithoutLaunching() != null) {
joiner.add(String.format("%savailableCapacityWithoutLaunching%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getAvailableCapacityWithoutLaunching()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `capacityLevel` to the URL query string
if (getCapacityLevel() != null) {
joiner.add(String.format("%scapacityLevel%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getCapacityLevel()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `executingRuns` to the URL query string
if (getExecutingRuns() != null) {
joiner.add(String.format("%sexecutingRuns%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getExecutingRuns()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `maxAvailableCapacity` to the URL query string
if (getMaxAvailableCapacity() != null) {
joiner.add(String.format("%smaxAvailableCapacity%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getMaxAvailableCapacity()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `maxConcurrentRuns` to the URL query string
if (getMaxConcurrentRuns() != null) {
joiner.add(String.format("%smaxConcurrentRuns%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getMaxConcurrentRuns()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `maxNumberOfExecutors` to the URL query string
if (getMaxNumberOfExecutors() != null) {
joiner.add(String.format("%smaxNumberOfExecutors%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getMaxNumberOfExecutors()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `numberOfExecutors` to the URL query string
if (getNumberOfExecutors() != null) {
joiner.add(String.format("%snumberOfExecutors%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getNumberOfExecutors()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `queuedRuns` to the URL query string
if (getQueuedRuns() != null) {
joiner.add(String.format("%squeuedRuns%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getQueuedRuns()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy