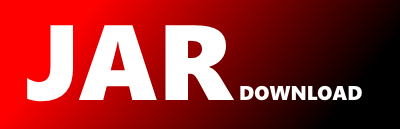
com.dominodatalab.pub.model.HardwareTierResourcesV1 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of domino-java-client Show documentation
Show all versions of domino-java-client Show documentation
Domino Data Lab API Client to connect to Domino web services using Java HTTP Client.
/*
* Domino Public API
* Domino Public API Endpoints
*
* The version of the OpenAPI document: 0.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.pub.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.dominodatalab.pub.model.InformationV1;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.dominodatalab.pub.invoker.ApiClient;
/**
* Compute resources for a hardware tier
*/
@JsonPropertyOrder({
HardwareTierResourcesV1.JSON_PROPERTY_ALLOW_SHARED_MEMORY_TO_EXCEED_DEFAULT,
HardwareTierResourcesV1.JSON_PROPERTY_CORES,
HardwareTierResourcesV1.JSON_PROPERTY_CORES_LIMIT,
HardwareTierResourcesV1.JSON_PROPERTY_MEMORY,
HardwareTierResourcesV1.JSON_PROPERTY_MEMORY_LIMIT,
HardwareTierResourcesV1.JSON_PROPERTY_MEMORY_SWAP_LIMIT,
HardwareTierResourcesV1.JSON_PROPERTY_SHARED_MEMORY_LIMIT
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-10-04T16:37:28.765500600-04:00[America/New_York]", comments = "Generator version: 7.8.0")
public class HardwareTierResourcesV1 {
public static final String JSON_PROPERTY_ALLOW_SHARED_MEMORY_TO_EXCEED_DEFAULT = "allowSharedMemoryToExceedDefault";
private Boolean allowSharedMemoryToExceedDefault;
public static final String JSON_PROPERTY_CORES = "cores";
private Double cores;
public static final String JSON_PROPERTY_CORES_LIMIT = "coresLimit";
private Double coresLimit;
public static final String JSON_PROPERTY_MEMORY = "memory";
private InformationV1 memory;
public static final String JSON_PROPERTY_MEMORY_LIMIT = "memoryLimit";
private InformationV1 memoryLimit;
public static final String JSON_PROPERTY_MEMORY_SWAP_LIMIT = "memorySwapLimit";
private InformationV1 memorySwapLimit;
public static final String JSON_PROPERTY_SHARED_MEMORY_LIMIT = "sharedMemoryLimit";
private InformationV1 sharedMemoryLimit;
public HardwareTierResourcesV1() {
}
public HardwareTierResourcesV1 allowSharedMemoryToExceedDefault(Boolean allowSharedMemoryToExceedDefault) {
this.allowSharedMemoryToExceedDefault = allowSharedMemoryToExceedDefault;
return this;
}
/**
* Get allowSharedMemoryToExceedDefault
* @return allowSharedMemoryToExceedDefault
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ALLOW_SHARED_MEMORY_TO_EXCEED_DEFAULT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getAllowSharedMemoryToExceedDefault() {
return allowSharedMemoryToExceedDefault;
}
@JsonProperty(JSON_PROPERTY_ALLOW_SHARED_MEMORY_TO_EXCEED_DEFAULT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setAllowSharedMemoryToExceedDefault(Boolean allowSharedMemoryToExceedDefault) {
this.allowSharedMemoryToExceedDefault = allowSharedMemoryToExceedDefault;
}
public HardwareTierResourcesV1 cores(Double cores) {
this.cores = cores;
return this;
}
/**
* Get cores
* @return cores
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_CORES)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Double getCores() {
return cores;
}
@JsonProperty(JSON_PROPERTY_CORES)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setCores(Double cores) {
this.cores = cores;
}
public HardwareTierResourcesV1 coresLimit(Double coresLimit) {
this.coresLimit = coresLimit;
return this;
}
/**
* Get coresLimit
* @return coresLimit
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_CORES_LIMIT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getCoresLimit() {
return coresLimit;
}
@JsonProperty(JSON_PROPERTY_CORES_LIMIT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCoresLimit(Double coresLimit) {
this.coresLimit = coresLimit;
}
public HardwareTierResourcesV1 memory(InformationV1 memory) {
this.memory = memory;
return this;
}
/**
* Get memory
* @return memory
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_MEMORY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public InformationV1 getMemory() {
return memory;
}
@JsonProperty(JSON_PROPERTY_MEMORY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setMemory(InformationV1 memory) {
this.memory = memory;
}
public HardwareTierResourcesV1 memoryLimit(InformationV1 memoryLimit) {
this.memoryLimit = memoryLimit;
return this;
}
/**
* Get memoryLimit
* @return memoryLimit
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_MEMORY_LIMIT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public InformationV1 getMemoryLimit() {
return memoryLimit;
}
@JsonProperty(JSON_PROPERTY_MEMORY_LIMIT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMemoryLimit(InformationV1 memoryLimit) {
this.memoryLimit = memoryLimit;
}
public HardwareTierResourcesV1 memorySwapLimit(InformationV1 memorySwapLimit) {
this.memorySwapLimit = memorySwapLimit;
return this;
}
/**
* Get memorySwapLimit
* @return memorySwapLimit
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_MEMORY_SWAP_LIMIT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public InformationV1 getMemorySwapLimit() {
return memorySwapLimit;
}
@JsonProperty(JSON_PROPERTY_MEMORY_SWAP_LIMIT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMemorySwapLimit(InformationV1 memorySwapLimit) {
this.memorySwapLimit = memorySwapLimit;
}
public HardwareTierResourcesV1 sharedMemoryLimit(InformationV1 sharedMemoryLimit) {
this.sharedMemoryLimit = sharedMemoryLimit;
return this;
}
/**
* Get sharedMemoryLimit
* @return sharedMemoryLimit
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_SHARED_MEMORY_LIMIT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public InformationV1 getSharedMemoryLimit() {
return sharedMemoryLimit;
}
@JsonProperty(JSON_PROPERTY_SHARED_MEMORY_LIMIT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSharedMemoryLimit(InformationV1 sharedMemoryLimit) {
this.sharedMemoryLimit = sharedMemoryLimit;
}
/**
* Return true if this HardwareTierResourcesV1 object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
HardwareTierResourcesV1 hardwareTierResourcesV1 = (HardwareTierResourcesV1) o;
return Objects.equals(this.allowSharedMemoryToExceedDefault, hardwareTierResourcesV1.allowSharedMemoryToExceedDefault) &&
Objects.equals(this.cores, hardwareTierResourcesV1.cores) &&
Objects.equals(this.coresLimit, hardwareTierResourcesV1.coresLimit) &&
Objects.equals(this.memory, hardwareTierResourcesV1.memory) &&
Objects.equals(this.memoryLimit, hardwareTierResourcesV1.memoryLimit) &&
Objects.equals(this.memorySwapLimit, hardwareTierResourcesV1.memorySwapLimit) &&
Objects.equals(this.sharedMemoryLimit, hardwareTierResourcesV1.sharedMemoryLimit);
}
@Override
public int hashCode() {
return Objects.hash(allowSharedMemoryToExceedDefault, cores, coresLimit, memory, memoryLimit, memorySwapLimit, sharedMemoryLimit);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class HardwareTierResourcesV1 {\n");
sb.append(" allowSharedMemoryToExceedDefault: ").append(toIndentedString(allowSharedMemoryToExceedDefault)).append("\n");
sb.append(" cores: ").append(toIndentedString(cores)).append("\n");
sb.append(" coresLimit: ").append(toIndentedString(coresLimit)).append("\n");
sb.append(" memory: ").append(toIndentedString(memory)).append("\n");
sb.append(" memoryLimit: ").append(toIndentedString(memoryLimit)).append("\n");
sb.append(" memorySwapLimit: ").append(toIndentedString(memorySwapLimit)).append("\n");
sb.append(" sharedMemoryLimit: ").append(toIndentedString(sharedMemoryLimit)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `allowSharedMemoryToExceedDefault` to the URL query string
if (getAllowSharedMemoryToExceedDefault() != null) {
joiner.add(String.format("%sallowSharedMemoryToExceedDefault%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getAllowSharedMemoryToExceedDefault()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `cores` to the URL query string
if (getCores() != null) {
joiner.add(String.format("%scores%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getCores()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `coresLimit` to the URL query string
if (getCoresLimit() != null) {
joiner.add(String.format("%scoresLimit%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getCoresLimit()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `memory` to the URL query string
if (getMemory() != null) {
joiner.add(getMemory().toUrlQueryString(prefix + "memory" + suffix));
}
// add `memoryLimit` to the URL query string
if (getMemoryLimit() != null) {
joiner.add(getMemoryLimit().toUrlQueryString(prefix + "memoryLimit" + suffix));
}
// add `memorySwapLimit` to the URL query string
if (getMemorySwapLimit() != null) {
joiner.add(getMemorySwapLimit().toUrlQueryString(prefix + "memorySwapLimit" + suffix));
}
// add `sharedMemoryLimit` to the URL query string
if (getSharedMemoryLimit() != null) {
joiner.add(getSharedMemoryLimit().toUrlQueryString(prefix + "sharedMemoryLimit" + suffix));
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy