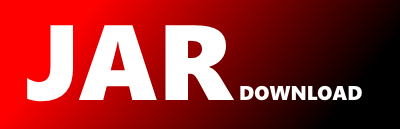
com.dominodatalab.pub.model.HardwareTierV1 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of domino-java-client Show documentation
Show all versions of domino-java-client Show documentation
Domino Data Lab API Client to connect to Domino web services using Java HTTP Client.
/*
* Domino Public API
* Domino Public API Endpoints
*
* The version of the OpenAPI document: 0.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.pub.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.dominodatalab.pub.model.HardwareTierCapacityV1;
import com.dominodatalab.pub.model.HardwareTierComputeClusterRestrictionsV1;
import com.dominodatalab.pub.model.HardwareTierFlagsV1;
import com.dominodatalab.pub.model.HardwareTierGpuConfigurationV1;
import com.dominodatalab.pub.model.HardwareTierOverProvisioningV1;
import com.dominodatalab.pub.model.HardwareTierPodCustomizationV1;
import com.dominodatalab.pub.model.HardwareTierResourcesV1;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.dominodatalab.pub.invoker.ApiClient;
/**
* HardwareTierV1
*/
@JsonPropertyOrder({
HardwareTierV1.JSON_PROPERTY_AVAILABILITY_ZONES,
HardwareTierV1.JSON_PROPERTY_CAPACITY,
HardwareTierV1.JSON_PROPERTY_CENTS_PER_MINUTE,
HardwareTierV1.JSON_PROPERTY_COMPUTE_CLUSTER_RESTRICTIONS,
HardwareTierV1.JSON_PROPERTY_CREATION_TIME,
HardwareTierV1.JSON_PROPERTY_DATA_PLANE_ID,
HardwareTierV1.JSON_PROPERTY_FLAGS,
HardwareTierV1.JSON_PROPERTY_GPU_CONFIGURATION,
HardwareTierV1.JSON_PROPERTY_ID,
HardwareTierV1.JSON_PROPERTY_MAX_SIMULTANEOUS_EXECUTIONS,
HardwareTierV1.JSON_PROPERTY_METADATA,
HardwareTierV1.JSON_PROPERTY_NAME,
HardwareTierV1.JSON_PROPERTY_NODE_POOL,
HardwareTierV1.JSON_PROPERTY_OVER_PROVISIONING,
HardwareTierV1.JSON_PROPERTY_POD_CUSTOMIZATION,
HardwareTierV1.JSON_PROPERTY_RESOURCES,
HardwareTierV1.JSON_PROPERTY_TAGS,
HardwareTierV1.JSON_PROPERTY_UPDATE_TIME
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-10-04T16:37:28.765500600-04:00[America/New_York]", comments = "Generator version: 7.8.0")
public class HardwareTierV1 {
public static final String JSON_PROPERTY_AVAILABILITY_ZONES = "availabilityZones";
private List availabilityZones = new ArrayList<>();
public static final String JSON_PROPERTY_CAPACITY = "capacity";
private HardwareTierCapacityV1 capacity;
public static final String JSON_PROPERTY_CENTS_PER_MINUTE = "centsPerMinute";
private Double centsPerMinute;
public static final String JSON_PROPERTY_COMPUTE_CLUSTER_RESTRICTIONS = "computeClusterRestrictions";
private HardwareTierComputeClusterRestrictionsV1 computeClusterRestrictions;
public static final String JSON_PROPERTY_CREATION_TIME = "creationTime";
private OffsetDateTime creationTime;
public static final String JSON_PROPERTY_DATA_PLANE_ID = "dataPlaneId";
private String dataPlaneId;
public static final String JSON_PROPERTY_FLAGS = "flags";
private HardwareTierFlagsV1 flags;
public static final String JSON_PROPERTY_GPU_CONFIGURATION = "gpuConfiguration";
private HardwareTierGpuConfigurationV1 gpuConfiguration;
public static final String JSON_PROPERTY_ID = "id";
private String id;
public static final String JSON_PROPERTY_MAX_SIMULTANEOUS_EXECUTIONS = "maxSimultaneousExecutions";
private Integer maxSimultaneousExecutions;
public static final String JSON_PROPERTY_METADATA = "metadata";
private Map metadata = new HashMap<>();
public static final String JSON_PROPERTY_NAME = "name";
private String name;
public static final String JSON_PROPERTY_NODE_POOL = "nodePool";
private String nodePool;
public static final String JSON_PROPERTY_OVER_PROVISIONING = "overProvisioning";
private HardwareTierOverProvisioningV1 overProvisioning;
public static final String JSON_PROPERTY_POD_CUSTOMIZATION = "podCustomization";
private HardwareTierPodCustomizationV1 podCustomization;
public static final String JSON_PROPERTY_RESOURCES = "resources";
private HardwareTierResourcesV1 resources;
public static final String JSON_PROPERTY_TAGS = "tags";
private List tags = new ArrayList<>();
public static final String JSON_PROPERTY_UPDATE_TIME = "updateTime";
private OffsetDateTime updateTime;
public HardwareTierV1() {
}
public HardwareTierV1 availabilityZones(List availabilityZones) {
this.availabilityZones = availabilityZones;
return this;
}
public HardwareTierV1 addAvailabilityZonesItem(String availabilityZonesItem) {
if (this.availabilityZones == null) {
this.availabilityZones = new ArrayList<>();
}
this.availabilityZones.add(availabilityZonesItem);
return this;
}
/**
* Get availabilityZones
* @return availabilityZones
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_AVAILABILITY_ZONES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getAvailabilityZones() {
return availabilityZones;
}
@JsonProperty(JSON_PROPERTY_AVAILABILITY_ZONES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAvailabilityZones(List availabilityZones) {
this.availabilityZones = availabilityZones;
}
public HardwareTierV1 capacity(HardwareTierCapacityV1 capacity) {
this.capacity = capacity;
return this;
}
/**
* Get capacity
* @return capacity
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_CAPACITY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HardwareTierCapacityV1 getCapacity() {
return capacity;
}
@JsonProperty(JSON_PROPERTY_CAPACITY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCapacity(HardwareTierCapacityV1 capacity) {
this.capacity = capacity;
}
public HardwareTierV1 centsPerMinute(Double centsPerMinute) {
this.centsPerMinute = centsPerMinute;
return this;
}
/**
* Cost per minute of using this hardware tier as defined by an Admin.
* @return centsPerMinute
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_CENTS_PER_MINUTE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Double getCentsPerMinute() {
return centsPerMinute;
}
@JsonProperty(JSON_PROPERTY_CENTS_PER_MINUTE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setCentsPerMinute(Double centsPerMinute) {
this.centsPerMinute = centsPerMinute;
}
public HardwareTierV1 computeClusterRestrictions(HardwareTierComputeClusterRestrictionsV1 computeClusterRestrictions) {
this.computeClusterRestrictions = computeClusterRestrictions;
return this;
}
/**
* Get computeClusterRestrictions
* @return computeClusterRestrictions
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_COMPUTE_CLUSTER_RESTRICTIONS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HardwareTierComputeClusterRestrictionsV1 getComputeClusterRestrictions() {
return computeClusterRestrictions;
}
@JsonProperty(JSON_PROPERTY_COMPUTE_CLUSTER_RESTRICTIONS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setComputeClusterRestrictions(HardwareTierComputeClusterRestrictionsV1 computeClusterRestrictions) {
this.computeClusterRestrictions = computeClusterRestrictions;
}
public HardwareTierV1 creationTime(OffsetDateTime creationTime) {
this.creationTime = creationTime;
return this;
}
/**
* When the hardware tier was created
* @return creationTime
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_CREATION_TIME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public OffsetDateTime getCreationTime() {
return creationTime;
}
@JsonProperty(JSON_PROPERTY_CREATION_TIME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setCreationTime(OffsetDateTime creationTime) {
this.creationTime = creationTime;
}
public HardwareTierV1 dataPlaneId(String dataPlaneId) {
this.dataPlaneId = dataPlaneId;
return this;
}
/**
* Get dataPlaneId
* @return dataPlaneId
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DATA_PLANE_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDataPlaneId() {
return dataPlaneId;
}
@JsonProperty(JSON_PROPERTY_DATA_PLANE_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDataPlaneId(String dataPlaneId) {
this.dataPlaneId = dataPlaneId;
}
public HardwareTierV1 flags(HardwareTierFlagsV1 flags) {
this.flags = flags;
return this;
}
/**
* Get flags
* @return flags
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_FLAGS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public HardwareTierFlagsV1 getFlags() {
return flags;
}
@JsonProperty(JSON_PROPERTY_FLAGS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setFlags(HardwareTierFlagsV1 flags) {
this.flags = flags;
}
public HardwareTierV1 gpuConfiguration(HardwareTierGpuConfigurationV1 gpuConfiguration) {
this.gpuConfiguration = gpuConfiguration;
return this;
}
/**
* Get gpuConfiguration
* @return gpuConfiguration
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_GPU_CONFIGURATION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HardwareTierGpuConfigurationV1 getGpuConfiguration() {
return gpuConfiguration;
}
@JsonProperty(JSON_PROPERTY_GPU_CONFIGURATION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setGpuConfiguration(HardwareTierGpuConfigurationV1 gpuConfiguration) {
this.gpuConfiguration = gpuConfiguration;
}
public HardwareTierV1 id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getId() {
return id;
}
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setId(String id) {
this.id = id;
}
public HardwareTierV1 maxSimultaneousExecutions(Integer maxSimultaneousExecutions) {
this.maxSimultaneousExecutions = maxSimultaneousExecutions;
return this;
}
/**
* Get maxSimultaneousExecutions
* @return maxSimultaneousExecutions
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_MAX_SIMULTANEOUS_EXECUTIONS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getMaxSimultaneousExecutions() {
return maxSimultaneousExecutions;
}
@JsonProperty(JSON_PROPERTY_MAX_SIMULTANEOUS_EXECUTIONS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMaxSimultaneousExecutions(Integer maxSimultaneousExecutions) {
this.maxSimultaneousExecutions = maxSimultaneousExecutions;
}
public HardwareTierV1 metadata(Map metadata) {
this.metadata = metadata;
return this;
}
public HardwareTierV1 putMetadataItem(String key, String metadataItem) {
if (this.metadata == null) {
this.metadata = new HashMap<>();
}
this.metadata.put(key, metadataItem);
return this;
}
/**
* Get metadata
* @return metadata
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_METADATA)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Map getMetadata() {
return metadata;
}
@JsonProperty(JSON_PROPERTY_METADATA)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setMetadata(Map metadata) {
this.metadata = metadata;
}
public HardwareTierV1 name(String name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getName() {
return name;
}
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setName(String name) {
this.name = name;
}
public HardwareTierV1 nodePool(String nodePool) {
this.nodePool = nodePool;
return this;
}
/**
* Get nodePool
* @return nodePool
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_NODE_POOL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getNodePool() {
return nodePool;
}
@JsonProperty(JSON_PROPERTY_NODE_POOL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setNodePool(String nodePool) {
this.nodePool = nodePool;
}
public HardwareTierV1 overProvisioning(HardwareTierOverProvisioningV1 overProvisioning) {
this.overProvisioning = overProvisioning;
return this;
}
/**
* Get overProvisioning
* @return overProvisioning
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_OVER_PROVISIONING)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HardwareTierOverProvisioningV1 getOverProvisioning() {
return overProvisioning;
}
@JsonProperty(JSON_PROPERTY_OVER_PROVISIONING)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setOverProvisioning(HardwareTierOverProvisioningV1 overProvisioning) {
this.overProvisioning = overProvisioning;
}
public HardwareTierV1 podCustomization(HardwareTierPodCustomizationV1 podCustomization) {
this.podCustomization = podCustomization;
return this;
}
/**
* Get podCustomization
* @return podCustomization
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_POD_CUSTOMIZATION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public HardwareTierPodCustomizationV1 getPodCustomization() {
return podCustomization;
}
@JsonProperty(JSON_PROPERTY_POD_CUSTOMIZATION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setPodCustomization(HardwareTierPodCustomizationV1 podCustomization) {
this.podCustomization = podCustomization;
}
public HardwareTierV1 resources(HardwareTierResourcesV1 resources) {
this.resources = resources;
return this;
}
/**
* Get resources
* @return resources
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_RESOURCES)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public HardwareTierResourcesV1 getResources() {
return resources;
}
@JsonProperty(JSON_PROPERTY_RESOURCES)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setResources(HardwareTierResourcesV1 resources) {
this.resources = resources;
}
public HardwareTierV1 tags(List tags) {
this.tags = tags;
return this;
}
public HardwareTierV1 addTagsItem(String tagsItem) {
if (this.tags == null) {
this.tags = new ArrayList<>();
}
this.tags.add(tagsItem);
return this;
}
/**
* Get tags
* @return tags
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_TAGS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getTags() {
return tags;
}
@JsonProperty(JSON_PROPERTY_TAGS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTags(List tags) {
this.tags = tags;
}
public HardwareTierV1 updateTime(OffsetDateTime updateTime) {
this.updateTime = updateTime;
return this;
}
/**
* When the hardwareTier was last updated
* @return updateTime
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_UPDATE_TIME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public OffsetDateTime getUpdateTime() {
return updateTime;
}
@JsonProperty(JSON_PROPERTY_UPDATE_TIME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setUpdateTime(OffsetDateTime updateTime) {
this.updateTime = updateTime;
}
/**
* Return true if this HardwareTierV1 object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
HardwareTierV1 hardwareTierV1 = (HardwareTierV1) o;
return Objects.equals(this.availabilityZones, hardwareTierV1.availabilityZones) &&
Objects.equals(this.capacity, hardwareTierV1.capacity) &&
Objects.equals(this.centsPerMinute, hardwareTierV1.centsPerMinute) &&
Objects.equals(this.computeClusterRestrictions, hardwareTierV1.computeClusterRestrictions) &&
Objects.equals(this.creationTime, hardwareTierV1.creationTime) &&
Objects.equals(this.dataPlaneId, hardwareTierV1.dataPlaneId) &&
Objects.equals(this.flags, hardwareTierV1.flags) &&
Objects.equals(this.gpuConfiguration, hardwareTierV1.gpuConfiguration) &&
Objects.equals(this.id, hardwareTierV1.id) &&
Objects.equals(this.maxSimultaneousExecutions, hardwareTierV1.maxSimultaneousExecutions) &&
Objects.equals(this.metadata, hardwareTierV1.metadata) &&
Objects.equals(this.name, hardwareTierV1.name) &&
Objects.equals(this.nodePool, hardwareTierV1.nodePool) &&
Objects.equals(this.overProvisioning, hardwareTierV1.overProvisioning) &&
Objects.equals(this.podCustomization, hardwareTierV1.podCustomization) &&
Objects.equals(this.resources, hardwareTierV1.resources) &&
Objects.equals(this.tags, hardwareTierV1.tags) &&
Objects.equals(this.updateTime, hardwareTierV1.updateTime);
}
@Override
public int hashCode() {
return Objects.hash(availabilityZones, capacity, centsPerMinute, computeClusterRestrictions, creationTime, dataPlaneId, flags, gpuConfiguration, id, maxSimultaneousExecutions, metadata, name, nodePool, overProvisioning, podCustomization, resources, tags, updateTime);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class HardwareTierV1 {\n");
sb.append(" availabilityZones: ").append(toIndentedString(availabilityZones)).append("\n");
sb.append(" capacity: ").append(toIndentedString(capacity)).append("\n");
sb.append(" centsPerMinute: ").append(toIndentedString(centsPerMinute)).append("\n");
sb.append(" computeClusterRestrictions: ").append(toIndentedString(computeClusterRestrictions)).append("\n");
sb.append(" creationTime: ").append(toIndentedString(creationTime)).append("\n");
sb.append(" dataPlaneId: ").append(toIndentedString(dataPlaneId)).append("\n");
sb.append(" flags: ").append(toIndentedString(flags)).append("\n");
sb.append(" gpuConfiguration: ").append(toIndentedString(gpuConfiguration)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" maxSimultaneousExecutions: ").append(toIndentedString(maxSimultaneousExecutions)).append("\n");
sb.append(" metadata: ").append(toIndentedString(metadata)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" nodePool: ").append(toIndentedString(nodePool)).append("\n");
sb.append(" overProvisioning: ").append(toIndentedString(overProvisioning)).append("\n");
sb.append(" podCustomization: ").append(toIndentedString(podCustomization)).append("\n");
sb.append(" resources: ").append(toIndentedString(resources)).append("\n");
sb.append(" tags: ").append(toIndentedString(tags)).append("\n");
sb.append(" updateTime: ").append(toIndentedString(updateTime)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `availabilityZones` to the URL query string
if (getAvailabilityZones() != null) {
for (int i = 0; i < getAvailabilityZones().size(); i++) {
joiner.add(String.format("%savailabilityZones%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(ApiClient.valueToString(getAvailabilityZones().get(i)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
// add `capacity` to the URL query string
if (getCapacity() != null) {
joiner.add(getCapacity().toUrlQueryString(prefix + "capacity" + suffix));
}
// add `centsPerMinute` to the URL query string
if (getCentsPerMinute() != null) {
joiner.add(String.format("%scentsPerMinute%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getCentsPerMinute()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `computeClusterRestrictions` to the URL query string
if (getComputeClusterRestrictions() != null) {
joiner.add(getComputeClusterRestrictions().toUrlQueryString(prefix + "computeClusterRestrictions" + suffix));
}
// add `creationTime` to the URL query string
if (getCreationTime() != null) {
joiner.add(String.format("%screationTime%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getCreationTime()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `dataPlaneId` to the URL query string
if (getDataPlaneId() != null) {
joiner.add(String.format("%sdataPlaneId%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getDataPlaneId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `flags` to the URL query string
if (getFlags() != null) {
joiner.add(getFlags().toUrlQueryString(prefix + "flags" + suffix));
}
// add `gpuConfiguration` to the URL query string
if (getGpuConfiguration() != null) {
joiner.add(getGpuConfiguration().toUrlQueryString(prefix + "gpuConfiguration" + suffix));
}
// add `id` to the URL query string
if (getId() != null) {
joiner.add(String.format("%sid%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `maxSimultaneousExecutions` to the URL query string
if (getMaxSimultaneousExecutions() != null) {
joiner.add(String.format("%smaxSimultaneousExecutions%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getMaxSimultaneousExecutions()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `metadata` to the URL query string
if (getMetadata() != null) {
for (String _key : getMetadata().keySet()) {
joiner.add(String.format("%smetadata%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, _key, containerSuffix),
getMetadata().get(_key), URLEncoder.encode(ApiClient.valueToString(getMetadata().get(_key)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
// add `name` to the URL query string
if (getName() != null) {
joiner.add(String.format("%sname%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getName()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `nodePool` to the URL query string
if (getNodePool() != null) {
joiner.add(String.format("%snodePool%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getNodePool()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `overProvisioning` to the URL query string
if (getOverProvisioning() != null) {
joiner.add(getOverProvisioning().toUrlQueryString(prefix + "overProvisioning" + suffix));
}
// add `podCustomization` to the URL query string
if (getPodCustomization() != null) {
joiner.add(getPodCustomization().toUrlQueryString(prefix + "podCustomization" + suffix));
}
// add `resources` to the URL query string
if (getResources() != null) {
joiner.add(getResources().toUrlQueryString(prefix + "resources" + suffix));
}
// add `tags` to the URL query string
if (getTags() != null) {
for (int i = 0; i < getTags().size(); i++) {
joiner.add(String.format("%stags%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(ApiClient.valueToString(getTags().get(i)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
// add `updateTime` to the URL query string
if (getUpdateTime() != null) {
joiner.add(String.format("%supdateTime%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getUpdateTime()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy