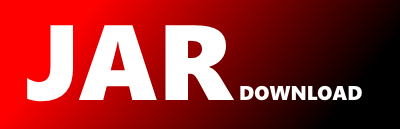
com.dominodatalab.pub.model.JobV1 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of domino-java-client Show documentation
Show all versions of domino-java-client Show documentation
Domino Data Lab API Client to connect to Domino web services using Java HTTP Client.
/*
* Domino Public API
* Domino Public API Endpoints
*
* The version of the OpenAPI document: 0.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.pub.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.dominodatalab.pub.model.CommitDetailsV1;
import com.dominodatalab.pub.model.ComputeClusterConfigV1;
import com.dominodatalab.pub.model.DatasetMountV1;
import com.dominodatalab.pub.model.DominoStatsV1;
import com.dominodatalab.pub.model.ExternalVolumeMountV1;
import com.dominodatalab.pub.model.GitRefV1;
import com.dominodatalab.pub.model.JobStatusV1;
import com.dominodatalab.pub.model.JobUsageV1;
import com.dominodatalab.pub.model.MountedGitRepoV1;
import com.dominodatalab.pub.model.MountedProjectV1;
import com.dominodatalab.pub.model.StageTimesV1;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.dominodatalab.pub.invoker.ApiClient;
/**
* JobV1
*/
@JsonPropertyOrder({
JobV1.JSON_PROPERTY_COMMIT_DETAILS,
JobV1.JSON_PROPERTY_COMPUTE_CLUSTER,
JobV1.JSON_PROPERTY_DATASET_MOUNTS,
JobV1.JSON_PROPERTY_DOMINO_STATS,
JobV1.JSON_PROPERTY_EXTERNAL_VOLUME_MOUNTS,
JobV1.JSON_PROPERTY_GIT_REPOS,
JobV1.JSON_PROPERTY_ID,
JobV1.JSON_PROPERTY_MAIN_REPO_GIT_REF,
JobV1.JSON_PROPERTY_NUMBER,
JobV1.JSON_PROPERTY_PROJECTS,
JobV1.JSON_PROPERTY_RUN_COMMAND,
JobV1.JSON_PROPERTY_RUN_LAUNCHER_ID,
JobV1.JSON_PROPERTY_STAGE_TIMES,
JobV1.JSON_PROPERTY_STARTED_BY_ID,
JobV1.JSON_PROPERTY_STATUS,
JobV1.JSON_PROPERTY_TITLE,
JobV1.JSON_PROPERTY_USAGE
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-10-04T16:37:28.765500600-04:00[America/New_York]", comments = "Generator version: 7.8.0")
public class JobV1 {
public static final String JSON_PROPERTY_COMMIT_DETAILS = "commitDetails";
private CommitDetailsV1 commitDetails;
public static final String JSON_PROPERTY_COMPUTE_CLUSTER = "computeCluster";
private ComputeClusterConfigV1 computeCluster;
public static final String JSON_PROPERTY_DATASET_MOUNTS = "datasetMounts";
private List datasetMounts = new ArrayList<>();
public static final String JSON_PROPERTY_DOMINO_STATS = "dominoStats";
private List dominoStats = new ArrayList<>();
public static final String JSON_PROPERTY_EXTERNAL_VOLUME_MOUNTS = "externalVolumeMounts";
private List externalVolumeMounts = new ArrayList<>();
public static final String JSON_PROPERTY_GIT_REPOS = "gitRepos";
private List gitRepos = new ArrayList<>();
public static final String JSON_PROPERTY_ID = "id";
private String id;
public static final String JSON_PROPERTY_MAIN_REPO_GIT_REF = "mainRepoGitRef";
private GitRefV1 mainRepoGitRef;
public static final String JSON_PROPERTY_NUMBER = "number";
private Integer number;
public static final String JSON_PROPERTY_PROJECTS = "projects";
private List projects = new ArrayList<>();
public static final String JSON_PROPERTY_RUN_COMMAND = "runCommand";
private String runCommand;
public static final String JSON_PROPERTY_RUN_LAUNCHER_ID = "runLauncherId";
private String runLauncherId;
public static final String JSON_PROPERTY_STAGE_TIMES = "stageTimes";
private StageTimesV1 stageTimes;
public static final String JSON_PROPERTY_STARTED_BY_ID = "startedById";
private String startedById;
public static final String JSON_PROPERTY_STATUS = "status";
private JobStatusV1 status;
public static final String JSON_PROPERTY_TITLE = "title";
private String title;
public static final String JSON_PROPERTY_USAGE = "usage";
private JobUsageV1 usage;
public JobV1() {
}
public JobV1 commitDetails(CommitDetailsV1 commitDetails) {
this.commitDetails = commitDetails;
return this;
}
/**
* Get commitDetails
* @return commitDetails
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_COMMIT_DETAILS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public CommitDetailsV1 getCommitDetails() {
return commitDetails;
}
@JsonProperty(JSON_PROPERTY_COMMIT_DETAILS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setCommitDetails(CommitDetailsV1 commitDetails) {
this.commitDetails = commitDetails;
}
public JobV1 computeCluster(ComputeClusterConfigV1 computeCluster) {
this.computeCluster = computeCluster;
return this;
}
/**
* Get computeCluster
* @return computeCluster
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_COMPUTE_CLUSTER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ComputeClusterConfigV1 getComputeCluster() {
return computeCluster;
}
@JsonProperty(JSON_PROPERTY_COMPUTE_CLUSTER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setComputeCluster(ComputeClusterConfigV1 computeCluster) {
this.computeCluster = computeCluster;
}
public JobV1 datasetMounts(List datasetMounts) {
this.datasetMounts = datasetMounts;
return this;
}
public JobV1 addDatasetMountsItem(DatasetMountV1 datasetMountsItem) {
if (this.datasetMounts == null) {
this.datasetMounts = new ArrayList<>();
}
this.datasetMounts.add(datasetMountsItem);
return this;
}
/**
* Get datasetMounts
* @return datasetMounts
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_DATASET_MOUNTS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getDatasetMounts() {
return datasetMounts;
}
@JsonProperty(JSON_PROPERTY_DATASET_MOUNTS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDatasetMounts(List datasetMounts) {
this.datasetMounts = datasetMounts;
}
public JobV1 dominoStats(List dominoStats) {
this.dominoStats = dominoStats;
return this;
}
public JobV1 addDominoStatsItem(DominoStatsV1 dominoStatsItem) {
if (this.dominoStats == null) {
this.dominoStats = new ArrayList<>();
}
this.dominoStats.add(dominoStatsItem);
return this;
}
/**
* Get dominoStats
* @return dominoStats
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_DOMINO_STATS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getDominoStats() {
return dominoStats;
}
@JsonProperty(JSON_PROPERTY_DOMINO_STATS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDominoStats(List dominoStats) {
this.dominoStats = dominoStats;
}
public JobV1 externalVolumeMounts(List externalVolumeMounts) {
this.externalVolumeMounts = externalVolumeMounts;
return this;
}
public JobV1 addExternalVolumeMountsItem(ExternalVolumeMountV1 externalVolumeMountsItem) {
if (this.externalVolumeMounts == null) {
this.externalVolumeMounts = new ArrayList<>();
}
this.externalVolumeMounts.add(externalVolumeMountsItem);
return this;
}
/**
* Get externalVolumeMounts
* @return externalVolumeMounts
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_EXTERNAL_VOLUME_MOUNTS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getExternalVolumeMounts() {
return externalVolumeMounts;
}
@JsonProperty(JSON_PROPERTY_EXTERNAL_VOLUME_MOUNTS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setExternalVolumeMounts(List externalVolumeMounts) {
this.externalVolumeMounts = externalVolumeMounts;
}
public JobV1 gitRepos(List gitRepos) {
this.gitRepos = gitRepos;
return this;
}
public JobV1 addGitReposItem(MountedGitRepoV1 gitReposItem) {
if (this.gitRepos == null) {
this.gitRepos = new ArrayList<>();
}
this.gitRepos.add(gitReposItem);
return this;
}
/**
* Get gitRepos
* @return gitRepos
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_GIT_REPOS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getGitRepos() {
return gitRepos;
}
@JsonProperty(JSON_PROPERTY_GIT_REPOS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setGitRepos(List gitRepos) {
this.gitRepos = gitRepos;
}
public JobV1 id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getId() {
return id;
}
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setId(String id) {
this.id = id;
}
public JobV1 mainRepoGitRef(GitRefV1 mainRepoGitRef) {
this.mainRepoGitRef = mainRepoGitRef;
return this;
}
/**
* Get mainRepoGitRef
* @return mainRepoGitRef
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_MAIN_REPO_GIT_REF)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public GitRefV1 getMainRepoGitRef() {
return mainRepoGitRef;
}
@JsonProperty(JSON_PROPERTY_MAIN_REPO_GIT_REF)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMainRepoGitRef(GitRefV1 mainRepoGitRef) {
this.mainRepoGitRef = mainRepoGitRef;
}
public JobV1 number(Integer number) {
this.number = number;
return this;
}
/**
* Get number
* @return number
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_NUMBER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Integer getNumber() {
return number;
}
@JsonProperty(JSON_PROPERTY_NUMBER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setNumber(Integer number) {
this.number = number;
}
public JobV1 projects(List projects) {
this.projects = projects;
return this;
}
public JobV1 addProjectsItem(MountedProjectV1 projectsItem) {
if (this.projects == null) {
this.projects = new ArrayList<>();
}
this.projects.add(projectsItem);
return this;
}
/**
* Get projects
* @return projects
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_PROJECTS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getProjects() {
return projects;
}
@JsonProperty(JSON_PROPERTY_PROJECTS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setProjects(List projects) {
this.projects = projects;
}
public JobV1 runCommand(String runCommand) {
this.runCommand = runCommand;
return this;
}
/**
* Get runCommand
* @return runCommand
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_RUN_COMMAND)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getRunCommand() {
return runCommand;
}
@JsonProperty(JSON_PROPERTY_RUN_COMMAND)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setRunCommand(String runCommand) {
this.runCommand = runCommand;
}
public JobV1 runLauncherId(String runLauncherId) {
this.runLauncherId = runLauncherId;
return this;
}
/**
* Get runLauncherId
* @return runLauncherId
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_RUN_LAUNCHER_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getRunLauncherId() {
return runLauncherId;
}
@JsonProperty(JSON_PROPERTY_RUN_LAUNCHER_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRunLauncherId(String runLauncherId) {
this.runLauncherId = runLauncherId;
}
public JobV1 stageTimes(StageTimesV1 stageTimes) {
this.stageTimes = stageTimes;
return this;
}
/**
* Get stageTimes
* @return stageTimes
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_STAGE_TIMES)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public StageTimesV1 getStageTimes() {
return stageTimes;
}
@JsonProperty(JSON_PROPERTY_STAGE_TIMES)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setStageTimes(StageTimesV1 stageTimes) {
this.stageTimes = stageTimes;
}
public JobV1 startedById(String startedById) {
this.startedById = startedById;
return this;
}
/**
* Get startedById
* @return startedById
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_STARTED_BY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getStartedById() {
return startedById;
}
@JsonProperty(JSON_PROPERTY_STARTED_BY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setStartedById(String startedById) {
this.startedById = startedById;
}
public JobV1 status(JobStatusV1 status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
*/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public JobStatusV1 getStatus() {
return status;
}
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setStatus(JobStatusV1 status) {
this.status = status;
}
public JobV1 title(String title) {
this.title = title;
return this;
}
/**
* Get title
* @return title
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_TITLE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getTitle() {
return title;
}
@JsonProperty(JSON_PROPERTY_TITLE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTitle(String title) {
this.title = title;
}
public JobV1 usage(JobUsageV1 usage) {
this.usage = usage;
return this;
}
/**
* Get usage
* @return usage
*/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_USAGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JobUsageV1 getUsage() {
return usage;
}
@JsonProperty(JSON_PROPERTY_USAGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUsage(JobUsageV1 usage) {
this.usage = usage;
}
/**
* Return true if this JobV1 object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
JobV1 jobV1 = (JobV1) o;
return Objects.equals(this.commitDetails, jobV1.commitDetails) &&
Objects.equals(this.computeCluster, jobV1.computeCluster) &&
Objects.equals(this.datasetMounts, jobV1.datasetMounts) &&
Objects.equals(this.dominoStats, jobV1.dominoStats) &&
Objects.equals(this.externalVolumeMounts, jobV1.externalVolumeMounts) &&
Objects.equals(this.gitRepos, jobV1.gitRepos) &&
Objects.equals(this.id, jobV1.id) &&
Objects.equals(this.mainRepoGitRef, jobV1.mainRepoGitRef) &&
Objects.equals(this.number, jobV1.number) &&
Objects.equals(this.projects, jobV1.projects) &&
Objects.equals(this.runCommand, jobV1.runCommand) &&
Objects.equals(this.runLauncherId, jobV1.runLauncherId) &&
Objects.equals(this.stageTimes, jobV1.stageTimes) &&
Objects.equals(this.startedById, jobV1.startedById) &&
Objects.equals(this.status, jobV1.status) &&
Objects.equals(this.title, jobV1.title) &&
Objects.equals(this.usage, jobV1.usage);
}
@Override
public int hashCode() {
return Objects.hash(commitDetails, computeCluster, datasetMounts, dominoStats, externalVolumeMounts, gitRepos, id, mainRepoGitRef, number, projects, runCommand, runLauncherId, stageTimes, startedById, status, title, usage);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class JobV1 {\n");
sb.append(" commitDetails: ").append(toIndentedString(commitDetails)).append("\n");
sb.append(" computeCluster: ").append(toIndentedString(computeCluster)).append("\n");
sb.append(" datasetMounts: ").append(toIndentedString(datasetMounts)).append("\n");
sb.append(" dominoStats: ").append(toIndentedString(dominoStats)).append("\n");
sb.append(" externalVolumeMounts: ").append(toIndentedString(externalVolumeMounts)).append("\n");
sb.append(" gitRepos: ").append(toIndentedString(gitRepos)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" mainRepoGitRef: ").append(toIndentedString(mainRepoGitRef)).append("\n");
sb.append(" number: ").append(toIndentedString(number)).append("\n");
sb.append(" projects: ").append(toIndentedString(projects)).append("\n");
sb.append(" runCommand: ").append(toIndentedString(runCommand)).append("\n");
sb.append(" runLauncherId: ").append(toIndentedString(runLauncherId)).append("\n");
sb.append(" stageTimes: ").append(toIndentedString(stageTimes)).append("\n");
sb.append(" startedById: ").append(toIndentedString(startedById)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" title: ").append(toIndentedString(title)).append("\n");
sb.append(" usage: ").append(toIndentedString(usage)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `commitDetails` to the URL query string
if (getCommitDetails() != null) {
joiner.add(getCommitDetails().toUrlQueryString(prefix + "commitDetails" + suffix));
}
// add `computeCluster` to the URL query string
if (getComputeCluster() != null) {
joiner.add(getComputeCluster().toUrlQueryString(prefix + "computeCluster" + suffix));
}
// add `datasetMounts` to the URL query string
if (getDatasetMounts() != null) {
for (int i = 0; i < getDatasetMounts().size(); i++) {
if (getDatasetMounts().get(i) != null) {
joiner.add(getDatasetMounts().get(i).toUrlQueryString(String.format("%sdatasetMounts%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `dominoStats` to the URL query string
if (getDominoStats() != null) {
for (int i = 0; i < getDominoStats().size(); i++) {
if (getDominoStats().get(i) != null) {
joiner.add(getDominoStats().get(i).toUrlQueryString(String.format("%sdominoStats%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `externalVolumeMounts` to the URL query string
if (getExternalVolumeMounts() != null) {
for (int i = 0; i < getExternalVolumeMounts().size(); i++) {
if (getExternalVolumeMounts().get(i) != null) {
joiner.add(getExternalVolumeMounts().get(i).toUrlQueryString(String.format("%sexternalVolumeMounts%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `gitRepos` to the URL query string
if (getGitRepos() != null) {
for (int i = 0; i < getGitRepos().size(); i++) {
if (getGitRepos().get(i) != null) {
joiner.add(getGitRepos().get(i).toUrlQueryString(String.format("%sgitRepos%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `id` to the URL query string
if (getId() != null) {
joiner.add(String.format("%sid%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `mainRepoGitRef` to the URL query string
if (getMainRepoGitRef() != null) {
joiner.add(getMainRepoGitRef().toUrlQueryString(prefix + "mainRepoGitRef" + suffix));
}
// add `number` to the URL query string
if (getNumber() != null) {
joiner.add(String.format("%snumber%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getNumber()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `projects` to the URL query string
if (getProjects() != null) {
for (int i = 0; i < getProjects().size(); i++) {
if (getProjects().get(i) != null) {
joiner.add(getProjects().get(i).toUrlQueryString(String.format("%sprojects%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `runCommand` to the URL query string
if (getRunCommand() != null) {
joiner.add(String.format("%srunCommand%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getRunCommand()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `runLauncherId` to the URL query string
if (getRunLauncherId() != null) {
joiner.add(String.format("%srunLauncherId%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getRunLauncherId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `stageTimes` to the URL query string
if (getStageTimes() != null) {
joiner.add(getStageTimes().toUrlQueryString(prefix + "stageTimes" + suffix));
}
// add `startedById` to the URL query string
if (getStartedById() != null) {
joiner.add(String.format("%sstartedById%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getStartedById()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `status` to the URL query string
if (getStatus() != null) {
joiner.add(getStatus().toUrlQueryString(prefix + "status" + suffix));
}
// add `title` to the URL query string
if (getTitle() != null) {
joiner.add(String.format("%stitle%s=%s", prefix, suffix, URLEncoder.encode(ApiClient.valueToString(getTitle()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `usage` to the URL query string
if (getUsage() != null) {
joiner.add(getUsage().toUrlQueryString(prefix + "usage" + suffix));
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy