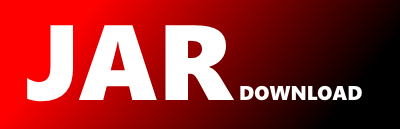
com.dominodatalab.pub.rest.CostApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of domino-java-client Show documentation
Show all versions of domino-java-client Show documentation
Domino Data Lab API Client to connect to Domino web services using Java HTTP Client.
/*
* Domino Public API
* Domino Public API Endpoints
*
* The version of the OpenAPI document: 0.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.pub.rest;
import com.dominodatalab.pub.invoker.ApiClient;
import com.dominodatalab.pub.invoker.ApiException;
import com.dominodatalab.pub.invoker.ApiResponse;
import com.dominodatalab.pub.invoker.Pair;
import com.dominodatalab.pub.model.AthenaBillingConfigsV1;
import com.dominodatalab.pub.model.CostAllocationEnvelopeV1;
import com.dominodatalab.pub.model.CostAssetsEnvelopeV1;
import com.dominodatalab.pub.model.FailureEnvelopeV1;
import com.dominodatalab.pub.model.GetAIGatewayAuditData400Response;
import com.dominodatalab.pub.model.KubecostLicenseResponseV1;
import com.dominodatalab.pub.model.KubecostLicenseV1;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.InputStream;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.IOException;
import java.io.OutputStream;
import java.net.http.HttpRequest;
import java.nio.channels.Channels;
import java.nio.channels.Pipe;
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.time.Duration;
import java.util.ArrayList;
import java.util.StringJoiner;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.function.Consumer;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-10-04T16:37:28.765500600-04:00[America/New_York]", comments = "Generator version: 7.8.0")
public class CostApi {
protected final HttpClient memberVarHttpClient;
protected final ObjectMapper memberVarObjectMapper;
protected final String memberVarBaseUri;
protected final Consumer memberVarInterceptor;
protected final Duration memberVarReadTimeout;
protected final Consumer> memberVarResponseInterceptor;
protected final Consumer> memberVarAsyncResponseInterceptor;
public CostApi() {
this(new ApiClient());
}
public CostApi(ApiClient apiClient) {
memberVarHttpClient = apiClient.getHttpClient();
memberVarObjectMapper = apiClient.getObjectMapper();
memberVarBaseUri = apiClient.getBaseUri();
memberVarInterceptor = apiClient.getRequestInterceptor();
memberVarReadTimeout = apiClient.getReadTimeout();
memberVarResponseInterceptor = apiClient.getResponseInterceptor();
memberVarAsyncResponseInterceptor = apiClient.getAsyncResponseInterceptor();
}
protected ApiException getApiException(String operationId, HttpResponse response) throws IOException {
String body = response.body() == null ? null : new String(response.body().readAllBytes());
String message = formatExceptionMessage(operationId, response.statusCode(), body);
return new ApiException(response.statusCode(), message, response.headers(), body);
}
protected String formatExceptionMessage(String operationId, int statusCode, String body) {
if (body == null || body.isEmpty()) {
body = "[no body]";
}
return operationId + " call failed with: " + statusCode + " - " + body;
}
/**
* Add kubecost license key
* Add kubecost license key
* @param kubecostLicenseV1 Kubecost License Key (required)
* @return KubecostLicenseResponseV1
* @throws ApiException if fails to make API call
*/
public KubecostLicenseResponseV1 addKubecostLicenseKey(KubecostLicenseV1 kubecostLicenseV1) throws ApiException {
ApiResponse localVarResponse = addKubecostLicenseKeyWithHttpInfo(kubecostLicenseV1);
return localVarResponse.getData();
}
/**
* Add kubecost license key
* Add kubecost license key
* @param kubecostLicenseV1 Kubecost License Key (required)
* @return ApiResponse<KubecostLicenseResponseV1>
* @throws ApiException if fails to make API call
*/
public ApiResponse addKubecostLicenseKeyWithHttpInfo(KubecostLicenseV1 kubecostLicenseV1) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = addKubecostLicenseKeyRequestBuilder(kubecostLicenseV1);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("addKubecostLicenseKey", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder addKubecostLicenseKeyRequestBuilder(KubecostLicenseV1 kubecostLicenseV1) throws ApiException {
// verify the required parameter 'kubecostLicenseV1' is set
if (kubecostLicenseV1 == null) {
throw new ApiException(400, "Missing the required parameter 'kubecostLicenseV1' when calling addKubecostLicenseKey");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/cost/v1/licenseKey";
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(kubecostLicenseV1);
localVarRequestBuilder.method("PUT", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Get the cost allocation over a time window
* Retrieve cost allocation
* @param window Duration of time over which to query. Accepts words like today, week, month, yesterday, lastweek, lastmonth; durations like 30m, 12h, 7d, or time like 2021-03-10T00:00:00Z,2021-03-11T00:00:00Z (required)
* @param aggregate Aggregate the cost allocation. Accepts kubecost aggregates cluster, namespace, pod, deployment, service, daemonset, statefulset, job, cronjob, replicaset, node, container, pv, pvc, storageclass, cluster (optional)
* @param start Start time of the query. Accepts RFC3339 format, must be inside the window (optional)
* @param end End time of the query. Accepts RFC3339 format, must be inside the window (optional)
* @param accumulate If the result will be accumulated. Default is false. If true, the result will be accumulated from the start time to the end time. (optional)
* @param filter Filter the result by the kubecost filter like cluster, namespace, pod, deployment, service, daemonset, statefulset, job, cronjob, replicaset, node, container, pv, pvc, storageclass, cluster. (optional)
* @param shareIdle if the idle cost will be shared (optional)
* @param shareNamespaces list of namespaces to share the costs (optional)
* @param shareSplit Determines how to split shared costs among non-idle, unshared allocations. (optional)
* @param shareCost The cost to share (optional)
* @param shareLabels The label to share the cost (optional)
* @param reconcile If the cost will be reconciled (optional)
* @param shareTenancyCosts If the tenancy costs will be shared (optional)
* @param idle If the idle cost will be calculated (optional)
* @param external If the external cost will be calculated (optional)
* @return CostAllocationEnvelopeV1
* @throws ApiException if fails to make API call
*/
public CostAllocationEnvelopeV1 getCostAllocation(String window, String aggregate, String start, String end, Boolean accumulate, String filter, Boolean shareIdle, String shareNamespaces, String shareSplit, String shareCost, String shareLabels, Boolean reconcile, Boolean shareTenancyCosts, Boolean idle, Boolean external) throws ApiException {
ApiResponse localVarResponse = getCostAllocationWithHttpInfo(window, aggregate, start, end, accumulate, filter, shareIdle, shareNamespaces, shareSplit, shareCost, shareLabels, reconcile, shareTenancyCosts, idle, external);
return localVarResponse.getData();
}
/**
* Get the cost allocation over a time window
* Retrieve cost allocation
* @param window Duration of time over which to query. Accepts words like today, week, month, yesterday, lastweek, lastmonth; durations like 30m, 12h, 7d, or time like 2021-03-10T00:00:00Z,2021-03-11T00:00:00Z (required)
* @param aggregate Aggregate the cost allocation. Accepts kubecost aggregates cluster, namespace, pod, deployment, service, daemonset, statefulset, job, cronjob, replicaset, node, container, pv, pvc, storageclass, cluster (optional)
* @param start Start time of the query. Accepts RFC3339 format, must be inside the window (optional)
* @param end End time of the query. Accepts RFC3339 format, must be inside the window (optional)
* @param accumulate If the result will be accumulated. Default is false. If true, the result will be accumulated from the start time to the end time. (optional)
* @param filter Filter the result by the kubecost filter like cluster, namespace, pod, deployment, service, daemonset, statefulset, job, cronjob, replicaset, node, container, pv, pvc, storageclass, cluster. (optional)
* @param shareIdle if the idle cost will be shared (optional)
* @param shareNamespaces list of namespaces to share the costs (optional)
* @param shareSplit Determines how to split shared costs among non-idle, unshared allocations. (optional)
* @param shareCost The cost to share (optional)
* @param shareLabels The label to share the cost (optional)
* @param reconcile If the cost will be reconciled (optional)
* @param shareTenancyCosts If the tenancy costs will be shared (optional)
* @param idle If the idle cost will be calculated (optional)
* @param external If the external cost will be calculated (optional)
* @return ApiResponse<CostAllocationEnvelopeV1>
* @throws ApiException if fails to make API call
*/
public ApiResponse getCostAllocationWithHttpInfo(String window, String aggregate, String start, String end, Boolean accumulate, String filter, Boolean shareIdle, String shareNamespaces, String shareSplit, String shareCost, String shareLabels, Boolean reconcile, Boolean shareTenancyCosts, Boolean idle, Boolean external) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getCostAllocationRequestBuilder(window, aggregate, start, end, accumulate, filter, shareIdle, shareNamespaces, shareSplit, shareCost, shareLabels, reconcile, shareTenancyCosts, idle, external);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getCostAllocation", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder getCostAllocationRequestBuilder(String window, String aggregate, String start, String end, Boolean accumulate, String filter, Boolean shareIdle, String shareNamespaces, String shareSplit, String shareCost, String shareLabels, Boolean reconcile, Boolean shareTenancyCosts, Boolean idle, Boolean external) throws ApiException {
// verify the required parameter 'window' is set
if (window == null) {
throw new ApiException(400, "Missing the required parameter 'window' when calling getCostAllocation");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/cost/v1/allocation";
List localVarQueryParams = new ArrayList<>();
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
localVarQueryParameterBaseName = "window";
localVarQueryParams.addAll(ApiClient.parameterToPairs("window", window));
localVarQueryParameterBaseName = "aggregate";
localVarQueryParams.addAll(ApiClient.parameterToPairs("aggregate", aggregate));
localVarQueryParameterBaseName = "start";
localVarQueryParams.addAll(ApiClient.parameterToPairs("start", start));
localVarQueryParameterBaseName = "end";
localVarQueryParams.addAll(ApiClient.parameterToPairs("end", end));
localVarQueryParameterBaseName = "accumulate";
localVarQueryParams.addAll(ApiClient.parameterToPairs("accumulate", accumulate));
localVarQueryParameterBaseName = "filter";
localVarQueryParams.addAll(ApiClient.parameterToPairs("filter", filter));
localVarQueryParameterBaseName = "shareIdle";
localVarQueryParams.addAll(ApiClient.parameterToPairs("shareIdle", shareIdle));
localVarQueryParameterBaseName = "shareNamespaces";
localVarQueryParams.addAll(ApiClient.parameterToPairs("shareNamespaces", shareNamespaces));
localVarQueryParameterBaseName = "shareSplit";
localVarQueryParams.addAll(ApiClient.parameterToPairs("shareSplit", shareSplit));
localVarQueryParameterBaseName = "shareCost";
localVarQueryParams.addAll(ApiClient.parameterToPairs("shareCost", shareCost));
localVarQueryParameterBaseName = "shareLabels";
localVarQueryParams.addAll(ApiClient.parameterToPairs("shareLabels", shareLabels));
localVarQueryParameterBaseName = "reconcile";
localVarQueryParams.addAll(ApiClient.parameterToPairs("reconcile", reconcile));
localVarQueryParameterBaseName = "shareTenancyCosts";
localVarQueryParams.addAll(ApiClient.parameterToPairs("shareTenancyCosts", shareTenancyCosts));
localVarQueryParameterBaseName = "idle";
localVarQueryParams.addAll(ApiClient.parameterToPairs("idle", idle));
localVarQueryParameterBaseName = "external";
localVarQueryParams.addAll(ApiClient.parameterToPairs("external", external));
if (!localVarQueryParams.isEmpty() || localVarQueryStringJoiner.length() != 0) {
StringJoiner queryJoiner = new StringJoiner("&");
localVarQueryParams.forEach(p -> queryJoiner.add(p.getName() + '=' + p.getValue()));
if (localVarQueryStringJoiner.length() != 0) {
queryJoiner.add(localVarQueryStringJoiner.toString());
}
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath + '?' + queryJoiner.toString()));
} else {
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
}
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Get the asset cost over a time window
* Retrieve asset cost
* @param window Duration of time over which to query. Accepts words like today, week, month, yesterday, lastweek, lastmonth; durations like 30m, 12h, 7d, or time like 2021-03-10T00:00:00Z,2021-03-11T00:00:00Z (required)
* @param aggregate Aggregate the cost allocation. Accepts kubecost aggregates cluster, namespace, pod, deployment, service, daemonset, statefulset, job, cronjob, replicaset, node, container, pv, pvc, storageclass, cluster (optional)
* @param start Start time of the query. Accepts RFC3339 format, must be inside the window (optional)
* @param end End time of the query. Accepts RFC3339 format, must be inside the window (optional)
* @param accumulate If the result will be accumulated. Default is false. If true, the result will be accumulated from the start time to the end time. (optional)
* @param filter Filter the result by the kubecost filter like cluster, namespace, pod, deployment, service, daemonset, statefulset (optional)
* @return CostAssetsEnvelopeV1
* @throws ApiException if fails to make API call
*/
public CostAssetsEnvelopeV1 getCostAssets(String window, String aggregate, String start, String end, Boolean accumulate, String filter) throws ApiException {
ApiResponse localVarResponse = getCostAssetsWithHttpInfo(window, aggregate, start, end, accumulate, filter);
return localVarResponse.getData();
}
/**
* Get the asset cost over a time window
* Retrieve asset cost
* @param window Duration of time over which to query. Accepts words like today, week, month, yesterday, lastweek, lastmonth; durations like 30m, 12h, 7d, or time like 2021-03-10T00:00:00Z,2021-03-11T00:00:00Z (required)
* @param aggregate Aggregate the cost allocation. Accepts kubecost aggregates cluster, namespace, pod, deployment, service, daemonset, statefulset, job, cronjob, replicaset, node, container, pv, pvc, storageclass, cluster (optional)
* @param start Start time of the query. Accepts RFC3339 format, must be inside the window (optional)
* @param end End time of the query. Accepts RFC3339 format, must be inside the window (optional)
* @param accumulate If the result will be accumulated. Default is false. If true, the result will be accumulated from the start time to the end time. (optional)
* @param filter Filter the result by the kubecost filter like cluster, namespace, pod, deployment, service, daemonset, statefulset (optional)
* @return ApiResponse<CostAssetsEnvelopeV1>
* @throws ApiException if fails to make API call
*/
public ApiResponse getCostAssetsWithHttpInfo(String window, String aggregate, String start, String end, Boolean accumulate, String filter) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getCostAssetsRequestBuilder(window, aggregate, start, end, accumulate, filter);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getCostAssets", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder getCostAssetsRequestBuilder(String window, String aggregate, String start, String end, Boolean accumulate, String filter) throws ApiException {
// verify the required parameter 'window' is set
if (window == null) {
throw new ApiException(400, "Missing the required parameter 'window' when calling getCostAssets");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/cost/v1/asset";
List localVarQueryParams = new ArrayList<>();
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
localVarQueryParameterBaseName = "window";
localVarQueryParams.addAll(ApiClient.parameterToPairs("window", window));
localVarQueryParameterBaseName = "aggregate";
localVarQueryParams.addAll(ApiClient.parameterToPairs("aggregate", aggregate));
localVarQueryParameterBaseName = "start";
localVarQueryParams.addAll(ApiClient.parameterToPairs("start", start));
localVarQueryParameterBaseName = "end";
localVarQueryParams.addAll(ApiClient.parameterToPairs("end", end));
localVarQueryParameterBaseName = "accumulate";
localVarQueryParams.addAll(ApiClient.parameterToPairs("accumulate", accumulate));
localVarQueryParameterBaseName = "filter";
localVarQueryParams.addAll(ApiClient.parameterToPairs("filter", filter));
if (!localVarQueryParams.isEmpty() || localVarQueryStringJoiner.length() != 0) {
StringJoiner queryJoiner = new StringJoiner("&");
localVarQueryParams.forEach(p -> queryJoiner.add(p.getName() + '=' + p.getValue()));
if (localVarQueryStringJoiner.length() != 0) {
queryJoiner.add(localVarQueryStringJoiner.toString());
}
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath + '?' + queryJoiner.toString()));
} else {
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
}
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Set AWS Billing API Configuration
* Set AWS Billing API Configuration
* @param athenaBillingConfigsV1 AWS Billing API Config (required)
* @return AthenaBillingConfigsV1
* @throws ApiException if fails to make API call
*/
public AthenaBillingConfigsV1 setAthenaConfigs(AthenaBillingConfigsV1 athenaBillingConfigsV1) throws ApiException {
ApiResponse localVarResponse = setAthenaConfigsWithHttpInfo(athenaBillingConfigsV1);
return localVarResponse.getData();
}
/**
* Set AWS Billing API Configuration
* Set AWS Billing API Configuration
* @param athenaBillingConfigsV1 AWS Billing API Config (required)
* @return ApiResponse<AthenaBillingConfigsV1>
* @throws ApiException if fails to make API call
*/
public ApiResponse setAthenaConfigsWithHttpInfo(AthenaBillingConfigsV1 athenaBillingConfigsV1) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = setAthenaConfigsRequestBuilder(athenaBillingConfigsV1);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("setAthenaConfigs", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder setAthenaConfigsRequestBuilder(AthenaBillingConfigsV1 athenaBillingConfigsV1) throws ApiException {
// verify the required parameter 'athenaBillingConfigsV1' is set
if (athenaBillingConfigsV1 == null) {
throw new ApiException(400, "Missing the required parameter 'athenaBillingConfigsV1' when calling setAthenaConfigs");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/cost/v1/athenaConfigs";
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(athenaBillingConfigsV1);
localVarRequestBuilder.method("PUT", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy