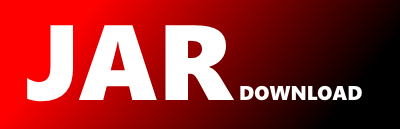
com.dominodatalab.pub.rest.DatasetRwApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of domino-java-client Show documentation
Show all versions of domino-java-client Show documentation
Domino Data Lab API Client to connect to Domino web services using Java HTTP Client.
/*
* Domino Public API
* Domino Public API Endpoints
*
* The version of the OpenAPI document: 0.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.pub.rest;
import com.dominodatalab.pub.invoker.ApiClient;
import com.dominodatalab.pub.invoker.ApiException;
import com.dominodatalab.pub.invoker.ApiResponse;
import com.dominodatalab.pub.invoker.Pair;
import com.dominodatalab.pub.model.DatasetRwEnvelopeV1;
import com.dominodatalab.pub.model.DatasetRwGrantDetailsEnvelopeV1;
import com.dominodatalab.pub.model.DatasetRwGrantEnvelopeV1;
import com.dominodatalab.pub.model.DatasetRwGrantV1;
import com.dominodatalab.pub.model.DatasetRwMetadataV1;
import com.dominodatalab.pub.model.DatasetRwPermissionV1;
import com.dominodatalab.pub.model.DatasetRwTagToAddV1;
import com.dominodatalab.pub.model.FailureEnvelopeV1;
import com.dominodatalab.pub.model.GetAIGatewayAuditData400Response;
import com.dominodatalab.pub.model.NewDatasetRwV1;
import com.dominodatalab.pub.model.NewSnapshotV1;
import com.dominodatalab.pub.model.PaginatedDatasetRwEnvelopeV1;
import com.dominodatalab.pub.model.PaginatedDatasetRwEnvelopeV2;
import com.dominodatalab.pub.model.PaginatedSnapshotEnvelopeV1;
import com.dominodatalab.pub.model.SnapshotEnvelopeV1;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.InputStream;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.IOException;
import java.io.OutputStream;
import java.net.http.HttpRequest;
import java.nio.channels.Channels;
import java.nio.channels.Pipe;
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.time.Duration;
import java.util.ArrayList;
import java.util.StringJoiner;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.function.Consumer;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-10-04T16:37:28.765500600-04:00[America/New_York]", comments = "Generator version: 7.8.0")
public class DatasetRwApi {
protected final HttpClient memberVarHttpClient;
protected final ObjectMapper memberVarObjectMapper;
protected final String memberVarBaseUri;
protected final Consumer memberVarInterceptor;
protected final Duration memberVarReadTimeout;
protected final Consumer> memberVarResponseInterceptor;
protected final Consumer> memberVarAsyncResponseInterceptor;
public DatasetRwApi() {
this(new ApiClient());
}
public DatasetRwApi(ApiClient apiClient) {
memberVarHttpClient = apiClient.getHttpClient();
memberVarObjectMapper = apiClient.getObjectMapper();
memberVarBaseUri = apiClient.getBaseUri();
memberVarInterceptor = apiClient.getRequestInterceptor();
memberVarReadTimeout = apiClient.getReadTimeout();
memberVarResponseInterceptor = apiClient.getResponseInterceptor();
memberVarAsyncResponseInterceptor = apiClient.getAsyncResponseInterceptor();
}
protected ApiException getApiException(String operationId, HttpResponse response) throws IOException {
String body = response.body() == null ? null : new String(response.body().readAllBytes());
String message = formatExceptionMessage(operationId, response.statusCode(), body);
return new ApiException(response.statusCode(), message, response.headers(), body);
}
protected String formatExceptionMessage(String operationId, int statusCode, String body) {
if (body == null || body.isEmpty()) {
body = "[no body]";
}
return operationId + " call failed with: " + statusCode + " - " + body;
}
/**
* Add a grant to a dataset's existing sequence of grants
* Add a grant to a dataset's existing sequence of grants. Requires EditSecurity access to the dataset.
* @param datasetId ID of dataset to add a grant to (required)
* @param datasetRwGrantV1 Grant to add (required)
* @return DatasetRwGrantEnvelopeV1
* @throws ApiException if fails to make API call
*/
public DatasetRwGrantEnvelopeV1 addDatasetGrant(String datasetId, DatasetRwGrantV1 datasetRwGrantV1) throws ApiException {
ApiResponse localVarResponse = addDatasetGrantWithHttpInfo(datasetId, datasetRwGrantV1);
return localVarResponse.getData();
}
/**
* Add a grant to a dataset's existing sequence of grants
* Add a grant to a dataset's existing sequence of grants. Requires EditSecurity access to the dataset.
* @param datasetId ID of dataset to add a grant to (required)
* @param datasetRwGrantV1 Grant to add (required)
* @return ApiResponse<DatasetRwGrantEnvelopeV1>
* @throws ApiException if fails to make API call
*/
public ApiResponse addDatasetGrantWithHttpInfo(String datasetId, DatasetRwGrantV1 datasetRwGrantV1) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = addDatasetGrantRequestBuilder(datasetId, datasetRwGrantV1);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("addDatasetGrant", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder addDatasetGrantRequestBuilder(String datasetId, DatasetRwGrantV1 datasetRwGrantV1) throws ApiException {
// verify the required parameter 'datasetId' is set
if (datasetId == null) {
throw new ApiException(400, "Missing the required parameter 'datasetId' when calling addDatasetGrant");
}
// verify the required parameter 'datasetRwGrantV1' is set
if (datasetRwGrantV1 == null) {
throw new ApiException(400, "Missing the required parameter 'datasetRwGrantV1' when calling addDatasetGrant");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/datasetrw/v1/datasets/{datasetId}/grants"
.replace("{datasetId}", ApiClient.urlEncode(datasetId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(datasetRwGrantV1);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Tag a snapshot in this Dataset
* Tag a snapshot in this Dataset with the given tagName. Requires Update access to the dataset
* @param datasetId Dataset ID (required)
* @param datasetRwTagToAddV1 Tag name and snapshot ID to apply it to (required)
* @return DatasetRwEnvelopeV1
* @throws ApiException if fails to make API call
*/
public DatasetRwEnvelopeV1 addDatasetTag(String datasetId, DatasetRwTagToAddV1 datasetRwTagToAddV1) throws ApiException {
ApiResponse localVarResponse = addDatasetTagWithHttpInfo(datasetId, datasetRwTagToAddV1);
return localVarResponse.getData();
}
/**
* Tag a snapshot in this Dataset
* Tag a snapshot in this Dataset with the given tagName. Requires Update access to the dataset
* @param datasetId Dataset ID (required)
* @param datasetRwTagToAddV1 Tag name and snapshot ID to apply it to (required)
* @return ApiResponse<DatasetRwEnvelopeV1>
* @throws ApiException if fails to make API call
*/
public ApiResponse addDatasetTagWithHttpInfo(String datasetId, DatasetRwTagToAddV1 datasetRwTagToAddV1) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = addDatasetTagRequestBuilder(datasetId, datasetRwTagToAddV1);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("addDatasetTag", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder addDatasetTagRequestBuilder(String datasetId, DatasetRwTagToAddV1 datasetRwTagToAddV1) throws ApiException {
// verify the required parameter 'datasetId' is set
if (datasetId == null) {
throw new ApiException(400, "Missing the required parameter 'datasetId' when calling addDatasetTag");
}
// verify the required parameter 'datasetRwTagToAddV1' is set
if (datasetRwTagToAddV1 == null) {
throw new ApiException(400, "Missing the required parameter 'datasetRwTagToAddV1' when calling addDatasetTag");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/datasetrw/v1/datasets/{datasetId}/tags"
.replace("{datasetId}", ApiClient.urlEncode(datasetId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(datasetRwTagToAddV1);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Create a dataset
* Create a new Dataset. Requires access to the project the dataset will originate from
* @param newDatasetRwV1 Dataset to create (required)
* @return DatasetRwEnvelopeV1
* @throws ApiException if fails to make API call
*/
public DatasetRwEnvelopeV1 createDataset(NewDatasetRwV1 newDatasetRwV1) throws ApiException {
ApiResponse localVarResponse = createDatasetWithHttpInfo(newDatasetRwV1);
return localVarResponse.getData();
}
/**
* Create a dataset
* Create a new Dataset. Requires access to the project the dataset will originate from
* @param newDatasetRwV1 Dataset to create (required)
* @return ApiResponse<DatasetRwEnvelopeV1>
* @throws ApiException if fails to make API call
*/
public ApiResponse createDatasetWithHttpInfo(NewDatasetRwV1 newDatasetRwV1) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = createDatasetRequestBuilder(newDatasetRwV1);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("createDataset", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder createDatasetRequestBuilder(NewDatasetRwV1 newDatasetRwV1) throws ApiException {
// verify the required parameter 'newDatasetRwV1' is set
if (newDatasetRwV1 == null) {
throw new ApiException(400, "Missing the required parameter 'newDatasetRwV1' when calling createDataset");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/datasetrw/v1/datasets";
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(newDatasetRwV1);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Create a snapshot
* Create a new Snapshot in a dataset. Requires Read access to the dataset and project access
* @param datasetId Dataset ID (required)
* @param newSnapshotV1 Snapshot to create (required)
* @return SnapshotEnvelopeV1
* @throws ApiException if fails to make API call
*/
public SnapshotEnvelopeV1 createSnapshot(String datasetId, NewSnapshotV1 newSnapshotV1) throws ApiException {
ApiResponse localVarResponse = createSnapshotWithHttpInfo(datasetId, newSnapshotV1);
return localVarResponse.getData();
}
/**
* Create a snapshot
* Create a new Snapshot in a dataset. Requires Read access to the dataset and project access
* @param datasetId Dataset ID (required)
* @param newSnapshotV1 Snapshot to create (required)
* @return ApiResponse<SnapshotEnvelopeV1>
* @throws ApiException if fails to make API call
*/
public ApiResponse createSnapshotWithHttpInfo(String datasetId, NewSnapshotV1 newSnapshotV1) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = createSnapshotRequestBuilder(datasetId, newSnapshotV1);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("createSnapshot", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder createSnapshotRequestBuilder(String datasetId, NewSnapshotV1 newSnapshotV1) throws ApiException {
// verify the required parameter 'datasetId' is set
if (datasetId == null) {
throw new ApiException(400, "Missing the required parameter 'datasetId' when calling createSnapshot");
}
// verify the required parameter 'newSnapshotV1' is set
if (newSnapshotV1 == null) {
throw new ApiException(400, "Missing the required parameter 'newSnapshotV1' when calling createSnapshot");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/datasetrw/v1/datasets/{datasetId}/snapshots"
.replace("{datasetId}", ApiClient.urlEncode(datasetId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(newSnapshotV1);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Delete Dataset
* Delete a dataset. Requires PermanentDelete access to the dataset
* @param datasetId ID of dataset to remove from project (required)
* @return DatasetRwEnvelopeV1
* @throws ApiException if fails to make API call
*/
public DatasetRwEnvelopeV1 deleteDataset(String datasetId) throws ApiException {
ApiResponse localVarResponse = deleteDatasetWithHttpInfo(datasetId);
return localVarResponse.getData();
}
/**
* Delete Dataset
* Delete a dataset. Requires PermanentDelete access to the dataset
* @param datasetId ID of dataset to remove from project (required)
* @return ApiResponse<DatasetRwEnvelopeV1>
* @throws ApiException if fails to make API call
*/
public ApiResponse deleteDatasetWithHttpInfo(String datasetId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = deleteDatasetRequestBuilder(datasetId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("deleteDataset", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder deleteDatasetRequestBuilder(String datasetId) throws ApiException {
// verify the required parameter 'datasetId' is set
if (datasetId == null) {
throw new ApiException(400, "Missing the required parameter 'datasetId' when calling deleteDataset");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/datasetrw/v1/datasets/{datasetId}"
.replace("{datasetId}", ApiClient.urlEncode(datasetId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("DELETE", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Get dataset by ID
* Get Dataset by ID. Requires List access to the dataset
* @param datasetId ID of dataset to retrieve (required)
* @return DatasetRwEnvelopeV1
* @throws ApiException if fails to make API call
*/
public DatasetRwEnvelopeV1 getDataset(String datasetId) throws ApiException {
ApiResponse localVarResponse = getDatasetWithHttpInfo(datasetId);
return localVarResponse.getData();
}
/**
* Get dataset by ID
* Get Dataset by ID. Requires List access to the dataset
* @param datasetId ID of dataset to retrieve (required)
* @return ApiResponse<DatasetRwEnvelopeV1>
* @throws ApiException if fails to make API call
*/
public ApiResponse getDatasetWithHttpInfo(String datasetId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getDatasetRequestBuilder(datasetId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getDataset", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder getDatasetRequestBuilder(String datasetId) throws ApiException {
// verify the required parameter 'datasetId' is set
if (datasetId == null) {
throw new ApiException(400, "Missing the required parameter 'datasetId' when calling getDataset");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/datasetrw/v1/datasets/{datasetId}"
.replace("{datasetId}", ApiClient.urlEncode(datasetId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Get dataset grants by ID
* Get Dataset grants by ID. Requires List access to the dataset
* @param datasetId ID of dataset to get grants for (required)
* @return DatasetRwGrantDetailsEnvelopeV1
* @throws ApiException if fails to make API call
*/
public DatasetRwGrantDetailsEnvelopeV1 getDatasetGrants(String datasetId) throws ApiException {
ApiResponse localVarResponse = getDatasetGrantsWithHttpInfo(datasetId);
return localVarResponse.getData();
}
/**
* Get dataset grants by ID
* Get Dataset grants by ID. Requires List access to the dataset
* @param datasetId ID of dataset to get grants for (required)
* @return ApiResponse<DatasetRwGrantDetailsEnvelopeV1>
* @throws ApiException if fails to make API call
*/
public ApiResponse getDatasetGrantsWithHttpInfo(String datasetId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getDatasetGrantsRequestBuilder(datasetId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getDatasetGrants", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder getDatasetGrantsRequestBuilder(String datasetId) throws ApiException {
// verify the required parameter 'datasetId' is set
if (datasetId == null) {
throw new ApiException(400, "Missing the required parameter 'datasetId' when calling getDatasetGrants");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/datasetrw/v1/datasets/{datasetId}/grants"
.replace("{datasetId}", ApiClient.urlEncode(datasetId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Get snapshots belonging to dataset
* Get Snapshots belonging to a dataset. Requires List access to the dataset
* @param datasetId ID of dataset to retrieve snapshots for (required)
* @param offset How many Snapshots from the start to skip. Defaults to 0. (optional)
* @param limit Max number of Snapshots to fetch. Defaults to 10. (optional)
* @return PaginatedSnapshotEnvelopeV1
* @throws ApiException if fails to make API call
*/
public PaginatedSnapshotEnvelopeV1 getDatasetSnapshots(String datasetId, Integer offset, Integer limit) throws ApiException {
ApiResponse localVarResponse = getDatasetSnapshotsWithHttpInfo(datasetId, offset, limit);
return localVarResponse.getData();
}
/**
* Get snapshots belonging to dataset
* Get Snapshots belonging to a dataset. Requires List access to the dataset
* @param datasetId ID of dataset to retrieve snapshots for (required)
* @param offset How many Snapshots from the start to skip. Defaults to 0. (optional)
* @param limit Max number of Snapshots to fetch. Defaults to 10. (optional)
* @return ApiResponse<PaginatedSnapshotEnvelopeV1>
* @throws ApiException if fails to make API call
*/
public ApiResponse getDatasetSnapshotsWithHttpInfo(String datasetId, Integer offset, Integer limit) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getDatasetSnapshotsRequestBuilder(datasetId, offset, limit);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getDatasetSnapshots", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder getDatasetSnapshotsRequestBuilder(String datasetId, Integer offset, Integer limit) throws ApiException {
// verify the required parameter 'datasetId' is set
if (datasetId == null) {
throw new ApiException(400, "Missing the required parameter 'datasetId' when calling getDatasetSnapshots");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/datasetrw/v1/datasets/{datasetId}/snapshots"
.replace("{datasetId}", ApiClient.urlEncode(datasetId.toString()));
List localVarQueryParams = new ArrayList<>();
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
localVarQueryParameterBaseName = "offset";
localVarQueryParams.addAll(ApiClient.parameterToPairs("offset", offset));
localVarQueryParameterBaseName = "limit";
localVarQueryParams.addAll(ApiClient.parameterToPairs("limit", limit));
if (!localVarQueryParams.isEmpty() || localVarQueryStringJoiner.length() != 0) {
StringJoiner queryJoiner = new StringJoiner("&");
localVarQueryParams.forEach(p -> queryJoiner.add(p.getName() + '=' + p.getValue()));
if (localVarQueryStringJoiner.length() != 0) {
queryJoiner.add(localVarQueryStringJoiner.toString());
}
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath + '?' + queryJoiner.toString()));
} else {
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
}
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Deprecated - Get datasets accessible to user
* Deprecated: Use GetDatasetsV2. Get Datasets that a user has access to
* @param projectId Project ID filter (optional)
* @param offset How many Datasets from the start to skip. Defaults to 0. (optional)
* @param limit Max number of Datasets to fetch. Defaults to 10. (optional)
* @return PaginatedDatasetRwEnvelopeV1
* @throws ApiException if fails to make API call
*/
public PaginatedDatasetRwEnvelopeV1 getDatasets(String projectId, Integer offset, Integer limit) throws ApiException {
ApiResponse localVarResponse = getDatasetsWithHttpInfo(projectId, offset, limit);
return localVarResponse.getData();
}
/**
* Deprecated - Get datasets accessible to user
* Deprecated: Use GetDatasetsV2. Get Datasets that a user has access to
* @param projectId Project ID filter (optional)
* @param offset How many Datasets from the start to skip. Defaults to 0. (optional)
* @param limit Max number of Datasets to fetch. Defaults to 10. (optional)
* @return ApiResponse<PaginatedDatasetRwEnvelopeV1>
* @throws ApiException if fails to make API call
*/
public ApiResponse getDatasetsWithHttpInfo(String projectId, Integer offset, Integer limit) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getDatasetsRequestBuilder(projectId, offset, limit);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getDatasets", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder getDatasetsRequestBuilder(String projectId, Integer offset, Integer limit) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/datasetrw/v1/datasets";
List localVarQueryParams = new ArrayList<>();
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
localVarQueryParameterBaseName = "projectId";
localVarQueryParams.addAll(ApiClient.parameterToPairs("projectId", projectId));
localVarQueryParameterBaseName = "offset";
localVarQueryParams.addAll(ApiClient.parameterToPairs("offset", offset));
localVarQueryParameterBaseName = "limit";
localVarQueryParams.addAll(ApiClient.parameterToPairs("limit", limit));
if (!localVarQueryParams.isEmpty() || localVarQueryStringJoiner.length() != 0) {
StringJoiner queryJoiner = new StringJoiner("&");
localVarQueryParams.forEach(p -> queryJoiner.add(p.getName() + '=' + p.getValue()));
if (localVarQueryStringJoiner.length() != 0) {
queryJoiner.add(localVarQueryStringJoiner.toString());
}
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath + '?' + queryJoiner.toString()));
} else {
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
}
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Get datasets the user has access to
* Get Datasets that a user has access to based on dataset permissions and input filters
* @param minimumPermission Filter for minimum dataset permission the principal needs to have in returned datasets. (optional)
* @param projectIdsToExclude ProjectIds of datasets to exclude from result. (optional
* @param projectIdsToInclude ProjectIds to get the datasets from. Should not be passed in if projectIdsToExclude is and vice versa. (optional
* @param includeProjectInfo Boolean to determine whether or not to return project-info in return objects. (optional)
* @param offset How many Datasets from the start to skip. Defaults to 0. (optional)
* @param limit Max number of Datasets to fetch. Defaults to 10. (optional)
* @return PaginatedDatasetRwEnvelopeV2
* @throws ApiException if fails to make API call
*/
public PaginatedDatasetRwEnvelopeV2 getDatasetsV2(DatasetRwPermissionV1 minimumPermission, List projectIdsToExclude, List projectIdsToInclude, Boolean includeProjectInfo, Integer offset, Integer limit) throws ApiException {
ApiResponse localVarResponse = getDatasetsV2WithHttpInfo(minimumPermission, projectIdsToExclude, projectIdsToInclude, includeProjectInfo, offset, limit);
return localVarResponse.getData();
}
/**
* Get datasets the user has access to
* Get Datasets that a user has access to based on dataset permissions and input filters
* @param minimumPermission Filter for minimum dataset permission the principal needs to have in returned datasets. (optional)
* @param projectIdsToExclude ProjectIds of datasets to exclude from result. (optional
* @param projectIdsToInclude ProjectIds to get the datasets from. Should not be passed in if projectIdsToExclude is and vice versa. (optional
* @param includeProjectInfo Boolean to determine whether or not to return project-info in return objects. (optional)
* @param offset How many Datasets from the start to skip. Defaults to 0. (optional)
* @param limit Max number of Datasets to fetch. Defaults to 10. (optional)
* @return ApiResponse<PaginatedDatasetRwEnvelopeV2>
* @throws ApiException if fails to make API call
*/
public ApiResponse getDatasetsV2WithHttpInfo(DatasetRwPermissionV1 minimumPermission, List projectIdsToExclude, List projectIdsToInclude, Boolean includeProjectInfo, Integer offset, Integer limit) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getDatasetsV2RequestBuilder(minimumPermission, projectIdsToExclude, projectIdsToInclude, includeProjectInfo, offset, limit);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getDatasetsV2", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder getDatasetsV2RequestBuilder(DatasetRwPermissionV1 minimumPermission, List projectIdsToExclude, List projectIdsToInclude, Boolean includeProjectInfo, Integer offset, Integer limit) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/datasetrw/v2/datasets";
List localVarQueryParams = new ArrayList<>();
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
localVarQueryParameterBaseName = "minimumPermission";
localVarQueryParams.addAll(ApiClient.parameterToPairs("minimumPermission", minimumPermission));
localVarQueryParameterBaseName = "projectIdsToExclude";
localVarQueryParams.addAll(ApiClient.parameterToPairs("multi", "projectIdsToExclude", projectIdsToExclude));
localVarQueryParameterBaseName = "projectIdsToInclude";
localVarQueryParams.addAll(ApiClient.parameterToPairs("multi", "projectIdsToInclude", projectIdsToInclude));
localVarQueryParameterBaseName = "includeProjectInfo";
localVarQueryParams.addAll(ApiClient.parameterToPairs("includeProjectInfo", includeProjectInfo));
localVarQueryParameterBaseName = "offset";
localVarQueryParams.addAll(ApiClient.parameterToPairs("offset", offset));
localVarQueryParameterBaseName = "limit";
localVarQueryParams.addAll(ApiClient.parameterToPairs("limit", limit));
if (!localVarQueryParams.isEmpty() || localVarQueryStringJoiner.length() != 0) {
StringJoiner queryJoiner = new StringJoiner("&");
localVarQueryParams.forEach(p -> queryJoiner.add(p.getName() + '=' + p.getValue()));
if (localVarQueryStringJoiner.length() != 0) {
queryJoiner.add(localVarQueryStringJoiner.toString());
}
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath + '?' + queryJoiner.toString()));
} else {
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
}
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Get snapshot
* Fetch a snapshot by ID. Requires List access to the dataset
* @param snapshotId Snapshot ID (required)
* @return SnapshotEnvelopeV1
* @throws ApiException if fails to make API call
*/
public SnapshotEnvelopeV1 getSnapshot(String snapshotId) throws ApiException {
ApiResponse localVarResponse = getSnapshotWithHttpInfo(snapshotId);
return localVarResponse.getData();
}
/**
* Get snapshot
* Fetch a snapshot by ID. Requires List access to the dataset
* @param snapshotId Snapshot ID (required)
* @return ApiResponse<SnapshotEnvelopeV1>
* @throws ApiException if fails to make API call
*/
public ApiResponse getSnapshotWithHttpInfo(String snapshotId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getSnapshotRequestBuilder(snapshotId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getSnapshot", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder getSnapshotRequestBuilder(String snapshotId) throws ApiException {
// verify the required parameter 'snapshotId' is set
if (snapshotId == null) {
throw new ApiException(400, "Missing the required parameter 'snapshotId' when calling getSnapshot");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/datasetrw/v1/snapshots/{snapshotId}"
.replace("{snapshotId}", ApiClient.urlEncode(snapshotId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Remove a grant from a dataset's existing sequence of grants
* Remove a grant from a dataset's existing sequence of grants. Requires EditSecurity access to the dataset
* @param datasetId ID of dataset to remove the grant from (required)
* @param datasetRwGrantV1 Grant to remove (required)
* @return DatasetRwGrantEnvelopeV1
* @throws ApiException if fails to make API call
*/
public DatasetRwGrantEnvelopeV1 removeDatasetGrant(String datasetId, DatasetRwGrantV1 datasetRwGrantV1) throws ApiException {
ApiResponse localVarResponse = removeDatasetGrantWithHttpInfo(datasetId, datasetRwGrantV1);
return localVarResponse.getData();
}
/**
* Remove a grant from a dataset's existing sequence of grants
* Remove a grant from a dataset's existing sequence of grants. Requires EditSecurity access to the dataset
* @param datasetId ID of dataset to remove the grant from (required)
* @param datasetRwGrantV1 Grant to remove (required)
* @return ApiResponse<DatasetRwGrantEnvelopeV1>
* @throws ApiException if fails to make API call
*/
public ApiResponse removeDatasetGrantWithHttpInfo(String datasetId, DatasetRwGrantV1 datasetRwGrantV1) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = removeDatasetGrantRequestBuilder(datasetId, datasetRwGrantV1);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("removeDatasetGrant", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder removeDatasetGrantRequestBuilder(String datasetId, DatasetRwGrantV1 datasetRwGrantV1) throws ApiException {
// verify the required parameter 'datasetId' is set
if (datasetId == null) {
throw new ApiException(400, "Missing the required parameter 'datasetId' when calling removeDatasetGrant");
}
// verify the required parameter 'datasetRwGrantV1' is set
if (datasetRwGrantV1 == null) {
throw new ApiException(400, "Missing the required parameter 'datasetRwGrantV1' when calling removeDatasetGrant");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/datasetrw/v1/datasets/{datasetId}/grants"
.replace("{datasetId}", ApiClient.urlEncode(datasetId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(datasetRwGrantV1);
localVarRequestBuilder.method("DELETE", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Remove a tag from a Dataset
* Remove a Tag from a dataset. Requires Update access to the dataset
* @param datasetId Dataset ID (required)
* @param tagName Name of tag to delete (required)
* @return DatasetRwEnvelopeV1
* @throws ApiException if fails to make API call
*/
public DatasetRwEnvelopeV1 removeDatasetTag(String datasetId, String tagName) throws ApiException {
ApiResponse localVarResponse = removeDatasetTagWithHttpInfo(datasetId, tagName);
return localVarResponse.getData();
}
/**
* Remove a tag from a Dataset
* Remove a Tag from a dataset. Requires Update access to the dataset
* @param datasetId Dataset ID (required)
* @param tagName Name of tag to delete (required)
* @return ApiResponse<DatasetRwEnvelopeV1>
* @throws ApiException if fails to make API call
*/
public ApiResponse removeDatasetTagWithHttpInfo(String datasetId, String tagName) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = removeDatasetTagRequestBuilder(datasetId, tagName);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("removeDatasetTag", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder removeDatasetTagRequestBuilder(String datasetId, String tagName) throws ApiException {
// verify the required parameter 'datasetId' is set
if (datasetId == null) {
throw new ApiException(400, "Missing the required parameter 'datasetId' when calling removeDatasetTag");
}
// verify the required parameter 'tagName' is set
if (tagName == null) {
throw new ApiException(400, "Missing the required parameter 'tagName' when calling removeDatasetTag");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/datasetrw/v1/datasets/{datasetId}/tags/{tagName}"
.replace("{datasetId}", ApiClient.urlEncode(datasetId.toString()))
.replace("{tagName}", ApiClient.urlEncode(tagName.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("DELETE", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Update dataset metadata
* Update Dataset name or description. Requires Update access to the dataset
* @param datasetId ID of dataset to update (required)
* @param datasetRwMetadataV1 Fields to update (required)
* @return DatasetRwEnvelopeV1
* @throws ApiException if fails to make API call
*/
public DatasetRwEnvelopeV1 updateDataset(String datasetId, DatasetRwMetadataV1 datasetRwMetadataV1) throws ApiException {
ApiResponse localVarResponse = updateDatasetWithHttpInfo(datasetId, datasetRwMetadataV1);
return localVarResponse.getData();
}
/**
* Update dataset metadata
* Update Dataset name or description. Requires Update access to the dataset
* @param datasetId ID of dataset to update (required)
* @param datasetRwMetadataV1 Fields to update (required)
* @return ApiResponse<DatasetRwEnvelopeV1>
* @throws ApiException if fails to make API call
*/
public ApiResponse updateDatasetWithHttpInfo(String datasetId, DatasetRwMetadataV1 datasetRwMetadataV1) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = updateDatasetRequestBuilder(datasetId, datasetRwMetadataV1);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("updateDataset", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder updateDatasetRequestBuilder(String datasetId, DatasetRwMetadataV1 datasetRwMetadataV1) throws ApiException {
// verify the required parameter 'datasetId' is set
if (datasetId == null) {
throw new ApiException(400, "Missing the required parameter 'datasetId' when calling updateDataset");
}
// verify the required parameter 'datasetRwMetadataV1' is set
if (datasetRwMetadataV1 == null) {
throw new ApiException(400, "Missing the required parameter 'datasetRwMetadataV1' when calling updateDataset");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/datasetrw/v1/datasets/{datasetId}"
.replace("{datasetId}", ApiClient.urlEncode(datasetId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(datasetRwMetadataV1);
localVarRequestBuilder.method("PATCH", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy