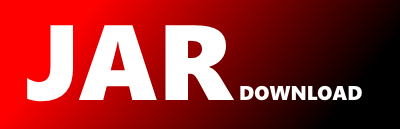
com.dominodatalab.pub.rest.ModelApiApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of domino-java-client Show documentation
Show all versions of domino-java-client Show documentation
Domino Data Lab API Client to connect to Domino web services using Java HTTP Client.
/*
* Domino Public API
* Domino Public API Endpoints
*
* The version of the OpenAPI document: 0.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.pub.rest;
import com.dominodatalab.pub.invoker.ApiClient;
import com.dominodatalab.pub.invoker.ApiException;
import com.dominodatalab.pub.invoker.ApiResponse;
import com.dominodatalab.pub.invoker.Pair;
import com.dominodatalab.pub.model.ModelApi;
import com.dominodatalab.pub.model.ModelApiAccessToken;
import com.dominodatalab.pub.model.ModelApiAccessTokenCreationRequest;
import com.dominodatalab.pub.model.ModelApiCollaboratorRole;
import com.dominodatalab.pub.model.ModelApiCreationRequest;
import com.dominodatalab.pub.model.ModelApiPaginatedList;
import com.dominodatalab.pub.model.ModelApiUpdateRequest;
import com.dominodatalab.pub.model.ModelApiVolume;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.InputStream;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.IOException;
import java.io.OutputStream;
import java.net.http.HttpRequest;
import java.nio.channels.Channels;
import java.nio.channels.Pipe;
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.time.Duration;
import java.util.ArrayList;
import java.util.StringJoiner;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.function.Consumer;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-10-04T16:37:28.765500600-04:00[America/New_York]", comments = "Generator version: 7.8.0")
public class ModelApiApi {
protected final HttpClient memberVarHttpClient;
protected final ObjectMapper memberVarObjectMapper;
protected final String memberVarBaseUri;
protected final Consumer memberVarInterceptor;
protected final Duration memberVarReadTimeout;
protected final Consumer> memberVarResponseInterceptor;
protected final Consumer> memberVarAsyncResponseInterceptor;
public ModelApiApi() {
this(new ApiClient());
}
public ModelApiApi(ApiClient apiClient) {
memberVarHttpClient = apiClient.getHttpClient();
memberVarObjectMapper = apiClient.getObjectMapper();
memberVarBaseUri = apiClient.getBaseUri();
memberVarInterceptor = apiClient.getRequestInterceptor();
memberVarReadTimeout = apiClient.getReadTimeout();
memberVarResponseInterceptor = apiClient.getResponseInterceptor();
memberVarAsyncResponseInterceptor = apiClient.getAsyncResponseInterceptor();
}
protected ApiException getApiException(String operationId, HttpResponse response) throws IOException {
String body = response.body() == null ? null : new String(response.body().readAllBytes());
String message = formatExceptionMessage(operationId, response.statusCode(), body);
return new ApiException(response.statusCode(), message, response.headers(), body);
}
protected String formatExceptionMessage(String operationId, int statusCode, String body) {
if (body == null || body.isEmpty()) {
body = "[no body]";
}
return operationId + " call failed with: " + statusCode + " - " + body;
}
/**
*
* Creates a new Model API with a single Model API version.
* @param modelApiCreationRequest (required)
* @return ModelApi
* @throws ApiException if fails to make API call
*/
public ModelApi createModelApi(ModelApiCreationRequest modelApiCreationRequest) throws ApiException {
ApiResponse localVarResponse = createModelApiWithHttpInfo(modelApiCreationRequest);
return localVarResponse.getData();
}
/**
*
* Creates a new Model API with a single Model API version.
* @param modelApiCreationRequest (required)
* @return ApiResponse<ModelApi>
* @throws ApiException if fails to make API call
*/
public ApiResponse createModelApiWithHttpInfo(ModelApiCreationRequest modelApiCreationRequest) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = createModelApiRequestBuilder(modelApiCreationRequest);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("createModelApi", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder createModelApiRequestBuilder(ModelApiCreationRequest modelApiCreationRequest) throws ApiException {
// verify the required parameter 'modelApiCreationRequest' is set
if (modelApiCreationRequest == null) {
throw new ApiException(400, "Missing the required parameter 'modelApiCreationRequest' when calling createModelApi");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/modelServing/v1/modelApis";
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(modelApiCreationRequest);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
*
* Creates an access token for a Model API.
* @param modelApiId The id of the Model API to create the access token for. (required)
* @param modelApiAccessTokenCreationRequest (required)
* @return ModelApiAccessToken
* @throws ApiException if fails to make API call
*/
public ModelApiAccessToken createModelApiAccessToken(String modelApiId, ModelApiAccessTokenCreationRequest modelApiAccessTokenCreationRequest) throws ApiException {
ApiResponse localVarResponse = createModelApiAccessTokenWithHttpInfo(modelApiId, modelApiAccessTokenCreationRequest);
return localVarResponse.getData();
}
/**
*
* Creates an access token for a Model API.
* @param modelApiId The id of the Model API to create the access token for. (required)
* @param modelApiAccessTokenCreationRequest (required)
* @return ApiResponse<ModelApiAccessToken>
* @throws ApiException if fails to make API call
*/
public ApiResponse createModelApiAccessTokenWithHttpInfo(String modelApiId, ModelApiAccessTokenCreationRequest modelApiAccessTokenCreationRequest) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = createModelApiAccessTokenRequestBuilder(modelApiId, modelApiAccessTokenCreationRequest);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("createModelApiAccessToken", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder createModelApiAccessTokenRequestBuilder(String modelApiId, ModelApiAccessTokenCreationRequest modelApiAccessTokenCreationRequest) throws ApiException {
// verify the required parameter 'modelApiId' is set
if (modelApiId == null) {
throw new ApiException(400, "Missing the required parameter 'modelApiId' when calling createModelApiAccessToken");
}
// verify the required parameter 'modelApiAccessTokenCreationRequest' is set
if (modelApiAccessTokenCreationRequest == null) {
throw new ApiException(400, "Missing the required parameter 'modelApiAccessTokenCreationRequest' when calling createModelApiAccessToken");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/modelServing/v1/modelApis/{modelApiId}/accessTokens"
.replace("{modelApiId}", ApiClient.urlEncode(modelApiId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(modelApiAccessTokenCreationRequest);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
*
* Adds a collaborator to a Model API.
* @param modelApiId The id of the Model API to add the collaborator to. (required)
* @param modelApiCollaboratorRole (required)
* @return ModelApiCollaboratorRole
* @throws ApiException if fails to make API call
*/
public ModelApiCollaboratorRole createModelApiCollaborator(String modelApiId, ModelApiCollaboratorRole modelApiCollaboratorRole) throws ApiException {
ApiResponse localVarResponse = createModelApiCollaboratorWithHttpInfo(modelApiId, modelApiCollaboratorRole);
return localVarResponse.getData();
}
/**
*
* Adds a collaborator to a Model API.
* @param modelApiId The id of the Model API to add the collaborator to. (required)
* @param modelApiCollaboratorRole (required)
* @return ApiResponse<ModelApiCollaboratorRole>
* @throws ApiException if fails to make API call
*/
public ApiResponse createModelApiCollaboratorWithHttpInfo(String modelApiId, ModelApiCollaboratorRole modelApiCollaboratorRole) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = createModelApiCollaboratorRequestBuilder(modelApiId, modelApiCollaboratorRole);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("createModelApiCollaborator", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder createModelApiCollaboratorRequestBuilder(String modelApiId, ModelApiCollaboratorRole modelApiCollaboratorRole) throws ApiException {
// verify the required parameter 'modelApiId' is set
if (modelApiId == null) {
throw new ApiException(400, "Missing the required parameter 'modelApiId' when calling createModelApiCollaborator");
}
// verify the required parameter 'modelApiCollaboratorRole' is set
if (modelApiCollaboratorRole == null) {
throw new ApiException(400, "Missing the required parameter 'modelApiCollaboratorRole' when calling createModelApiCollaborator");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/modelServing/v1/modelApis/{modelApiId}/collaborators"
.replace("{modelApiId}", ApiClient.urlEncode(modelApiId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(modelApiCollaboratorRole);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
*
* Creates a volume for a Model API.
* @param modelApiId The id of the Model API to create the volume for. (required)
* @param modelApiVolume (required)
* @return ModelApiVolume
* @throws ApiException if fails to make API call
*/
public ModelApiVolume createModelApiVolume(String modelApiId, ModelApiVolume modelApiVolume) throws ApiException {
ApiResponse localVarResponse = createModelApiVolumeWithHttpInfo(modelApiId, modelApiVolume);
return localVarResponse.getData();
}
/**
*
* Creates a volume for a Model API.
* @param modelApiId The id of the Model API to create the volume for. (required)
* @param modelApiVolume (required)
* @return ApiResponse<ModelApiVolume>
* @throws ApiException if fails to make API call
*/
public ApiResponse createModelApiVolumeWithHttpInfo(String modelApiId, ModelApiVolume modelApiVolume) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = createModelApiVolumeRequestBuilder(modelApiId, modelApiVolume);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("createModelApiVolume", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder createModelApiVolumeRequestBuilder(String modelApiId, ModelApiVolume modelApiVolume) throws ApiException {
// verify the required parameter 'modelApiId' is set
if (modelApiId == null) {
throw new ApiException(400, "Missing the required parameter 'modelApiId' when calling createModelApiVolume");
}
// verify the required parameter 'modelApiVolume' is set
if (modelApiVolume == null) {
throw new ApiException(400, "Missing the required parameter 'modelApiVolume' when calling createModelApiVolume");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/modelServing/v1/modelApis/{modelApiId}/volumes"
.replace("{modelApiId}", ApiClient.urlEncode(modelApiId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(modelApiVolume);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
*
* Archives a Model API.
* @param modelApiId The id of the Model API to archive. (required)
* @throws ApiException if fails to make API call
*/
public void deleteModelApi(String modelApiId) throws ApiException {
deleteModelApiWithHttpInfo(modelApiId);
}
/**
*
* Archives a Model API.
* @param modelApiId The id of the Model API to archive. (required)
* @return ApiResponse<Void>
* @throws ApiException if fails to make API call
*/
public ApiResponse deleteModelApiWithHttpInfo(String modelApiId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = deleteModelApiRequestBuilder(modelApiId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("deleteModelApi", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
null
);
} finally {
// Drain the InputStream
while (localVarResponse.body().read() != -1) {
// Ignore
}
localVarResponse.body().close();
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder deleteModelApiRequestBuilder(String modelApiId) throws ApiException {
// verify the required parameter 'modelApiId' is set
if (modelApiId == null) {
throw new ApiException(400, "Missing the required parameter 'modelApiId' when calling deleteModelApi");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/modelServing/v1/modelApis/{modelApiId}"
.replace("{modelApiId}", ApiClient.urlEncode(modelApiId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("DELETE", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
*
*
* @param modelApiId The id of the Model API to delete the access token for. (required)
* @param accessTokenId The id of the access token to delete. (required)
* @throws ApiException if fails to make API call
*/
public void deleteModelApiAccessToken(String modelApiId, String accessTokenId) throws ApiException {
deleteModelApiAccessTokenWithHttpInfo(modelApiId, accessTokenId);
}
/**
*
*
* @param modelApiId The id of the Model API to delete the access token for. (required)
* @param accessTokenId The id of the access token to delete. (required)
* @return ApiResponse<Void>
* @throws ApiException if fails to make API call
*/
public ApiResponse deleteModelApiAccessTokenWithHttpInfo(String modelApiId, String accessTokenId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = deleteModelApiAccessTokenRequestBuilder(modelApiId, accessTokenId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("deleteModelApiAccessToken", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
null
);
} finally {
// Drain the InputStream
while (localVarResponse.body().read() != -1) {
// Ignore
}
localVarResponse.body().close();
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder deleteModelApiAccessTokenRequestBuilder(String modelApiId, String accessTokenId) throws ApiException {
// verify the required parameter 'modelApiId' is set
if (modelApiId == null) {
throw new ApiException(400, "Missing the required parameter 'modelApiId' when calling deleteModelApiAccessToken");
}
// verify the required parameter 'accessTokenId' is set
if (accessTokenId == null) {
throw new ApiException(400, "Missing the required parameter 'accessTokenId' when calling deleteModelApiAccessToken");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/modelServing/v1/modelApis/{modelApiId}/accessTokens/{accessTokenId}"
.replace("{modelApiId}", ApiClient.urlEncode(modelApiId.toString()))
.replace("{accessTokenId}", ApiClient.urlEncode(accessTokenId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("DELETE", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
*
* Deletes a Model API environment variable.
* @param modelApiId The id of the Model API to delete the environment variable from. (required)
* @param environmentVariableName The name of the environment variable to delete. (required)
* @throws ApiException if fails to make API call
*/
public void deleteModelApiEnvironmentVariable(String modelApiId, String environmentVariableName) throws ApiException {
deleteModelApiEnvironmentVariableWithHttpInfo(modelApiId, environmentVariableName);
}
/**
*
* Deletes a Model API environment variable.
* @param modelApiId The id of the Model API to delete the environment variable from. (required)
* @param environmentVariableName The name of the environment variable to delete. (required)
* @return ApiResponse<Void>
* @throws ApiException if fails to make API call
*/
public ApiResponse deleteModelApiEnvironmentVariableWithHttpInfo(String modelApiId, String environmentVariableName) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = deleteModelApiEnvironmentVariableRequestBuilder(modelApiId, environmentVariableName);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("deleteModelApiEnvironmentVariable", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
null
);
} finally {
// Drain the InputStream
while (localVarResponse.body().read() != -1) {
// Ignore
}
localVarResponse.body().close();
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder deleteModelApiEnvironmentVariableRequestBuilder(String modelApiId, String environmentVariableName) throws ApiException {
// verify the required parameter 'modelApiId' is set
if (modelApiId == null) {
throw new ApiException(400, "Missing the required parameter 'modelApiId' when calling deleteModelApiEnvironmentVariable");
}
// verify the required parameter 'environmentVariableName' is set
if (environmentVariableName == null) {
throw new ApiException(400, "Missing the required parameter 'environmentVariableName' when calling deleteModelApiEnvironmentVariable");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/modelServing/v1/modelApis/{modelApiId}/environmentVariables/{environmentVariableName}"
.replace("{modelApiId}", ApiClient.urlEncode(modelApiId.toString()))
.replace("{environmentVariableName}", ApiClient.urlEncode(environmentVariableName.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("DELETE", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
*
* Deletes a Model API volume.
* @param modelApiId The id of the Model API to delete the volume for. (required)
* @param volumeName The name of the volume to delete. (required)
* @throws ApiException if fails to make API call
*/
public void deleteModelApiVolume(String modelApiId, String volumeName) throws ApiException {
deleteModelApiVolumeWithHttpInfo(modelApiId, volumeName);
}
/**
*
* Deletes a Model API volume.
* @param modelApiId The id of the Model API to delete the volume for. (required)
* @param volumeName The name of the volume to delete. (required)
* @return ApiResponse<Void>
* @throws ApiException if fails to make API call
*/
public ApiResponse deleteModelApiVolumeWithHttpInfo(String modelApiId, String volumeName) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = deleteModelApiVolumeRequestBuilder(modelApiId, volumeName);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("deleteModelApiVolume", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
null
);
} finally {
// Drain the InputStream
while (localVarResponse.body().read() != -1) {
// Ignore
}
localVarResponse.body().close();
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder deleteModelApiVolumeRequestBuilder(String modelApiId, String volumeName) throws ApiException {
// verify the required parameter 'modelApiId' is set
if (modelApiId == null) {
throw new ApiException(400, "Missing the required parameter 'modelApiId' when calling deleteModelApiVolume");
}
// verify the required parameter 'volumeName' is set
if (volumeName == null) {
throw new ApiException(400, "Missing the required parameter 'volumeName' when calling deleteModelApiVolume");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/modelServing/v1/modelApis/{modelApiId}/volumes/{volumeName}"
.replace("{modelApiId}", ApiClient.urlEncode(modelApiId.toString()))
.replace("{volumeName}", ApiClient.urlEncode(volumeName.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("DELETE", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
*
* Retrieves a single Model API.
* @param modelApiId The id of the Model API to retrieve. (required)
* @return ModelApi
* @throws ApiException if fails to make API call
*/
public ModelApi getModelApi(String modelApiId) throws ApiException {
ApiResponse localVarResponse = getModelApiWithHttpInfo(modelApiId);
return localVarResponse.getData();
}
/**
*
* Retrieves a single Model API.
* @param modelApiId The id of the Model API to retrieve. (required)
* @return ApiResponse<ModelApi>
* @throws ApiException if fails to make API call
*/
public ApiResponse getModelApiWithHttpInfo(String modelApiId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getModelApiRequestBuilder(modelApiId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getModelApi", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder getModelApiRequestBuilder(String modelApiId) throws ApiException {
// verify the required parameter 'modelApiId' is set
if (modelApiId == null) {
throw new ApiException(400, "Missing the required parameter 'modelApiId' when calling getModelApi");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/modelServing/v1/modelApis/{modelApiId}"
.replace("{modelApiId}", ApiClient.urlEncode(modelApiId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
*
*
* @param modelApiId The id of the Model API to retrieve the access token for. (required)
* @param accessTokenId The id of the access token to retrieve. (required)
* @return ModelApiAccessToken
* @throws ApiException if fails to make API call
*/
public ModelApiAccessToken getModelApiAccessToken(String modelApiId, String accessTokenId) throws ApiException {
ApiResponse localVarResponse = getModelApiAccessTokenWithHttpInfo(modelApiId, accessTokenId);
return localVarResponse.getData();
}
/**
*
*
* @param modelApiId The id of the Model API to retrieve the access token for. (required)
* @param accessTokenId The id of the access token to retrieve. (required)
* @return ApiResponse<ModelApiAccessToken>
* @throws ApiException if fails to make API call
*/
public ApiResponse getModelApiAccessTokenWithHttpInfo(String modelApiId, String accessTokenId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getModelApiAccessTokenRequestBuilder(modelApiId, accessTokenId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getModelApiAccessToken", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder getModelApiAccessTokenRequestBuilder(String modelApiId, String accessTokenId) throws ApiException {
// verify the required parameter 'modelApiId' is set
if (modelApiId == null) {
throw new ApiException(400, "Missing the required parameter 'modelApiId' when calling getModelApiAccessToken");
}
// verify the required parameter 'accessTokenId' is set
if (accessTokenId == null) {
throw new ApiException(400, "Missing the required parameter 'accessTokenId' when calling getModelApiAccessToken");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/modelServing/v1/modelApis/{modelApiId}/accessTokens/{accessTokenId}"
.replace("{modelApiId}", ApiClient.urlEncode(modelApiId.toString()))
.replace("{accessTokenId}", ApiClient.urlEncode(accessTokenId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
*
* Retrieves a single collaborator for a Model API.
* @param modelApiId The id of the Model API to retrieve the collaborator for. (required)
* @param collaborator The reference of the collaborator to retrieve. (required)
* @return ModelApiCollaboratorRole
* @throws ApiException if fails to make API call
*/
public ModelApiCollaboratorRole getModelApiCollaborator(String modelApiId, String collaborator) throws ApiException {
ApiResponse localVarResponse = getModelApiCollaboratorWithHttpInfo(modelApiId, collaborator);
return localVarResponse.getData();
}
/**
*
* Retrieves a single collaborator for a Model API.
* @param modelApiId The id of the Model API to retrieve the collaborator for. (required)
* @param collaborator The reference of the collaborator to retrieve. (required)
* @return ApiResponse<ModelApiCollaboratorRole>
* @throws ApiException if fails to make API call
*/
public ApiResponse getModelApiCollaboratorWithHttpInfo(String modelApiId, String collaborator) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getModelApiCollaboratorRequestBuilder(modelApiId, collaborator);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getModelApiCollaborator", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder getModelApiCollaboratorRequestBuilder(String modelApiId, String collaborator) throws ApiException {
// verify the required parameter 'modelApiId' is set
if (modelApiId == null) {
throw new ApiException(400, "Missing the required parameter 'modelApiId' when calling getModelApiCollaborator");
}
// verify the required parameter 'collaborator' is set
if (collaborator == null) {
throw new ApiException(400, "Missing the required parameter 'collaborator' when calling getModelApiCollaborator");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/modelServing/v1/modelApis/{modelApiId}/collaborators/{collaborator}"
.replace("{modelApiId}", ApiClient.urlEncode(modelApiId.toString()))
.replace("{collaborator}", ApiClient.urlEncode(collaborator.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
*
* Lists Model APIs based on the query filters. Returns only Model APIs visible to the requesting user.
* @param environmentId The id of the environment to filter the Model APIs by. (optional)
* @param projectId The id of the project to filter the Model APIs by. (optional)
* @param name The name of the Model APIs. Can be a regular expression. (optional)
* @param registeredModelName The name of the registered model that is the source of the Model API. (optional)
* @param registeredModelVersion The version of the registered model that is the source of the Model API. Can only be present along with registeredModelName. (optional)
* @param limit The number of result to retrieve. Defaults to 25. (optional)
* @param offset The offset from the first element to start retrieving from. (optional, default to 25)
* @param orderBy Field to order results by. Format is the name of the field optionally followed by a space and either ASC or DESC. If not specified defaults to ASC. (optional)
* @return ModelApiPaginatedList
* @throws ApiException if fails to make API call
*/
public ModelApiPaginatedList listModelAPIs(String environmentId, String projectId, String name, String registeredModelName, Integer registeredModelVersion, Integer limit, Integer offset, String orderBy) throws ApiException {
ApiResponse localVarResponse = listModelAPIsWithHttpInfo(environmentId, projectId, name, registeredModelName, registeredModelVersion, limit, offset, orderBy);
return localVarResponse.getData();
}
/**
*
* Lists Model APIs based on the query filters. Returns only Model APIs visible to the requesting user.
* @param environmentId The id of the environment to filter the Model APIs by. (optional)
* @param projectId The id of the project to filter the Model APIs by. (optional)
* @param name The name of the Model APIs. Can be a regular expression. (optional)
* @param registeredModelName The name of the registered model that is the source of the Model API. (optional)
* @param registeredModelVersion The version of the registered model that is the source of the Model API. Can only be present along with registeredModelName. (optional)
* @param limit The number of result to retrieve. Defaults to 25. (optional)
* @param offset The offset from the first element to start retrieving from. (optional, default to 25)
* @param orderBy Field to order results by. Format is the name of the field optionally followed by a space and either ASC or DESC. If not specified defaults to ASC. (optional)
* @return ApiResponse<ModelApiPaginatedList>
* @throws ApiException if fails to make API call
*/
public ApiResponse listModelAPIsWithHttpInfo(String environmentId, String projectId, String name, String registeredModelName, Integer registeredModelVersion, Integer limit, Integer offset, String orderBy) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = listModelAPIsRequestBuilder(environmentId, projectId, name, registeredModelName, registeredModelVersion, limit, offset, orderBy);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("listModelAPIs", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder listModelAPIsRequestBuilder(String environmentId, String projectId, String name, String registeredModelName, Integer registeredModelVersion, Integer limit, Integer offset, String orderBy) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/modelServing/v1/modelApis";
List localVarQueryParams = new ArrayList<>();
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
localVarQueryParameterBaseName = "environmentId";
localVarQueryParams.addAll(ApiClient.parameterToPairs("environmentId", environmentId));
localVarQueryParameterBaseName = "projectId";
localVarQueryParams.addAll(ApiClient.parameterToPairs("projectId", projectId));
localVarQueryParameterBaseName = "name";
localVarQueryParams.addAll(ApiClient.parameterToPairs("name", name));
localVarQueryParameterBaseName = "registeredModelName";
localVarQueryParams.addAll(ApiClient.parameterToPairs("registeredModelName", registeredModelName));
localVarQueryParameterBaseName = "registeredModelVersion";
localVarQueryParams.addAll(ApiClient.parameterToPairs("registeredModelVersion", registeredModelVersion));
localVarQueryParameterBaseName = "limit";
localVarQueryParams.addAll(ApiClient.parameterToPairs("limit", limit));
localVarQueryParameterBaseName = "offset";
localVarQueryParams.addAll(ApiClient.parameterToPairs("offset", offset));
localVarQueryParameterBaseName = "orderBy";
localVarQueryParams.addAll(ApiClient.parameterToPairs("orderBy", orderBy));
if (!localVarQueryParams.isEmpty() || localVarQueryStringJoiner.length() != 0) {
StringJoiner queryJoiner = new StringJoiner("&");
localVarQueryParams.forEach(p -> queryJoiner.add(p.getName() + '=' + p.getValue()));
if (localVarQueryStringJoiner.length() != 0) {
queryJoiner.add(localVarQueryStringJoiner.toString());
}
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath + '?' + queryJoiner.toString()));
} else {
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
}
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
*
* Lists the access tokens of a Model API.
* @param modelApiId The id of the Model API to list the access tokens for. (required)
* @return List<ModelApiAccessToken>
* @throws ApiException if fails to make API call
*/
public List listModelApiAccessTokens(String modelApiId) throws ApiException {
ApiResponse> localVarResponse = listModelApiAccessTokensWithHttpInfo(modelApiId);
return localVarResponse.getData();
}
/**
*
* Lists the access tokens of a Model API.
* @param modelApiId The id of the Model API to list the access tokens for. (required)
* @return ApiResponse<List<ModelApiAccessToken>>
* @throws ApiException if fails to make API call
*/
public ApiResponse> listModelApiAccessTokensWithHttpInfo(String modelApiId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = listModelApiAccessTokensRequestBuilder(modelApiId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("listModelApiAccessTokens", localVarResponse);
}
return new ApiResponse>(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference>() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder listModelApiAccessTokensRequestBuilder(String modelApiId) throws ApiException {
// verify the required parameter 'modelApiId' is set
if (modelApiId == null) {
throw new ApiException(400, "Missing the required parameter 'modelApiId' when calling listModelApiAccessTokens");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/modelServing/v1/modelApis/{modelApiId}/accessTokens"
.replace("{modelApiId}", ApiClient.urlEncode(modelApiId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
*
* Lists the collaborators of a Model API.
* @param modelApiId The id of the Model API to list the collaborators for. (required)
* @return List<ModelApiCollaboratorRole>
* @throws ApiException if fails to make API call
*/
public List listModelApiCollaborators(String modelApiId) throws ApiException {
ApiResponse> localVarResponse = listModelApiCollaboratorsWithHttpInfo(modelApiId);
return localVarResponse.getData();
}
/**
*
* Lists the collaborators of a Model API.
* @param modelApiId The id of the Model API to list the collaborators for. (required)
* @return ApiResponse<List<ModelApiCollaboratorRole>>
* @throws ApiException if fails to make API call
*/
public ApiResponse> listModelApiCollaboratorsWithHttpInfo(String modelApiId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = listModelApiCollaboratorsRequestBuilder(modelApiId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("listModelApiCollaborators", localVarResponse);
}
return new ApiResponse>(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference>() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder listModelApiCollaboratorsRequestBuilder(String modelApiId) throws ApiException {
// verify the required parameter 'modelApiId' is set
if (modelApiId == null) {
throw new ApiException(400, "Missing the required parameter 'modelApiId' when calling listModelApiCollaborators");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/modelServing/v1/modelApis/{modelApiId}/collaborators"
.replace("{modelApiId}", ApiClient.urlEncode(modelApiId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
*
* Lists the volumes of a Model API.
* @param modelApiId The id of the Model API to list the volumes for. (required)
* @return List<ModelApiVolume>
* @throws ApiException if fails to make API call
*/
public List listModelApiVolumes(String modelApiId) throws ApiException {
ApiResponse> localVarResponse = listModelApiVolumesWithHttpInfo(modelApiId);
return localVarResponse.getData();
}
/**
*
* Lists the volumes of a Model API.
* @param modelApiId The id of the Model API to list the volumes for. (required)
* @return ApiResponse<List<ModelApiVolume>>
* @throws ApiException if fails to make API call
*/
public ApiResponse> listModelApiVolumesWithHttpInfo(String modelApiId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = listModelApiVolumesRequestBuilder(modelApiId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("listModelApiVolumes", localVarResponse);
}
return new ApiResponse>(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference>() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder listModelApiVolumesRequestBuilder(String modelApiId) throws ApiException {
// verify the required parameter 'modelApiId' is set
if (modelApiId == null) {
throw new ApiException(400, "Missing the required parameter 'modelApiId' when calling listModelApiVolumes");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/modelServing/v1/modelApis/{modelApiId}/volumes"
.replace("{modelApiId}", ApiClient.urlEncode(modelApiId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
*
*
* @param modelApiId The id of the Model API to remove the collaborator from. (required)
* @param collaborator The reference of the collaborator to remove. (required)
* @throws ApiException if fails to make API call
*/
public void removeModelApiCollaborator(String modelApiId, String collaborator) throws ApiException {
removeModelApiCollaboratorWithHttpInfo(modelApiId, collaborator);
}
/**
*
*
* @param modelApiId The id of the Model API to remove the collaborator from. (required)
* @param collaborator The reference of the collaborator to remove. (required)
* @return ApiResponse<Void>
* @throws ApiException if fails to make API call
*/
public ApiResponse removeModelApiCollaboratorWithHttpInfo(String modelApiId, String collaborator) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = removeModelApiCollaboratorRequestBuilder(modelApiId, collaborator);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("removeModelApiCollaborator", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
null
);
} finally {
// Drain the InputStream
while (localVarResponse.body().read() != -1) {
// Ignore
}
localVarResponse.body().close();
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder removeModelApiCollaboratorRequestBuilder(String modelApiId, String collaborator) throws ApiException {
// verify the required parameter 'modelApiId' is set
if (modelApiId == null) {
throw new ApiException(400, "Missing the required parameter 'modelApiId' when calling removeModelApiCollaborator");
}
// verify the required parameter 'collaborator' is set
if (collaborator == null) {
throw new ApiException(400, "Missing the required parameter 'collaborator' when calling removeModelApiCollaborator");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/modelServing/v1/modelApis/{modelApiId}/collaborators/{collaborator}"
.replace("{modelApiId}", ApiClient.urlEncode(modelApiId.toString()))
.replace("{collaborator}", ApiClient.urlEncode(collaborator.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("DELETE", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
*
* Updates a Model API.
* @param modelApiId The id of the Model API to update. (required)
* @param modelApiUpdateRequest (required)
* @return ModelApi
* @throws ApiException if fails to make API call
*/
public ModelApi updateModelApi(String modelApiId, ModelApiUpdateRequest modelApiUpdateRequest) throws ApiException {
ApiResponse localVarResponse = updateModelApiWithHttpInfo(modelApiId, modelApiUpdateRequest);
return localVarResponse.getData();
}
/**
*
* Updates a Model API.
* @param modelApiId The id of the Model API to update. (required)
* @param modelApiUpdateRequest (required)
* @return ApiResponse<ModelApi>
* @throws ApiException if fails to make API call
*/
public ApiResponse updateModelApiWithHttpInfo(String modelApiId, ModelApiUpdateRequest modelApiUpdateRequest) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = updateModelApiRequestBuilder(modelApiId, modelApiUpdateRequest);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("updateModelApi", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder updateModelApiRequestBuilder(String modelApiId, ModelApiUpdateRequest modelApiUpdateRequest) throws ApiException {
// verify the required parameter 'modelApiId' is set
if (modelApiId == null) {
throw new ApiException(400, "Missing the required parameter 'modelApiId' when calling updateModelApi");
}
// verify the required parameter 'modelApiUpdateRequest' is set
if (modelApiUpdateRequest == null) {
throw new ApiException(400, "Missing the required parameter 'modelApiUpdateRequest' when calling updateModelApi");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/modelServing/v1/modelApis/{modelApiId}"
.replace("{modelApiId}", ApiClient.urlEncode(modelApiId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(modelApiUpdateRequest);
localVarRequestBuilder.method("PUT", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
*
* Updates a Model API access token.
* @param modelApiId The id of the Model API to update the access token for. (required)
* @param accessTokenId The id of the access token to update. (required)
* @param modelApiAccessTokenCreationRequest (required)
* @return ModelApiAccessToken
* @throws ApiException if fails to make API call
*/
public ModelApiAccessToken updateModelApiAccessToken(String modelApiId, String accessTokenId, ModelApiAccessTokenCreationRequest modelApiAccessTokenCreationRequest) throws ApiException {
ApiResponse localVarResponse = updateModelApiAccessTokenWithHttpInfo(modelApiId, accessTokenId, modelApiAccessTokenCreationRequest);
return localVarResponse.getData();
}
/**
*
* Updates a Model API access token.
* @param modelApiId The id of the Model API to update the access token for. (required)
* @param accessTokenId The id of the access token to update. (required)
* @param modelApiAccessTokenCreationRequest (required)
* @return ApiResponse<ModelApiAccessToken>
* @throws ApiException if fails to make API call
*/
public ApiResponse updateModelApiAccessTokenWithHttpInfo(String modelApiId, String accessTokenId, ModelApiAccessTokenCreationRequest modelApiAccessTokenCreationRequest) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = updateModelApiAccessTokenRequestBuilder(modelApiId, accessTokenId, modelApiAccessTokenCreationRequest);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("updateModelApiAccessToken", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder updateModelApiAccessTokenRequestBuilder(String modelApiId, String accessTokenId, ModelApiAccessTokenCreationRequest modelApiAccessTokenCreationRequest) throws ApiException {
// verify the required parameter 'modelApiId' is set
if (modelApiId == null) {
throw new ApiException(400, "Missing the required parameter 'modelApiId' when calling updateModelApiAccessToken");
}
// verify the required parameter 'accessTokenId' is set
if (accessTokenId == null) {
throw new ApiException(400, "Missing the required parameter 'accessTokenId' when calling updateModelApiAccessToken");
}
// verify the required parameter 'modelApiAccessTokenCreationRequest' is set
if (modelApiAccessTokenCreationRequest == null) {
throw new ApiException(400, "Missing the required parameter 'modelApiAccessTokenCreationRequest' when calling updateModelApiAccessToken");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/modelServing/v1/modelApis/{modelApiId}/accessTokens/{accessTokenId}"
.replace("{modelApiId}", ApiClient.urlEncode(modelApiId.toString()))
.replace("{accessTokenId}", ApiClient.urlEncode(accessTokenId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(modelApiAccessTokenCreationRequest);
localVarRequestBuilder.method("PUT", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
*
* Updates a Model API collaborator.
* @param modelApiId The id of the Model API to update the collaborator for. (required)
* @param collaborator The reference of the collaborator to update. (required)
* @param modelApiCollaboratorRole (required)
* @return ModelApiCollaboratorRole
* @throws ApiException if fails to make API call
*/
public ModelApiCollaboratorRole updateModelApiCollaborator(String modelApiId, String collaborator, ModelApiCollaboratorRole modelApiCollaboratorRole) throws ApiException {
ApiResponse localVarResponse = updateModelApiCollaboratorWithHttpInfo(modelApiId, collaborator, modelApiCollaboratorRole);
return localVarResponse.getData();
}
/**
*
* Updates a Model API collaborator.
* @param modelApiId The id of the Model API to update the collaborator for. (required)
* @param collaborator The reference of the collaborator to update. (required)
* @param modelApiCollaboratorRole (required)
* @return ApiResponse<ModelApiCollaboratorRole>
* @throws ApiException if fails to make API call
*/
public ApiResponse updateModelApiCollaboratorWithHttpInfo(String modelApiId, String collaborator, ModelApiCollaboratorRole modelApiCollaboratorRole) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = updateModelApiCollaboratorRequestBuilder(modelApiId, collaborator, modelApiCollaboratorRole);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("updateModelApiCollaborator", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
protected HttpRequest.Builder updateModelApiCollaboratorRequestBuilder(String modelApiId, String collaborator, ModelApiCollaboratorRole modelApiCollaboratorRole) throws ApiException {
// verify the required parameter 'modelApiId' is set
if (modelApiId == null) {
throw new ApiException(400, "Missing the required parameter 'modelApiId' when calling updateModelApiCollaborator");
}
// verify the required parameter 'collaborator' is set
if (collaborator == null) {
throw new ApiException(400, "Missing the required parameter 'collaborator' when calling updateModelApiCollaborator");
}
// verify the required parameter 'modelApiCollaboratorRole' is set
if (modelApiCollaboratorRole == null) {
throw new ApiException(400, "Missing the required parameter 'modelApiCollaboratorRole' when calling updateModelApiCollaborator");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/api/modelServing/v1/modelApis/{modelApiId}/collaborators/{collaborator}"
.replace("{modelApiId}", ApiClient.urlEncode(modelApiId.toString()))
.replace("{collaborator}", ApiClient.urlEncode(collaborator.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(modelApiCollaboratorRole);
localVarRequestBuilder.method("PUT", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy