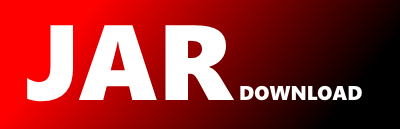
com.dominodatalab.api.model.DominoAdminInterfaceExecutionOverview Maven / Gradle / Ivy
/*
* Domino Data Lab API v4
* This API is going to provide access to all the Domino functions available in the user interface. To authenticate your requests, include your API Key (which you can find on your account page) with the header X-Domino-Api-Key.
*
* The version of the OpenAPI document: 4.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.api.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.dominodatalab.api.model.DominoAdminInterfaceComputeClusterOverview;
import com.dominodatalab.api.model.DominoAdminInterfaceHardwareTierOverview;
import com.dominodatalab.api.model.DominoAdminInterfaceOnDemandSparkClusterOverview;
import com.dominodatalab.api.model.DominoAdminInterfaceOnDemandSparkExecutionUnitOverview;
import com.dominodatalab.api.model.DominoCommonProjectId;
import com.dominodatalab.api.model.DominoComputegridExecutionUnitOverview;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* DominoAdminInterfaceExecutionOverview
*/
@JsonPropertyOrder({
DominoAdminInterfaceExecutionOverview.JSON_PROPERTY_ID,
DominoAdminInterfaceExecutionOverview.JSON_PROPERTY_COMPUTE_GRID_TYPE,
DominoAdminInterfaceExecutionOverview.JSON_PROPERTY_PROJECT_ID,
DominoAdminInterfaceExecutionOverview.JSON_PROPERTY_PROJECT_IDENTIFIER,
DominoAdminInterfaceExecutionOverview.JSON_PROPERTY_STARTING_USER_ID,
DominoAdminInterfaceExecutionOverview.JSON_PROPERTY_STARTING_USERNAME,
DominoAdminInterfaceExecutionOverview.JSON_PROPERTY_HARDWARE_TIER,
DominoAdminInterfaceExecutionOverview.JSON_PROPERTY_STATUS,
DominoAdminInterfaceExecutionOverview.JSON_PROPERTY_EXECUTION_UNITS,
DominoAdminInterfaceExecutionOverview.JSON_PROPERTY_CREATED,
DominoAdminInterfaceExecutionOverview.JSON_PROPERTY_TITLE,
DominoAdminInterfaceExecutionOverview.JSON_PROPERTY_WORKLOAD_TYPE,
DominoAdminInterfaceExecutionOverview.JSON_PROPERTY_COMPUTE_CLUSTER_OVERVIEWS,
DominoAdminInterfaceExecutionOverview.JSON_PROPERTY_ON_DEMAND_SPARK_CLUSTER_PROPERTIES,
DominoAdminInterfaceExecutionOverview.JSON_PROPERTY_ON_DEMAND_SPARK_EXECUTION_UNITS
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2023-10-17T15:20:46.682098100-04:00[America/New_York]")
public class DominoAdminInterfaceExecutionOverview {
public static final String JSON_PROPERTY_ID = "id";
private String id;
/**
* Gets or Sets computeGridType
*/
public enum ComputeGridTypeEnum {
CLASSICONPREMISES("ClassicOnPremises"),
CLASSICAWS("ClassicAWS"),
KUBERNETES("Kubernetes");
private String value;
ComputeGridTypeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static ComputeGridTypeEnum fromValue(String value) {
for (ComputeGridTypeEnum b : ComputeGridTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_COMPUTE_GRID_TYPE = "computeGridType";
private ComputeGridTypeEnum computeGridType;
public static final String JSON_PROPERTY_PROJECT_ID = "projectId";
private String projectId;
public static final String JSON_PROPERTY_PROJECT_IDENTIFIER = "projectIdentifier";
private DominoCommonProjectId projectIdentifier;
public static final String JSON_PROPERTY_STARTING_USER_ID = "startingUserId";
private String startingUserId;
public static final String JSON_PROPERTY_STARTING_USERNAME = "startingUsername";
private String startingUsername;
public static final String JSON_PROPERTY_HARDWARE_TIER = "hardwareTier";
private DominoAdminInterfaceHardwareTierOverview hardwareTier;
public static final String JSON_PROPERTY_STATUS = "status";
private String status;
public static final String JSON_PROPERTY_EXECUTION_UNITS = "executionUnits";
private List executionUnits = new ArrayList<>();
public static final String JSON_PROPERTY_CREATED = "created";
private OffsetDateTime created;
public static final String JSON_PROPERTY_TITLE = "title";
private String title;
public static final String JSON_PROPERTY_WORKLOAD_TYPE = "workloadType";
private String workloadType;
public static final String JSON_PROPERTY_COMPUTE_CLUSTER_OVERVIEWS = "computeClusterOverviews";
private List computeClusterOverviews = new ArrayList<>();
public static final String JSON_PROPERTY_ON_DEMAND_SPARK_CLUSTER_PROPERTIES = "onDemandSparkClusterProperties";
private DominoAdminInterfaceOnDemandSparkClusterOverview onDemandSparkClusterProperties;
public static final String JSON_PROPERTY_ON_DEMAND_SPARK_EXECUTION_UNITS = "onDemandSparkExecutionUnits";
private List onDemandSparkExecutionUnits = new ArrayList<>();
public DominoAdminInterfaceExecutionOverview() {
}
public DominoAdminInterfaceExecutionOverview id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getId() {
return id;
}
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setId(String id) {
this.id = id;
}
public DominoAdminInterfaceExecutionOverview computeGridType(ComputeGridTypeEnum computeGridType) {
this.computeGridType = computeGridType;
return this;
}
/**
* Get computeGridType
* @return computeGridType
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_COMPUTE_GRID_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public ComputeGridTypeEnum getComputeGridType() {
return computeGridType;
}
@JsonProperty(JSON_PROPERTY_COMPUTE_GRID_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setComputeGridType(ComputeGridTypeEnum computeGridType) {
this.computeGridType = computeGridType;
}
public DominoAdminInterfaceExecutionOverview projectId(String projectId) {
this.projectId = projectId;
return this;
}
/**
* Get projectId
* @return projectId
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_PROJECT_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getProjectId() {
return projectId;
}
@JsonProperty(JSON_PROPERTY_PROJECT_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setProjectId(String projectId) {
this.projectId = projectId;
}
public DominoAdminInterfaceExecutionOverview projectIdentifier(DominoCommonProjectId projectIdentifier) {
this.projectIdentifier = projectIdentifier;
return this;
}
/**
* Get projectIdentifier
* @return projectIdentifier
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_PROJECT_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public DominoCommonProjectId getProjectIdentifier() {
return projectIdentifier;
}
@JsonProperty(JSON_PROPERTY_PROJECT_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setProjectIdentifier(DominoCommonProjectId projectIdentifier) {
this.projectIdentifier = projectIdentifier;
}
public DominoAdminInterfaceExecutionOverview startingUserId(String startingUserId) {
this.startingUserId = startingUserId;
return this;
}
/**
* Get startingUserId
* @return startingUserId
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_STARTING_USER_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getStartingUserId() {
return startingUserId;
}
@JsonProperty(JSON_PROPERTY_STARTING_USER_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setStartingUserId(String startingUserId) {
this.startingUserId = startingUserId;
}
public DominoAdminInterfaceExecutionOverview startingUsername(String startingUsername) {
this.startingUsername = startingUsername;
return this;
}
/**
* Get startingUsername
* @return startingUsername
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_STARTING_USERNAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getStartingUsername() {
return startingUsername;
}
@JsonProperty(JSON_PROPERTY_STARTING_USERNAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setStartingUsername(String startingUsername) {
this.startingUsername = startingUsername;
}
public DominoAdminInterfaceExecutionOverview hardwareTier(DominoAdminInterfaceHardwareTierOverview hardwareTier) {
this.hardwareTier = hardwareTier;
return this;
}
/**
* Get hardwareTier
* @return hardwareTier
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_HARDWARE_TIER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public DominoAdminInterfaceHardwareTierOverview getHardwareTier() {
return hardwareTier;
}
@JsonProperty(JSON_PROPERTY_HARDWARE_TIER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setHardwareTier(DominoAdminInterfaceHardwareTierOverview hardwareTier) {
this.hardwareTier = hardwareTier;
}
public DominoAdminInterfaceExecutionOverview status(String status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getStatus() {
return status;
}
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setStatus(String status) {
this.status = status;
}
public DominoAdminInterfaceExecutionOverview executionUnits(List executionUnits) {
this.executionUnits = executionUnits;
return this;
}
public DominoAdminInterfaceExecutionOverview addExecutionUnitsItem(DominoComputegridExecutionUnitOverview executionUnitsItem) {
if (this.executionUnits == null) {
this.executionUnits = new ArrayList<>();
}
this.executionUnits.add(executionUnitsItem);
return this;
}
/**
* Get executionUnits
* @return executionUnits
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_EXECUTION_UNITS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getExecutionUnits() {
return executionUnits;
}
@JsonProperty(JSON_PROPERTY_EXECUTION_UNITS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setExecutionUnits(List executionUnits) {
this.executionUnits = executionUnits;
}
public DominoAdminInterfaceExecutionOverview created(OffsetDateTime created) {
this.created = created;
return this;
}
/**
* Get created
* @return created
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_CREATED)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public OffsetDateTime getCreated() {
return created;
}
@JsonProperty(JSON_PROPERTY_CREATED)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setCreated(OffsetDateTime created) {
this.created = created;
}
public DominoAdminInterfaceExecutionOverview title(String title) {
this.title = title;
return this;
}
/**
* Get title
* @return title
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_TITLE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getTitle() {
return title;
}
@JsonProperty(JSON_PROPERTY_TITLE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTitle(String title) {
this.title = title;
}
public DominoAdminInterfaceExecutionOverview workloadType(String workloadType) {
this.workloadType = workloadType;
return this;
}
/**
* Get workloadType
* @return workloadType
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_WORKLOAD_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getWorkloadType() {
return workloadType;
}
@JsonProperty(JSON_PROPERTY_WORKLOAD_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setWorkloadType(String workloadType) {
this.workloadType = workloadType;
}
public DominoAdminInterfaceExecutionOverview computeClusterOverviews(List computeClusterOverviews) {
this.computeClusterOverviews = computeClusterOverviews;
return this;
}
public DominoAdminInterfaceExecutionOverview addComputeClusterOverviewsItem(DominoAdminInterfaceComputeClusterOverview computeClusterOverviewsItem) {
if (this.computeClusterOverviews == null) {
this.computeClusterOverviews = new ArrayList<>();
}
this.computeClusterOverviews.add(computeClusterOverviewsItem);
return this;
}
/**
* Get computeClusterOverviews
* @return computeClusterOverviews
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_COMPUTE_CLUSTER_OVERVIEWS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getComputeClusterOverviews() {
return computeClusterOverviews;
}
@JsonProperty(JSON_PROPERTY_COMPUTE_CLUSTER_OVERVIEWS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setComputeClusterOverviews(List computeClusterOverviews) {
this.computeClusterOverviews = computeClusterOverviews;
}
public DominoAdminInterfaceExecutionOverview onDemandSparkClusterProperties(DominoAdminInterfaceOnDemandSparkClusterOverview onDemandSparkClusterProperties) {
this.onDemandSparkClusterProperties = onDemandSparkClusterProperties;
return this;
}
/**
* Get onDemandSparkClusterProperties
* @return onDemandSparkClusterProperties
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_ON_DEMAND_SPARK_CLUSTER_PROPERTIES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DominoAdminInterfaceOnDemandSparkClusterOverview getOnDemandSparkClusterProperties() {
return onDemandSparkClusterProperties;
}
@JsonProperty(JSON_PROPERTY_ON_DEMAND_SPARK_CLUSTER_PROPERTIES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setOnDemandSparkClusterProperties(DominoAdminInterfaceOnDemandSparkClusterOverview onDemandSparkClusterProperties) {
this.onDemandSparkClusterProperties = onDemandSparkClusterProperties;
}
public DominoAdminInterfaceExecutionOverview onDemandSparkExecutionUnits(List onDemandSparkExecutionUnits) {
this.onDemandSparkExecutionUnits = onDemandSparkExecutionUnits;
return this;
}
public DominoAdminInterfaceExecutionOverview addOnDemandSparkExecutionUnitsItem(DominoAdminInterfaceOnDemandSparkExecutionUnitOverview onDemandSparkExecutionUnitsItem) {
if (this.onDemandSparkExecutionUnits == null) {
this.onDemandSparkExecutionUnits = new ArrayList<>();
}
this.onDemandSparkExecutionUnits.add(onDemandSparkExecutionUnitsItem);
return this;
}
/**
* Get onDemandSparkExecutionUnits
* @return onDemandSparkExecutionUnits
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ON_DEMAND_SPARK_EXECUTION_UNITS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getOnDemandSparkExecutionUnits() {
return onDemandSparkExecutionUnits;
}
@JsonProperty(JSON_PROPERTY_ON_DEMAND_SPARK_EXECUTION_UNITS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setOnDemandSparkExecutionUnits(List onDemandSparkExecutionUnits) {
this.onDemandSparkExecutionUnits = onDemandSparkExecutionUnits;
}
/**
* Return true if this domino.admin.interface.ExecutionOverview object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DominoAdminInterfaceExecutionOverview dominoAdminInterfaceExecutionOverview = (DominoAdminInterfaceExecutionOverview) o;
return Objects.equals(this.id, dominoAdminInterfaceExecutionOverview.id) &&
Objects.equals(this.computeGridType, dominoAdminInterfaceExecutionOverview.computeGridType) &&
Objects.equals(this.projectId, dominoAdminInterfaceExecutionOverview.projectId) &&
Objects.equals(this.projectIdentifier, dominoAdminInterfaceExecutionOverview.projectIdentifier) &&
Objects.equals(this.startingUserId, dominoAdminInterfaceExecutionOverview.startingUserId) &&
Objects.equals(this.startingUsername, dominoAdminInterfaceExecutionOverview.startingUsername) &&
Objects.equals(this.hardwareTier, dominoAdminInterfaceExecutionOverview.hardwareTier) &&
Objects.equals(this.status, dominoAdminInterfaceExecutionOverview.status) &&
Objects.equals(this.executionUnits, dominoAdminInterfaceExecutionOverview.executionUnits) &&
Objects.equals(this.created, dominoAdminInterfaceExecutionOverview.created) &&
Objects.equals(this.title, dominoAdminInterfaceExecutionOverview.title) &&
Objects.equals(this.workloadType, dominoAdminInterfaceExecutionOverview.workloadType) &&
Objects.equals(this.computeClusterOverviews, dominoAdminInterfaceExecutionOverview.computeClusterOverviews) &&
Objects.equals(this.onDemandSparkClusterProperties, dominoAdminInterfaceExecutionOverview.onDemandSparkClusterProperties) &&
Objects.equals(this.onDemandSparkExecutionUnits, dominoAdminInterfaceExecutionOverview.onDemandSparkExecutionUnits);
}
@Override
public int hashCode() {
return Objects.hash(id, computeGridType, projectId, projectIdentifier, startingUserId, startingUsername, hardwareTier, status, executionUnits, created, title, workloadType, computeClusterOverviews, onDemandSparkClusterProperties, onDemandSparkExecutionUnits);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DominoAdminInterfaceExecutionOverview {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" computeGridType: ").append(toIndentedString(computeGridType)).append("\n");
sb.append(" projectId: ").append(toIndentedString(projectId)).append("\n");
sb.append(" projectIdentifier: ").append(toIndentedString(projectIdentifier)).append("\n");
sb.append(" startingUserId: ").append(toIndentedString(startingUserId)).append("\n");
sb.append(" startingUsername: ").append(toIndentedString(startingUsername)).append("\n");
sb.append(" hardwareTier: ").append(toIndentedString(hardwareTier)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" executionUnits: ").append(toIndentedString(executionUnits)).append("\n");
sb.append(" created: ").append(toIndentedString(created)).append("\n");
sb.append(" title: ").append(toIndentedString(title)).append("\n");
sb.append(" workloadType: ").append(toIndentedString(workloadType)).append("\n");
sb.append(" computeClusterOverviews: ").append(toIndentedString(computeClusterOverviews)).append("\n");
sb.append(" onDemandSparkClusterProperties: ").append(toIndentedString(onDemandSparkClusterProperties)).append("\n");
sb.append(" onDemandSparkExecutionUnits: ").append(toIndentedString(onDemandSparkExecutionUnits)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `id` to the URL query string
if (getId() != null) {
joiner.add(String.format("%sid%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `computeGridType` to the URL query string
if (getComputeGridType() != null) {
joiner.add(String.format("%scomputeGridType%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getComputeGridType()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `projectId` to the URL query string
if (getProjectId() != null) {
joiner.add(String.format("%sprojectId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getProjectId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `projectIdentifier` to the URL query string
if (getProjectIdentifier() != null) {
joiner.add(getProjectIdentifier().toUrlQueryString(prefix + "projectIdentifier" + suffix));
}
// add `startingUserId` to the URL query string
if (getStartingUserId() != null) {
joiner.add(String.format("%sstartingUserId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getStartingUserId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `startingUsername` to the URL query string
if (getStartingUsername() != null) {
joiner.add(String.format("%sstartingUsername%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getStartingUsername()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `hardwareTier` to the URL query string
if (getHardwareTier() != null) {
joiner.add(getHardwareTier().toUrlQueryString(prefix + "hardwareTier" + suffix));
}
// add `status` to the URL query string
if (getStatus() != null) {
joiner.add(String.format("%sstatus%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getStatus()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `executionUnits` to the URL query string
if (getExecutionUnits() != null) {
for (int i = 0; i < getExecutionUnits().size(); i++) {
if (getExecutionUnits().get(i) != null) {
joiner.add(getExecutionUnits().get(i).toUrlQueryString(String.format("%sexecutionUnits%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `created` to the URL query string
if (getCreated() != null) {
joiner.add(String.format("%screated%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getCreated()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `title` to the URL query string
if (getTitle() != null) {
joiner.add(String.format("%stitle%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getTitle()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `workloadType` to the URL query string
if (getWorkloadType() != null) {
joiner.add(String.format("%sworkloadType%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getWorkloadType()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `computeClusterOverviews` to the URL query string
if (getComputeClusterOverviews() != null) {
for (int i = 0; i < getComputeClusterOverviews().size(); i++) {
if (getComputeClusterOverviews().get(i) != null) {
joiner.add(getComputeClusterOverviews().get(i).toUrlQueryString(String.format("%scomputeClusterOverviews%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `onDemandSparkClusterProperties` to the URL query string
if (getOnDemandSparkClusterProperties() != null) {
joiner.add(getOnDemandSparkClusterProperties().toUrlQueryString(prefix + "onDemandSparkClusterProperties" + suffix));
}
// add `onDemandSparkExecutionUnits` to the URL query string
if (getOnDemandSparkExecutionUnits() != null) {
for (int i = 0; i < getOnDemandSparkExecutionUnits().size(); i++) {
if (getOnDemandSparkExecutionUnits().get(i) != null) {
joiner.add(getOnDemandSparkExecutionUnits().get(i).toUrlQueryString(String.format("%sonDemandSparkExecutionUnits%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy