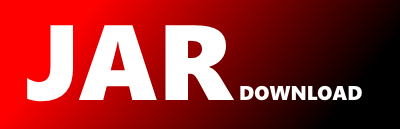
com.dominodatalab.api.model.DominoComputegridExecutionEventDto Maven / Gradle / Ivy
/*
* Domino Data Lab API v4
* This API is going to provide access to all the Domino functions available in the user interface. To authenticate your requests, include your API Key (which you can find on your account page) with the header X-Domino-Api-Key.
*
* The version of the OpenAPI document: 4.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.api.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.time.OffsetDateTime;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* DominoComputegridExecutionEventDto
*/
@JsonPropertyOrder({
DominoComputegridExecutionEventDto.JSON_PROPERTY_REASON,
DominoComputegridExecutionEventDto.JSON_PROPERTY_METRIC_NAME,
DominoComputegridExecutionEventDto.JSON_PROPERTY_CREATION_TIME,
DominoComputegridExecutionEventDto.JSON_PROPERTY_EXECUTION_TYPE,
DominoComputegridExecutionEventDto.JSON_PROPERTY_DESCRIPTION,
DominoComputegridExecutionEventDto.JSON_PROPERTY_SOURCE,
DominoComputegridExecutionEventDto.JSON_PROPERTY_ATTEMPT,
DominoComputegridExecutionEventDto.JSON_PROPERTY_RESOURCE_KIND,
DominoComputegridExecutionEventDto.JSON_PROPERTY_PAYLOAD,
DominoComputegridExecutionEventDto.JSON_PROPERTY_ID,
DominoComputegridExecutionEventDto.JSON_PROPERTY_STATE,
DominoComputegridExecutionEventDto.JSON_PROPERTY_OUTCOME,
DominoComputegridExecutionEventDto.JSON_PROPERTY_TIMESTAMP,
DominoComputegridExecutionEventDto.JSON_PROPERTY_KIND,
DominoComputegridExecutionEventDto.JSON_PROPERTY_OUTCOME_MESSAGE,
DominoComputegridExecutionEventDto.JSON_PROPERTY_SAGA_ID,
DominoComputegridExecutionEventDto.JSON_PROPERTY_DURATION_MILLIS,
DominoComputegridExecutionEventDto.JSON_PROPERTY_UPDATE_TIME,
DominoComputegridExecutionEventDto.JSON_PROPERTY_ELAPSED_MILLIS,
DominoComputegridExecutionEventDto.JSON_PROPERTY_OUTCOME_DETAILS,
DominoComputegridExecutionEventDto.JSON_PROPERTY_MAX_ATTEMPTS,
DominoComputegridExecutionEventDto.JSON_PROPERTY_EXECUTION_ID,
DominoComputegridExecutionEventDto.JSON_PROPERTY_SAGA_NAME,
DominoComputegridExecutionEventDto.JSON_PROPERTY_ATTEMPT_CORRELATION_ID,
DominoComputegridExecutionEventDto.JSON_PROPERTY_NEXT_STATE
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2023-10-17T15:20:46.682098100-04:00[America/New_York]")
public class DominoComputegridExecutionEventDto {
public static final String JSON_PROPERTY_REASON = "reason";
private String reason;
public static final String JSON_PROPERTY_METRIC_NAME = "metricName";
private String metricName;
public static final String JSON_PROPERTY_CREATION_TIME = "creationTime";
private OffsetDateTime creationTime;
/**
* Gets or Sets executionType
*/
public enum ExecutionTypeEnum {
OTHER("Other"),
SSHPROXY("SSHProxy"),
MODEL_API("Model API"),
ENDPOINT("Endpoint"),
APP("App"),
SCHEDULED("Scheduled"),
LAUNCHER("Launcher"),
BATCH("Batch"),
WORKSPACE("Workspace");
private String value;
ExecutionTypeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static ExecutionTypeEnum fromValue(String value) {
for (ExecutionTypeEnum b : ExecutionTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return null;
}
}
public static final String JSON_PROPERTY_EXECUTION_TYPE = "executionType";
private ExecutionTypeEnum executionType;
public static final String JSON_PROPERTY_DESCRIPTION = "description";
private String description;
/**
* Gets or Sets source
*/
public enum SourceEnum {
DOMINO("Domino"),
KUBERNETES("Kubernetes"),
METRICS("Metrics");
private String value;
SourceEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static SourceEnum fromValue(String value) {
for (SourceEnum b : SourceEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_SOURCE = "source";
private SourceEnum source;
public static final String JSON_PROPERTY_ATTEMPT = "attempt";
private Integer attempt;
/**
* Gets or Sets resourceKind
*/
public enum ResourceKindEnum {
OTHER("Other"),
EVENT("Event"),
DEPLOYMENT("Deployment"),
JOB("Job"),
SERVICE("Service"),
POD("Pod");
private String value;
ResourceKindEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static ResourceKindEnum fromValue(String value) {
for (ResourceKindEnum b : ResourceKindEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return null;
}
}
public static final String JSON_PROPERTY_RESOURCE_KIND = "resourceKind";
private ResourceKindEnum resourceKind;
public static final String JSON_PROPERTY_PAYLOAD = "payload";
private Object payload;
public static final String JSON_PROPERTY_ID = "id";
private String id;
public static final String JSON_PROPERTY_STATE = "state";
private String state;
/**
* Gets or Sets outcome
*/
public enum OutcomeEnum {
ERROR("Error"),
RETRY("Retry"),
FAILURE("Failure"),
IGNORED("Ignored"),
SUCCESS("Success");
private String value;
OutcomeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static OutcomeEnum fromValue(String value) {
for (OutcomeEnum b : OutcomeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return null;
}
}
public static final String JSON_PROPERTY_OUTCOME = "outcome";
private OutcomeEnum outcome;
public static final String JSON_PROPERTY_TIMESTAMP = "timestamp";
private OffsetDateTime timestamp;
public static final String JSON_PROPERTY_KIND = "kind";
private String kind;
public static final String JSON_PROPERTY_OUTCOME_MESSAGE = "outcomeMessage";
private String outcomeMessage;
public static final String JSON_PROPERTY_SAGA_ID = "sagaId";
private String sagaId;
public static final String JSON_PROPERTY_DURATION_MILLIS = "durationMillis";
private Long durationMillis;
public static final String JSON_PROPERTY_UPDATE_TIME = "updateTime";
private OffsetDateTime updateTime;
public static final String JSON_PROPERTY_ELAPSED_MILLIS = "elapsedMillis";
private Long elapsedMillis;
public static final String JSON_PROPERTY_OUTCOME_DETAILS = "outcomeDetails";
private String outcomeDetails;
public static final String JSON_PROPERTY_MAX_ATTEMPTS = "maxAttempts";
private Integer maxAttempts;
public static final String JSON_PROPERTY_EXECUTION_ID = "executionId";
private String executionId;
public static final String JSON_PROPERTY_SAGA_NAME = "sagaName";
private String sagaName;
public static final String JSON_PROPERTY_ATTEMPT_CORRELATION_ID = "attemptCorrelationId";
private String attemptCorrelationId;
public static final String JSON_PROPERTY_NEXT_STATE = "nextState";
private String nextState;
public DominoComputegridExecutionEventDto() {
}
public DominoComputegridExecutionEventDto reason(String reason) {
this.reason = reason;
return this;
}
/**
* Get reason
* @return reason
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_REASON)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getReason() {
return reason;
}
@JsonProperty(JSON_PROPERTY_REASON)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setReason(String reason) {
this.reason = reason;
}
public DominoComputegridExecutionEventDto metricName(String metricName) {
this.metricName = metricName;
return this;
}
/**
* Get metricName
* @return metricName
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_METRIC_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getMetricName() {
return metricName;
}
@JsonProperty(JSON_PROPERTY_METRIC_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMetricName(String metricName) {
this.metricName = metricName;
}
public DominoComputegridExecutionEventDto creationTime(OffsetDateTime creationTime) {
this.creationTime = creationTime;
return this;
}
/**
* Get creationTime
* @return creationTime
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_CREATION_TIME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public OffsetDateTime getCreationTime() {
return creationTime;
}
@JsonProperty(JSON_PROPERTY_CREATION_TIME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setCreationTime(OffsetDateTime creationTime) {
this.creationTime = creationTime;
}
public DominoComputegridExecutionEventDto executionType(ExecutionTypeEnum executionType) {
this.executionType = executionType;
return this;
}
/**
* Get executionType
* @return executionType
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_EXECUTION_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ExecutionTypeEnum getExecutionType() {
return executionType;
}
@JsonProperty(JSON_PROPERTY_EXECUTION_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setExecutionType(ExecutionTypeEnum executionType) {
this.executionType = executionType;
}
public DominoComputegridExecutionEventDto description(String description) {
this.description = description;
return this;
}
/**
* Get description
* @return description
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDescription() {
return description;
}
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDescription(String description) {
this.description = description;
}
public DominoComputegridExecutionEventDto source(SourceEnum source) {
this.source = source;
return this;
}
/**
* Get source
* @return source
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_SOURCE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public SourceEnum getSource() {
return source;
}
@JsonProperty(JSON_PROPERTY_SOURCE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setSource(SourceEnum source) {
this.source = source;
}
public DominoComputegridExecutionEventDto attempt(Integer attempt) {
this.attempt = attempt;
return this;
}
/**
* Get attempt
* @return attempt
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ATTEMPT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Integer getAttempt() {
return attempt;
}
@JsonProperty(JSON_PROPERTY_ATTEMPT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setAttempt(Integer attempt) {
this.attempt = attempt;
}
public DominoComputegridExecutionEventDto resourceKind(ResourceKindEnum resourceKind) {
this.resourceKind = resourceKind;
return this;
}
/**
* Get resourceKind
* @return resourceKind
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_RESOURCE_KIND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ResourceKindEnum getResourceKind() {
return resourceKind;
}
@JsonProperty(JSON_PROPERTY_RESOURCE_KIND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setResourceKind(ResourceKindEnum resourceKind) {
this.resourceKind = resourceKind;
}
public DominoComputegridExecutionEventDto payload(Object payload) {
this.payload = payload;
return this;
}
/**
* Get payload
* @return payload
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_PAYLOAD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Object getPayload() {
return payload;
}
@JsonProperty(JSON_PROPERTY_PAYLOAD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPayload(Object payload) {
this.payload = payload;
}
public DominoComputegridExecutionEventDto id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getId() {
return id;
}
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setId(String id) {
this.id = id;
}
public DominoComputegridExecutionEventDto state(String state) {
this.state = state;
return this;
}
/**
* Get state
* @return state
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_STATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getState() {
return state;
}
@JsonProperty(JSON_PROPERTY_STATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setState(String state) {
this.state = state;
}
public DominoComputegridExecutionEventDto outcome(OutcomeEnum outcome) {
this.outcome = outcome;
return this;
}
/**
* Get outcome
* @return outcome
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_OUTCOME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OutcomeEnum getOutcome() {
return outcome;
}
@JsonProperty(JSON_PROPERTY_OUTCOME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setOutcome(OutcomeEnum outcome) {
this.outcome = outcome;
}
public DominoComputegridExecutionEventDto timestamp(OffsetDateTime timestamp) {
this.timestamp = timestamp;
return this;
}
/**
* Get timestamp
* @return timestamp
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_TIMESTAMP)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public OffsetDateTime getTimestamp() {
return timestamp;
}
@JsonProperty(JSON_PROPERTY_TIMESTAMP)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setTimestamp(OffsetDateTime timestamp) {
this.timestamp = timestamp;
}
public DominoComputegridExecutionEventDto kind(String kind) {
this.kind = kind;
return this;
}
/**
* Get kind
* @return kind
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_KIND)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getKind() {
return kind;
}
@JsonProperty(JSON_PROPERTY_KIND)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setKind(String kind) {
this.kind = kind;
}
public DominoComputegridExecutionEventDto outcomeMessage(String outcomeMessage) {
this.outcomeMessage = outcomeMessage;
return this;
}
/**
* Get outcomeMessage
* @return outcomeMessage
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_OUTCOME_MESSAGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getOutcomeMessage() {
return outcomeMessage;
}
@JsonProperty(JSON_PROPERTY_OUTCOME_MESSAGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setOutcomeMessage(String outcomeMessage) {
this.outcomeMessage = outcomeMessage;
}
public DominoComputegridExecutionEventDto sagaId(String sagaId) {
this.sagaId = sagaId;
return this;
}
/**
* Get sagaId
* @return sagaId
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_SAGA_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getSagaId() {
return sagaId;
}
@JsonProperty(JSON_PROPERTY_SAGA_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSagaId(String sagaId) {
this.sagaId = sagaId;
}
public DominoComputegridExecutionEventDto durationMillis(Long durationMillis) {
this.durationMillis = durationMillis;
return this;
}
/**
* Get durationMillis
* @return durationMillis
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DURATION_MILLIS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getDurationMillis() {
return durationMillis;
}
@JsonProperty(JSON_PROPERTY_DURATION_MILLIS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDurationMillis(Long durationMillis) {
this.durationMillis = durationMillis;
}
public DominoComputegridExecutionEventDto updateTime(OffsetDateTime updateTime) {
this.updateTime = updateTime;
return this;
}
/**
* Get updateTime
* @return updateTime
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_UPDATE_TIME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public OffsetDateTime getUpdateTime() {
return updateTime;
}
@JsonProperty(JSON_PROPERTY_UPDATE_TIME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setUpdateTime(OffsetDateTime updateTime) {
this.updateTime = updateTime;
}
public DominoComputegridExecutionEventDto elapsedMillis(Long elapsedMillis) {
this.elapsedMillis = elapsedMillis;
return this;
}
/**
* Get elapsedMillis
* @return elapsedMillis
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ELAPSED_MILLIS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Long getElapsedMillis() {
return elapsedMillis;
}
@JsonProperty(JSON_PROPERTY_ELAPSED_MILLIS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setElapsedMillis(Long elapsedMillis) {
this.elapsedMillis = elapsedMillis;
}
public DominoComputegridExecutionEventDto outcomeDetails(String outcomeDetails) {
this.outcomeDetails = outcomeDetails;
return this;
}
/**
* Get outcomeDetails
* @return outcomeDetails
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_OUTCOME_DETAILS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getOutcomeDetails() {
return outcomeDetails;
}
@JsonProperty(JSON_PROPERTY_OUTCOME_DETAILS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setOutcomeDetails(String outcomeDetails) {
this.outcomeDetails = outcomeDetails;
}
public DominoComputegridExecutionEventDto maxAttempts(Integer maxAttempts) {
this.maxAttempts = maxAttempts;
return this;
}
/**
* Get maxAttempts
* @return maxAttempts
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_MAX_ATTEMPTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getMaxAttempts() {
return maxAttempts;
}
@JsonProperty(JSON_PROPERTY_MAX_ATTEMPTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMaxAttempts(Integer maxAttempts) {
this.maxAttempts = maxAttempts;
}
public DominoComputegridExecutionEventDto executionId(String executionId) {
this.executionId = executionId;
return this;
}
/**
* Get executionId
* @return executionId
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_EXECUTION_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getExecutionId() {
return executionId;
}
@JsonProperty(JSON_PROPERTY_EXECUTION_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setExecutionId(String executionId) {
this.executionId = executionId;
}
public DominoComputegridExecutionEventDto sagaName(String sagaName) {
this.sagaName = sagaName;
return this;
}
/**
* Get sagaName
* @return sagaName
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_SAGA_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getSagaName() {
return sagaName;
}
@JsonProperty(JSON_PROPERTY_SAGA_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSagaName(String sagaName) {
this.sagaName = sagaName;
}
public DominoComputegridExecutionEventDto attemptCorrelationId(String attemptCorrelationId) {
this.attemptCorrelationId = attemptCorrelationId;
return this;
}
/**
* Get attemptCorrelationId
* @return attemptCorrelationId
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ATTEMPT_CORRELATION_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getAttemptCorrelationId() {
return attemptCorrelationId;
}
@JsonProperty(JSON_PROPERTY_ATTEMPT_CORRELATION_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setAttemptCorrelationId(String attemptCorrelationId) {
this.attemptCorrelationId = attemptCorrelationId;
}
public DominoComputegridExecutionEventDto nextState(String nextState) {
this.nextState = nextState;
return this;
}
/**
* Get nextState
* @return nextState
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_NEXT_STATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getNextState() {
return nextState;
}
@JsonProperty(JSON_PROPERTY_NEXT_STATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setNextState(String nextState) {
this.nextState = nextState;
}
/**
* Return true if this domino.computegrid.ExecutionEventDto object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DominoComputegridExecutionEventDto dominoComputegridExecutionEventDto = (DominoComputegridExecutionEventDto) o;
return Objects.equals(this.reason, dominoComputegridExecutionEventDto.reason) &&
Objects.equals(this.metricName, dominoComputegridExecutionEventDto.metricName) &&
Objects.equals(this.creationTime, dominoComputegridExecutionEventDto.creationTime) &&
Objects.equals(this.executionType, dominoComputegridExecutionEventDto.executionType) &&
Objects.equals(this.description, dominoComputegridExecutionEventDto.description) &&
Objects.equals(this.source, dominoComputegridExecutionEventDto.source) &&
Objects.equals(this.attempt, dominoComputegridExecutionEventDto.attempt) &&
Objects.equals(this.resourceKind, dominoComputegridExecutionEventDto.resourceKind) &&
Objects.equals(this.payload, dominoComputegridExecutionEventDto.payload) &&
Objects.equals(this.id, dominoComputegridExecutionEventDto.id) &&
Objects.equals(this.state, dominoComputegridExecutionEventDto.state) &&
Objects.equals(this.outcome, dominoComputegridExecutionEventDto.outcome) &&
Objects.equals(this.timestamp, dominoComputegridExecutionEventDto.timestamp) &&
Objects.equals(this.kind, dominoComputegridExecutionEventDto.kind) &&
Objects.equals(this.outcomeMessage, dominoComputegridExecutionEventDto.outcomeMessage) &&
Objects.equals(this.sagaId, dominoComputegridExecutionEventDto.sagaId) &&
Objects.equals(this.durationMillis, dominoComputegridExecutionEventDto.durationMillis) &&
Objects.equals(this.updateTime, dominoComputegridExecutionEventDto.updateTime) &&
Objects.equals(this.elapsedMillis, dominoComputegridExecutionEventDto.elapsedMillis) &&
Objects.equals(this.outcomeDetails, dominoComputegridExecutionEventDto.outcomeDetails) &&
Objects.equals(this.maxAttempts, dominoComputegridExecutionEventDto.maxAttempts) &&
Objects.equals(this.executionId, dominoComputegridExecutionEventDto.executionId) &&
Objects.equals(this.sagaName, dominoComputegridExecutionEventDto.sagaName) &&
Objects.equals(this.attemptCorrelationId, dominoComputegridExecutionEventDto.attemptCorrelationId) &&
Objects.equals(this.nextState, dominoComputegridExecutionEventDto.nextState);
}
@Override
public int hashCode() {
return Objects.hash(reason, metricName, creationTime, executionType, description, source, attempt, resourceKind, payload, id, state, outcome, timestamp, kind, outcomeMessage, sagaId, durationMillis, updateTime, elapsedMillis, outcomeDetails, maxAttempts, executionId, sagaName, attemptCorrelationId, nextState);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DominoComputegridExecutionEventDto {\n");
sb.append(" reason: ").append(toIndentedString(reason)).append("\n");
sb.append(" metricName: ").append(toIndentedString(metricName)).append("\n");
sb.append(" creationTime: ").append(toIndentedString(creationTime)).append("\n");
sb.append(" executionType: ").append(toIndentedString(executionType)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" source: ").append(toIndentedString(source)).append("\n");
sb.append(" attempt: ").append(toIndentedString(attempt)).append("\n");
sb.append(" resourceKind: ").append(toIndentedString(resourceKind)).append("\n");
sb.append(" payload: ").append(toIndentedString(payload)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" state: ").append(toIndentedString(state)).append("\n");
sb.append(" outcome: ").append(toIndentedString(outcome)).append("\n");
sb.append(" timestamp: ").append(toIndentedString(timestamp)).append("\n");
sb.append(" kind: ").append(toIndentedString(kind)).append("\n");
sb.append(" outcomeMessage: ").append(toIndentedString(outcomeMessage)).append("\n");
sb.append(" sagaId: ").append(toIndentedString(sagaId)).append("\n");
sb.append(" durationMillis: ").append(toIndentedString(durationMillis)).append("\n");
sb.append(" updateTime: ").append(toIndentedString(updateTime)).append("\n");
sb.append(" elapsedMillis: ").append(toIndentedString(elapsedMillis)).append("\n");
sb.append(" outcomeDetails: ").append(toIndentedString(outcomeDetails)).append("\n");
sb.append(" maxAttempts: ").append(toIndentedString(maxAttempts)).append("\n");
sb.append(" executionId: ").append(toIndentedString(executionId)).append("\n");
sb.append(" sagaName: ").append(toIndentedString(sagaName)).append("\n");
sb.append(" attemptCorrelationId: ").append(toIndentedString(attemptCorrelationId)).append("\n");
sb.append(" nextState: ").append(toIndentedString(nextState)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `reason` to the URL query string
if (getReason() != null) {
joiner.add(String.format("%sreason%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getReason()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `metricName` to the URL query string
if (getMetricName() != null) {
joiner.add(String.format("%smetricName%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getMetricName()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `creationTime` to the URL query string
if (getCreationTime() != null) {
joiner.add(String.format("%screationTime%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getCreationTime()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `executionType` to the URL query string
if (getExecutionType() != null) {
joiner.add(String.format("%sexecutionType%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getExecutionType()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `description` to the URL query string
if (getDescription() != null) {
joiner.add(String.format("%sdescription%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getDescription()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `source` to the URL query string
if (getSource() != null) {
joiner.add(String.format("%ssource%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getSource()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `attempt` to the URL query string
if (getAttempt() != null) {
joiner.add(String.format("%sattempt%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getAttempt()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `resourceKind` to the URL query string
if (getResourceKind() != null) {
joiner.add(String.format("%sresourceKind%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getResourceKind()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `payload` to the URL query string
if (getPayload() != null) {
joiner.add(String.format("%spayload%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getPayload()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `id` to the URL query string
if (getId() != null) {
joiner.add(String.format("%sid%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `state` to the URL query string
if (getState() != null) {
joiner.add(String.format("%sstate%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getState()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `outcome` to the URL query string
if (getOutcome() != null) {
joiner.add(String.format("%soutcome%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getOutcome()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `timestamp` to the URL query string
if (getTimestamp() != null) {
joiner.add(String.format("%stimestamp%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getTimestamp()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `kind` to the URL query string
if (getKind() != null) {
joiner.add(String.format("%skind%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getKind()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `outcomeMessage` to the URL query string
if (getOutcomeMessage() != null) {
joiner.add(String.format("%soutcomeMessage%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getOutcomeMessage()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `sagaId` to the URL query string
if (getSagaId() != null) {
joiner.add(String.format("%ssagaId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getSagaId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `durationMillis` to the URL query string
if (getDurationMillis() != null) {
joiner.add(String.format("%sdurationMillis%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getDurationMillis()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `updateTime` to the URL query string
if (getUpdateTime() != null) {
joiner.add(String.format("%supdateTime%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getUpdateTime()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `elapsedMillis` to the URL query string
if (getElapsedMillis() != null) {
joiner.add(String.format("%selapsedMillis%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getElapsedMillis()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `outcomeDetails` to the URL query string
if (getOutcomeDetails() != null) {
joiner.add(String.format("%soutcomeDetails%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getOutcomeDetails()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `maxAttempts` to the URL query string
if (getMaxAttempts() != null) {
joiner.add(String.format("%smaxAttempts%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getMaxAttempts()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `executionId` to the URL query string
if (getExecutionId() != null) {
joiner.add(String.format("%sexecutionId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getExecutionId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `sagaName` to the URL query string
if (getSagaName() != null) {
joiner.add(String.format("%ssagaName%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getSagaName()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `attemptCorrelationId` to the URL query string
if (getAttemptCorrelationId() != null) {
joiner.add(String.format("%sattemptCorrelationId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getAttemptCorrelationId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `nextState` to the URL query string
if (getNextState() != null) {
joiner.add(String.format("%snextState%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getNextState()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy