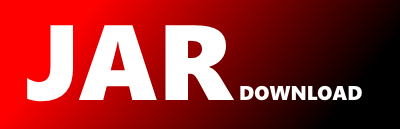
com.dominodatalab.api.model.DominoDatasetApiDatasetSnapshotDto Maven / Gradle / Ivy
/*
* Domino Data Lab API v4
* This API is going to provide access to all the Domino functions available in the user interface. To authenticate your requests, include your API Key (which you can find on your account page) with the header X-Domino-Api-Key.
*
* The version of the OpenAPI document: 4.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.api.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.Arrays;
import java.util.HashMap;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* DominoDatasetApiDatasetSnapshotDto
*/
@JsonPropertyOrder({
DominoDatasetApiDatasetSnapshotDto.JSON_PROPERTY_RESOURCE_ID,
DominoDatasetApiDatasetSnapshotDto.JSON_PROPERTY_CREATION_TIME,
DominoDatasetApiDatasetSnapshotDto.JSON_PROPERTY_AUTHOR,
DominoDatasetApiDatasetSnapshotDto.JSON_PROPERTY_DELETED_BY_USER,
DominoDatasetApiDatasetSnapshotDto.JSON_PROPERTY_DESCRIPTION,
DominoDatasetApiDatasetSnapshotDto.JSON_PROPERTY_LAST_USED_TIME,
DominoDatasetApiDatasetSnapshotDto.JSON_PROPERTY_VERSION,
DominoDatasetApiDatasetSnapshotDto.JSON_PROPERTY_LABELS,
DominoDatasetApiDatasetSnapshotDto.JSON_PROPERTY_MARKED_FOR_DELETION_TIME,
DominoDatasetApiDatasetSnapshotDto.JSON_PROPERTY_DELETED,
DominoDatasetApiDatasetSnapshotDto.JSON_PROPERTY_DELETE_TIME,
DominoDatasetApiDatasetSnapshotDto.JSON_PROPERTY_STORAGE_SIZE,
DominoDatasetApiDatasetSnapshotDto.JSON_PROPERTY_DATASET_ID,
DominoDatasetApiDatasetSnapshotDto.JSON_PROPERTY_ID,
DominoDatasetApiDatasetSnapshotDto.JSON_PROPERTY_IS_PARTIAL_SIZE,
DominoDatasetApiDatasetSnapshotDto.JSON_PROPERTY_STATUS
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2023-10-17T15:20:46.682098100-04:00[America/New_York]")
public class DominoDatasetApiDatasetSnapshotDto {
public static final String JSON_PROPERTY_RESOURCE_ID = "resourceId";
private String resourceId;
public static final String JSON_PROPERTY_CREATION_TIME = "creationTime";
private Long creationTime;
public static final String JSON_PROPERTY_AUTHOR = "author";
private String author;
public static final String JSON_PROPERTY_DELETED_BY_USER = "deletedByUser";
private String deletedByUser;
public static final String JSON_PROPERTY_DESCRIPTION = "description";
private String description;
public static final String JSON_PROPERTY_LAST_USED_TIME = "lastUsedTime";
private Long lastUsedTime;
public static final String JSON_PROPERTY_VERSION = "version";
private Integer version;
public static final String JSON_PROPERTY_LABELS = "labels";
private Map labels = new HashMap<>();
public static final String JSON_PROPERTY_MARKED_FOR_DELETION_TIME = "markedForDeletionTime";
private Long markedForDeletionTime;
public static final String JSON_PROPERTY_DELETED = "deleted";
private Boolean deleted;
public static final String JSON_PROPERTY_DELETE_TIME = "deleteTime";
private Long deleteTime;
public static final String JSON_PROPERTY_STORAGE_SIZE = "storageSize";
private Long storageSize;
public static final String JSON_PROPERTY_DATASET_ID = "datasetId";
private String datasetId;
public static final String JSON_PROPERTY_ID = "id";
private String id;
public static final String JSON_PROPERTY_IS_PARTIAL_SIZE = "isPartialSize";
private Boolean isPartialSize;
public static final String JSON_PROPERTY_STATUS = "status";
private String status;
public DominoDatasetApiDatasetSnapshotDto() {
}
public DominoDatasetApiDatasetSnapshotDto resourceId(String resourceId) {
this.resourceId = resourceId;
return this;
}
/**
* Get resourceId
* @return resourceId
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_RESOURCE_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getResourceId() {
return resourceId;
}
@JsonProperty(JSON_PROPERTY_RESOURCE_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setResourceId(String resourceId) {
this.resourceId = resourceId;
}
public DominoDatasetApiDatasetSnapshotDto creationTime(Long creationTime) {
this.creationTime = creationTime;
return this;
}
/**
* Get creationTime
* @return creationTime
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_CREATION_TIME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Long getCreationTime() {
return creationTime;
}
@JsonProperty(JSON_PROPERTY_CREATION_TIME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setCreationTime(Long creationTime) {
this.creationTime = creationTime;
}
public DominoDatasetApiDatasetSnapshotDto author(String author) {
this.author = author;
return this;
}
/**
* Get author
* @return author
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_AUTHOR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getAuthor() {
return author;
}
@JsonProperty(JSON_PROPERTY_AUTHOR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAuthor(String author) {
this.author = author;
}
public DominoDatasetApiDatasetSnapshotDto deletedByUser(String deletedByUser) {
this.deletedByUser = deletedByUser;
return this;
}
/**
* Get deletedByUser
* @return deletedByUser
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DELETED_BY_USER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDeletedByUser() {
return deletedByUser;
}
@JsonProperty(JSON_PROPERTY_DELETED_BY_USER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDeletedByUser(String deletedByUser) {
this.deletedByUser = deletedByUser;
}
public DominoDatasetApiDatasetSnapshotDto description(String description) {
this.description = description;
return this;
}
/**
* Get description
* @return description
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDescription() {
return description;
}
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDescription(String description) {
this.description = description;
}
public DominoDatasetApiDatasetSnapshotDto lastUsedTime(Long lastUsedTime) {
this.lastUsedTime = lastUsedTime;
return this;
}
/**
* Get lastUsedTime
* @return lastUsedTime
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_LAST_USED_TIME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getLastUsedTime() {
return lastUsedTime;
}
@JsonProperty(JSON_PROPERTY_LAST_USED_TIME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLastUsedTime(Long lastUsedTime) {
this.lastUsedTime = lastUsedTime;
}
public DominoDatasetApiDatasetSnapshotDto version(Integer version) {
this.version = version;
return this;
}
/**
* Get version
* @return version
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_VERSION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Integer getVersion() {
return version;
}
@JsonProperty(JSON_PROPERTY_VERSION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setVersion(Integer version) {
this.version = version;
}
public DominoDatasetApiDatasetSnapshotDto labels(Map labels) {
this.labels = labels;
return this;
}
public DominoDatasetApiDatasetSnapshotDto putLabelsItem(String key, String labelsItem) {
if (this.labels == null) {
this.labels = new HashMap<>();
}
this.labels.put(key, labelsItem);
return this;
}
/**
* Get labels
* @return labels
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_LABELS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Map getLabels() {
return labels;
}
@JsonProperty(JSON_PROPERTY_LABELS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setLabels(Map labels) {
this.labels = labels;
}
public DominoDatasetApiDatasetSnapshotDto markedForDeletionTime(Long markedForDeletionTime) {
this.markedForDeletionTime = markedForDeletionTime;
return this;
}
/**
* Get markedForDeletionTime
* @return markedForDeletionTime
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_MARKED_FOR_DELETION_TIME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getMarkedForDeletionTime() {
return markedForDeletionTime;
}
@JsonProperty(JSON_PROPERTY_MARKED_FOR_DELETION_TIME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMarkedForDeletionTime(Long markedForDeletionTime) {
this.markedForDeletionTime = markedForDeletionTime;
}
public DominoDatasetApiDatasetSnapshotDto deleted(Boolean deleted) {
this.deleted = deleted;
return this;
}
/**
* Get deleted
* @return deleted
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_DELETED)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getDeleted() {
return deleted;
}
@JsonProperty(JSON_PROPERTY_DELETED)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDeleted(Boolean deleted) {
this.deleted = deleted;
}
public DominoDatasetApiDatasetSnapshotDto deleteTime(Long deleteTime) {
this.deleteTime = deleteTime;
return this;
}
/**
* Get deleteTime
* @return deleteTime
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DELETE_TIME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getDeleteTime() {
return deleteTime;
}
@JsonProperty(JSON_PROPERTY_DELETE_TIME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDeleteTime(Long deleteTime) {
this.deleteTime = deleteTime;
}
public DominoDatasetApiDatasetSnapshotDto storageSize(Long storageSize) {
this.storageSize = storageSize;
return this;
}
/**
* Get storageSize
* @return storageSize
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_STORAGE_SIZE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Long getStorageSize() {
return storageSize;
}
@JsonProperty(JSON_PROPERTY_STORAGE_SIZE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setStorageSize(Long storageSize) {
this.storageSize = storageSize;
}
public DominoDatasetApiDatasetSnapshotDto datasetId(String datasetId) {
this.datasetId = datasetId;
return this;
}
/**
* Get datasetId
* @return datasetId
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_DATASET_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getDatasetId() {
return datasetId;
}
@JsonProperty(JSON_PROPERTY_DATASET_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDatasetId(String datasetId) {
this.datasetId = datasetId;
}
public DominoDatasetApiDatasetSnapshotDto id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getId() {
return id;
}
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setId(String id) {
this.id = id;
}
public DominoDatasetApiDatasetSnapshotDto isPartialSize(Boolean isPartialSize) {
this.isPartialSize = isPartialSize;
return this;
}
/**
* Get isPartialSize
* @return isPartialSize
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_IS_PARTIAL_SIZE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getIsPartialSize() {
return isPartialSize;
}
@JsonProperty(JSON_PROPERTY_IS_PARTIAL_SIZE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setIsPartialSize(Boolean isPartialSize) {
this.isPartialSize = isPartialSize;
}
public DominoDatasetApiDatasetSnapshotDto status(String status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getStatus() {
return status;
}
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setStatus(String status) {
this.status = status;
}
/**
* Return true if this domino.dataset.api.DatasetSnapshotDto object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DominoDatasetApiDatasetSnapshotDto dominoDatasetApiDatasetSnapshotDto = (DominoDatasetApiDatasetSnapshotDto) o;
return Objects.equals(this.resourceId, dominoDatasetApiDatasetSnapshotDto.resourceId) &&
Objects.equals(this.creationTime, dominoDatasetApiDatasetSnapshotDto.creationTime) &&
Objects.equals(this.author, dominoDatasetApiDatasetSnapshotDto.author) &&
Objects.equals(this.deletedByUser, dominoDatasetApiDatasetSnapshotDto.deletedByUser) &&
Objects.equals(this.description, dominoDatasetApiDatasetSnapshotDto.description) &&
Objects.equals(this.lastUsedTime, dominoDatasetApiDatasetSnapshotDto.lastUsedTime) &&
Objects.equals(this.version, dominoDatasetApiDatasetSnapshotDto.version) &&
Objects.equals(this.labels, dominoDatasetApiDatasetSnapshotDto.labels) &&
Objects.equals(this.markedForDeletionTime, dominoDatasetApiDatasetSnapshotDto.markedForDeletionTime) &&
Objects.equals(this.deleted, dominoDatasetApiDatasetSnapshotDto.deleted) &&
Objects.equals(this.deleteTime, dominoDatasetApiDatasetSnapshotDto.deleteTime) &&
Objects.equals(this.storageSize, dominoDatasetApiDatasetSnapshotDto.storageSize) &&
Objects.equals(this.datasetId, dominoDatasetApiDatasetSnapshotDto.datasetId) &&
Objects.equals(this.id, dominoDatasetApiDatasetSnapshotDto.id) &&
Objects.equals(this.isPartialSize, dominoDatasetApiDatasetSnapshotDto.isPartialSize) &&
Objects.equals(this.status, dominoDatasetApiDatasetSnapshotDto.status);
}
@Override
public int hashCode() {
return Objects.hash(resourceId, creationTime, author, deletedByUser, description, lastUsedTime, version, labels, markedForDeletionTime, deleted, deleteTime, storageSize, datasetId, id, isPartialSize, status);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DominoDatasetApiDatasetSnapshotDto {\n");
sb.append(" resourceId: ").append(toIndentedString(resourceId)).append("\n");
sb.append(" creationTime: ").append(toIndentedString(creationTime)).append("\n");
sb.append(" author: ").append(toIndentedString(author)).append("\n");
sb.append(" deletedByUser: ").append(toIndentedString(deletedByUser)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" lastUsedTime: ").append(toIndentedString(lastUsedTime)).append("\n");
sb.append(" version: ").append(toIndentedString(version)).append("\n");
sb.append(" labels: ").append(toIndentedString(labels)).append("\n");
sb.append(" markedForDeletionTime: ").append(toIndentedString(markedForDeletionTime)).append("\n");
sb.append(" deleted: ").append(toIndentedString(deleted)).append("\n");
sb.append(" deleteTime: ").append(toIndentedString(deleteTime)).append("\n");
sb.append(" storageSize: ").append(toIndentedString(storageSize)).append("\n");
sb.append(" datasetId: ").append(toIndentedString(datasetId)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" isPartialSize: ").append(toIndentedString(isPartialSize)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `resourceId` to the URL query string
if (getResourceId() != null) {
joiner.add(String.format("%sresourceId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getResourceId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `creationTime` to the URL query string
if (getCreationTime() != null) {
joiner.add(String.format("%screationTime%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getCreationTime()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `author` to the URL query string
if (getAuthor() != null) {
joiner.add(String.format("%sauthor%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getAuthor()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `deletedByUser` to the URL query string
if (getDeletedByUser() != null) {
joiner.add(String.format("%sdeletedByUser%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getDeletedByUser()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `description` to the URL query string
if (getDescription() != null) {
joiner.add(String.format("%sdescription%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getDescription()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `lastUsedTime` to the URL query string
if (getLastUsedTime() != null) {
joiner.add(String.format("%slastUsedTime%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getLastUsedTime()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `version` to the URL query string
if (getVersion() != null) {
joiner.add(String.format("%sversion%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getVersion()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `labels` to the URL query string
if (getLabels() != null) {
for (String _key : getLabels().keySet()) {
joiner.add(String.format("%slabels%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, _key, containerSuffix),
getLabels().get(_key), URLEncoder.encode(String.valueOf(getLabels().get(_key)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
// add `markedForDeletionTime` to the URL query string
if (getMarkedForDeletionTime() != null) {
joiner.add(String.format("%smarkedForDeletionTime%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getMarkedForDeletionTime()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `deleted` to the URL query string
if (getDeleted() != null) {
joiner.add(String.format("%sdeleted%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getDeleted()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `deleteTime` to the URL query string
if (getDeleteTime() != null) {
joiner.add(String.format("%sdeleteTime%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getDeleteTime()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `storageSize` to the URL query string
if (getStorageSize() != null) {
joiner.add(String.format("%sstorageSize%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getStorageSize()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `datasetId` to the URL query string
if (getDatasetId() != null) {
joiner.add(String.format("%sdatasetId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getDatasetId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `id` to the URL query string
if (getId() != null) {
joiner.add(String.format("%sid%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `isPartialSize` to the URL query string
if (getIsPartialSize() != null) {
joiner.add(String.format("%sisPartialSize%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getIsPartialSize()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `status` to the URL query string
if (getStatus() != null) {
joiner.add(String.format("%sstatus%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getStatus()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy