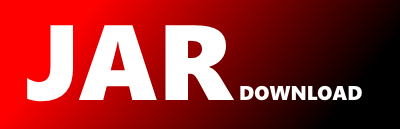
com.dominodatalab.api.model.DominoDatasourceWebCheckDataSourceConnectionRequest Maven / Gradle / Ivy
/*
* Domino Data Lab API v4
* This API is going to provide access to all the Domino functions available in the user interface. To authenticate your requests, include your API Key (which you can find on your account page) with the header X-Domino-Api-Key.
*
* The version of the OpenAPI document: 4.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.api.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* DominoDatasourceWebCheckDataSourceConnectionRequest
*/
@JsonPropertyOrder({
DominoDatasourceWebCheckDataSourceConnectionRequest.JSON_PROPERTY_ACCOUNT_I_D,
DominoDatasourceWebCheckDataSourceConnectionRequest.JSON_PROPERTY_ACCOUNT_NAME,
DominoDatasourceWebCheckDataSourceConnectionRequest.JSON_PROPERTY_AUTH_TYPE,
DominoDatasourceWebCheckDataSourceConnectionRequest.JSON_PROPERTY_BUCKET,
DominoDatasourceWebCheckDataSourceConnectionRequest.JSON_PROPERTY_CATALOG,
DominoDatasourceWebCheckDataSourceConnectionRequest.JSON_PROPERTY_CREDENTIAL_TYPE,
DominoDatasourceWebCheckDataSourceConnectionRequest.JSON_PROPERTY_DATA_SOURCE_TYPE,
DominoDatasourceWebCheckDataSourceConnectionRequest.JSON_PROPERTY_DATABASE,
DominoDatasourceWebCheckDataSourceConnectionRequest.JSON_PROPERTY_HOST,
DominoDatasourceWebCheckDataSourceConnectionRequest.JSON_PROPERTY_PORT,
DominoDatasourceWebCheckDataSourceConnectionRequest.JSON_PROPERTY_PROJECT,
DominoDatasourceWebCheckDataSourceConnectionRequest.JSON_PROPERTY_REGION,
DominoDatasourceWebCheckDataSourceConnectionRequest.JSON_PROPERTY_ROLE,
DominoDatasourceWebCheckDataSourceConnectionRequest.JSON_PROPERTY_SCHEMA,
DominoDatasourceWebCheckDataSourceConnectionRequest.JSON_PROPERTY_SECRET_CREDENTIAL,
DominoDatasourceWebCheckDataSourceConnectionRequest.JSON_PROPERTY_VISIBLE_CREDENTIAL,
DominoDatasourceWebCheckDataSourceConnectionRequest.JSON_PROPERTY_WAREHOUSE
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2023-10-17T15:20:46.682098100-04:00[America/New_York]")
public class DominoDatasourceWebCheckDataSourceConnectionRequest {
public static final String JSON_PROPERTY_ACCOUNT_I_D = "accountID";
private String accountID;
public static final String JSON_PROPERTY_ACCOUNT_NAME = "accountName";
private String accountName;
/**
* Gets or Sets authType
*/
public enum AuthTypeEnum {
AZUREBASIC("AzureBasic"),
BASIC("Basic"),
GCPBASIC("GCPBasic"),
AWSIAMBASIC("AWSIAMBasic"),
AWSIAMBASICNOOVERRIDE("AWSIAMBasicNoOverride"),
AWSIAMROLE("AWSIAMRole"),
AWSIAMROLEWITHUSERNAME("AWSIAMRoleWithUsername"),
OAUTH("OAuth"),
USERONLY("UserOnly"),
BASICOPTIONAL("BasicOptional"),
NOAUTH("NoAuth"),
CLIENTIDSECRET("ClientIdSecret");
private String value;
AuthTypeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static AuthTypeEnum fromValue(String value) {
for (AuthTypeEnum b : AuthTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_AUTH_TYPE = "authType";
private AuthTypeEnum authType;
public static final String JSON_PROPERTY_BUCKET = "bucket";
private String bucket;
public static final String JSON_PROPERTY_CATALOG = "catalog";
private String catalog;
/**
* Gets or Sets credentialType
*/
public enum CredentialTypeEnum {
INDIVIDUAL("Individual"),
SHARED("Shared");
private String value;
CredentialTypeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static CredentialTypeEnum fromValue(String value) {
for (CredentialTypeEnum b : CredentialTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_CREDENTIAL_TYPE = "credentialType";
private CredentialTypeEnum credentialType;
/**
* Gets or Sets dataSourceType
*/
public enum DataSourceTypeEnum {
ADLSCONFIG("ADLSConfig"),
BIGQUERYCONFIG("BigQueryConfig"),
GCSCONFIG("GCSConfig"),
GENERICS3CONFIG("GenericS3Config"),
MONGODBCONFIG("MongoDBConfig"),
MYSQLCONFIG("MySQLConfig"),
ORACLECONFIG("OracleConfig"),
POSTGRESQLCONFIG("PostgreSQLConfig"),
REDSHIFTCONFIG("RedshiftConfig"),
S3CONFIG("S3Config"),
SQLSERVERCONFIG("SQLServerConfig"),
SNOWFLAKECONFIG("SnowflakeConfig"),
TABULARS3GLUECONFIG("TabularS3GlueConfig"),
TERADATACONFIG("TeradataConfig"),
TRINOCONFIG("TrinoConfig"),
PALANTIRCONFIG("PalantirConfig");
private String value;
DataSourceTypeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static DataSourceTypeEnum fromValue(String value) {
for (DataSourceTypeEnum b : DataSourceTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_DATA_SOURCE_TYPE = "dataSourceType";
private DataSourceTypeEnum dataSourceType;
public static final String JSON_PROPERTY_DATABASE = "database";
private String database;
public static final String JSON_PROPERTY_HOST = "host";
private String host;
public static final String JSON_PROPERTY_PORT = "port";
private String port;
public static final String JSON_PROPERTY_PROJECT = "project";
private String project;
public static final String JSON_PROPERTY_REGION = "region";
private String region;
public static final String JSON_PROPERTY_ROLE = "role";
private String role;
public static final String JSON_PROPERTY_SCHEMA = "schema";
private String schema;
public static final String JSON_PROPERTY_SECRET_CREDENTIAL = "secretCredential";
private String secretCredential;
public static final String JSON_PROPERTY_VISIBLE_CREDENTIAL = "visibleCredential";
private String visibleCredential;
public static final String JSON_PROPERTY_WAREHOUSE = "warehouse";
private String warehouse;
public DominoDatasourceWebCheckDataSourceConnectionRequest() {
}
public DominoDatasourceWebCheckDataSourceConnectionRequest accountID(String accountID) {
this.accountID = accountID;
return this;
}
/**
* Get accountID
* @return accountID
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_ACCOUNT_I_D)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getAccountID() {
return accountID;
}
@JsonProperty(JSON_PROPERTY_ACCOUNT_I_D)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAccountID(String accountID) {
this.accountID = accountID;
}
public DominoDatasourceWebCheckDataSourceConnectionRequest accountName(String accountName) {
this.accountName = accountName;
return this;
}
/**
* Get accountName
* @return accountName
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_ACCOUNT_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getAccountName() {
return accountName;
}
@JsonProperty(JSON_PROPERTY_ACCOUNT_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAccountName(String accountName) {
this.accountName = accountName;
}
public DominoDatasourceWebCheckDataSourceConnectionRequest authType(AuthTypeEnum authType) {
this.authType = authType;
return this;
}
/**
* Get authType
* @return authType
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_AUTH_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public AuthTypeEnum getAuthType() {
return authType;
}
@JsonProperty(JSON_PROPERTY_AUTH_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setAuthType(AuthTypeEnum authType) {
this.authType = authType;
}
public DominoDatasourceWebCheckDataSourceConnectionRequest bucket(String bucket) {
this.bucket = bucket;
return this;
}
/**
* Get bucket
* @return bucket
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_BUCKET)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getBucket() {
return bucket;
}
@JsonProperty(JSON_PROPERTY_BUCKET)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setBucket(String bucket) {
this.bucket = bucket;
}
public DominoDatasourceWebCheckDataSourceConnectionRequest catalog(String catalog) {
this.catalog = catalog;
return this;
}
/**
* Get catalog
* @return catalog
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_CATALOG)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getCatalog() {
return catalog;
}
@JsonProperty(JSON_PROPERTY_CATALOG)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCatalog(String catalog) {
this.catalog = catalog;
}
public DominoDatasourceWebCheckDataSourceConnectionRequest credentialType(CredentialTypeEnum credentialType) {
this.credentialType = credentialType;
return this;
}
/**
* Get credentialType
* @return credentialType
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_CREDENTIAL_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public CredentialTypeEnum getCredentialType() {
return credentialType;
}
@JsonProperty(JSON_PROPERTY_CREDENTIAL_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setCredentialType(CredentialTypeEnum credentialType) {
this.credentialType = credentialType;
}
public DominoDatasourceWebCheckDataSourceConnectionRequest dataSourceType(DataSourceTypeEnum dataSourceType) {
this.dataSourceType = dataSourceType;
return this;
}
/**
* Get dataSourceType
* @return dataSourceType
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_DATA_SOURCE_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public DataSourceTypeEnum getDataSourceType() {
return dataSourceType;
}
@JsonProperty(JSON_PROPERTY_DATA_SOURCE_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDataSourceType(DataSourceTypeEnum dataSourceType) {
this.dataSourceType = dataSourceType;
}
public DominoDatasourceWebCheckDataSourceConnectionRequest database(String database) {
this.database = database;
return this;
}
/**
* Get database
* @return database
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DATABASE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDatabase() {
return database;
}
@JsonProperty(JSON_PROPERTY_DATABASE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDatabase(String database) {
this.database = database;
}
public DominoDatasourceWebCheckDataSourceConnectionRequest host(String host) {
this.host = host;
return this;
}
/**
* Get host
* @return host
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_HOST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getHost() {
return host;
}
@JsonProperty(JSON_PROPERTY_HOST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setHost(String host) {
this.host = host;
}
public DominoDatasourceWebCheckDataSourceConnectionRequest port(String port) {
this.port = port;
return this;
}
/**
* Get port
* @return port
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_PORT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getPort() {
return port;
}
@JsonProperty(JSON_PROPERTY_PORT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPort(String port) {
this.port = port;
}
public DominoDatasourceWebCheckDataSourceConnectionRequest project(String project) {
this.project = project;
return this;
}
/**
* Get project
* @return project
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_PROJECT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getProject() {
return project;
}
@JsonProperty(JSON_PROPERTY_PROJECT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setProject(String project) {
this.project = project;
}
public DominoDatasourceWebCheckDataSourceConnectionRequest region(String region) {
this.region = region;
return this;
}
/**
* Get region
* @return region
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_REGION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getRegion() {
return region;
}
@JsonProperty(JSON_PROPERTY_REGION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRegion(String region) {
this.region = region;
}
public DominoDatasourceWebCheckDataSourceConnectionRequest role(String role) {
this.role = role;
return this;
}
/**
* Get role
* @return role
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_ROLE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getRole() {
return role;
}
@JsonProperty(JSON_PROPERTY_ROLE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRole(String role) {
this.role = role;
}
public DominoDatasourceWebCheckDataSourceConnectionRequest schema(String schema) {
this.schema = schema;
return this;
}
/**
* Get schema
* @return schema
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_SCHEMA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getSchema() {
return schema;
}
@JsonProperty(JSON_PROPERTY_SCHEMA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSchema(String schema) {
this.schema = schema;
}
public DominoDatasourceWebCheckDataSourceConnectionRequest secretCredential(String secretCredential) {
this.secretCredential = secretCredential;
return this;
}
/**
* Get secretCredential
* @return secretCredential
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_SECRET_CREDENTIAL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getSecretCredential() {
return secretCredential;
}
@JsonProperty(JSON_PROPERTY_SECRET_CREDENTIAL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSecretCredential(String secretCredential) {
this.secretCredential = secretCredential;
}
public DominoDatasourceWebCheckDataSourceConnectionRequest visibleCredential(String visibleCredential) {
this.visibleCredential = visibleCredential;
return this;
}
/**
* Get visibleCredential
* @return visibleCredential
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_VISIBLE_CREDENTIAL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getVisibleCredential() {
return visibleCredential;
}
@JsonProperty(JSON_PROPERTY_VISIBLE_CREDENTIAL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setVisibleCredential(String visibleCredential) {
this.visibleCredential = visibleCredential;
}
public DominoDatasourceWebCheckDataSourceConnectionRequest warehouse(String warehouse) {
this.warehouse = warehouse;
return this;
}
/**
* Get warehouse
* @return warehouse
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_WAREHOUSE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getWarehouse() {
return warehouse;
}
@JsonProperty(JSON_PROPERTY_WAREHOUSE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setWarehouse(String warehouse) {
this.warehouse = warehouse;
}
/**
* Return true if this domino.datasource.web.CheckDataSourceConnectionRequest object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DominoDatasourceWebCheckDataSourceConnectionRequest dominoDatasourceWebCheckDataSourceConnectionRequest = (DominoDatasourceWebCheckDataSourceConnectionRequest) o;
return Objects.equals(this.accountID, dominoDatasourceWebCheckDataSourceConnectionRequest.accountID) &&
Objects.equals(this.accountName, dominoDatasourceWebCheckDataSourceConnectionRequest.accountName) &&
Objects.equals(this.authType, dominoDatasourceWebCheckDataSourceConnectionRequest.authType) &&
Objects.equals(this.bucket, dominoDatasourceWebCheckDataSourceConnectionRequest.bucket) &&
Objects.equals(this.catalog, dominoDatasourceWebCheckDataSourceConnectionRequest.catalog) &&
Objects.equals(this.credentialType, dominoDatasourceWebCheckDataSourceConnectionRequest.credentialType) &&
Objects.equals(this.dataSourceType, dominoDatasourceWebCheckDataSourceConnectionRequest.dataSourceType) &&
Objects.equals(this.database, dominoDatasourceWebCheckDataSourceConnectionRequest.database) &&
Objects.equals(this.host, dominoDatasourceWebCheckDataSourceConnectionRequest.host) &&
Objects.equals(this.port, dominoDatasourceWebCheckDataSourceConnectionRequest.port) &&
Objects.equals(this.project, dominoDatasourceWebCheckDataSourceConnectionRequest.project) &&
Objects.equals(this.region, dominoDatasourceWebCheckDataSourceConnectionRequest.region) &&
Objects.equals(this.role, dominoDatasourceWebCheckDataSourceConnectionRequest.role) &&
Objects.equals(this.schema, dominoDatasourceWebCheckDataSourceConnectionRequest.schema) &&
Objects.equals(this.secretCredential, dominoDatasourceWebCheckDataSourceConnectionRequest.secretCredential) &&
Objects.equals(this.visibleCredential, dominoDatasourceWebCheckDataSourceConnectionRequest.visibleCredential) &&
Objects.equals(this.warehouse, dominoDatasourceWebCheckDataSourceConnectionRequest.warehouse);
}
@Override
public int hashCode() {
return Objects.hash(accountID, accountName, authType, bucket, catalog, credentialType, dataSourceType, database, host, port, project, region, role, schema, secretCredential, visibleCredential, warehouse);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DominoDatasourceWebCheckDataSourceConnectionRequest {\n");
sb.append(" accountID: ").append(toIndentedString(accountID)).append("\n");
sb.append(" accountName: ").append(toIndentedString(accountName)).append("\n");
sb.append(" authType: ").append(toIndentedString(authType)).append("\n");
sb.append(" bucket: ").append(toIndentedString(bucket)).append("\n");
sb.append(" catalog: ").append(toIndentedString(catalog)).append("\n");
sb.append(" credentialType: ").append(toIndentedString(credentialType)).append("\n");
sb.append(" dataSourceType: ").append(toIndentedString(dataSourceType)).append("\n");
sb.append(" database: ").append(toIndentedString(database)).append("\n");
sb.append(" host: ").append(toIndentedString(host)).append("\n");
sb.append(" port: ").append(toIndentedString(port)).append("\n");
sb.append(" project: ").append(toIndentedString(project)).append("\n");
sb.append(" region: ").append(toIndentedString(region)).append("\n");
sb.append(" role: ").append(toIndentedString(role)).append("\n");
sb.append(" schema: ").append(toIndentedString(schema)).append("\n");
sb.append(" secretCredential: ").append(toIndentedString(secretCredential)).append("\n");
sb.append(" visibleCredential: ").append(toIndentedString(visibleCredential)).append("\n");
sb.append(" warehouse: ").append(toIndentedString(warehouse)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `accountID` to the URL query string
if (getAccountID() != null) {
joiner.add(String.format("%saccountID%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getAccountID()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `accountName` to the URL query string
if (getAccountName() != null) {
joiner.add(String.format("%saccountName%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getAccountName()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `authType` to the URL query string
if (getAuthType() != null) {
joiner.add(String.format("%sauthType%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getAuthType()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `bucket` to the URL query string
if (getBucket() != null) {
joiner.add(String.format("%sbucket%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getBucket()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `catalog` to the URL query string
if (getCatalog() != null) {
joiner.add(String.format("%scatalog%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getCatalog()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `credentialType` to the URL query string
if (getCredentialType() != null) {
joiner.add(String.format("%scredentialType%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getCredentialType()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `dataSourceType` to the URL query string
if (getDataSourceType() != null) {
joiner.add(String.format("%sdataSourceType%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getDataSourceType()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `database` to the URL query string
if (getDatabase() != null) {
joiner.add(String.format("%sdatabase%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getDatabase()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `host` to the URL query string
if (getHost() != null) {
joiner.add(String.format("%shost%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getHost()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `port` to the URL query string
if (getPort() != null) {
joiner.add(String.format("%sport%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getPort()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `project` to the URL query string
if (getProject() != null) {
joiner.add(String.format("%sproject%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getProject()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `region` to the URL query string
if (getRegion() != null) {
joiner.add(String.format("%sregion%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getRegion()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `role` to the URL query string
if (getRole() != null) {
joiner.add(String.format("%srole%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getRole()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `schema` to the URL query string
if (getSchema() != null) {
joiner.add(String.format("%sschema%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getSchema()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `secretCredential` to the URL query string
if (getSecretCredential() != null) {
joiner.add(String.format("%ssecretCredential%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getSecretCredential()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `visibleCredential` to the URL query string
if (getVisibleCredential() != null) {
joiner.add(String.format("%svisibleCredential%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getVisibleCredential()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `warehouse` to the URL query string
if (getWarehouse() != null) {
joiner.add(String.format("%swarehouse%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getWarehouse()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy