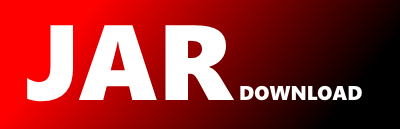
com.dominodatalab.api.model.DominoHardwaretierApiNewHardwareTierDto Maven / Gradle / Ivy
/*
* Domino Data Lab API v4
* This API is going to provide access to all the Domino functions available in the user interface. To authenticate your requests, include your API Key (which you can find on your account page) with the header X-Domino-Api-Key.
*
* The version of the OpenAPI document: 4.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.api.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.dominodatalab.api.model.DominoCommonExecutorRunRunMemoryLimitDto;
import com.dominodatalab.api.model.DominoHardwaretierApiHardwareTierComputeClusterRestrictionsDto;
import com.dominodatalab.api.model.DominoHardwaretierApiHardwareTierGpuConfigurationDto;
import com.dominodatalab.api.model.DominoHardwaretierApiHardwareTierOverprovisioningDto;
import com.dominodatalab.api.model.DominoHardwaretierApiHardwareTierPodCustomizationDto;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* DominoHardwaretierApiNewHardwareTierDto
*/
@JsonPropertyOrder({
DominoHardwaretierApiNewHardwareTierDto.JSON_PROPERTY_ID,
DominoHardwaretierApiNewHardwareTierDto.JSON_PROPERTY_NAME,
DominoHardwaretierApiNewHardwareTierDto.JSON_PROPERTY_CORES,
DominoHardwaretierApiNewHardwareTierDto.JSON_PROPERTY_CORES_LIMIT,
DominoHardwaretierApiNewHardwareTierDto.JSON_PROPERTY_MEMORY,
DominoHardwaretierApiNewHardwareTierDto.JSON_PROPERTY_ALLOW_SHARED_MEMORY_TO_EXCEED_DEFAULT,
DominoHardwaretierApiNewHardwareTierDto.JSON_PROPERTY_CLUSTER_TYPE,
DominoHardwaretierApiNewHardwareTierDto.JSON_PROPERTY_GPU_CONFIGURATION,
DominoHardwaretierApiNewHardwareTierDto.JSON_PROPERTY_RUN_MEMORY_LIMIT,
DominoHardwaretierApiNewHardwareTierDto.JSON_PROPERTY_IS_DEFAULT,
DominoHardwaretierApiNewHardwareTierDto.JSON_PROPERTY_CENTS_PER_MINUTE,
DominoHardwaretierApiNewHardwareTierDto.JSON_PROPERTY_IS_FREE,
DominoHardwaretierApiNewHardwareTierDto.JSON_PROPERTY_IS_ALLOWED_DURING_TRIAL,
DominoHardwaretierApiNewHardwareTierDto.JSON_PROPERTY_IS_VISIBLE,
DominoHardwaretierApiNewHardwareTierDto.JSON_PROPERTY_IS_GLOBAL,
DominoHardwaretierApiNewHardwareTierDto.JSON_PROPERTY_NODE_POOL,
DominoHardwaretierApiNewHardwareTierDto.JSON_PROPERTY_COMPUTE_CLUSTER_RESTRICTIONS,
DominoHardwaretierApiNewHardwareTierDto.JSON_PROPERTY_MAX_SIMULTANEOUS_EXECUTIONS,
DominoHardwaretierApiNewHardwareTierDto.JSON_PROPERTY_OVERPROVISIONING,
DominoHardwaretierApiNewHardwareTierDto.JSON_PROPERTY_POD_CUSTOMIZATION,
DominoHardwaretierApiNewHardwareTierDto.JSON_PROPERTY_DATA_PLANE_ID,
DominoHardwaretierApiNewHardwareTierDto.JSON_PROPERTY_IS_DATA_ANALYST_TIER,
DominoHardwaretierApiNewHardwareTierDto.JSON_PROPERTY_TAGS,
DominoHardwaretierApiNewHardwareTierDto.JSON_PROPERTY_AVAILABILITY_ZONES,
DominoHardwaretierApiNewHardwareTierDto.JSON_PROPERTY_SHARED_MEMORY_LIMIT_GI_B
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2023-10-17T15:20:46.682098100-04:00[America/New_York]")
public class DominoHardwaretierApiNewHardwareTierDto {
public static final String JSON_PROPERTY_ID = "id";
private String id;
public static final String JSON_PROPERTY_NAME = "name";
private String name;
public static final String JSON_PROPERTY_CORES = "cores";
private Double cores;
public static final String JSON_PROPERTY_CORES_LIMIT = "coresLimit";
private Double coresLimit;
public static final String JSON_PROPERTY_MEMORY = "memory";
private Double memory;
public static final String JSON_PROPERTY_ALLOW_SHARED_MEMORY_TO_EXCEED_DEFAULT = "allowSharedMemoryToExceedDefault";
private Boolean allowSharedMemoryToExceedDefault;
/**
* Gets or Sets clusterType
*/
public enum ClusterTypeEnum {
CLASSICONPREMISES("ClassicOnPremises"),
CLASSICAWS("ClassicAWS"),
KUBERNETES("Kubernetes");
private String value;
ClusterTypeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static ClusterTypeEnum fromValue(String value) {
for (ClusterTypeEnum b : ClusterTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return null;
}
}
public static final String JSON_PROPERTY_CLUSTER_TYPE = "clusterType";
private ClusterTypeEnum clusterType;
public static final String JSON_PROPERTY_GPU_CONFIGURATION = "gpuConfiguration";
private DominoHardwaretierApiHardwareTierGpuConfigurationDto gpuConfiguration;
public static final String JSON_PROPERTY_RUN_MEMORY_LIMIT = "runMemoryLimit";
private DominoCommonExecutorRunRunMemoryLimitDto runMemoryLimit;
public static final String JSON_PROPERTY_IS_DEFAULT = "isDefault";
private Boolean isDefault;
public static final String JSON_PROPERTY_CENTS_PER_MINUTE = "centsPerMinute";
private Double centsPerMinute;
public static final String JSON_PROPERTY_IS_FREE = "isFree";
private Boolean isFree;
public static final String JSON_PROPERTY_IS_ALLOWED_DURING_TRIAL = "isAllowedDuringTrial";
private Boolean isAllowedDuringTrial;
public static final String JSON_PROPERTY_IS_VISIBLE = "isVisible";
private Boolean isVisible;
public static final String JSON_PROPERTY_IS_GLOBAL = "isGlobal";
private Boolean isGlobal;
public static final String JSON_PROPERTY_NODE_POOL = "nodePool";
private String nodePool;
public static final String JSON_PROPERTY_COMPUTE_CLUSTER_RESTRICTIONS = "computeClusterRestrictions";
private DominoHardwaretierApiHardwareTierComputeClusterRestrictionsDto computeClusterRestrictions;
public static final String JSON_PROPERTY_MAX_SIMULTANEOUS_EXECUTIONS = "maxSimultaneousExecutions";
private Integer maxSimultaneousExecutions;
public static final String JSON_PROPERTY_OVERPROVISIONING = "overprovisioning";
private DominoHardwaretierApiHardwareTierOverprovisioningDto overprovisioning;
public static final String JSON_PROPERTY_POD_CUSTOMIZATION = "podCustomization";
private DominoHardwaretierApiHardwareTierPodCustomizationDto podCustomization;
public static final String JSON_PROPERTY_DATA_PLANE_ID = "dataPlaneId";
private String dataPlaneId;
public static final String JSON_PROPERTY_IS_DATA_ANALYST_TIER = "isDataAnalystTier";
private Boolean isDataAnalystTier;
public static final String JSON_PROPERTY_TAGS = "tags";
private List tags;
public static final String JSON_PROPERTY_AVAILABILITY_ZONES = "availabilityZones";
private List availabilityZones;
public static final String JSON_PROPERTY_SHARED_MEMORY_LIMIT_GI_B = "sharedMemoryLimitGiB";
private Double sharedMemoryLimitGiB;
public DominoHardwaretierApiNewHardwareTierDto() {
}
public DominoHardwaretierApiNewHardwareTierDto id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getId() {
return id;
}
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setId(String id) {
this.id = id;
}
public DominoHardwaretierApiNewHardwareTierDto name(String name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getName() {
return name;
}
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setName(String name) {
this.name = name;
}
public DominoHardwaretierApiNewHardwareTierDto cores(Double cores) {
this.cores = cores;
return this;
}
/**
* Get cores
* @return cores
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_CORES)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Double getCores() {
return cores;
}
@JsonProperty(JSON_PROPERTY_CORES)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setCores(Double cores) {
this.cores = cores;
}
public DominoHardwaretierApiNewHardwareTierDto coresLimit(Double coresLimit) {
this.coresLimit = coresLimit;
return this;
}
/**
* Get coresLimit
* @return coresLimit
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_CORES_LIMIT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getCoresLimit() {
return coresLimit;
}
@JsonProperty(JSON_PROPERTY_CORES_LIMIT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCoresLimit(Double coresLimit) {
this.coresLimit = coresLimit;
}
public DominoHardwaretierApiNewHardwareTierDto memory(Double memory) {
this.memory = memory;
return this;
}
/**
* Get memory
* @return memory
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_MEMORY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Double getMemory() {
return memory;
}
@JsonProperty(JSON_PROPERTY_MEMORY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setMemory(Double memory) {
this.memory = memory;
}
public DominoHardwaretierApiNewHardwareTierDto allowSharedMemoryToExceedDefault(Boolean allowSharedMemoryToExceedDefault) {
this.allowSharedMemoryToExceedDefault = allowSharedMemoryToExceedDefault;
return this;
}
/**
* Get allowSharedMemoryToExceedDefault
* @return allowSharedMemoryToExceedDefault
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ALLOW_SHARED_MEMORY_TO_EXCEED_DEFAULT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getAllowSharedMemoryToExceedDefault() {
return allowSharedMemoryToExceedDefault;
}
@JsonProperty(JSON_PROPERTY_ALLOW_SHARED_MEMORY_TO_EXCEED_DEFAULT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setAllowSharedMemoryToExceedDefault(Boolean allowSharedMemoryToExceedDefault) {
this.allowSharedMemoryToExceedDefault = allowSharedMemoryToExceedDefault;
}
public DominoHardwaretierApiNewHardwareTierDto clusterType(ClusterTypeEnum clusterType) {
this.clusterType = clusterType;
return this;
}
/**
* Get clusterType
* @return clusterType
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_CLUSTER_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ClusterTypeEnum getClusterType() {
return clusterType;
}
@JsonProperty(JSON_PROPERTY_CLUSTER_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setClusterType(ClusterTypeEnum clusterType) {
this.clusterType = clusterType;
}
public DominoHardwaretierApiNewHardwareTierDto gpuConfiguration(DominoHardwaretierApiHardwareTierGpuConfigurationDto gpuConfiguration) {
this.gpuConfiguration = gpuConfiguration;
return this;
}
/**
* Get gpuConfiguration
* @return gpuConfiguration
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_GPU_CONFIGURATION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DominoHardwaretierApiHardwareTierGpuConfigurationDto getGpuConfiguration() {
return gpuConfiguration;
}
@JsonProperty(JSON_PROPERTY_GPU_CONFIGURATION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setGpuConfiguration(DominoHardwaretierApiHardwareTierGpuConfigurationDto gpuConfiguration) {
this.gpuConfiguration = gpuConfiguration;
}
public DominoHardwaretierApiNewHardwareTierDto runMemoryLimit(DominoCommonExecutorRunRunMemoryLimitDto runMemoryLimit) {
this.runMemoryLimit = runMemoryLimit;
return this;
}
/**
* Get runMemoryLimit
* @return runMemoryLimit
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_RUN_MEMORY_LIMIT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DominoCommonExecutorRunRunMemoryLimitDto getRunMemoryLimit() {
return runMemoryLimit;
}
@JsonProperty(JSON_PROPERTY_RUN_MEMORY_LIMIT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRunMemoryLimit(DominoCommonExecutorRunRunMemoryLimitDto runMemoryLimit) {
this.runMemoryLimit = runMemoryLimit;
}
public DominoHardwaretierApiNewHardwareTierDto isDefault(Boolean isDefault) {
this.isDefault = isDefault;
return this;
}
/**
* Get isDefault
* @return isDefault
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_IS_DEFAULT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getIsDefault() {
return isDefault;
}
@JsonProperty(JSON_PROPERTY_IS_DEFAULT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setIsDefault(Boolean isDefault) {
this.isDefault = isDefault;
}
public DominoHardwaretierApiNewHardwareTierDto centsPerMinute(Double centsPerMinute) {
this.centsPerMinute = centsPerMinute;
return this;
}
/**
* Get centsPerMinute
* @return centsPerMinute
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_CENTS_PER_MINUTE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Double getCentsPerMinute() {
return centsPerMinute;
}
@JsonProperty(JSON_PROPERTY_CENTS_PER_MINUTE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setCentsPerMinute(Double centsPerMinute) {
this.centsPerMinute = centsPerMinute;
}
public DominoHardwaretierApiNewHardwareTierDto isFree(Boolean isFree) {
this.isFree = isFree;
return this;
}
/**
* Get isFree
* @return isFree
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_IS_FREE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getIsFree() {
return isFree;
}
@JsonProperty(JSON_PROPERTY_IS_FREE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setIsFree(Boolean isFree) {
this.isFree = isFree;
}
public DominoHardwaretierApiNewHardwareTierDto isAllowedDuringTrial(Boolean isAllowedDuringTrial) {
this.isAllowedDuringTrial = isAllowedDuringTrial;
return this;
}
/**
* Get isAllowedDuringTrial
* @return isAllowedDuringTrial
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_IS_ALLOWED_DURING_TRIAL)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getIsAllowedDuringTrial() {
return isAllowedDuringTrial;
}
@JsonProperty(JSON_PROPERTY_IS_ALLOWED_DURING_TRIAL)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setIsAllowedDuringTrial(Boolean isAllowedDuringTrial) {
this.isAllowedDuringTrial = isAllowedDuringTrial;
}
public DominoHardwaretierApiNewHardwareTierDto isVisible(Boolean isVisible) {
this.isVisible = isVisible;
return this;
}
/**
* Get isVisible
* @return isVisible
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_IS_VISIBLE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getIsVisible() {
return isVisible;
}
@JsonProperty(JSON_PROPERTY_IS_VISIBLE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setIsVisible(Boolean isVisible) {
this.isVisible = isVisible;
}
public DominoHardwaretierApiNewHardwareTierDto isGlobal(Boolean isGlobal) {
this.isGlobal = isGlobal;
return this;
}
/**
* Get isGlobal
* @return isGlobal
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_IS_GLOBAL)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getIsGlobal() {
return isGlobal;
}
@JsonProperty(JSON_PROPERTY_IS_GLOBAL)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setIsGlobal(Boolean isGlobal) {
this.isGlobal = isGlobal;
}
public DominoHardwaretierApiNewHardwareTierDto nodePool(String nodePool) {
this.nodePool = nodePool;
return this;
}
/**
* Get nodePool
* @return nodePool
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_NODE_POOL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getNodePool() {
return nodePool;
}
@JsonProperty(JSON_PROPERTY_NODE_POOL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setNodePool(String nodePool) {
this.nodePool = nodePool;
}
public DominoHardwaretierApiNewHardwareTierDto computeClusterRestrictions(DominoHardwaretierApiHardwareTierComputeClusterRestrictionsDto computeClusterRestrictions) {
this.computeClusterRestrictions = computeClusterRestrictions;
return this;
}
/**
* Get computeClusterRestrictions
* @return computeClusterRestrictions
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_COMPUTE_CLUSTER_RESTRICTIONS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public DominoHardwaretierApiHardwareTierComputeClusterRestrictionsDto getComputeClusterRestrictions() {
return computeClusterRestrictions;
}
@JsonProperty(JSON_PROPERTY_COMPUTE_CLUSTER_RESTRICTIONS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setComputeClusterRestrictions(DominoHardwaretierApiHardwareTierComputeClusterRestrictionsDto computeClusterRestrictions) {
this.computeClusterRestrictions = computeClusterRestrictions;
}
public DominoHardwaretierApiNewHardwareTierDto maxSimultaneousExecutions(Integer maxSimultaneousExecutions) {
this.maxSimultaneousExecutions = maxSimultaneousExecutions;
return this;
}
/**
* Get maxSimultaneousExecutions
* @return maxSimultaneousExecutions
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_MAX_SIMULTANEOUS_EXECUTIONS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getMaxSimultaneousExecutions() {
return maxSimultaneousExecutions;
}
@JsonProperty(JSON_PROPERTY_MAX_SIMULTANEOUS_EXECUTIONS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMaxSimultaneousExecutions(Integer maxSimultaneousExecutions) {
this.maxSimultaneousExecutions = maxSimultaneousExecutions;
}
public DominoHardwaretierApiNewHardwareTierDto overprovisioning(DominoHardwaretierApiHardwareTierOverprovisioningDto overprovisioning) {
this.overprovisioning = overprovisioning;
return this;
}
/**
* Get overprovisioning
* @return overprovisioning
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_OVERPROVISIONING)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DominoHardwaretierApiHardwareTierOverprovisioningDto getOverprovisioning() {
return overprovisioning;
}
@JsonProperty(JSON_PROPERTY_OVERPROVISIONING)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setOverprovisioning(DominoHardwaretierApiHardwareTierOverprovisioningDto overprovisioning) {
this.overprovisioning = overprovisioning;
}
public DominoHardwaretierApiNewHardwareTierDto podCustomization(DominoHardwaretierApiHardwareTierPodCustomizationDto podCustomization) {
this.podCustomization = podCustomization;
return this;
}
/**
* Get podCustomization
* @return podCustomization
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_POD_CUSTOMIZATION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public DominoHardwaretierApiHardwareTierPodCustomizationDto getPodCustomization() {
return podCustomization;
}
@JsonProperty(JSON_PROPERTY_POD_CUSTOMIZATION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setPodCustomization(DominoHardwaretierApiHardwareTierPodCustomizationDto podCustomization) {
this.podCustomization = podCustomization;
}
public DominoHardwaretierApiNewHardwareTierDto dataPlaneId(String dataPlaneId) {
this.dataPlaneId = dataPlaneId;
return this;
}
/**
* Get dataPlaneId
* @return dataPlaneId
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DATA_PLANE_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDataPlaneId() {
return dataPlaneId;
}
@JsonProperty(JSON_PROPERTY_DATA_PLANE_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDataPlaneId(String dataPlaneId) {
this.dataPlaneId = dataPlaneId;
}
public DominoHardwaretierApiNewHardwareTierDto isDataAnalystTier(Boolean isDataAnalystTier) {
this.isDataAnalystTier = isDataAnalystTier;
return this;
}
/**
* Get isDataAnalystTier
* @return isDataAnalystTier
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_IS_DATA_ANALYST_TIER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getIsDataAnalystTier() {
return isDataAnalystTier;
}
@JsonProperty(JSON_PROPERTY_IS_DATA_ANALYST_TIER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setIsDataAnalystTier(Boolean isDataAnalystTier) {
this.isDataAnalystTier = isDataAnalystTier;
}
public DominoHardwaretierApiNewHardwareTierDto tags(List tags) {
this.tags = tags;
return this;
}
public DominoHardwaretierApiNewHardwareTierDto addTagsItem(String tagsItem) {
if (this.tags == null) {
this.tags = new ArrayList<>();
}
this.tags.add(tagsItem);
return this;
}
/**
* Get tags
* @return tags
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_TAGS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getTags() {
return tags;
}
@JsonProperty(JSON_PROPERTY_TAGS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTags(List tags) {
this.tags = tags;
}
public DominoHardwaretierApiNewHardwareTierDto availabilityZones(List availabilityZones) {
this.availabilityZones = availabilityZones;
return this;
}
public DominoHardwaretierApiNewHardwareTierDto addAvailabilityZonesItem(String availabilityZonesItem) {
if (this.availabilityZones == null) {
this.availabilityZones = new ArrayList<>();
}
this.availabilityZones.add(availabilityZonesItem);
return this;
}
/**
* Get availabilityZones
* @return availabilityZones
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_AVAILABILITY_ZONES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getAvailabilityZones() {
return availabilityZones;
}
@JsonProperty(JSON_PROPERTY_AVAILABILITY_ZONES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAvailabilityZones(List availabilityZones) {
this.availabilityZones = availabilityZones;
}
public DominoHardwaretierApiNewHardwareTierDto sharedMemoryLimitGiB(Double sharedMemoryLimitGiB) {
this.sharedMemoryLimitGiB = sharedMemoryLimitGiB;
return this;
}
/**
* Get sharedMemoryLimitGiB
* @return sharedMemoryLimitGiB
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_SHARED_MEMORY_LIMIT_GI_B)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Double getSharedMemoryLimitGiB() {
return sharedMemoryLimitGiB;
}
@JsonProperty(JSON_PROPERTY_SHARED_MEMORY_LIMIT_GI_B)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSharedMemoryLimitGiB(Double sharedMemoryLimitGiB) {
this.sharedMemoryLimitGiB = sharedMemoryLimitGiB;
}
/**
* Return true if this domino.hardwaretier.api.NewHardwareTierDto object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DominoHardwaretierApiNewHardwareTierDto dominoHardwaretierApiNewHardwareTierDto = (DominoHardwaretierApiNewHardwareTierDto) o;
return Objects.equals(this.id, dominoHardwaretierApiNewHardwareTierDto.id) &&
Objects.equals(this.name, dominoHardwaretierApiNewHardwareTierDto.name) &&
Objects.equals(this.cores, dominoHardwaretierApiNewHardwareTierDto.cores) &&
Objects.equals(this.coresLimit, dominoHardwaretierApiNewHardwareTierDto.coresLimit) &&
Objects.equals(this.memory, dominoHardwaretierApiNewHardwareTierDto.memory) &&
Objects.equals(this.allowSharedMemoryToExceedDefault, dominoHardwaretierApiNewHardwareTierDto.allowSharedMemoryToExceedDefault) &&
Objects.equals(this.clusterType, dominoHardwaretierApiNewHardwareTierDto.clusterType) &&
Objects.equals(this.gpuConfiguration, dominoHardwaretierApiNewHardwareTierDto.gpuConfiguration) &&
Objects.equals(this.runMemoryLimit, dominoHardwaretierApiNewHardwareTierDto.runMemoryLimit) &&
Objects.equals(this.isDefault, dominoHardwaretierApiNewHardwareTierDto.isDefault) &&
Objects.equals(this.centsPerMinute, dominoHardwaretierApiNewHardwareTierDto.centsPerMinute) &&
Objects.equals(this.isFree, dominoHardwaretierApiNewHardwareTierDto.isFree) &&
Objects.equals(this.isAllowedDuringTrial, dominoHardwaretierApiNewHardwareTierDto.isAllowedDuringTrial) &&
Objects.equals(this.isVisible, dominoHardwaretierApiNewHardwareTierDto.isVisible) &&
Objects.equals(this.isGlobal, dominoHardwaretierApiNewHardwareTierDto.isGlobal) &&
Objects.equals(this.nodePool, dominoHardwaretierApiNewHardwareTierDto.nodePool) &&
Objects.equals(this.computeClusterRestrictions, dominoHardwaretierApiNewHardwareTierDto.computeClusterRestrictions) &&
Objects.equals(this.maxSimultaneousExecutions, dominoHardwaretierApiNewHardwareTierDto.maxSimultaneousExecutions) &&
Objects.equals(this.overprovisioning, dominoHardwaretierApiNewHardwareTierDto.overprovisioning) &&
Objects.equals(this.podCustomization, dominoHardwaretierApiNewHardwareTierDto.podCustomization) &&
Objects.equals(this.dataPlaneId, dominoHardwaretierApiNewHardwareTierDto.dataPlaneId) &&
Objects.equals(this.isDataAnalystTier, dominoHardwaretierApiNewHardwareTierDto.isDataAnalystTier) &&
Objects.equals(this.tags, dominoHardwaretierApiNewHardwareTierDto.tags) &&
Objects.equals(this.availabilityZones, dominoHardwaretierApiNewHardwareTierDto.availabilityZones) &&
Objects.equals(this.sharedMemoryLimitGiB, dominoHardwaretierApiNewHardwareTierDto.sharedMemoryLimitGiB);
}
@Override
public int hashCode() {
return Objects.hash(id, name, cores, coresLimit, memory, allowSharedMemoryToExceedDefault, clusterType, gpuConfiguration, runMemoryLimit, isDefault, centsPerMinute, isFree, isAllowedDuringTrial, isVisible, isGlobal, nodePool, computeClusterRestrictions, maxSimultaneousExecutions, overprovisioning, podCustomization, dataPlaneId, isDataAnalystTier, tags, availabilityZones, sharedMemoryLimitGiB);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DominoHardwaretierApiNewHardwareTierDto {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" cores: ").append(toIndentedString(cores)).append("\n");
sb.append(" coresLimit: ").append(toIndentedString(coresLimit)).append("\n");
sb.append(" memory: ").append(toIndentedString(memory)).append("\n");
sb.append(" allowSharedMemoryToExceedDefault: ").append(toIndentedString(allowSharedMemoryToExceedDefault)).append("\n");
sb.append(" clusterType: ").append(toIndentedString(clusterType)).append("\n");
sb.append(" gpuConfiguration: ").append(toIndentedString(gpuConfiguration)).append("\n");
sb.append(" runMemoryLimit: ").append(toIndentedString(runMemoryLimit)).append("\n");
sb.append(" isDefault: ").append(toIndentedString(isDefault)).append("\n");
sb.append(" centsPerMinute: ").append(toIndentedString(centsPerMinute)).append("\n");
sb.append(" isFree: ").append(toIndentedString(isFree)).append("\n");
sb.append(" isAllowedDuringTrial: ").append(toIndentedString(isAllowedDuringTrial)).append("\n");
sb.append(" isVisible: ").append(toIndentedString(isVisible)).append("\n");
sb.append(" isGlobal: ").append(toIndentedString(isGlobal)).append("\n");
sb.append(" nodePool: ").append(toIndentedString(nodePool)).append("\n");
sb.append(" computeClusterRestrictions: ").append(toIndentedString(computeClusterRestrictions)).append("\n");
sb.append(" maxSimultaneousExecutions: ").append(toIndentedString(maxSimultaneousExecutions)).append("\n");
sb.append(" overprovisioning: ").append(toIndentedString(overprovisioning)).append("\n");
sb.append(" podCustomization: ").append(toIndentedString(podCustomization)).append("\n");
sb.append(" dataPlaneId: ").append(toIndentedString(dataPlaneId)).append("\n");
sb.append(" isDataAnalystTier: ").append(toIndentedString(isDataAnalystTier)).append("\n");
sb.append(" tags: ").append(toIndentedString(tags)).append("\n");
sb.append(" availabilityZones: ").append(toIndentedString(availabilityZones)).append("\n");
sb.append(" sharedMemoryLimitGiB: ").append(toIndentedString(sharedMemoryLimitGiB)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `id` to the URL query string
if (getId() != null) {
joiner.add(String.format("%sid%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `name` to the URL query string
if (getName() != null) {
joiner.add(String.format("%sname%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getName()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `cores` to the URL query string
if (getCores() != null) {
joiner.add(String.format("%scores%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getCores()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `coresLimit` to the URL query string
if (getCoresLimit() != null) {
joiner.add(String.format("%scoresLimit%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getCoresLimit()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `memory` to the URL query string
if (getMemory() != null) {
joiner.add(String.format("%smemory%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getMemory()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `allowSharedMemoryToExceedDefault` to the URL query string
if (getAllowSharedMemoryToExceedDefault() != null) {
joiner.add(String.format("%sallowSharedMemoryToExceedDefault%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getAllowSharedMemoryToExceedDefault()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `clusterType` to the URL query string
if (getClusterType() != null) {
joiner.add(String.format("%sclusterType%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getClusterType()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `gpuConfiguration` to the URL query string
if (getGpuConfiguration() != null) {
joiner.add(getGpuConfiguration().toUrlQueryString(prefix + "gpuConfiguration" + suffix));
}
// add `runMemoryLimit` to the URL query string
if (getRunMemoryLimit() != null) {
joiner.add(getRunMemoryLimit().toUrlQueryString(prefix + "runMemoryLimit" + suffix));
}
// add `isDefault` to the URL query string
if (getIsDefault() != null) {
joiner.add(String.format("%sisDefault%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getIsDefault()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `centsPerMinute` to the URL query string
if (getCentsPerMinute() != null) {
joiner.add(String.format("%scentsPerMinute%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getCentsPerMinute()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `isFree` to the URL query string
if (getIsFree() != null) {
joiner.add(String.format("%sisFree%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getIsFree()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `isAllowedDuringTrial` to the URL query string
if (getIsAllowedDuringTrial() != null) {
joiner.add(String.format("%sisAllowedDuringTrial%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getIsAllowedDuringTrial()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `isVisible` to the URL query string
if (getIsVisible() != null) {
joiner.add(String.format("%sisVisible%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getIsVisible()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `isGlobal` to the URL query string
if (getIsGlobal() != null) {
joiner.add(String.format("%sisGlobal%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getIsGlobal()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `nodePool` to the URL query string
if (getNodePool() != null) {
joiner.add(String.format("%snodePool%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getNodePool()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `computeClusterRestrictions` to the URL query string
if (getComputeClusterRestrictions() != null) {
joiner.add(getComputeClusterRestrictions().toUrlQueryString(prefix + "computeClusterRestrictions" + suffix));
}
// add `maxSimultaneousExecutions` to the URL query string
if (getMaxSimultaneousExecutions() != null) {
joiner.add(String.format("%smaxSimultaneousExecutions%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getMaxSimultaneousExecutions()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `overprovisioning` to the URL query string
if (getOverprovisioning() != null) {
joiner.add(getOverprovisioning().toUrlQueryString(prefix + "overprovisioning" + suffix));
}
// add `podCustomization` to the URL query string
if (getPodCustomization() != null) {
joiner.add(getPodCustomization().toUrlQueryString(prefix + "podCustomization" + suffix));
}
// add `dataPlaneId` to the URL query string
if (getDataPlaneId() != null) {
joiner.add(String.format("%sdataPlaneId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getDataPlaneId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `isDataAnalystTier` to the URL query string
if (getIsDataAnalystTier() != null) {
joiner.add(String.format("%sisDataAnalystTier%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getIsDataAnalystTier()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `tags` to the URL query string
if (getTags() != null) {
for (int i = 0; i < getTags().size(); i++) {
joiner.add(String.format("%stags%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getTags().get(i)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
// add `availabilityZones` to the URL query string
if (getAvailabilityZones() != null) {
for (int i = 0; i < getAvailabilityZones().size(); i++) {
joiner.add(String.format("%savailabilityZones%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getAvailabilityZones().get(i)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
// add `sharedMemoryLimitGiB` to the URL query string
if (getSharedMemoryLimitGiB() != null) {
joiner.add(String.format("%ssharedMemoryLimitGiB%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getSharedMemoryLimitGiB()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy