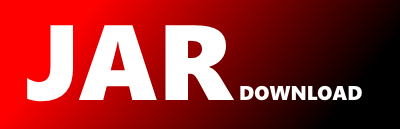
com.dominodatalab.api.model.DominoJobsInterfaceJob Maven / Gradle / Ivy
/*
* Domino Data Lab API v4
* This API is going to provide access to all the Domino functions available in the user interface. To authenticate your requests, include your API Key (which you can find on your account page) with the header X-Domino-Api-Key.
*
* The version of the OpenAPI document: 4.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.api.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.dominodatalab.api.model.DominoDataplaneDataPlaneDto;
import com.dominodatalab.api.model.DominoJobsInterfaceCommitDetails;
import com.dominodatalab.api.model.DominoJobsInterfaceComputeClusterConfigSpecDto;
import com.dominodatalab.api.model.DominoJobsInterfaceDependentDatasetMount;
import com.dominodatalab.api.model.DominoJobsInterfaceDependentExternalVolumeMount;
import com.dominodatalab.api.model.DominoJobsInterfaceDependentProject;
import com.dominodatalab.api.model.DominoJobsInterfaceDependentRepository;
import com.dominodatalab.api.model.DominoJobsInterfaceDominoStats;
import com.dominodatalab.api.model.DominoJobsInterfaceJobStartedBy;
import com.dominodatalab.api.model.DominoJobsInterfaceJobStatuses;
import com.dominodatalab.api.model.DominoJobsInterfaceJobUsageInJob;
import com.dominodatalab.api.model.DominoJobsInterfaceQueuedJobHistoryDetails;
import com.dominodatalab.api.model.DominoJobsInterfaceStageTime;
import com.dominodatalab.api.model.DominoJobsInterfaceTagApplication;
import com.dominodatalab.api.model.DominoProjectsApiRepositoriesReferenceDTO;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* DominoJobsInterfaceJob
*/
@JsonPropertyOrder({
DominoJobsInterfaceJob.JSON_PROPERTY_NUMBER,
DominoJobsInterfaceJob.JSON_PROPERTY_ID,
DominoJobsInterfaceJob.JSON_PROPERTY_QUEUED_JOB_HISTORY_DETAILS,
DominoJobsInterfaceJob.JSON_PROPERTY_STAGE_TIME,
DominoJobsInterfaceJob.JSON_PROPERTY_TAGS,
DominoJobsInterfaceJob.JSON_PROPERTY_JOB_RUN_COMMAND,
DominoJobsInterfaceJob.JSON_PROPERTY_USAGE,
DominoJobsInterfaceJob.JSON_PROPERTY_STARTED_BY,
DominoJobsInterfaceJob.JSON_PROPERTY_COMMENTS_COUNT,
DominoJobsInterfaceJob.JSON_PROPERTY_TITLE,
DominoJobsInterfaceJob.JSON_PROPERTY_COMMIT_DETAILS,
DominoJobsInterfaceJob.JSON_PROPERTY_DOMINO_STATS,
DominoJobsInterfaceJob.JSON_PROPERTY_STATUSES,
DominoJobsInterfaceJob.JSON_PROPERTY_MAIN_REPO_GIT_REF,
DominoJobsInterfaceJob.JSON_PROPERTY_DEPENDENT_REPOSITORIES,
DominoJobsInterfaceJob.JSON_PROPERTY_DEPENDENT_DATASET_MOUNTS,
DominoJobsInterfaceJob.JSON_PROPERTY_DEPENDENT_PROJECTS,
DominoJobsInterfaceJob.JSON_PROPERTY_SUGGEST_DATASETS,
DominoJobsInterfaceJob.JSON_PROPERTY_GOAL_IDS,
DominoJobsInterfaceJob.JSON_PROPERTY_RUN_LAUNCHER_ID,
DominoJobsInterfaceJob.JSON_PROPERTY_DEPENDENT_EXTERNAL_VOLUME_MOUNTS,
DominoJobsInterfaceJob.JSON_PROPERTY_COMPUTE_CLUSTER,
DominoJobsInterfaceJob.JSON_PROPERTY_DATA_PLANE,
DominoJobsInterfaceJob.JSON_PROPERTY_SNAPSHOT_DATASETS_ON_COMPLETION
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2023-10-17T15:20:46.682098100-04:00[America/New_York]")
public class DominoJobsInterfaceJob {
public static final String JSON_PROPERTY_NUMBER = "number";
private Integer number;
public static final String JSON_PROPERTY_ID = "id";
private String id;
public static final String JSON_PROPERTY_QUEUED_JOB_HISTORY_DETAILS = "queuedJobHistoryDetails";
private DominoJobsInterfaceQueuedJobHistoryDetails queuedJobHistoryDetails;
public static final String JSON_PROPERTY_STAGE_TIME = "stageTime";
private DominoJobsInterfaceStageTime stageTime;
public static final String JSON_PROPERTY_TAGS = "tags";
private List tags = new ArrayList<>();
public static final String JSON_PROPERTY_JOB_RUN_COMMAND = "jobRunCommand";
private String jobRunCommand;
public static final String JSON_PROPERTY_USAGE = "usage";
private DominoJobsInterfaceJobUsageInJob usage;
public static final String JSON_PROPERTY_STARTED_BY = "startedBy";
private DominoJobsInterfaceJobStartedBy startedBy;
public static final String JSON_PROPERTY_COMMENTS_COUNT = "commentsCount";
private Integer commentsCount;
public static final String JSON_PROPERTY_TITLE = "title";
private String title;
public static final String JSON_PROPERTY_COMMIT_DETAILS = "commitDetails";
private DominoJobsInterfaceCommitDetails commitDetails;
public static final String JSON_PROPERTY_DOMINO_STATS = "dominoStats";
private List dominoStats = new ArrayList<>();
public static final String JSON_PROPERTY_STATUSES = "statuses";
private DominoJobsInterfaceJobStatuses statuses;
public static final String JSON_PROPERTY_MAIN_REPO_GIT_REF = "mainRepoGitRef";
private DominoProjectsApiRepositoriesReferenceDTO mainRepoGitRef;
public static final String JSON_PROPERTY_DEPENDENT_REPOSITORIES = "dependentRepositories";
private List dependentRepositories = new ArrayList<>();
public static final String JSON_PROPERTY_DEPENDENT_DATASET_MOUNTS = "dependentDatasetMounts";
private List dependentDatasetMounts = new ArrayList<>();
public static final String JSON_PROPERTY_DEPENDENT_PROJECTS = "dependentProjects";
private List dependentProjects = new ArrayList<>();
public static final String JSON_PROPERTY_SUGGEST_DATASETS = "suggestDatasets";
private Boolean suggestDatasets;
public static final String JSON_PROPERTY_GOAL_IDS = "goalIds";
private List goalIds = new ArrayList<>();
public static final String JSON_PROPERTY_RUN_LAUNCHER_ID = "runLauncherId";
private String runLauncherId;
public static final String JSON_PROPERTY_DEPENDENT_EXTERNAL_VOLUME_MOUNTS = "dependentExternalVolumeMounts";
private List dependentExternalVolumeMounts = new ArrayList<>();
public static final String JSON_PROPERTY_COMPUTE_CLUSTER = "computeCluster";
private DominoJobsInterfaceComputeClusterConfigSpecDto computeCluster;
public static final String JSON_PROPERTY_DATA_PLANE = "dataPlane";
private DominoDataplaneDataPlaneDto dataPlane;
public static final String JSON_PROPERTY_SNAPSHOT_DATASETS_ON_COMPLETION = "snapshotDatasetsOnCompletion";
private Boolean snapshotDatasetsOnCompletion;
public DominoJobsInterfaceJob() {
}
public DominoJobsInterfaceJob number(Integer number) {
this.number = number;
return this;
}
/**
* Get number
* @return number
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_NUMBER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Integer getNumber() {
return number;
}
@JsonProperty(JSON_PROPERTY_NUMBER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setNumber(Integer number) {
this.number = number;
}
public DominoJobsInterfaceJob id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getId() {
return id;
}
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setId(String id) {
this.id = id;
}
public DominoJobsInterfaceJob queuedJobHistoryDetails(DominoJobsInterfaceQueuedJobHistoryDetails queuedJobHistoryDetails) {
this.queuedJobHistoryDetails = queuedJobHistoryDetails;
return this;
}
/**
* Get queuedJobHistoryDetails
* @return queuedJobHistoryDetails
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_QUEUED_JOB_HISTORY_DETAILS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DominoJobsInterfaceQueuedJobHistoryDetails getQueuedJobHistoryDetails() {
return queuedJobHistoryDetails;
}
@JsonProperty(JSON_PROPERTY_QUEUED_JOB_HISTORY_DETAILS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setQueuedJobHistoryDetails(DominoJobsInterfaceQueuedJobHistoryDetails queuedJobHistoryDetails) {
this.queuedJobHistoryDetails = queuedJobHistoryDetails;
}
public DominoJobsInterfaceJob stageTime(DominoJobsInterfaceStageTime stageTime) {
this.stageTime = stageTime;
return this;
}
/**
* Get stageTime
* @return stageTime
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_STAGE_TIME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public DominoJobsInterfaceStageTime getStageTime() {
return stageTime;
}
@JsonProperty(JSON_PROPERTY_STAGE_TIME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setStageTime(DominoJobsInterfaceStageTime stageTime) {
this.stageTime = stageTime;
}
public DominoJobsInterfaceJob tags(List tags) {
this.tags = tags;
return this;
}
public DominoJobsInterfaceJob addTagsItem(DominoJobsInterfaceTagApplication tagsItem) {
if (this.tags == null) {
this.tags = new ArrayList<>();
}
this.tags.add(tagsItem);
return this;
}
/**
* Get tags
* @return tags
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_TAGS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getTags() {
return tags;
}
@JsonProperty(JSON_PROPERTY_TAGS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setTags(List tags) {
this.tags = tags;
}
public DominoJobsInterfaceJob jobRunCommand(String jobRunCommand) {
this.jobRunCommand = jobRunCommand;
return this;
}
/**
* Get jobRunCommand
* @return jobRunCommand
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_JOB_RUN_COMMAND)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getJobRunCommand() {
return jobRunCommand;
}
@JsonProperty(JSON_PROPERTY_JOB_RUN_COMMAND)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setJobRunCommand(String jobRunCommand) {
this.jobRunCommand = jobRunCommand;
}
public DominoJobsInterfaceJob usage(DominoJobsInterfaceJobUsageInJob usage) {
this.usage = usage;
return this;
}
/**
* Get usage
* @return usage
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_USAGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DominoJobsInterfaceJobUsageInJob getUsage() {
return usage;
}
@JsonProperty(JSON_PROPERTY_USAGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUsage(DominoJobsInterfaceJobUsageInJob usage) {
this.usage = usage;
}
public DominoJobsInterfaceJob startedBy(DominoJobsInterfaceJobStartedBy startedBy) {
this.startedBy = startedBy;
return this;
}
/**
* Get startedBy
* @return startedBy
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_STARTED_BY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DominoJobsInterfaceJobStartedBy getStartedBy() {
return startedBy;
}
@JsonProperty(JSON_PROPERTY_STARTED_BY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setStartedBy(DominoJobsInterfaceJobStartedBy startedBy) {
this.startedBy = startedBy;
}
public DominoJobsInterfaceJob commentsCount(Integer commentsCount) {
this.commentsCount = commentsCount;
return this;
}
/**
* Get commentsCount
* @return commentsCount
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_COMMENTS_COUNT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Integer getCommentsCount() {
return commentsCount;
}
@JsonProperty(JSON_PROPERTY_COMMENTS_COUNT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setCommentsCount(Integer commentsCount) {
this.commentsCount = commentsCount;
}
public DominoJobsInterfaceJob title(String title) {
this.title = title;
return this;
}
/**
* Get title
* @return title
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_TITLE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getTitle() {
return title;
}
@JsonProperty(JSON_PROPERTY_TITLE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTitle(String title) {
this.title = title;
}
public DominoJobsInterfaceJob commitDetails(DominoJobsInterfaceCommitDetails commitDetails) {
this.commitDetails = commitDetails;
return this;
}
/**
* Get commitDetails
* @return commitDetails
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_COMMIT_DETAILS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public DominoJobsInterfaceCommitDetails getCommitDetails() {
return commitDetails;
}
@JsonProperty(JSON_PROPERTY_COMMIT_DETAILS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setCommitDetails(DominoJobsInterfaceCommitDetails commitDetails) {
this.commitDetails = commitDetails;
}
public DominoJobsInterfaceJob dominoStats(List dominoStats) {
this.dominoStats = dominoStats;
return this;
}
public DominoJobsInterfaceJob addDominoStatsItem(DominoJobsInterfaceDominoStats dominoStatsItem) {
if (this.dominoStats == null) {
this.dominoStats = new ArrayList<>();
}
this.dominoStats.add(dominoStatsItem);
return this;
}
/**
* Get dominoStats
* @return dominoStats
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_DOMINO_STATS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getDominoStats() {
return dominoStats;
}
@JsonProperty(JSON_PROPERTY_DOMINO_STATS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDominoStats(List dominoStats) {
this.dominoStats = dominoStats;
}
public DominoJobsInterfaceJob statuses(DominoJobsInterfaceJobStatuses statuses) {
this.statuses = statuses;
return this;
}
/**
* Get statuses
* @return statuses
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_STATUSES)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public DominoJobsInterfaceJobStatuses getStatuses() {
return statuses;
}
@JsonProperty(JSON_PROPERTY_STATUSES)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setStatuses(DominoJobsInterfaceJobStatuses statuses) {
this.statuses = statuses;
}
public DominoJobsInterfaceJob mainRepoGitRef(DominoProjectsApiRepositoriesReferenceDTO mainRepoGitRef) {
this.mainRepoGitRef = mainRepoGitRef;
return this;
}
/**
* Get mainRepoGitRef
* @return mainRepoGitRef
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_MAIN_REPO_GIT_REF)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DominoProjectsApiRepositoriesReferenceDTO getMainRepoGitRef() {
return mainRepoGitRef;
}
@JsonProperty(JSON_PROPERTY_MAIN_REPO_GIT_REF)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMainRepoGitRef(DominoProjectsApiRepositoriesReferenceDTO mainRepoGitRef) {
this.mainRepoGitRef = mainRepoGitRef;
}
public DominoJobsInterfaceJob dependentRepositories(List dependentRepositories) {
this.dependentRepositories = dependentRepositories;
return this;
}
public DominoJobsInterfaceJob addDependentRepositoriesItem(DominoJobsInterfaceDependentRepository dependentRepositoriesItem) {
if (this.dependentRepositories == null) {
this.dependentRepositories = new ArrayList<>();
}
this.dependentRepositories.add(dependentRepositoriesItem);
return this;
}
/**
* Get dependentRepositories
* @return dependentRepositories
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_DEPENDENT_REPOSITORIES)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getDependentRepositories() {
return dependentRepositories;
}
@JsonProperty(JSON_PROPERTY_DEPENDENT_REPOSITORIES)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDependentRepositories(List dependentRepositories) {
this.dependentRepositories = dependentRepositories;
}
public DominoJobsInterfaceJob dependentDatasetMounts(List dependentDatasetMounts) {
this.dependentDatasetMounts = dependentDatasetMounts;
return this;
}
public DominoJobsInterfaceJob addDependentDatasetMountsItem(DominoJobsInterfaceDependentDatasetMount dependentDatasetMountsItem) {
if (this.dependentDatasetMounts == null) {
this.dependentDatasetMounts = new ArrayList<>();
}
this.dependentDatasetMounts.add(dependentDatasetMountsItem);
return this;
}
/**
* Get dependentDatasetMounts
* @return dependentDatasetMounts
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_DEPENDENT_DATASET_MOUNTS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getDependentDatasetMounts() {
return dependentDatasetMounts;
}
@JsonProperty(JSON_PROPERTY_DEPENDENT_DATASET_MOUNTS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDependentDatasetMounts(List dependentDatasetMounts) {
this.dependentDatasetMounts = dependentDatasetMounts;
}
public DominoJobsInterfaceJob dependentProjects(List dependentProjects) {
this.dependentProjects = dependentProjects;
return this;
}
public DominoJobsInterfaceJob addDependentProjectsItem(DominoJobsInterfaceDependentProject dependentProjectsItem) {
if (this.dependentProjects == null) {
this.dependentProjects = new ArrayList<>();
}
this.dependentProjects.add(dependentProjectsItem);
return this;
}
/**
* Get dependentProjects
* @return dependentProjects
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_DEPENDENT_PROJECTS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getDependentProjects() {
return dependentProjects;
}
@JsonProperty(JSON_PROPERTY_DEPENDENT_PROJECTS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDependentProjects(List dependentProjects) {
this.dependentProjects = dependentProjects;
}
public DominoJobsInterfaceJob suggestDatasets(Boolean suggestDatasets) {
this.suggestDatasets = suggestDatasets;
return this;
}
/**
* Get suggestDatasets
* @return suggestDatasets
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_SUGGEST_DATASETS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getSuggestDatasets() {
return suggestDatasets;
}
@JsonProperty(JSON_PROPERTY_SUGGEST_DATASETS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setSuggestDatasets(Boolean suggestDatasets) {
this.suggestDatasets = suggestDatasets;
}
public DominoJobsInterfaceJob goalIds(List goalIds) {
this.goalIds = goalIds;
return this;
}
public DominoJobsInterfaceJob addGoalIdsItem(String goalIdsItem) {
if (this.goalIds == null) {
this.goalIds = new ArrayList<>();
}
this.goalIds.add(goalIdsItem);
return this;
}
/**
* Get goalIds
* @return goalIds
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_GOAL_IDS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getGoalIds() {
return goalIds;
}
@JsonProperty(JSON_PROPERTY_GOAL_IDS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setGoalIds(List goalIds) {
this.goalIds = goalIds;
}
public DominoJobsInterfaceJob runLauncherId(String runLauncherId) {
this.runLauncherId = runLauncherId;
return this;
}
/**
* Get runLauncherId
* @return runLauncherId
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_RUN_LAUNCHER_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getRunLauncherId() {
return runLauncherId;
}
@JsonProperty(JSON_PROPERTY_RUN_LAUNCHER_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRunLauncherId(String runLauncherId) {
this.runLauncherId = runLauncherId;
}
public DominoJobsInterfaceJob dependentExternalVolumeMounts(List dependentExternalVolumeMounts) {
this.dependentExternalVolumeMounts = dependentExternalVolumeMounts;
return this;
}
public DominoJobsInterfaceJob addDependentExternalVolumeMountsItem(DominoJobsInterfaceDependentExternalVolumeMount dependentExternalVolumeMountsItem) {
if (this.dependentExternalVolumeMounts == null) {
this.dependentExternalVolumeMounts = new ArrayList<>();
}
this.dependentExternalVolumeMounts.add(dependentExternalVolumeMountsItem);
return this;
}
/**
* Get dependentExternalVolumeMounts
* @return dependentExternalVolumeMounts
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_DEPENDENT_EXTERNAL_VOLUME_MOUNTS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getDependentExternalVolumeMounts() {
return dependentExternalVolumeMounts;
}
@JsonProperty(JSON_PROPERTY_DEPENDENT_EXTERNAL_VOLUME_MOUNTS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDependentExternalVolumeMounts(List dependentExternalVolumeMounts) {
this.dependentExternalVolumeMounts = dependentExternalVolumeMounts;
}
public DominoJobsInterfaceJob computeCluster(DominoJobsInterfaceComputeClusterConfigSpecDto computeCluster) {
this.computeCluster = computeCluster;
return this;
}
/**
* Get computeCluster
* @return computeCluster
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_COMPUTE_CLUSTER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DominoJobsInterfaceComputeClusterConfigSpecDto getComputeCluster() {
return computeCluster;
}
@JsonProperty(JSON_PROPERTY_COMPUTE_CLUSTER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setComputeCluster(DominoJobsInterfaceComputeClusterConfigSpecDto computeCluster) {
this.computeCluster = computeCluster;
}
public DominoJobsInterfaceJob dataPlane(DominoDataplaneDataPlaneDto dataPlane) {
this.dataPlane = dataPlane;
return this;
}
/**
* Get dataPlane
* @return dataPlane
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DATA_PLANE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DominoDataplaneDataPlaneDto getDataPlane() {
return dataPlane;
}
@JsonProperty(JSON_PROPERTY_DATA_PLANE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDataPlane(DominoDataplaneDataPlaneDto dataPlane) {
this.dataPlane = dataPlane;
}
public DominoJobsInterfaceJob snapshotDatasetsOnCompletion(Boolean snapshotDatasetsOnCompletion) {
this.snapshotDatasetsOnCompletion = snapshotDatasetsOnCompletion;
return this;
}
/**
* Get snapshotDatasetsOnCompletion
* @return snapshotDatasetsOnCompletion
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_SNAPSHOT_DATASETS_ON_COMPLETION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getSnapshotDatasetsOnCompletion() {
return snapshotDatasetsOnCompletion;
}
@JsonProperty(JSON_PROPERTY_SNAPSHOT_DATASETS_ON_COMPLETION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setSnapshotDatasetsOnCompletion(Boolean snapshotDatasetsOnCompletion) {
this.snapshotDatasetsOnCompletion = snapshotDatasetsOnCompletion;
}
/**
* Return true if this domino.jobs.interface.Job object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DominoJobsInterfaceJob dominoJobsInterfaceJob = (DominoJobsInterfaceJob) o;
return Objects.equals(this.number, dominoJobsInterfaceJob.number) &&
Objects.equals(this.id, dominoJobsInterfaceJob.id) &&
Objects.equals(this.queuedJobHistoryDetails, dominoJobsInterfaceJob.queuedJobHistoryDetails) &&
Objects.equals(this.stageTime, dominoJobsInterfaceJob.stageTime) &&
Objects.equals(this.tags, dominoJobsInterfaceJob.tags) &&
Objects.equals(this.jobRunCommand, dominoJobsInterfaceJob.jobRunCommand) &&
Objects.equals(this.usage, dominoJobsInterfaceJob.usage) &&
Objects.equals(this.startedBy, dominoJobsInterfaceJob.startedBy) &&
Objects.equals(this.commentsCount, dominoJobsInterfaceJob.commentsCount) &&
Objects.equals(this.title, dominoJobsInterfaceJob.title) &&
Objects.equals(this.commitDetails, dominoJobsInterfaceJob.commitDetails) &&
Objects.equals(this.dominoStats, dominoJobsInterfaceJob.dominoStats) &&
Objects.equals(this.statuses, dominoJobsInterfaceJob.statuses) &&
Objects.equals(this.mainRepoGitRef, dominoJobsInterfaceJob.mainRepoGitRef) &&
Objects.equals(this.dependentRepositories, dominoJobsInterfaceJob.dependentRepositories) &&
Objects.equals(this.dependentDatasetMounts, dominoJobsInterfaceJob.dependentDatasetMounts) &&
Objects.equals(this.dependentProjects, dominoJobsInterfaceJob.dependentProjects) &&
Objects.equals(this.suggestDatasets, dominoJobsInterfaceJob.suggestDatasets) &&
Objects.equals(this.goalIds, dominoJobsInterfaceJob.goalIds) &&
Objects.equals(this.runLauncherId, dominoJobsInterfaceJob.runLauncherId) &&
Objects.equals(this.dependentExternalVolumeMounts, dominoJobsInterfaceJob.dependentExternalVolumeMounts) &&
Objects.equals(this.computeCluster, dominoJobsInterfaceJob.computeCluster) &&
Objects.equals(this.dataPlane, dominoJobsInterfaceJob.dataPlane) &&
Objects.equals(this.snapshotDatasetsOnCompletion, dominoJobsInterfaceJob.snapshotDatasetsOnCompletion);
}
@Override
public int hashCode() {
return Objects.hash(number, id, queuedJobHistoryDetails, stageTime, tags, jobRunCommand, usage, startedBy, commentsCount, title, commitDetails, dominoStats, statuses, mainRepoGitRef, dependentRepositories, dependentDatasetMounts, dependentProjects, suggestDatasets, goalIds, runLauncherId, dependentExternalVolumeMounts, computeCluster, dataPlane, snapshotDatasetsOnCompletion);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DominoJobsInterfaceJob {\n");
sb.append(" number: ").append(toIndentedString(number)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" queuedJobHistoryDetails: ").append(toIndentedString(queuedJobHistoryDetails)).append("\n");
sb.append(" stageTime: ").append(toIndentedString(stageTime)).append("\n");
sb.append(" tags: ").append(toIndentedString(tags)).append("\n");
sb.append(" jobRunCommand: ").append(toIndentedString(jobRunCommand)).append("\n");
sb.append(" usage: ").append(toIndentedString(usage)).append("\n");
sb.append(" startedBy: ").append(toIndentedString(startedBy)).append("\n");
sb.append(" commentsCount: ").append(toIndentedString(commentsCount)).append("\n");
sb.append(" title: ").append(toIndentedString(title)).append("\n");
sb.append(" commitDetails: ").append(toIndentedString(commitDetails)).append("\n");
sb.append(" dominoStats: ").append(toIndentedString(dominoStats)).append("\n");
sb.append(" statuses: ").append(toIndentedString(statuses)).append("\n");
sb.append(" mainRepoGitRef: ").append(toIndentedString(mainRepoGitRef)).append("\n");
sb.append(" dependentRepositories: ").append(toIndentedString(dependentRepositories)).append("\n");
sb.append(" dependentDatasetMounts: ").append(toIndentedString(dependentDatasetMounts)).append("\n");
sb.append(" dependentProjects: ").append(toIndentedString(dependentProjects)).append("\n");
sb.append(" suggestDatasets: ").append(toIndentedString(suggestDatasets)).append("\n");
sb.append(" goalIds: ").append(toIndentedString(goalIds)).append("\n");
sb.append(" runLauncherId: ").append(toIndentedString(runLauncherId)).append("\n");
sb.append(" dependentExternalVolumeMounts: ").append(toIndentedString(dependentExternalVolumeMounts)).append("\n");
sb.append(" computeCluster: ").append(toIndentedString(computeCluster)).append("\n");
sb.append(" dataPlane: ").append(toIndentedString(dataPlane)).append("\n");
sb.append(" snapshotDatasetsOnCompletion: ").append(toIndentedString(snapshotDatasetsOnCompletion)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `number` to the URL query string
if (getNumber() != null) {
joiner.add(String.format("%snumber%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getNumber()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `id` to the URL query string
if (getId() != null) {
joiner.add(String.format("%sid%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `queuedJobHistoryDetails` to the URL query string
if (getQueuedJobHistoryDetails() != null) {
joiner.add(getQueuedJobHistoryDetails().toUrlQueryString(prefix + "queuedJobHistoryDetails" + suffix));
}
// add `stageTime` to the URL query string
if (getStageTime() != null) {
joiner.add(getStageTime().toUrlQueryString(prefix + "stageTime" + suffix));
}
// add `tags` to the URL query string
if (getTags() != null) {
for (int i = 0; i < getTags().size(); i++) {
if (getTags().get(i) != null) {
joiner.add(getTags().get(i).toUrlQueryString(String.format("%stags%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `jobRunCommand` to the URL query string
if (getJobRunCommand() != null) {
joiner.add(String.format("%sjobRunCommand%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getJobRunCommand()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `usage` to the URL query string
if (getUsage() != null) {
joiner.add(getUsage().toUrlQueryString(prefix + "usage" + suffix));
}
// add `startedBy` to the URL query string
if (getStartedBy() != null) {
joiner.add(getStartedBy().toUrlQueryString(prefix + "startedBy" + suffix));
}
// add `commentsCount` to the URL query string
if (getCommentsCount() != null) {
joiner.add(String.format("%scommentsCount%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getCommentsCount()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `title` to the URL query string
if (getTitle() != null) {
joiner.add(String.format("%stitle%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getTitle()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `commitDetails` to the URL query string
if (getCommitDetails() != null) {
joiner.add(getCommitDetails().toUrlQueryString(prefix + "commitDetails" + suffix));
}
// add `dominoStats` to the URL query string
if (getDominoStats() != null) {
for (int i = 0; i < getDominoStats().size(); i++) {
if (getDominoStats().get(i) != null) {
joiner.add(getDominoStats().get(i).toUrlQueryString(String.format("%sdominoStats%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `statuses` to the URL query string
if (getStatuses() != null) {
joiner.add(getStatuses().toUrlQueryString(prefix + "statuses" + suffix));
}
// add `mainRepoGitRef` to the URL query string
if (getMainRepoGitRef() != null) {
joiner.add(getMainRepoGitRef().toUrlQueryString(prefix + "mainRepoGitRef" + suffix));
}
// add `dependentRepositories` to the URL query string
if (getDependentRepositories() != null) {
for (int i = 0; i < getDependentRepositories().size(); i++) {
if (getDependentRepositories().get(i) != null) {
joiner.add(getDependentRepositories().get(i).toUrlQueryString(String.format("%sdependentRepositories%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `dependentDatasetMounts` to the URL query string
if (getDependentDatasetMounts() != null) {
for (int i = 0; i < getDependentDatasetMounts().size(); i++) {
if (getDependentDatasetMounts().get(i) != null) {
joiner.add(getDependentDatasetMounts().get(i).toUrlQueryString(String.format("%sdependentDatasetMounts%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `dependentProjects` to the URL query string
if (getDependentProjects() != null) {
for (int i = 0; i < getDependentProjects().size(); i++) {
if (getDependentProjects().get(i) != null) {
joiner.add(getDependentProjects().get(i).toUrlQueryString(String.format("%sdependentProjects%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `suggestDatasets` to the URL query string
if (getSuggestDatasets() != null) {
joiner.add(String.format("%ssuggestDatasets%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getSuggestDatasets()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `goalIds` to the URL query string
if (getGoalIds() != null) {
for (int i = 0; i < getGoalIds().size(); i++) {
joiner.add(String.format("%sgoalIds%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getGoalIds().get(i)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
// add `runLauncherId` to the URL query string
if (getRunLauncherId() != null) {
joiner.add(String.format("%srunLauncherId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getRunLauncherId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `dependentExternalVolumeMounts` to the URL query string
if (getDependentExternalVolumeMounts() != null) {
for (int i = 0; i < getDependentExternalVolumeMounts().size(); i++) {
if (getDependentExternalVolumeMounts().get(i) != null) {
joiner.add(getDependentExternalVolumeMounts().get(i).toUrlQueryString(String.format("%sdependentExternalVolumeMounts%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `computeCluster` to the URL query string
if (getComputeCluster() != null) {
joiner.add(getComputeCluster().toUrlQueryString(prefix + "computeCluster" + suffix));
}
// add `dataPlane` to the URL query string
if (getDataPlane() != null) {
joiner.add(getDataPlane().toUrlQueryString(prefix + "dataPlane" + suffix));
}
// add `snapshotDatasetsOnCompletion` to the URL query string
if (getSnapshotDatasetsOnCompletion() != null) {
joiner.add(String.format("%ssnapshotDatasetsOnCompletion%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getSnapshotDatasetsOnCompletion()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy