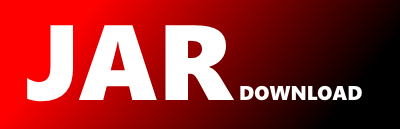
com.dominodatalab.api.model.DominoProjectManagementApiPmComment Maven / Gradle / Ivy
/*
* Domino Data Lab API v4
* This API is going to provide access to all the Domino functions available in the user interface. To authenticate your requests, include your API Key (which you can find on your account page) with the header X-Domino-Api-Key.
*
* The version of the OpenAPI document: 4.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.api.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.dominodatalab.api.model.DominoProjectManagementApiDominoEntity;
import com.dominodatalab.api.model.DominoProjectManagementApiPmId;
import com.dominodatalab.api.model.DominoProjectManagementApiPmUser;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* DominoProjectManagementApiPmComment
*/
@JsonPropertyOrder({
DominoProjectManagementApiPmComment.JSON_PROPERTY_ID,
DominoProjectManagementApiPmComment.JSON_PROPERTY_COMMENT_BODY,
DominoProjectManagementApiPmComment.JSON_PROPERTY_CREATED,
DominoProjectManagementApiPmComment.JSON_PROPERTY_COMMENTER,
DominoProjectManagementApiPmComment.JSON_PROPERTY_UPDATED_AT,
DominoProjectManagementApiPmComment.JSON_PROPERTY_DOMINO_ENTITY
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2023-10-17T15:20:46.682098100-04:00[America/New_York]")
public class DominoProjectManagementApiPmComment {
public static final String JSON_PROPERTY_ID = "id";
private DominoProjectManagementApiPmId id;
public static final String JSON_PROPERTY_COMMENT_BODY = "commentBody";
private String commentBody;
public static final String JSON_PROPERTY_CREATED = "created";
private Long created;
public static final String JSON_PROPERTY_COMMENTER = "commenter";
private DominoProjectManagementApiPmUser commenter;
public static final String JSON_PROPERTY_UPDATED_AT = "updatedAt";
private Long updatedAt;
public static final String JSON_PROPERTY_DOMINO_ENTITY = "dominoEntity";
private DominoProjectManagementApiDominoEntity dominoEntity;
public DominoProjectManagementApiPmComment() {
}
public DominoProjectManagementApiPmComment id(DominoProjectManagementApiPmId id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public DominoProjectManagementApiPmId getId() {
return id;
}
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setId(DominoProjectManagementApiPmId id) {
this.id = id;
}
public DominoProjectManagementApiPmComment commentBody(String commentBody) {
this.commentBody = commentBody;
return this;
}
/**
* Get commentBody
* @return commentBody
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_COMMENT_BODY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getCommentBody() {
return commentBody;
}
@JsonProperty(JSON_PROPERTY_COMMENT_BODY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setCommentBody(String commentBody) {
this.commentBody = commentBody;
}
public DominoProjectManagementApiPmComment created(Long created) {
this.created = created;
return this;
}
/**
* Get created
* @return created
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_CREATED)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Long getCreated() {
return created;
}
@JsonProperty(JSON_PROPERTY_CREATED)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setCreated(Long created) {
this.created = created;
}
public DominoProjectManagementApiPmComment commenter(DominoProjectManagementApiPmUser commenter) {
this.commenter = commenter;
return this;
}
/**
* Get commenter
* @return commenter
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_COMMENTER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DominoProjectManagementApiPmUser getCommenter() {
return commenter;
}
@JsonProperty(JSON_PROPERTY_COMMENTER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCommenter(DominoProjectManagementApiPmUser commenter) {
this.commenter = commenter;
}
public DominoProjectManagementApiPmComment updatedAt(Long updatedAt) {
this.updatedAt = updatedAt;
return this;
}
/**
* Get updatedAt
* @return updatedAt
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_UPDATED_AT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Long getUpdatedAt() {
return updatedAt;
}
@JsonProperty(JSON_PROPERTY_UPDATED_AT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setUpdatedAt(Long updatedAt) {
this.updatedAt = updatedAt;
}
public DominoProjectManagementApiPmComment dominoEntity(DominoProjectManagementApiDominoEntity dominoEntity) {
this.dominoEntity = dominoEntity;
return this;
}
/**
* Get dominoEntity
* @return dominoEntity
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DOMINO_ENTITY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DominoProjectManagementApiDominoEntity getDominoEntity() {
return dominoEntity;
}
@JsonProperty(JSON_PROPERTY_DOMINO_ENTITY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDominoEntity(DominoProjectManagementApiDominoEntity dominoEntity) {
this.dominoEntity = dominoEntity;
}
/**
* Return true if this domino.projectManagement.api.PmComment object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DominoProjectManagementApiPmComment dominoProjectManagementApiPmComment = (DominoProjectManagementApiPmComment) o;
return Objects.equals(this.id, dominoProjectManagementApiPmComment.id) &&
Objects.equals(this.commentBody, dominoProjectManagementApiPmComment.commentBody) &&
Objects.equals(this.created, dominoProjectManagementApiPmComment.created) &&
Objects.equals(this.commenter, dominoProjectManagementApiPmComment.commenter) &&
Objects.equals(this.updatedAt, dominoProjectManagementApiPmComment.updatedAt) &&
Objects.equals(this.dominoEntity, dominoProjectManagementApiPmComment.dominoEntity);
}
@Override
public int hashCode() {
return Objects.hash(id, commentBody, created, commenter, updatedAt, dominoEntity);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DominoProjectManagementApiPmComment {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" commentBody: ").append(toIndentedString(commentBody)).append("\n");
sb.append(" created: ").append(toIndentedString(created)).append("\n");
sb.append(" commenter: ").append(toIndentedString(commenter)).append("\n");
sb.append(" updatedAt: ").append(toIndentedString(updatedAt)).append("\n");
sb.append(" dominoEntity: ").append(toIndentedString(dominoEntity)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `id` to the URL query string
if (getId() != null) {
joiner.add(getId().toUrlQueryString(prefix + "id" + suffix));
}
// add `commentBody` to the URL query string
if (getCommentBody() != null) {
joiner.add(String.format("%scommentBody%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getCommentBody()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `created` to the URL query string
if (getCreated() != null) {
joiner.add(String.format("%screated%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getCreated()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `commenter` to the URL query string
if (getCommenter() != null) {
joiner.add(getCommenter().toUrlQueryString(prefix + "commenter" + suffix));
}
// add `updatedAt` to the URL query string
if (getUpdatedAt() != null) {
joiner.add(String.format("%supdatedAt%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getUpdatedAt()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `dominoEntity` to the URL query string
if (getDominoEntity() != null) {
joiner.add(getDominoEntity().toUrlQueryString(prefix + "dominoEntity" + suffix));
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy