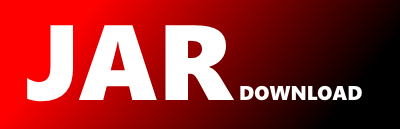
com.dominodatalab.api.model.DominoScheduledjobApiComputeClusterConfigSpecDto Maven / Gradle / Ivy
/*
* Domino Data Lab API v4
* This API is going to provide access to all the Domino functions available in the user interface. To authenticate your requests, include your API Key (which you can find on your account page) with the header X-Domino-Api-Key.
*
* The version of the OpenAPI document: 4.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.api.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.dominodatalab.api.model.ComputeClusterType;
import com.dominodatalab.api.model.DominoHardwaretierApiHardwareTierIdentifier;
import com.dominodatalab.api.model.DominoScheduledjobApiComputeClusterConfigSpecDtoComputeEnvironmentRevisionSpec;
import com.dominodatalab.api.model.Information;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.Arrays;
import java.util.HashMap;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* DominoScheduledjobApiComputeClusterConfigSpecDto
*/
@JsonPropertyOrder({
DominoScheduledjobApiComputeClusterConfigSpecDto.JSON_PROPERTY_CLUSTER_TYPE,
DominoScheduledjobApiComputeClusterConfigSpecDto.JSON_PROPERTY_COMPUTE_ENVIRONMENT_ID,
DominoScheduledjobApiComputeClusterConfigSpecDto.JSON_PROPERTY_COMPUTE_ENVIRONMENT_REVISION_SPEC,
DominoScheduledjobApiComputeClusterConfigSpecDto.JSON_PROPERTY_MASTER_HARDWARE_TIER_ID,
DominoScheduledjobApiComputeClusterConfigSpecDto.JSON_PROPERTY_WORKER_COUNT,
DominoScheduledjobApiComputeClusterConfigSpecDto.JSON_PROPERTY_MAX_WORKER_COUNT,
DominoScheduledjobApiComputeClusterConfigSpecDto.JSON_PROPERTY_WORKER_HARDWARE_TIER_ID,
DominoScheduledjobApiComputeClusterConfigSpecDto.JSON_PROPERTY_WORKER_STORAGE,
DominoScheduledjobApiComputeClusterConfigSpecDto.JSON_PROPERTY_EXTRA_CONFIGS
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2023-10-17T15:20:46.682098100-04:00[America/New_York]")
public class DominoScheduledjobApiComputeClusterConfigSpecDto {
public static final String JSON_PROPERTY_CLUSTER_TYPE = "clusterType";
private ComputeClusterType clusterType;
public static final String JSON_PROPERTY_COMPUTE_ENVIRONMENT_ID = "computeEnvironmentId";
private String computeEnvironmentId;
public static final String JSON_PROPERTY_COMPUTE_ENVIRONMENT_REVISION_SPEC = "computeEnvironmentRevisionSpec";
private DominoScheduledjobApiComputeClusterConfigSpecDtoComputeEnvironmentRevisionSpec computeEnvironmentRevisionSpec;
public static final String JSON_PROPERTY_MASTER_HARDWARE_TIER_ID = "masterHardwareTierId";
private DominoHardwaretierApiHardwareTierIdentifier masterHardwareTierId;
public static final String JSON_PROPERTY_WORKER_COUNT = "workerCount";
private Integer workerCount;
public static final String JSON_PROPERTY_MAX_WORKER_COUNT = "maxWorkerCount";
private Integer maxWorkerCount;
public static final String JSON_PROPERTY_WORKER_HARDWARE_TIER_ID = "workerHardwareTierId";
private DominoHardwaretierApiHardwareTierIdentifier workerHardwareTierId;
public static final String JSON_PROPERTY_WORKER_STORAGE = "workerStorage";
private Information workerStorage;
public static final String JSON_PROPERTY_EXTRA_CONFIGS = "extraConfigs";
private Map extraConfigs;
public DominoScheduledjobApiComputeClusterConfigSpecDto() {
}
public DominoScheduledjobApiComputeClusterConfigSpecDto clusterType(ComputeClusterType clusterType) {
this.clusterType = clusterType;
return this;
}
/**
* Get clusterType
* @return clusterType
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_CLUSTER_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public ComputeClusterType getClusterType() {
return clusterType;
}
@JsonProperty(JSON_PROPERTY_CLUSTER_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setClusterType(ComputeClusterType clusterType) {
this.clusterType = clusterType;
}
public DominoScheduledjobApiComputeClusterConfigSpecDto computeEnvironmentId(String computeEnvironmentId) {
this.computeEnvironmentId = computeEnvironmentId;
return this;
}
/**
* Get computeEnvironmentId
* @return computeEnvironmentId
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_COMPUTE_ENVIRONMENT_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getComputeEnvironmentId() {
return computeEnvironmentId;
}
@JsonProperty(JSON_PROPERTY_COMPUTE_ENVIRONMENT_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setComputeEnvironmentId(String computeEnvironmentId) {
this.computeEnvironmentId = computeEnvironmentId;
}
public DominoScheduledjobApiComputeClusterConfigSpecDto computeEnvironmentRevisionSpec(DominoScheduledjobApiComputeClusterConfigSpecDtoComputeEnvironmentRevisionSpec computeEnvironmentRevisionSpec) {
this.computeEnvironmentRevisionSpec = computeEnvironmentRevisionSpec;
return this;
}
/**
* Get computeEnvironmentRevisionSpec
* @return computeEnvironmentRevisionSpec
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_COMPUTE_ENVIRONMENT_REVISION_SPEC)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DominoScheduledjobApiComputeClusterConfigSpecDtoComputeEnvironmentRevisionSpec getComputeEnvironmentRevisionSpec() {
return computeEnvironmentRevisionSpec;
}
@JsonProperty(JSON_PROPERTY_COMPUTE_ENVIRONMENT_REVISION_SPEC)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setComputeEnvironmentRevisionSpec(DominoScheduledjobApiComputeClusterConfigSpecDtoComputeEnvironmentRevisionSpec computeEnvironmentRevisionSpec) {
this.computeEnvironmentRevisionSpec = computeEnvironmentRevisionSpec;
}
public DominoScheduledjobApiComputeClusterConfigSpecDto masterHardwareTierId(DominoHardwaretierApiHardwareTierIdentifier masterHardwareTierId) {
this.masterHardwareTierId = masterHardwareTierId;
return this;
}
/**
* Get masterHardwareTierId
* @return masterHardwareTierId
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_MASTER_HARDWARE_TIER_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DominoHardwaretierApiHardwareTierIdentifier getMasterHardwareTierId() {
return masterHardwareTierId;
}
@JsonProperty(JSON_PROPERTY_MASTER_HARDWARE_TIER_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMasterHardwareTierId(DominoHardwaretierApiHardwareTierIdentifier masterHardwareTierId) {
this.masterHardwareTierId = masterHardwareTierId;
}
public DominoScheduledjobApiComputeClusterConfigSpecDto workerCount(Integer workerCount) {
this.workerCount = workerCount;
return this;
}
/**
* Get workerCount
* @return workerCount
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_WORKER_COUNT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Integer getWorkerCount() {
return workerCount;
}
@JsonProperty(JSON_PROPERTY_WORKER_COUNT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setWorkerCount(Integer workerCount) {
this.workerCount = workerCount;
}
public DominoScheduledjobApiComputeClusterConfigSpecDto maxWorkerCount(Integer maxWorkerCount) {
this.maxWorkerCount = maxWorkerCount;
return this;
}
/**
* Get maxWorkerCount
* @return maxWorkerCount
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_MAX_WORKER_COUNT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getMaxWorkerCount() {
return maxWorkerCount;
}
@JsonProperty(JSON_PROPERTY_MAX_WORKER_COUNT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMaxWorkerCount(Integer maxWorkerCount) {
this.maxWorkerCount = maxWorkerCount;
}
public DominoScheduledjobApiComputeClusterConfigSpecDto workerHardwareTierId(DominoHardwaretierApiHardwareTierIdentifier workerHardwareTierId) {
this.workerHardwareTierId = workerHardwareTierId;
return this;
}
/**
* Get workerHardwareTierId
* @return workerHardwareTierId
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_WORKER_HARDWARE_TIER_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public DominoHardwaretierApiHardwareTierIdentifier getWorkerHardwareTierId() {
return workerHardwareTierId;
}
@JsonProperty(JSON_PROPERTY_WORKER_HARDWARE_TIER_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setWorkerHardwareTierId(DominoHardwaretierApiHardwareTierIdentifier workerHardwareTierId) {
this.workerHardwareTierId = workerHardwareTierId;
}
public DominoScheduledjobApiComputeClusterConfigSpecDto workerStorage(Information workerStorage) {
this.workerStorage = workerStorage;
return this;
}
/**
* Get workerStorage
* @return workerStorage
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_WORKER_STORAGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Information getWorkerStorage() {
return workerStorage;
}
@JsonProperty(JSON_PROPERTY_WORKER_STORAGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setWorkerStorage(Information workerStorage) {
this.workerStorage = workerStorage;
}
public DominoScheduledjobApiComputeClusterConfigSpecDto extraConfigs(Map extraConfigs) {
this.extraConfigs = extraConfigs;
return this;
}
public DominoScheduledjobApiComputeClusterConfigSpecDto putExtraConfigsItem(String key, String extraConfigsItem) {
if (this.extraConfigs == null) {
this.extraConfigs = new HashMap<>();
}
this.extraConfigs.put(key, extraConfigsItem);
return this;
}
/**
* Get extraConfigs
* @return extraConfigs
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_EXTRA_CONFIGS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Map getExtraConfigs() {
return extraConfigs;
}
@JsonProperty(JSON_PROPERTY_EXTRA_CONFIGS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setExtraConfigs(Map extraConfigs) {
this.extraConfigs = extraConfigs;
}
/**
* Return true if this domino.scheduledjob.api.ComputeClusterConfigSpecDto object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DominoScheduledjobApiComputeClusterConfigSpecDto dominoScheduledjobApiComputeClusterConfigSpecDto = (DominoScheduledjobApiComputeClusterConfigSpecDto) o;
return Objects.equals(this.clusterType, dominoScheduledjobApiComputeClusterConfigSpecDto.clusterType) &&
Objects.equals(this.computeEnvironmentId, dominoScheduledjobApiComputeClusterConfigSpecDto.computeEnvironmentId) &&
Objects.equals(this.computeEnvironmentRevisionSpec, dominoScheduledjobApiComputeClusterConfigSpecDto.computeEnvironmentRevisionSpec) &&
Objects.equals(this.masterHardwareTierId, dominoScheduledjobApiComputeClusterConfigSpecDto.masterHardwareTierId) &&
Objects.equals(this.workerCount, dominoScheduledjobApiComputeClusterConfigSpecDto.workerCount) &&
Objects.equals(this.maxWorkerCount, dominoScheduledjobApiComputeClusterConfigSpecDto.maxWorkerCount) &&
Objects.equals(this.workerHardwareTierId, dominoScheduledjobApiComputeClusterConfigSpecDto.workerHardwareTierId) &&
Objects.equals(this.workerStorage, dominoScheduledjobApiComputeClusterConfigSpecDto.workerStorage) &&
Objects.equals(this.extraConfigs, dominoScheduledjobApiComputeClusterConfigSpecDto.extraConfigs);
}
@Override
public int hashCode() {
return Objects.hash(clusterType, computeEnvironmentId, computeEnvironmentRevisionSpec, masterHardwareTierId, workerCount, maxWorkerCount, workerHardwareTierId, workerStorage, extraConfigs);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DominoScheduledjobApiComputeClusterConfigSpecDto {\n");
sb.append(" clusterType: ").append(toIndentedString(clusterType)).append("\n");
sb.append(" computeEnvironmentId: ").append(toIndentedString(computeEnvironmentId)).append("\n");
sb.append(" computeEnvironmentRevisionSpec: ").append(toIndentedString(computeEnvironmentRevisionSpec)).append("\n");
sb.append(" masterHardwareTierId: ").append(toIndentedString(masterHardwareTierId)).append("\n");
sb.append(" workerCount: ").append(toIndentedString(workerCount)).append("\n");
sb.append(" maxWorkerCount: ").append(toIndentedString(maxWorkerCount)).append("\n");
sb.append(" workerHardwareTierId: ").append(toIndentedString(workerHardwareTierId)).append("\n");
sb.append(" workerStorage: ").append(toIndentedString(workerStorage)).append("\n");
sb.append(" extraConfigs: ").append(toIndentedString(extraConfigs)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `clusterType` to the URL query string
if (getClusterType() != null) {
joiner.add(String.format("%sclusterType%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getClusterType()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `computeEnvironmentId` to the URL query string
if (getComputeEnvironmentId() != null) {
joiner.add(String.format("%scomputeEnvironmentId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getComputeEnvironmentId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `computeEnvironmentRevisionSpec` to the URL query string
if (getComputeEnvironmentRevisionSpec() != null) {
joiner.add(getComputeEnvironmentRevisionSpec().toUrlQueryString(prefix + "computeEnvironmentRevisionSpec" + suffix));
}
// add `masterHardwareTierId` to the URL query string
if (getMasterHardwareTierId() != null) {
joiner.add(getMasterHardwareTierId().toUrlQueryString(prefix + "masterHardwareTierId" + suffix));
}
// add `workerCount` to the URL query string
if (getWorkerCount() != null) {
joiner.add(String.format("%sworkerCount%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getWorkerCount()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `maxWorkerCount` to the URL query string
if (getMaxWorkerCount() != null) {
joiner.add(String.format("%smaxWorkerCount%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getMaxWorkerCount()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `workerHardwareTierId` to the URL query string
if (getWorkerHardwareTierId() != null) {
joiner.add(getWorkerHardwareTierId().toUrlQueryString(prefix + "workerHardwareTierId" + suffix));
}
// add `workerStorage` to the URL query string
if (getWorkerStorage() != null) {
joiner.add(getWorkerStorage().toUrlQueryString(prefix + "workerStorage" + suffix));
}
// add `extraConfigs` to the URL query string
if (getExtraConfigs() != null) {
for (String _key : getExtraConfigs().keySet()) {
joiner.add(String.format("%sextraConfigs%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, _key, containerSuffix),
getExtraConfigs().get(_key), URLEncoder.encode(String.valueOf(getExtraConfigs().get(_key)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy