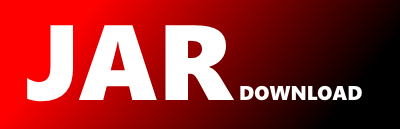
com.dominodatalab.api.model.DominoScheduledjobApiUpdatedScheduledJobDTO Maven / Gradle / Ivy
/*
* Domino Data Lab API v4
* This API is going to provide access to all the Domino functions available in the user interface. To authenticate your requests, include your API Key (which you can find on your account page) with the header X-Domino-Api-Key.
*
* The version of the OpenAPI document: 4.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.api.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.dominodatalab.api.model.DominoProjectsApiRepositoriesReferenceDTO;
import com.dominodatalab.api.model.DominoScheduledjobApiComputeClusterConfigSpecDto;
import com.dominodatalab.api.model.DominoScheduledjobApiEditCronScheduleDTO;
import com.dominodatalab.api.model.DominoScheduledjobApiScheduledJobDtoEnvironmentRevisionSpec;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* DominoScheduledjobApiUpdatedScheduledJobDTO
*/
@JsonPropertyOrder({
DominoScheduledjobApiUpdatedScheduledJobDTO.JSON_PROPERTY_TITLE,
DominoScheduledjobApiUpdatedScheduledJobDTO.JSON_PROPERTY_COMMAND,
DominoScheduledjobApiUpdatedScheduledJobDTO.JSON_PROPERTY_SCHEDULE,
DominoScheduledjobApiUpdatedScheduledJobDTO.JSON_PROPERTY_TIMEZONE_ID,
DominoScheduledjobApiUpdatedScheduledJobDTO.JSON_PROPERTY_IS_PAUSED,
DominoScheduledjobApiUpdatedScheduledJobDTO.JSON_PROPERTY_PUBLISH_MODEL_ID,
DominoScheduledjobApiUpdatedScheduledJobDTO.JSON_PROPERTY_ALLOW_CONCURRENT_EXECUTION,
DominoScheduledjobApiUpdatedScheduledJobDTO.JSON_PROPERTY_HARDWARE_TIER_IDENTIFIER,
DominoScheduledjobApiUpdatedScheduledJobDTO.JSON_PROPERTY_OVERRIDE_ENVIRONMENT_ID,
DominoScheduledjobApiUpdatedScheduledJobDTO.JSON_PROPERTY_ENVIRONMENT_REVISION_SPEC,
DominoScheduledjobApiUpdatedScheduledJobDTO.JSON_PROPERTY_SCHEDULED_BY_USER_ID,
DominoScheduledjobApiUpdatedScheduledJobDTO.JSON_PROPERTY_NOTIFY_ON_COMPLETE_EMAIL_ADDRESSES,
DominoScheduledjobApiUpdatedScheduledJobDTO.JSON_PROPERTY_DATASET_CONFIG,
DominoScheduledjobApiUpdatedScheduledJobDTO.JSON_PROPERTY_COMPUTE_CLUSTER_PROPERTIES,
DominoScheduledjobApiUpdatedScheduledJobDTO.JSON_PROPERTY_VOLUME_SPECIFICATION_OVERRIDE,
DominoScheduledjobApiUpdatedScheduledJobDTO.JSON_PROPERTY_MAIN_REPO_GIT_REF,
DominoScheduledjobApiUpdatedScheduledJobDTO.JSON_PROPERTY_SNAPSHOT_DATASETS_ON_COMPLETION
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2023-10-17T15:20:46.682098100-04:00[America/New_York]")
public class DominoScheduledjobApiUpdatedScheduledJobDTO {
public static final String JSON_PROPERTY_TITLE = "title";
private String title;
public static final String JSON_PROPERTY_COMMAND = "command";
private String command;
public static final String JSON_PROPERTY_SCHEDULE = "schedule";
private DominoScheduledjobApiEditCronScheduleDTO schedule;
public static final String JSON_PROPERTY_TIMEZONE_ID = "timezoneId";
private String timezoneId;
public static final String JSON_PROPERTY_IS_PAUSED = "isPaused";
private Boolean isPaused;
public static final String JSON_PROPERTY_PUBLISH_MODEL_ID = "publishModelId";
private String publishModelId;
public static final String JSON_PROPERTY_ALLOW_CONCURRENT_EXECUTION = "allowConcurrentExecution";
private Boolean allowConcurrentExecution;
public static final String JSON_PROPERTY_HARDWARE_TIER_IDENTIFIER = "hardwareTierIdentifier";
private String hardwareTierIdentifier;
public static final String JSON_PROPERTY_OVERRIDE_ENVIRONMENT_ID = "overrideEnvironmentId";
private String overrideEnvironmentId;
public static final String JSON_PROPERTY_ENVIRONMENT_REVISION_SPEC = "environmentRevisionSpec";
private DominoScheduledjobApiScheduledJobDtoEnvironmentRevisionSpec environmentRevisionSpec;
public static final String JSON_PROPERTY_SCHEDULED_BY_USER_ID = "scheduledByUserId";
private String scheduledByUserId;
public static final String JSON_PROPERTY_NOTIFY_ON_COMPLETE_EMAIL_ADDRESSES = "notifyOnCompleteEmailAddresses";
private List notifyOnCompleteEmailAddresses = new ArrayList<>();
public static final String JSON_PROPERTY_DATASET_CONFIG = "datasetConfig";
private String datasetConfig;
public static final String JSON_PROPERTY_COMPUTE_CLUSTER_PROPERTIES = "computeClusterProperties";
private DominoScheduledjobApiComputeClusterConfigSpecDto computeClusterProperties;
public static final String JSON_PROPERTY_VOLUME_SPECIFICATION_OVERRIDE = "volumeSpecificationOverride";
private Map volumeSpecificationOverride;
public static final String JSON_PROPERTY_MAIN_REPO_GIT_REF = "mainRepoGitRef";
private DominoProjectsApiRepositoriesReferenceDTO mainRepoGitRef;
public static final String JSON_PROPERTY_SNAPSHOT_DATASETS_ON_COMPLETION = "snapshotDatasetsOnCompletion";
private Boolean snapshotDatasetsOnCompletion;
public DominoScheduledjobApiUpdatedScheduledJobDTO() {
}
public DominoScheduledjobApiUpdatedScheduledJobDTO title(String title) {
this.title = title;
return this;
}
/**
* Get title
* @return title
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_TITLE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getTitle() {
return title;
}
@JsonProperty(JSON_PROPERTY_TITLE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setTitle(String title) {
this.title = title;
}
public DominoScheduledjobApiUpdatedScheduledJobDTO command(String command) {
this.command = command;
return this;
}
/**
* Get command
* @return command
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_COMMAND)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getCommand() {
return command;
}
@JsonProperty(JSON_PROPERTY_COMMAND)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setCommand(String command) {
this.command = command;
}
public DominoScheduledjobApiUpdatedScheduledJobDTO schedule(DominoScheduledjobApiEditCronScheduleDTO schedule) {
this.schedule = schedule;
return this;
}
/**
* Get schedule
* @return schedule
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_SCHEDULE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public DominoScheduledjobApiEditCronScheduleDTO getSchedule() {
return schedule;
}
@JsonProperty(JSON_PROPERTY_SCHEDULE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setSchedule(DominoScheduledjobApiEditCronScheduleDTO schedule) {
this.schedule = schedule;
}
public DominoScheduledjobApiUpdatedScheduledJobDTO timezoneId(String timezoneId) {
this.timezoneId = timezoneId;
return this;
}
/**
* Get timezoneId
* @return timezoneId
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_TIMEZONE_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getTimezoneId() {
return timezoneId;
}
@JsonProperty(JSON_PROPERTY_TIMEZONE_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setTimezoneId(String timezoneId) {
this.timezoneId = timezoneId;
}
public DominoScheduledjobApiUpdatedScheduledJobDTO isPaused(Boolean isPaused) {
this.isPaused = isPaused;
return this;
}
/**
* Get isPaused
* @return isPaused
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_IS_PAUSED)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getIsPaused() {
return isPaused;
}
@JsonProperty(JSON_PROPERTY_IS_PAUSED)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setIsPaused(Boolean isPaused) {
this.isPaused = isPaused;
}
public DominoScheduledjobApiUpdatedScheduledJobDTO publishModelId(String publishModelId) {
this.publishModelId = publishModelId;
return this;
}
/**
* Get publishModelId
* @return publishModelId
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_PUBLISH_MODEL_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getPublishModelId() {
return publishModelId;
}
@JsonProperty(JSON_PROPERTY_PUBLISH_MODEL_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPublishModelId(String publishModelId) {
this.publishModelId = publishModelId;
}
public DominoScheduledjobApiUpdatedScheduledJobDTO allowConcurrentExecution(Boolean allowConcurrentExecution) {
this.allowConcurrentExecution = allowConcurrentExecution;
return this;
}
/**
* Get allowConcurrentExecution
* @return allowConcurrentExecution
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ALLOW_CONCURRENT_EXECUTION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getAllowConcurrentExecution() {
return allowConcurrentExecution;
}
@JsonProperty(JSON_PROPERTY_ALLOW_CONCURRENT_EXECUTION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setAllowConcurrentExecution(Boolean allowConcurrentExecution) {
this.allowConcurrentExecution = allowConcurrentExecution;
}
public DominoScheduledjobApiUpdatedScheduledJobDTO hardwareTierIdentifier(String hardwareTierIdentifier) {
this.hardwareTierIdentifier = hardwareTierIdentifier;
return this;
}
/**
* Get hardwareTierIdentifier
* @return hardwareTierIdentifier
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_HARDWARE_TIER_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getHardwareTierIdentifier() {
return hardwareTierIdentifier;
}
@JsonProperty(JSON_PROPERTY_HARDWARE_TIER_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setHardwareTierIdentifier(String hardwareTierIdentifier) {
this.hardwareTierIdentifier = hardwareTierIdentifier;
}
public DominoScheduledjobApiUpdatedScheduledJobDTO overrideEnvironmentId(String overrideEnvironmentId) {
this.overrideEnvironmentId = overrideEnvironmentId;
return this;
}
/**
* Get overrideEnvironmentId
* @return overrideEnvironmentId
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_OVERRIDE_ENVIRONMENT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getOverrideEnvironmentId() {
return overrideEnvironmentId;
}
@JsonProperty(JSON_PROPERTY_OVERRIDE_ENVIRONMENT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setOverrideEnvironmentId(String overrideEnvironmentId) {
this.overrideEnvironmentId = overrideEnvironmentId;
}
public DominoScheduledjobApiUpdatedScheduledJobDTO environmentRevisionSpec(DominoScheduledjobApiScheduledJobDtoEnvironmentRevisionSpec environmentRevisionSpec) {
this.environmentRevisionSpec = environmentRevisionSpec;
return this;
}
/**
* Get environmentRevisionSpec
* @return environmentRevisionSpec
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ENVIRONMENT_REVISION_SPEC)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public DominoScheduledjobApiScheduledJobDtoEnvironmentRevisionSpec getEnvironmentRevisionSpec() {
return environmentRevisionSpec;
}
@JsonProperty(JSON_PROPERTY_ENVIRONMENT_REVISION_SPEC)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setEnvironmentRevisionSpec(DominoScheduledjobApiScheduledJobDtoEnvironmentRevisionSpec environmentRevisionSpec) {
this.environmentRevisionSpec = environmentRevisionSpec;
}
public DominoScheduledjobApiUpdatedScheduledJobDTO scheduledByUserId(String scheduledByUserId) {
this.scheduledByUserId = scheduledByUserId;
return this;
}
/**
* Get scheduledByUserId
* @return scheduledByUserId
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_SCHEDULED_BY_USER_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getScheduledByUserId() {
return scheduledByUserId;
}
@JsonProperty(JSON_PROPERTY_SCHEDULED_BY_USER_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setScheduledByUserId(String scheduledByUserId) {
this.scheduledByUserId = scheduledByUserId;
}
public DominoScheduledjobApiUpdatedScheduledJobDTO notifyOnCompleteEmailAddresses(List notifyOnCompleteEmailAddresses) {
this.notifyOnCompleteEmailAddresses = notifyOnCompleteEmailAddresses;
return this;
}
public DominoScheduledjobApiUpdatedScheduledJobDTO addNotifyOnCompleteEmailAddressesItem(String notifyOnCompleteEmailAddressesItem) {
if (this.notifyOnCompleteEmailAddresses == null) {
this.notifyOnCompleteEmailAddresses = new ArrayList<>();
}
this.notifyOnCompleteEmailAddresses.add(notifyOnCompleteEmailAddressesItem);
return this;
}
/**
* Get notifyOnCompleteEmailAddresses
* @return notifyOnCompleteEmailAddresses
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_NOTIFY_ON_COMPLETE_EMAIL_ADDRESSES)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getNotifyOnCompleteEmailAddresses() {
return notifyOnCompleteEmailAddresses;
}
@JsonProperty(JSON_PROPERTY_NOTIFY_ON_COMPLETE_EMAIL_ADDRESSES)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setNotifyOnCompleteEmailAddresses(List notifyOnCompleteEmailAddresses) {
this.notifyOnCompleteEmailAddresses = notifyOnCompleteEmailAddresses;
}
public DominoScheduledjobApiUpdatedScheduledJobDTO datasetConfig(String datasetConfig) {
this.datasetConfig = datasetConfig;
return this;
}
/**
* Get datasetConfig
* @return datasetConfig
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DATASET_CONFIG)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDatasetConfig() {
return datasetConfig;
}
@JsonProperty(JSON_PROPERTY_DATASET_CONFIG)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDatasetConfig(String datasetConfig) {
this.datasetConfig = datasetConfig;
}
public DominoScheduledjobApiUpdatedScheduledJobDTO computeClusterProperties(DominoScheduledjobApiComputeClusterConfigSpecDto computeClusterProperties) {
this.computeClusterProperties = computeClusterProperties;
return this;
}
/**
* Get computeClusterProperties
* @return computeClusterProperties
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_COMPUTE_CLUSTER_PROPERTIES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DominoScheduledjobApiComputeClusterConfigSpecDto getComputeClusterProperties() {
return computeClusterProperties;
}
@JsonProperty(JSON_PROPERTY_COMPUTE_CLUSTER_PROPERTIES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setComputeClusterProperties(DominoScheduledjobApiComputeClusterConfigSpecDto computeClusterProperties) {
this.computeClusterProperties = computeClusterProperties;
}
public DominoScheduledjobApiUpdatedScheduledJobDTO volumeSpecificationOverride(Map volumeSpecificationOverride) {
this.volumeSpecificationOverride = volumeSpecificationOverride;
return this;
}
public DominoScheduledjobApiUpdatedScheduledJobDTO putVolumeSpecificationOverrideItem(String key, Object volumeSpecificationOverrideItem) {
if (this.volumeSpecificationOverride == null) {
this.volumeSpecificationOverride = new HashMap<>();
}
this.volumeSpecificationOverride.put(key, volumeSpecificationOverrideItem);
return this;
}
/**
* Get volumeSpecificationOverride
* @return volumeSpecificationOverride
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_VOLUME_SPECIFICATION_OVERRIDE)
@JsonInclude(content = JsonInclude.Include.ALWAYS, value = JsonInclude.Include.USE_DEFAULTS)
public Map getVolumeSpecificationOverride() {
return volumeSpecificationOverride;
}
@JsonProperty(JSON_PROPERTY_VOLUME_SPECIFICATION_OVERRIDE)
@JsonInclude(content = JsonInclude.Include.ALWAYS, value = JsonInclude.Include.USE_DEFAULTS)
public void setVolumeSpecificationOverride(Map volumeSpecificationOverride) {
this.volumeSpecificationOverride = volumeSpecificationOverride;
}
public DominoScheduledjobApiUpdatedScheduledJobDTO mainRepoGitRef(DominoProjectsApiRepositoriesReferenceDTO mainRepoGitRef) {
this.mainRepoGitRef = mainRepoGitRef;
return this;
}
/**
* Get mainRepoGitRef
* @return mainRepoGitRef
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_MAIN_REPO_GIT_REF)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DominoProjectsApiRepositoriesReferenceDTO getMainRepoGitRef() {
return mainRepoGitRef;
}
@JsonProperty(JSON_PROPERTY_MAIN_REPO_GIT_REF)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMainRepoGitRef(DominoProjectsApiRepositoriesReferenceDTO mainRepoGitRef) {
this.mainRepoGitRef = mainRepoGitRef;
}
public DominoScheduledjobApiUpdatedScheduledJobDTO snapshotDatasetsOnCompletion(Boolean snapshotDatasetsOnCompletion) {
this.snapshotDatasetsOnCompletion = snapshotDatasetsOnCompletion;
return this;
}
/**
* Get snapshotDatasetsOnCompletion
* @return snapshotDatasetsOnCompletion
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_SNAPSHOT_DATASETS_ON_COMPLETION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getSnapshotDatasetsOnCompletion() {
return snapshotDatasetsOnCompletion;
}
@JsonProperty(JSON_PROPERTY_SNAPSHOT_DATASETS_ON_COMPLETION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSnapshotDatasetsOnCompletion(Boolean snapshotDatasetsOnCompletion) {
this.snapshotDatasetsOnCompletion = snapshotDatasetsOnCompletion;
}
/**
* Return true if this domino.scheduledjob.api.UpdatedScheduledJobDTO object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DominoScheduledjobApiUpdatedScheduledJobDTO dominoScheduledjobApiUpdatedScheduledJobDTO = (DominoScheduledjobApiUpdatedScheduledJobDTO) o;
return Objects.equals(this.title, dominoScheduledjobApiUpdatedScheduledJobDTO.title) &&
Objects.equals(this.command, dominoScheduledjobApiUpdatedScheduledJobDTO.command) &&
Objects.equals(this.schedule, dominoScheduledjobApiUpdatedScheduledJobDTO.schedule) &&
Objects.equals(this.timezoneId, dominoScheduledjobApiUpdatedScheduledJobDTO.timezoneId) &&
Objects.equals(this.isPaused, dominoScheduledjobApiUpdatedScheduledJobDTO.isPaused) &&
Objects.equals(this.publishModelId, dominoScheduledjobApiUpdatedScheduledJobDTO.publishModelId) &&
Objects.equals(this.allowConcurrentExecution, dominoScheduledjobApiUpdatedScheduledJobDTO.allowConcurrentExecution) &&
Objects.equals(this.hardwareTierIdentifier, dominoScheduledjobApiUpdatedScheduledJobDTO.hardwareTierIdentifier) &&
Objects.equals(this.overrideEnvironmentId, dominoScheduledjobApiUpdatedScheduledJobDTO.overrideEnvironmentId) &&
Objects.equals(this.environmentRevisionSpec, dominoScheduledjobApiUpdatedScheduledJobDTO.environmentRevisionSpec) &&
Objects.equals(this.scheduledByUserId, dominoScheduledjobApiUpdatedScheduledJobDTO.scheduledByUserId) &&
Objects.equals(this.notifyOnCompleteEmailAddresses, dominoScheduledjobApiUpdatedScheduledJobDTO.notifyOnCompleteEmailAddresses) &&
Objects.equals(this.datasetConfig, dominoScheduledjobApiUpdatedScheduledJobDTO.datasetConfig) &&
Objects.equals(this.computeClusterProperties, dominoScheduledjobApiUpdatedScheduledJobDTO.computeClusterProperties) &&
Objects.equals(this.volumeSpecificationOverride, dominoScheduledjobApiUpdatedScheduledJobDTO.volumeSpecificationOverride) &&
Objects.equals(this.mainRepoGitRef, dominoScheduledjobApiUpdatedScheduledJobDTO.mainRepoGitRef) &&
Objects.equals(this.snapshotDatasetsOnCompletion, dominoScheduledjobApiUpdatedScheduledJobDTO.snapshotDatasetsOnCompletion);
}
@Override
public int hashCode() {
return Objects.hash(title, command, schedule, timezoneId, isPaused, publishModelId, allowConcurrentExecution, hardwareTierIdentifier, overrideEnvironmentId, environmentRevisionSpec, scheduledByUserId, notifyOnCompleteEmailAddresses, datasetConfig, computeClusterProperties, volumeSpecificationOverride, mainRepoGitRef, snapshotDatasetsOnCompletion);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DominoScheduledjobApiUpdatedScheduledJobDTO {\n");
sb.append(" title: ").append(toIndentedString(title)).append("\n");
sb.append(" command: ").append(toIndentedString(command)).append("\n");
sb.append(" schedule: ").append(toIndentedString(schedule)).append("\n");
sb.append(" timezoneId: ").append(toIndentedString(timezoneId)).append("\n");
sb.append(" isPaused: ").append(toIndentedString(isPaused)).append("\n");
sb.append(" publishModelId: ").append(toIndentedString(publishModelId)).append("\n");
sb.append(" allowConcurrentExecution: ").append(toIndentedString(allowConcurrentExecution)).append("\n");
sb.append(" hardwareTierIdentifier: ").append(toIndentedString(hardwareTierIdentifier)).append("\n");
sb.append(" overrideEnvironmentId: ").append(toIndentedString(overrideEnvironmentId)).append("\n");
sb.append(" environmentRevisionSpec: ").append(toIndentedString(environmentRevisionSpec)).append("\n");
sb.append(" scheduledByUserId: ").append(toIndentedString(scheduledByUserId)).append("\n");
sb.append(" notifyOnCompleteEmailAddresses: ").append(toIndentedString(notifyOnCompleteEmailAddresses)).append("\n");
sb.append(" datasetConfig: ").append(toIndentedString(datasetConfig)).append("\n");
sb.append(" computeClusterProperties: ").append(toIndentedString(computeClusterProperties)).append("\n");
sb.append(" volumeSpecificationOverride: ").append(toIndentedString(volumeSpecificationOverride)).append("\n");
sb.append(" mainRepoGitRef: ").append(toIndentedString(mainRepoGitRef)).append("\n");
sb.append(" snapshotDatasetsOnCompletion: ").append(toIndentedString(snapshotDatasetsOnCompletion)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `title` to the URL query string
if (getTitle() != null) {
joiner.add(String.format("%stitle%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getTitle()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `command` to the URL query string
if (getCommand() != null) {
joiner.add(String.format("%scommand%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getCommand()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `schedule` to the URL query string
if (getSchedule() != null) {
joiner.add(getSchedule().toUrlQueryString(prefix + "schedule" + suffix));
}
// add `timezoneId` to the URL query string
if (getTimezoneId() != null) {
joiner.add(String.format("%stimezoneId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getTimezoneId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `isPaused` to the URL query string
if (getIsPaused() != null) {
joiner.add(String.format("%sisPaused%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getIsPaused()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `publishModelId` to the URL query string
if (getPublishModelId() != null) {
joiner.add(String.format("%spublishModelId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getPublishModelId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `allowConcurrentExecution` to the URL query string
if (getAllowConcurrentExecution() != null) {
joiner.add(String.format("%sallowConcurrentExecution%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getAllowConcurrentExecution()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `hardwareTierIdentifier` to the URL query string
if (getHardwareTierIdentifier() != null) {
joiner.add(String.format("%shardwareTierIdentifier%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getHardwareTierIdentifier()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `overrideEnvironmentId` to the URL query string
if (getOverrideEnvironmentId() != null) {
joiner.add(String.format("%soverrideEnvironmentId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getOverrideEnvironmentId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `environmentRevisionSpec` to the URL query string
if (getEnvironmentRevisionSpec() != null) {
joiner.add(getEnvironmentRevisionSpec().toUrlQueryString(prefix + "environmentRevisionSpec" + suffix));
}
// add `scheduledByUserId` to the URL query string
if (getScheduledByUserId() != null) {
joiner.add(String.format("%sscheduledByUserId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getScheduledByUserId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `notifyOnCompleteEmailAddresses` to the URL query string
if (getNotifyOnCompleteEmailAddresses() != null) {
for (int i = 0; i < getNotifyOnCompleteEmailAddresses().size(); i++) {
joiner.add(String.format("%snotifyOnCompleteEmailAddresses%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getNotifyOnCompleteEmailAddresses().get(i)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
// add `datasetConfig` to the URL query string
if (getDatasetConfig() != null) {
joiner.add(String.format("%sdatasetConfig%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getDatasetConfig()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `computeClusterProperties` to the URL query string
if (getComputeClusterProperties() != null) {
joiner.add(getComputeClusterProperties().toUrlQueryString(prefix + "computeClusterProperties" + suffix));
}
// add `volumeSpecificationOverride` to the URL query string
if (getVolumeSpecificationOverride() != null) {
for (String _key : getVolumeSpecificationOverride().keySet()) {
joiner.add(String.format("%svolumeSpecificationOverride%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, _key, containerSuffix),
getVolumeSpecificationOverride().get(_key), URLEncoder.encode(String.valueOf(getVolumeSpecificationOverride().get(_key)), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
}
// add `mainRepoGitRef` to the URL query string
if (getMainRepoGitRef() != null) {
joiner.add(getMainRepoGitRef().toUrlQueryString(prefix + "mainRepoGitRef" + suffix));
}
// add `snapshotDatasetsOnCompletion` to the URL query string
if (getSnapshotDatasetsOnCompletion() != null) {
joiner.add(String.format("%ssnapshotDatasetsOnCompletion%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getSnapshotDatasetsOnCompletion()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy