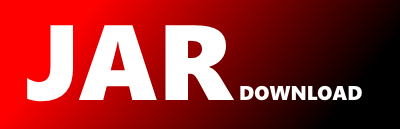
com.dominodatalab.api.model.DominoServerControlcenterUserStatsDTO Maven / Gradle / Ivy
/*
* Domino Data Lab API v4
* This API is going to provide access to all the Domino functions available in the user interface. To authenticate your requests, include your API Key (which you can find on your account page) with the header X-Domino-Api-Key.
*
* The version of the OpenAPI document: 4.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.api.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.dominodatalab.api.model.DominoServerControlcenterMoneyDTO;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* DominoServerControlcenterUserStatsDTO
*/
@JsonPropertyOrder({
DominoServerControlcenterUserStatsDTO.JSON_PROPERTY_ID,
DominoServerControlcenterUserStatsDTO.JSON_PROPERTY_FULL_NAME,
DominoServerControlcenterUserStatsDTO.JSON_PROPERTY_TOTAL_COMPUTE_TIME_IN_MILLIS,
DominoServerControlcenterUserStatsDTO.JSON_PROPERTY_TOTAL_COMPUTE_SPEND,
DominoServerControlcenterUserStatsDTO.JSON_PROPERTY_NUMBER_OF_RUNS,
DominoServerControlcenterUserStatsDTO.JSON_PROPERTY_NUMBER_OF_PROJECTS_WITH_RUNS,
DominoServerControlcenterUserStatsDTO.JSON_PROPERTY_USERNAME
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2023-10-17T15:20:46.682098100-04:00[America/New_York]")
public class DominoServerControlcenterUserStatsDTO {
public static final String JSON_PROPERTY_ID = "id";
private String id;
public static final String JSON_PROPERTY_FULL_NAME = "fullName";
private String fullName;
public static final String JSON_PROPERTY_TOTAL_COMPUTE_TIME_IN_MILLIS = "totalComputeTimeInMillis";
private Long totalComputeTimeInMillis;
public static final String JSON_PROPERTY_TOTAL_COMPUTE_SPEND = "totalComputeSpend";
private DominoServerControlcenterMoneyDTO totalComputeSpend;
public static final String JSON_PROPERTY_NUMBER_OF_RUNS = "numberOfRuns";
private Integer numberOfRuns;
public static final String JSON_PROPERTY_NUMBER_OF_PROJECTS_WITH_RUNS = "numberOfProjectsWithRuns";
private Integer numberOfProjectsWithRuns;
public static final String JSON_PROPERTY_USERNAME = "username";
private String username;
public DominoServerControlcenterUserStatsDTO() {
}
public DominoServerControlcenterUserStatsDTO id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getId() {
return id;
}
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setId(String id) {
this.id = id;
}
public DominoServerControlcenterUserStatsDTO fullName(String fullName) {
this.fullName = fullName;
return this;
}
/**
* Get fullName
* @return fullName
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_FULL_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getFullName() {
return fullName;
}
@JsonProperty(JSON_PROPERTY_FULL_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setFullName(String fullName) {
this.fullName = fullName;
}
public DominoServerControlcenterUserStatsDTO totalComputeTimeInMillis(Long totalComputeTimeInMillis) {
this.totalComputeTimeInMillis = totalComputeTimeInMillis;
return this;
}
/**
* Get totalComputeTimeInMillis
* @return totalComputeTimeInMillis
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_TOTAL_COMPUTE_TIME_IN_MILLIS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Long getTotalComputeTimeInMillis() {
return totalComputeTimeInMillis;
}
@JsonProperty(JSON_PROPERTY_TOTAL_COMPUTE_TIME_IN_MILLIS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setTotalComputeTimeInMillis(Long totalComputeTimeInMillis) {
this.totalComputeTimeInMillis = totalComputeTimeInMillis;
}
public DominoServerControlcenterUserStatsDTO totalComputeSpend(DominoServerControlcenterMoneyDTO totalComputeSpend) {
this.totalComputeSpend = totalComputeSpend;
return this;
}
/**
* Get totalComputeSpend
* @return totalComputeSpend
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_TOTAL_COMPUTE_SPEND)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public DominoServerControlcenterMoneyDTO getTotalComputeSpend() {
return totalComputeSpend;
}
@JsonProperty(JSON_PROPERTY_TOTAL_COMPUTE_SPEND)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setTotalComputeSpend(DominoServerControlcenterMoneyDTO totalComputeSpend) {
this.totalComputeSpend = totalComputeSpend;
}
public DominoServerControlcenterUserStatsDTO numberOfRuns(Integer numberOfRuns) {
this.numberOfRuns = numberOfRuns;
return this;
}
/**
* Get numberOfRuns
* @return numberOfRuns
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_NUMBER_OF_RUNS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Integer getNumberOfRuns() {
return numberOfRuns;
}
@JsonProperty(JSON_PROPERTY_NUMBER_OF_RUNS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setNumberOfRuns(Integer numberOfRuns) {
this.numberOfRuns = numberOfRuns;
}
public DominoServerControlcenterUserStatsDTO numberOfProjectsWithRuns(Integer numberOfProjectsWithRuns) {
this.numberOfProjectsWithRuns = numberOfProjectsWithRuns;
return this;
}
/**
* Get numberOfProjectsWithRuns
* @return numberOfProjectsWithRuns
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_NUMBER_OF_PROJECTS_WITH_RUNS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Integer getNumberOfProjectsWithRuns() {
return numberOfProjectsWithRuns;
}
@JsonProperty(JSON_PROPERTY_NUMBER_OF_PROJECTS_WITH_RUNS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setNumberOfProjectsWithRuns(Integer numberOfProjectsWithRuns) {
this.numberOfProjectsWithRuns = numberOfProjectsWithRuns;
}
public DominoServerControlcenterUserStatsDTO username(String username) {
this.username = username;
return this;
}
/**
* Get username
* @return username
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_USERNAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getUsername() {
return username;
}
@JsonProperty(JSON_PROPERTY_USERNAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setUsername(String username) {
this.username = username;
}
/**
* Return true if this domino.server.controlcenter.UserStatsDTO object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DominoServerControlcenterUserStatsDTO dominoServerControlcenterUserStatsDTO = (DominoServerControlcenterUserStatsDTO) o;
return Objects.equals(this.id, dominoServerControlcenterUserStatsDTO.id) &&
Objects.equals(this.fullName, dominoServerControlcenterUserStatsDTO.fullName) &&
Objects.equals(this.totalComputeTimeInMillis, dominoServerControlcenterUserStatsDTO.totalComputeTimeInMillis) &&
Objects.equals(this.totalComputeSpend, dominoServerControlcenterUserStatsDTO.totalComputeSpend) &&
Objects.equals(this.numberOfRuns, dominoServerControlcenterUserStatsDTO.numberOfRuns) &&
Objects.equals(this.numberOfProjectsWithRuns, dominoServerControlcenterUserStatsDTO.numberOfProjectsWithRuns) &&
Objects.equals(this.username, dominoServerControlcenterUserStatsDTO.username);
}
@Override
public int hashCode() {
return Objects.hash(id, fullName, totalComputeTimeInMillis, totalComputeSpend, numberOfRuns, numberOfProjectsWithRuns, username);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DominoServerControlcenterUserStatsDTO {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" fullName: ").append(toIndentedString(fullName)).append("\n");
sb.append(" totalComputeTimeInMillis: ").append(toIndentedString(totalComputeTimeInMillis)).append("\n");
sb.append(" totalComputeSpend: ").append(toIndentedString(totalComputeSpend)).append("\n");
sb.append(" numberOfRuns: ").append(toIndentedString(numberOfRuns)).append("\n");
sb.append(" numberOfProjectsWithRuns: ").append(toIndentedString(numberOfProjectsWithRuns)).append("\n");
sb.append(" username: ").append(toIndentedString(username)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `id` to the URL query string
if (getId() != null) {
joiner.add(String.format("%sid%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `fullName` to the URL query string
if (getFullName() != null) {
joiner.add(String.format("%sfullName%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getFullName()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `totalComputeTimeInMillis` to the URL query string
if (getTotalComputeTimeInMillis() != null) {
joiner.add(String.format("%stotalComputeTimeInMillis%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getTotalComputeTimeInMillis()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `totalComputeSpend` to the URL query string
if (getTotalComputeSpend() != null) {
joiner.add(getTotalComputeSpend().toUrlQueryString(prefix + "totalComputeSpend" + suffix));
}
// add `numberOfRuns` to the URL query string
if (getNumberOfRuns() != null) {
joiner.add(String.format("%snumberOfRuns%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getNumberOfRuns()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `numberOfProjectsWithRuns` to the URL query string
if (getNumberOfProjectsWithRuns() != null) {
joiner.add(String.format("%snumberOfProjectsWithRuns%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getNumberOfProjectsWithRuns()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `username` to the URL query string
if (getUsername() != null) {
joiner.add(String.format("%susername%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getUsername()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy