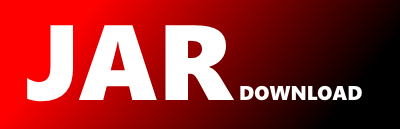
com.dominodatalab.api.model.DominoWorkspacesApiDependentDatasetMount Maven / Gradle / Ivy
/*
* Domino Data Lab API v4
* This API is going to provide access to all the Domino functions available in the user interface. To authenticate your requests, include your API Key (which you can find on your account page) with the header X-Domino-Api-Key.
*
* The version of the OpenAPI document: 4.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.api.model;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.StringJoiner;
import java.util.Objects;
import java.util.Map;
import java.util.HashMap;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* DominoWorkspacesApiDependentDatasetMount
*/
@JsonPropertyOrder({
DominoWorkspacesApiDependentDatasetMount.JSON_PROPERTY_DATASET_ID,
DominoWorkspacesApiDependentDatasetMount.JSON_PROPERTY_SNAPSHOT_ID,
DominoWorkspacesApiDependentDatasetMount.JSON_PROPERTY_SNAPSHOT_VERSION,
DominoWorkspacesApiDependentDatasetMount.JSON_PROPERTY_DATASET_NAME,
DominoWorkspacesApiDependentDatasetMount.JSON_PROPERTY_PROJECT_ID,
DominoWorkspacesApiDependentDatasetMount.JSON_PROPERTY_IS_INPUT,
DominoWorkspacesApiDependentDatasetMount.JSON_PROPERTY_CONTAINER_PATH
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2023-10-17T15:20:46.682098100-04:00[America/New_York]")
public class DominoWorkspacesApiDependentDatasetMount {
public static final String JSON_PROPERTY_DATASET_ID = "datasetId";
private String datasetId;
public static final String JSON_PROPERTY_SNAPSHOT_ID = "snapshotId";
private String snapshotId;
public static final String JSON_PROPERTY_SNAPSHOT_VERSION = "snapshotVersion";
private Integer snapshotVersion;
public static final String JSON_PROPERTY_DATASET_NAME = "datasetName";
private String datasetName;
public static final String JSON_PROPERTY_PROJECT_ID = "projectId";
private String projectId;
public static final String JSON_PROPERTY_IS_INPUT = "isInput";
private Boolean isInput;
public static final String JSON_PROPERTY_CONTAINER_PATH = "containerPath";
private String containerPath;
public DominoWorkspacesApiDependentDatasetMount() {
}
public DominoWorkspacesApiDependentDatasetMount datasetId(String datasetId) {
this.datasetId = datasetId;
return this;
}
/**
* Get datasetId
* @return datasetId
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_DATASET_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getDatasetId() {
return datasetId;
}
@JsonProperty(JSON_PROPERTY_DATASET_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDatasetId(String datasetId) {
this.datasetId = datasetId;
}
public DominoWorkspacesApiDependentDatasetMount snapshotId(String snapshotId) {
this.snapshotId = snapshotId;
return this;
}
/**
* Get snapshotId
* @return snapshotId
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_SNAPSHOT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getSnapshotId() {
return snapshotId;
}
@JsonProperty(JSON_PROPERTY_SNAPSHOT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSnapshotId(String snapshotId) {
this.snapshotId = snapshotId;
}
public DominoWorkspacesApiDependentDatasetMount snapshotVersion(Integer snapshotVersion) {
this.snapshotVersion = snapshotVersion;
return this;
}
/**
* Get snapshotVersion
* @return snapshotVersion
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_SNAPSHOT_VERSION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getSnapshotVersion() {
return snapshotVersion;
}
@JsonProperty(JSON_PROPERTY_SNAPSHOT_VERSION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSnapshotVersion(Integer snapshotVersion) {
this.snapshotVersion = snapshotVersion;
}
public DominoWorkspacesApiDependentDatasetMount datasetName(String datasetName) {
this.datasetName = datasetName;
return this;
}
/**
* Get datasetName
* @return datasetName
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_DATASET_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getDatasetName() {
return datasetName;
}
@JsonProperty(JSON_PROPERTY_DATASET_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDatasetName(String datasetName) {
this.datasetName = datasetName;
}
public DominoWorkspacesApiDependentDatasetMount projectId(String projectId) {
this.projectId = projectId;
return this;
}
/**
* Get projectId
* @return projectId
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_PROJECT_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getProjectId() {
return projectId;
}
@JsonProperty(JSON_PROPERTY_PROJECT_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setProjectId(String projectId) {
this.projectId = projectId;
}
public DominoWorkspacesApiDependentDatasetMount isInput(Boolean isInput) {
this.isInput = isInput;
return this;
}
/**
* Get isInput
* @return isInput
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_IS_INPUT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getIsInput() {
return isInput;
}
@JsonProperty(JSON_PROPERTY_IS_INPUT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setIsInput(Boolean isInput) {
this.isInput = isInput;
}
public DominoWorkspacesApiDependentDatasetMount containerPath(String containerPath) {
this.containerPath = containerPath;
return this;
}
/**
* Get containerPath
* @return containerPath
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_CONTAINER_PATH)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getContainerPath() {
return containerPath;
}
@JsonProperty(JSON_PROPERTY_CONTAINER_PATH)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setContainerPath(String containerPath) {
this.containerPath = containerPath;
}
/**
* Return true if this domino.workspaces.api.DependentDatasetMount object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DominoWorkspacesApiDependentDatasetMount dominoWorkspacesApiDependentDatasetMount = (DominoWorkspacesApiDependentDatasetMount) o;
return Objects.equals(this.datasetId, dominoWorkspacesApiDependentDatasetMount.datasetId) &&
Objects.equals(this.snapshotId, dominoWorkspacesApiDependentDatasetMount.snapshotId) &&
Objects.equals(this.snapshotVersion, dominoWorkspacesApiDependentDatasetMount.snapshotVersion) &&
Objects.equals(this.datasetName, dominoWorkspacesApiDependentDatasetMount.datasetName) &&
Objects.equals(this.projectId, dominoWorkspacesApiDependentDatasetMount.projectId) &&
Objects.equals(this.isInput, dominoWorkspacesApiDependentDatasetMount.isInput) &&
Objects.equals(this.containerPath, dominoWorkspacesApiDependentDatasetMount.containerPath);
}
@Override
public int hashCode() {
return Objects.hash(datasetId, snapshotId, snapshotVersion, datasetName, projectId, isInput, containerPath);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DominoWorkspacesApiDependentDatasetMount {\n");
sb.append(" datasetId: ").append(toIndentedString(datasetId)).append("\n");
sb.append(" snapshotId: ").append(toIndentedString(snapshotId)).append("\n");
sb.append(" snapshotVersion: ").append(toIndentedString(snapshotVersion)).append("\n");
sb.append(" datasetName: ").append(toIndentedString(datasetName)).append("\n");
sb.append(" projectId: ").append(toIndentedString(projectId)).append("\n");
sb.append(" isInput: ").append(toIndentedString(isInput)).append("\n");
sb.append(" containerPath: ").append(toIndentedString(containerPath)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `datasetId` to the URL query string
if (getDatasetId() != null) {
joiner.add(String.format("%sdatasetId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getDatasetId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `snapshotId` to the URL query string
if (getSnapshotId() != null) {
joiner.add(String.format("%ssnapshotId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getSnapshotId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `snapshotVersion` to the URL query string
if (getSnapshotVersion() != null) {
joiner.add(String.format("%ssnapshotVersion%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getSnapshotVersion()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `datasetName` to the URL query string
if (getDatasetName() != null) {
joiner.add(String.format("%sdatasetName%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getDatasetName()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `projectId` to the URL query string
if (getProjectId() != null) {
joiner.add(String.format("%sprojectId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getProjectId()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `isInput` to the URL query string
if (getIsInput() != null) {
joiner.add(String.format("%sisInput%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getIsInput()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
// add `containerPath` to the URL query string
if (getContainerPath() != null) {
joiner.add(String.format("%scontainerPath%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getContainerPath()), StandardCharsets.UTF_8).replaceAll("\\+", "%20")));
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy