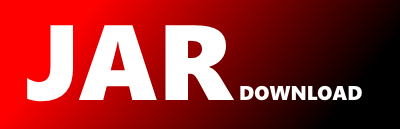
com.dominodatalab.api.rest.ActionableInsightsApi Maven / Gradle / Ivy
/*
* Domino Data Lab API v4
* This API is going to provide access to all the Domino functions available in the user interface. To authenticate your requests, include your API Key (which you can find on your account page) with the header X-Domino-Api-Key.
*
* The version of the OpenAPI document: 4.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.api.rest;
import com.dominodatalab.api.invoker.ApiClient;
import com.dominodatalab.api.invoker.ApiException;
import com.dominodatalab.api.invoker.ApiResponse;
import com.dominodatalab.api.invoker.Pair;
import com.dominodatalab.api.model.DominoApiErrorResponse;
import com.dominodatalab.api.model.DominoProjectsApiProjectSummary;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.InputStream;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.IOException;
import java.io.OutputStream;
import java.net.http.HttpRequest;
import java.nio.channels.Channels;
import java.nio.channels.Pipe;
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.time.Duration;
import java.util.ArrayList;
import java.util.StringJoiner;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.function.Consumer;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2023-10-17T15:20:46.682098100-04:00[America/New_York]")
public class ActionableInsightsApi {
private final HttpClient memberVarHttpClient;
private final ObjectMapper memberVarObjectMapper;
private final String memberVarBaseUri;
private final Consumer memberVarInterceptor;
private final Duration memberVarReadTimeout;
private final Consumer> memberVarResponseInterceptor;
private final Consumer> memberVarAsyncResponseInterceptor;
public ActionableInsightsApi() {
this(new ApiClient());
}
public ActionableInsightsApi(ApiClient apiClient) {
memberVarHttpClient = apiClient.getHttpClient();
memberVarObjectMapper = apiClient.getObjectMapper();
memberVarBaseUri = apiClient.getBaseUri();
memberVarInterceptor = apiClient.getRequestInterceptor();
memberVarReadTimeout = apiClient.getReadTimeout();
memberVarResponseInterceptor = apiClient.getResponseInterceptor();
memberVarAsyncResponseInterceptor = apiClient.getAsyncResponseInterceptor();
}
protected ApiException getApiException(String operationId, HttpResponse response) throws IOException {
String body = response.body() == null ? null : new String(response.body().readAllBytes());
String message = formatExceptionMessage(operationId, response.statusCode(), body);
return new ApiException(response.statusCode(), message, response.headers(), body);
}
private String formatExceptionMessage(String operationId, int statusCode, String body) {
if (body == null || body.isEmpty()) {
body = "[no body]";
}
return operationId + " call failed with: " + statusCode + " - " + body;
}
/**
* retrieves the project to be used for Actionable Insights for a Model
*
* @param userEmail Id of the user (required)
* @param projectId Id of the project (optional)
* @return DominoProjectsApiProjectSummary
* @throws ApiException if fails to make API call
*/
public DominoProjectsApiProjectSummary getProjectForActionableInsights(String userEmail, String projectId) throws ApiException {
ApiResponse localVarResponse = getProjectForActionableInsightsWithHttpInfo(userEmail, projectId);
return localVarResponse.getData();
}
/**
* retrieves the project to be used for Actionable Insights for a Model
*
* @param userEmail Id of the user (required)
* @param projectId Id of the project (optional)
* @return ApiResponse<DominoProjectsApiProjectSummary>
* @throws ApiException if fails to make API call
*/
public ApiResponse getProjectForActionableInsightsWithHttpInfo(String userEmail, String projectId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getProjectForActionableInsightsRequestBuilder(userEmail, projectId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getProjectForActionableInsights", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getProjectForActionableInsightsRequestBuilder(String userEmail, String projectId) throws ApiException {
// verify the required parameter 'userEmail' is set
if (userEmail == null) {
throw new ApiException(400, "Missing the required parameter 'userEmail' when calling getProjectForActionableInsights");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/projects/actionableInsights/projects";
List localVarQueryParams = new ArrayList<>();
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
localVarQueryParameterBaseName = "userEmail";
localVarQueryParams.addAll(ApiClient.parameterToPairs("userEmail", userEmail));
localVarQueryParameterBaseName = "projectId";
localVarQueryParams.addAll(ApiClient.parameterToPairs("projectId", projectId));
if (!localVarQueryParams.isEmpty() || localVarQueryStringJoiner.length() != 0) {
StringJoiner queryJoiner = new StringJoiner("&");
localVarQueryParams.forEach(p -> queryJoiner.add(p.getName() + '=' + p.getValue()));
if (localVarQueryStringJoiner.length() != 0) {
queryJoiner.add(localVarQueryStringJoiner.toString());
}
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath + '?' + queryJoiner.toString()));
} else {
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
}
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Run Actionable Insights Job
*
* @param userEmail Email of the user (required)
* @param projectId Id of the project (required)
* @param commandParameters Command parameters that needs to be passed to the job (required)
* @return DominoProjectsApiProjectSummary
* @throws ApiException if fails to make API call
*/
public DominoProjectsApiProjectSummary startActionableInsightsJob(String userEmail, String projectId, String commandParameters) throws ApiException {
ApiResponse localVarResponse = startActionableInsightsJobWithHttpInfo(userEmail, projectId, commandParameters);
return localVarResponse.getData();
}
/**
* Run Actionable Insights Job
*
* @param userEmail Email of the user (required)
* @param projectId Id of the project (required)
* @param commandParameters Command parameters that needs to be passed to the job (required)
* @return ApiResponse<DominoProjectsApiProjectSummary>
* @throws ApiException if fails to make API call
*/
public ApiResponse startActionableInsightsJobWithHttpInfo(String userEmail, String projectId, String commandParameters) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = startActionableInsightsJobRequestBuilder(userEmail, projectId, commandParameters);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("startActionableInsightsJob", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder startActionableInsightsJobRequestBuilder(String userEmail, String projectId, String commandParameters) throws ApiException {
// verify the required parameter 'userEmail' is set
if (userEmail == null) {
throw new ApiException(400, "Missing the required parameter 'userEmail' when calling startActionableInsightsJob");
}
// verify the required parameter 'projectId' is set
if (projectId == null) {
throw new ApiException(400, "Missing the required parameter 'projectId' when calling startActionableInsightsJob");
}
// verify the required parameter 'commandParameters' is set
if (commandParameters == null) {
throw new ApiException(400, "Missing the required parameter 'commandParameters' when calling startActionableInsightsJob");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/jobs/actionableInsights/startJob";
List localVarQueryParams = new ArrayList<>();
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
localVarQueryParameterBaseName = "userEmail";
localVarQueryParams.addAll(ApiClient.parameterToPairs("userEmail", userEmail));
localVarQueryParameterBaseName = "projectId";
localVarQueryParams.addAll(ApiClient.parameterToPairs("projectId", projectId));
localVarQueryParameterBaseName = "commandParameters";
localVarQueryParams.addAll(ApiClient.parameterToPairs("commandParameters", commandParameters));
if (!localVarQueryParams.isEmpty() || localVarQueryStringJoiner.length() != 0) {
StringJoiner queryJoiner = new StringJoiner("&");
localVarQueryParams.forEach(p -> queryJoiner.add(p.getName() + '=' + p.getValue()));
if (localVarQueryStringJoiner.length() != 0) {
queryJoiner.add(localVarQueryStringJoiner.toString());
}
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath + '?' + queryJoiner.toString()));
} else {
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
}
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy