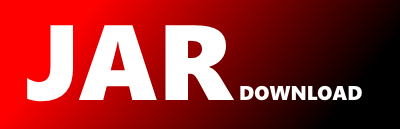
com.dominodatalab.api.rest.AdminApi Maven / Gradle / Ivy
/*
* Domino Data Lab API v4
* This API is going to provide access to all the Domino functions available in the user interface. To authenticate your requests, include your API Key (which you can find on your account page) with the header X-Domino-Api-Key.
*
* The version of the OpenAPI document: 4.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.api.rest;
import com.dominodatalab.api.invoker.ApiClient;
import com.dominodatalab.api.invoker.ApiException;
import com.dominodatalab.api.invoker.ApiResponse;
import com.dominodatalab.api.invoker.Pair;
import com.dominodatalab.api.model.DominoAdminInterfaceComputeNodeInfrastructureInfo;
import com.dominodatalab.api.model.DominoAdminInterfaceComputeNodeOverview;
import com.dominodatalab.api.model.DominoAdminInterfaceExecutionOverview;
import com.dominodatalab.api.model.DominoAdminInterfaceProjectSearchResultsDto;
import com.dominodatalab.api.model.DominoApiErrorResponse;
import java.io.File;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.InputStream;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.IOException;
import java.io.OutputStream;
import java.net.http.HttpRequest;
import java.nio.channels.Channels;
import java.nio.channels.Pipe;
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.time.Duration;
import java.util.ArrayList;
import java.util.StringJoiner;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.function.Consumer;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2023-10-17T15:20:46.682098100-04:00[America/New_York]")
public class AdminApi {
private final HttpClient memberVarHttpClient;
private final ObjectMapper memberVarObjectMapper;
private final String memberVarBaseUri;
private final Consumer memberVarInterceptor;
private final Duration memberVarReadTimeout;
private final Consumer> memberVarResponseInterceptor;
private final Consumer> memberVarAsyncResponseInterceptor;
public AdminApi() {
this(new ApiClient());
}
public AdminApi(ApiClient apiClient) {
memberVarHttpClient = apiClient.getHttpClient();
memberVarObjectMapper = apiClient.getObjectMapper();
memberVarBaseUri = apiClient.getBaseUri();
memberVarInterceptor = apiClient.getRequestInterceptor();
memberVarReadTimeout = apiClient.getReadTimeout();
memberVarResponseInterceptor = apiClient.getResponseInterceptor();
memberVarAsyncResponseInterceptor = apiClient.getAsyncResponseInterceptor();
}
protected ApiException getApiException(String operationId, HttpResponse response) throws IOException {
String body = response.body() == null ? null : new String(response.body().readAllBytes());
String message = formatExceptionMessage(operationId, response.statusCode(), body);
return new ApiException(response.statusCode(), message, response.headers(), body);
}
private String formatExceptionMessage(String operationId, int statusCode, String body) {
if (body == null || body.isEmpty()) {
body = "[no body]";
}
return operationId + " call failed with: " + statusCode + " - " + body;
}
/**
* Gets all non-completed executions (running on the Kubernetes Compute Grid, for now)
*
* @return List<DominoAdminInterfaceExecutionOverview>
* @throws ApiException if fails to make API call
*/
public List getCurrentExecutions() throws ApiException {
ApiResponse> localVarResponse = getCurrentExecutionsWithHttpInfo();
return localVarResponse.getData();
}
/**
* Gets all non-completed executions (running on the Kubernetes Compute Grid, for now)
*
* @return ApiResponse<List<DominoAdminInterfaceExecutionOverview>>
* @throws ApiException if fails to make API call
*/
public ApiResponse> getCurrentExecutionsWithHttpInfo() throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getCurrentExecutionsRequestBuilder();
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getCurrentExecutions", localVarResponse);
}
return new ApiResponse>(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference>() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getCurrentExecutionsRequestBuilder() throws ApiException {
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/admin/executions";
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Gets all dashboard entries
*
* @param offset The number of items to skip relative to the checkpoint project before starting to collect the page. If no checkpoint project is provided, will skip relative to the first project of the first user. Can be negative. (optional, default to 0)
* @param pageSize The size of the page to return. (optional, default to 50)
* @param checkpointProjectId The projectId to construct the page relative to. (optional)
* @param searchString Filters projects by owner name and project name substrings (optional)
* @param sortBy A column name to sort over. (optional)
* @param sortOrder order of sort (optional)
* @return DominoAdminInterfaceProjectSearchResultsDto
* @throws ApiException if fails to make API call
*/
public DominoAdminInterfaceProjectSearchResultsDto getDashboardEntries(Integer offset, Integer pageSize, String checkpointProjectId, String searchString, String sortBy, String sortOrder) throws ApiException {
ApiResponse localVarResponse = getDashboardEntriesWithHttpInfo(offset, pageSize, checkpointProjectId, searchString, sortBy, sortOrder);
return localVarResponse.getData();
}
/**
* Gets all dashboard entries
*
* @param offset The number of items to skip relative to the checkpoint project before starting to collect the page. If no checkpoint project is provided, will skip relative to the first project of the first user. Can be negative. (optional, default to 0)
* @param pageSize The size of the page to return. (optional, default to 50)
* @param checkpointProjectId The projectId to construct the page relative to. (optional)
* @param searchString Filters projects by owner name and project name substrings (optional)
* @param sortBy A column name to sort over. (optional)
* @param sortOrder order of sort (optional)
* @return ApiResponse<DominoAdminInterfaceProjectSearchResultsDto>
* @throws ApiException if fails to make API call
*/
public ApiResponse getDashboardEntriesWithHttpInfo(Integer offset, Integer pageSize, String checkpointProjectId, String searchString, String sortBy, String sortOrder) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getDashboardEntriesRequestBuilder(offset, pageSize, checkpointProjectId, searchString, sortBy, sortOrder);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getDashboardEntries", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getDashboardEntriesRequestBuilder(Integer offset, Integer pageSize, String checkpointProjectId, String searchString, String sortBy, String sortOrder) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/admin/dashboardEntries";
List localVarQueryParams = new ArrayList<>();
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
localVarQueryParameterBaseName = "offset";
localVarQueryParams.addAll(ApiClient.parameterToPairs("offset", offset));
localVarQueryParameterBaseName = "pageSize";
localVarQueryParams.addAll(ApiClient.parameterToPairs("pageSize", pageSize));
localVarQueryParameterBaseName = "checkpointProjectId";
localVarQueryParams.addAll(ApiClient.parameterToPairs("checkpointProjectId", checkpointProjectId));
localVarQueryParameterBaseName = "searchString";
localVarQueryParams.addAll(ApiClient.parameterToPairs("searchString", searchString));
localVarQueryParameterBaseName = "sortBy";
localVarQueryParams.addAll(ApiClient.parameterToPairs("sortBy", sortBy));
localVarQueryParameterBaseName = "sortOrder";
localVarQueryParams.addAll(ApiClient.parameterToPairs("sortOrder", sortOrder));
if (!localVarQueryParams.isEmpty() || localVarQueryStringJoiner.length() != 0) {
StringJoiner queryJoiner = new StringJoiner("&");
localVarQueryParams.forEach(p -> queryJoiner.add(p.getName() + '=' + p.getValue()));
if (localVarQueryStringJoiner.length() != 0) {
queryJoiner.add(localVarQueryStringJoiner.toString());
}
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath + '?' + queryJoiner.toString()));
} else {
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
}
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Gets a zipfile containing useful information about an executionId, FIXME should this path be /executions/executionId/supportbundle
*
* @param executionId (required)
* @return File
* @throws ApiException if fails to make API call
*/
public File getExecutionSupportBundle(String executionId) throws ApiException {
ApiResponse localVarResponse = getExecutionSupportBundleWithHttpInfo(executionId);
return localVarResponse.getData();
}
/**
* Gets a zipfile containing useful information about an executionId, FIXME should this path be /executions/executionId/supportbundle
*
* @param executionId (required)
* @return ApiResponse<File>
* @throws ApiException if fails to make API call
*/
public ApiResponse getExecutionSupportBundleWithHttpInfo(String executionId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getExecutionSupportBundleRequestBuilder(executionId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getExecutionSupportBundle", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getExecutionSupportBundleRequestBuilder(String executionId) throws ApiException {
// verify the required parameter 'executionId' is set
if (executionId == null) {
throw new ApiException(400, "Missing the required parameter 'executionId' when calling getExecutionSupportBundle");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/admin/supportbundle/{executionId}"
.replace("{executionId}", ApiClient.urlEncode(executionId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Gets information about all the nodes' names, instance types, and node pools in the cluster
*
* @return List<DominoAdminInterfaceComputeNodeInfrastructureInfo>
* @throws ApiException if fails to make API call
*/
public List getInfrastructureInfo() throws ApiException {
ApiResponse> localVarResponse = getInfrastructureInfoWithHttpInfo();
return localVarResponse.getData();
}
/**
* Gets information about all the nodes' names, instance types, and node pools in the cluster
*
* @return ApiResponse<List<DominoAdminInterfaceComputeNodeInfrastructureInfo>>
* @throws ApiException if fails to make API call
*/
public ApiResponse> getInfrastructureInfoWithHttpInfo() throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getInfrastructureInfoRequestBuilder();
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getInfrastructureInfo", localVarResponse);
}
return new ApiResponse>(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference>() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getInfrastructureInfoRequestBuilder() throws ApiException {
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/admin/infrastructure";
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Gets information about nodes in the compute cluster
*
* @return List<DominoAdminInterfaceComputeNodeOverview>
* @throws ApiException if fails to make API call
*/
public List getNodes() throws ApiException {
ApiResponse> localVarResponse = getNodesWithHttpInfo();
return localVarResponse.getData();
}
/**
* Gets information about nodes in the compute cluster
*
* @return ApiResponse<List<DominoAdminInterfaceComputeNodeOverview>>
* @throws ApiException if fails to make API call
*/
public ApiResponse> getNodesWithHttpInfo() throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getNodesRequestBuilder();
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getNodes", localVarResponse);
}
return new ApiResponse>(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference>() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getNodesRequestBuilder() throws ApiException {
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/admin/nodes";
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy