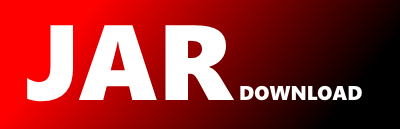
com.dominodatalab.api.rest.DatasetApi Maven / Gradle / Ivy
/*
* Domino Data Lab API v4
* This API is going to provide access to all the Domino functions available in the user interface. To authenticate your requests, include your API Key (which you can find on your account page) with the header X-Domino-Api-Key.
*
* The version of the OpenAPI document: 4.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.api.rest;
import com.dominodatalab.api.invoker.ApiClient;
import com.dominodatalab.api.invoker.ApiException;
import com.dominodatalab.api.invoker.ApiResponse;
import com.dominodatalab.api.invoker.Pair;
import com.dominodatalab.api.model.DominoApiErrorResponse;
import com.dominodatalab.api.model.DominoDatasetApiConsumedSnapshotDto;
import com.dominodatalab.api.model.DominoDatasetApiDatasetDto;
import com.dominodatalab.api.model.DominoDatasetApiDatasetScratchSpaceDto;
import com.dominodatalab.api.model.DominoDatasetApiDatasetSnapshotDto;
import com.dominodatalab.api.model.DominoDatasetApiDatasetSnapshotSummaryDto;
import com.dominodatalab.api.model.DominoNucleusDatasetUiAddDataSetTagInput;
import com.dominodatalab.api.model.DominoNucleusDatasetUiAddMountInput;
import com.dominodatalab.api.model.DominoNucleusDatasetUiCreateDatasetInput;
import com.dominodatalab.api.model.DominoNucleusDatasetUiCreateSnapshotWithRunInput;
import com.dominodatalab.api.model.DominoNucleusDatasetUiCreateSnapshotWithWorkspaceInput;
import com.dominodatalab.api.model.DominoNucleusDatasetUiDataSetFileBrowserRow;
import com.dominodatalab.api.model.DominoNucleusDatasetUiDataSetFileBrowserViewModel;
import com.dominodatalab.api.model.DominoNucleusDatasetUiDataSetRun;
import com.dominodatalab.api.model.DominoNucleusDatasetUiDatasetProjectMountSummaryViewModel;
import com.dominodatalab.api.model.DominoNucleusDatasetUiDatasetViewModel;
import com.dominodatalab.api.model.DominoNucleusDatasetUiDatasetWorkspace;
import com.dominodatalab.api.model.DominoNucleusDatasetUiMountConfigViewModel;
import com.dominodatalab.api.model.DominoNucleusDatasetUiSetLimitOverrideInput;
import com.dominodatalab.api.model.DominoNucleusDatasetUiUpdateDatasetInput;
import com.dominodatalab.api.model.DominoNucleusDatasetUiUpdateSnapshotStatusInput;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.InputStream;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.IOException;
import java.io.OutputStream;
import java.net.http.HttpRequest;
import java.nio.channels.Channels;
import java.nio.channels.Pipe;
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.time.Duration;
import java.util.ArrayList;
import java.util.StringJoiner;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.function.Consumer;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2023-10-17T15:20:46.682098100-04:00[America/New_York]")
public class DatasetApi {
private final HttpClient memberVarHttpClient;
private final ObjectMapper memberVarObjectMapper;
private final String memberVarBaseUri;
private final Consumer memberVarInterceptor;
private final Duration memberVarReadTimeout;
private final Consumer> memberVarResponseInterceptor;
private final Consumer> memberVarAsyncResponseInterceptor;
public DatasetApi() {
this(new ApiClient());
}
public DatasetApi(ApiClient apiClient) {
memberVarHttpClient = apiClient.getHttpClient();
memberVarObjectMapper = apiClient.getObjectMapper();
memberVarBaseUri = apiClient.getBaseUri();
memberVarInterceptor = apiClient.getRequestInterceptor();
memberVarReadTimeout = apiClient.getReadTimeout();
memberVarResponseInterceptor = apiClient.getResponseInterceptor();
memberVarAsyncResponseInterceptor = apiClient.getAsyncResponseInterceptor();
}
protected ApiException getApiException(String operationId, HttpResponse response) throws IOException {
String body = response.body() == null ? null : new String(response.body().readAllBytes());
String message = formatExceptionMessage(operationId, response.statusCode(), body);
return new ApiException(response.statusCode(), message, response.headers(), body);
}
private String formatExceptionMessage(String operationId, int statusCode, String body) {
if (body == null || body.isEmpty()) {
body = "[no body]";
}
return operationId + " call failed with: " + statusCode + " - " + body;
}
/**
* add project mount
*
* @param projectId the project id (required)
* @param dominoNucleusDatasetUiAddMountInput JSON object with information for new dataset tag (required)
* @return List<DominoNucleusDatasetUiMountConfigViewModel>
* @throws ApiException if fails to make API call
*/
public List addImportedProjectMount(String projectId, DominoNucleusDatasetUiAddMountInput dominoNucleusDatasetUiAddMountInput) throws ApiException {
ApiResponse> localVarResponse = addImportedProjectMountWithHttpInfo(projectId, dominoNucleusDatasetUiAddMountInput);
return localVarResponse.getData();
}
/**
* add project mount
*
* @param projectId the project id (required)
* @param dominoNucleusDatasetUiAddMountInput JSON object with information for new dataset tag (required)
* @return ApiResponse<List<DominoNucleusDatasetUiMountConfigViewModel>>
* @throws ApiException if fails to make API call
*/
public ApiResponse> addImportedProjectMountWithHttpInfo(String projectId, DominoNucleusDatasetUiAddMountInput dominoNucleusDatasetUiAddMountInput) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = addImportedProjectMountRequestBuilder(projectId, dominoNucleusDatasetUiAddMountInput);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("addImportedProjectMount", localVarResponse);
}
return new ApiResponse>(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference>() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder addImportedProjectMountRequestBuilder(String projectId, DominoNucleusDatasetUiAddMountInput dominoNucleusDatasetUiAddMountInput) throws ApiException {
// verify the required parameter 'projectId' is set
if (projectId == null) {
throw new ApiException(400, "Missing the required parameter 'projectId' when calling addImportedProjectMount");
}
// verify the required parameter 'dominoNucleusDatasetUiAddMountInput' is set
if (dominoNucleusDatasetUiAddMountInput == null) {
throw new ApiException(400, "Missing the required parameter 'dominoNucleusDatasetUiAddMountInput' when calling addImportedProjectMount");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/mounts/{projectId}/imported"
.replace("{projectId}", ApiClient.urlEncode(projectId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(dominoNucleusDatasetUiAddMountInput);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* adds a tag to a dataset
*
* @param datasetId Id of the collection of datasets (required)
* @param dominoNucleusDatasetUiAddDataSetTagInput JSON object with information for new dataset tag (required)
* @return DominoNucleusDatasetUiDatasetViewModel
* @throws ApiException if fails to make API call
*/
public DominoNucleusDatasetUiDatasetViewModel addTag(String datasetId, DominoNucleusDatasetUiAddDataSetTagInput dominoNucleusDatasetUiAddDataSetTagInput) throws ApiException {
ApiResponse localVarResponse = addTagWithHttpInfo(datasetId, dominoNucleusDatasetUiAddDataSetTagInput);
return localVarResponse.getData();
}
/**
* adds a tag to a dataset
*
* @param datasetId Id of the collection of datasets (required)
* @param dominoNucleusDatasetUiAddDataSetTagInput JSON object with information for new dataset tag (required)
* @return ApiResponse<DominoNucleusDatasetUiDatasetViewModel>
* @throws ApiException if fails to make API call
*/
public ApiResponse addTagWithHttpInfo(String datasetId, DominoNucleusDatasetUiAddDataSetTagInput dominoNucleusDatasetUiAddDataSetTagInput) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = addTagRequestBuilder(datasetId, dominoNucleusDatasetUiAddDataSetTagInput);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("addTag", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder addTagRequestBuilder(String datasetId, DominoNucleusDatasetUiAddDataSetTagInput dominoNucleusDatasetUiAddDataSetTagInput) throws ApiException {
// verify the required parameter 'datasetId' is set
if (datasetId == null) {
throw new ApiException(400, "Missing the required parameter 'datasetId' when calling addTag");
}
// verify the required parameter 'dominoNucleusDatasetUiAddDataSetTagInput' is set
if (dominoNucleusDatasetUiAddDataSetTagInput == null) {
throw new ApiException(400, "Missing the required parameter 'dominoNucleusDatasetUiAddDataSetTagInput' when calling addTag");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/collections/{datasetId}/tags"
.replace("{datasetId}", ApiClient.urlEncode(datasetId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(dominoNucleusDatasetUiAddDataSetTagInput);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* archive a dataset
*
* @param datasetId Id of the collection of datasets (required)
* @return DominoDatasetApiDatasetDto
* @throws ApiException if fails to make API call
*/
public DominoDatasetApiDatasetDto archiveDataset(String datasetId) throws ApiException {
ApiResponse localVarResponse = archiveDatasetWithHttpInfo(datasetId);
return localVarResponse.getData();
}
/**
* archive a dataset
*
* @param datasetId Id of the collection of datasets (required)
* @return ApiResponse<DominoDatasetApiDatasetDto>
* @throws ApiException if fails to make API call
*/
public ApiResponse archiveDatasetWithHttpInfo(String datasetId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = archiveDatasetRequestBuilder(datasetId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("archiveDataset", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder archiveDatasetRequestBuilder(String datasetId) throws ApiException {
// verify the required parameter 'datasetId' is set
if (datasetId == null) {
throw new ApiException(400, "Missing the required parameter 'datasetId' when calling archiveDataset");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/collections/{datasetId}/archive"
.replace("{datasetId}", ApiClient.urlEncode(datasetId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("DELETE", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Determine if a dataset can be added to a project
*
* @param projectId Project identifier (required)
* @return Boolean
* @throws ApiException if fails to make API call
*/
public Boolean canAddDatasetUi(String projectId) throws ApiException {
ApiResponse localVarResponse = canAddDatasetUiWithHttpInfo(projectId);
return localVarResponse.getData();
}
/**
* Determine if a dataset can be added to a project
*
* @param projectId Project identifier (required)
* @return ApiResponse<Boolean>
* @throws ApiException if fails to make API call
*/
public ApiResponse canAddDatasetUiWithHttpInfo(String projectId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = canAddDatasetUiRequestBuilder(projectId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("canAddDatasetUi", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder canAddDatasetUiRequestBuilder(String projectId) throws ApiException {
// verify the required parameter 'projectId' is set
if (projectId == null) {
throw new ApiException(400, "Missing the required parameter 'projectId' when calling canAddDatasetUi");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/project/{projectId}/canAddDataset"
.replace("{projectId}", ApiClient.urlEncode(projectId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* indicates the cancellation of the chunked file uploads
*
* @param datasetId Id of the collection of datasets (required)
* @param uploadKey Unique identifier for upload session (required)
* @throws ApiException if fails to make API call
*/
public void cancelSnapshotUpload(String datasetId, String uploadKey) throws ApiException {
cancelSnapshotUploadWithHttpInfo(datasetId, uploadKey);
}
/**
* indicates the cancellation of the chunked file uploads
*
* @param datasetId Id of the collection of datasets (required)
* @param uploadKey Unique identifier for upload session (required)
* @return ApiResponse<Void>
* @throws ApiException if fails to make API call
*/
public ApiResponse cancelSnapshotUploadWithHttpInfo(String datasetId, String uploadKey) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = cancelSnapshotUploadRequestBuilder(datasetId, uploadKey);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("cancelSnapshotUpload", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
null
);
} finally {
// Drain the InputStream
while (localVarResponse.body().read() != -1) {
// Ignore
}
localVarResponse.body().close();
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder cancelSnapshotUploadRequestBuilder(String datasetId, String uploadKey) throws ApiException {
// verify the required parameter 'datasetId' is set
if (datasetId == null) {
throw new ApiException(400, "Missing the required parameter 'datasetId' when calling cancelSnapshotUpload");
}
// verify the required parameter 'uploadKey' is set
if (uploadKey == null) {
throw new ApiException(400, "Missing the required parameter 'uploadKey' when calling cancelSnapshotUpload");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/collections/{datasetId}/snapshot/file/cancel/{uploadKey}"
.replace("{datasetId}", ApiClient.urlEncode(datasetId.toString()))
.replace("{uploadKey}", ApiClient.urlEncode(uploadKey.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* create a new dataset collection
*
* @param dominoNucleusDatasetUiCreateDatasetInput JSON object with information for new dataset collection creation (required)
* @return DominoNucleusDatasetUiDatasetViewModel
* @throws ApiException if fails to make API call
*/
public DominoNucleusDatasetUiDatasetViewModel createDatasetCollection(DominoNucleusDatasetUiCreateDatasetInput dominoNucleusDatasetUiCreateDatasetInput) throws ApiException {
ApiResponse localVarResponse = createDatasetCollectionWithHttpInfo(dominoNucleusDatasetUiCreateDatasetInput);
return localVarResponse.getData();
}
/**
* create a new dataset collection
*
* @param dominoNucleusDatasetUiCreateDatasetInput JSON object with information for new dataset collection creation (required)
* @return ApiResponse<DominoNucleusDatasetUiDatasetViewModel>
* @throws ApiException if fails to make API call
*/
public ApiResponse createDatasetCollectionWithHttpInfo(DominoNucleusDatasetUiCreateDatasetInput dominoNucleusDatasetUiCreateDatasetInput) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = createDatasetCollectionRequestBuilder(dominoNucleusDatasetUiCreateDatasetInput);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("createDatasetCollection", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder createDatasetCollectionRequestBuilder(DominoNucleusDatasetUiCreateDatasetInput dominoNucleusDatasetUiCreateDatasetInput) throws ApiException {
// verify the required parameter 'dominoNucleusDatasetUiCreateDatasetInput' is set
if (dominoNucleusDatasetUiCreateDatasetInput == null) {
throw new ApiException(400, "Missing the required parameter 'dominoNucleusDatasetUiCreateDatasetInput' when calling createDatasetCollection");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/collections";
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(dominoNucleusDatasetUiCreateDatasetInput);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Promotes a scratch space into a dataset snapshot
*
* @param datasetId (required)
* @param projectId (required)
* @return DominoDatasetApiDatasetSnapshotDto
* @throws ApiException if fails to make API call
*/
public DominoDatasetApiDatasetSnapshotDto createSnapshotFromScratchSpace(String datasetId, String projectId) throws ApiException {
ApiResponse localVarResponse = createSnapshotFromScratchSpaceWithHttpInfo(datasetId, projectId);
return localVarResponse.getData();
}
/**
* Promotes a scratch space into a dataset snapshot
*
* @param datasetId (required)
* @param projectId (required)
* @return ApiResponse<DominoDatasetApiDatasetSnapshotDto>
* @throws ApiException if fails to make API call
*/
public ApiResponse createSnapshotFromScratchSpaceWithHttpInfo(String datasetId, String projectId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = createSnapshotFromScratchSpaceRequestBuilder(datasetId, projectId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("createSnapshotFromScratchSpace", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder createSnapshotFromScratchSpaceRequestBuilder(String datasetId, String projectId) throws ApiException {
// verify the required parameter 'datasetId' is set
if (datasetId == null) {
throw new ApiException(400, "Missing the required parameter 'datasetId' when calling createSnapshotFromScratchSpace");
}
// verify the required parameter 'projectId' is set
if (projectId == null) {
throw new ApiException(400, "Missing the required parameter 'projectId' when calling createSnapshotFromScratchSpace");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/collections/{datasetId}/project/{projectId}/snapshot"
.replace("{datasetId}", ApiClient.urlEncode(datasetId.toString()))
.replace("{projectId}", ApiClient.urlEncode(projectId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* starts a run to create a new dataset
*
* @param collectionId the collectionId id (required)
* @param dominoNucleusDatasetUiCreateSnapshotWithRunInput JSON object with information for run (required)
* @return DominoNucleusDatasetUiDataSetRun
* @throws ApiException if fails to make API call
*/
public DominoNucleusDatasetUiDataSetRun createSnapshotWithRun(String collectionId, DominoNucleusDatasetUiCreateSnapshotWithRunInput dominoNucleusDatasetUiCreateSnapshotWithRunInput) throws ApiException {
ApiResponse localVarResponse = createSnapshotWithRunWithHttpInfo(collectionId, dominoNucleusDatasetUiCreateSnapshotWithRunInput);
return localVarResponse.getData();
}
/**
* starts a run to create a new dataset
*
* @param collectionId the collectionId id (required)
* @param dominoNucleusDatasetUiCreateSnapshotWithRunInput JSON object with information for run (required)
* @return ApiResponse<DominoNucleusDatasetUiDataSetRun>
* @throws ApiException if fails to make API call
*/
public ApiResponse createSnapshotWithRunWithHttpInfo(String collectionId, DominoNucleusDatasetUiCreateSnapshotWithRunInput dominoNucleusDatasetUiCreateSnapshotWithRunInput) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = createSnapshotWithRunRequestBuilder(collectionId, dominoNucleusDatasetUiCreateSnapshotWithRunInput);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("createSnapshotWithRun", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder createSnapshotWithRunRequestBuilder(String collectionId, DominoNucleusDatasetUiCreateSnapshotWithRunInput dominoNucleusDatasetUiCreateSnapshotWithRunInput) throws ApiException {
// verify the required parameter 'collectionId' is set
if (collectionId == null) {
throw new ApiException(400, "Missing the required parameter 'collectionId' when calling createSnapshotWithRun");
}
// verify the required parameter 'dominoNucleusDatasetUiCreateSnapshotWithRunInput' is set
if (dominoNucleusDatasetUiCreateSnapshotWithRunInput == null) {
throw new ApiException(400, "Missing the required parameter 'dominoNucleusDatasetUiCreateSnapshotWithRunInput' when calling createSnapshotWithRun");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/run/{collectionId}"
.replace("{collectionId}", ApiClient.urlEncode(collectionId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(dominoNucleusDatasetUiCreateSnapshotWithRunInput);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* launches a workspace to create a new dataset
*
* @param collectionId the collectionId id (required)
* @param dominoNucleusDatasetUiCreateSnapshotWithWorkspaceInput JSON object with information for run (required)
* @return DominoNucleusDatasetUiDatasetWorkspace
* @throws ApiException if fails to make API call
*/
public DominoNucleusDatasetUiDatasetWorkspace createSnapshotWithWorkspace(String collectionId, DominoNucleusDatasetUiCreateSnapshotWithWorkspaceInput dominoNucleusDatasetUiCreateSnapshotWithWorkspaceInput) throws ApiException {
ApiResponse localVarResponse = createSnapshotWithWorkspaceWithHttpInfo(collectionId, dominoNucleusDatasetUiCreateSnapshotWithWorkspaceInput);
return localVarResponse.getData();
}
/**
* launches a workspace to create a new dataset
*
* @param collectionId the collectionId id (required)
* @param dominoNucleusDatasetUiCreateSnapshotWithWorkspaceInput JSON object with information for run (required)
* @return ApiResponse<DominoNucleusDatasetUiDatasetWorkspace>
* @throws ApiException if fails to make API call
*/
public ApiResponse createSnapshotWithWorkspaceWithHttpInfo(String collectionId, DominoNucleusDatasetUiCreateSnapshotWithWorkspaceInput dominoNucleusDatasetUiCreateSnapshotWithWorkspaceInput) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = createSnapshotWithWorkspaceRequestBuilder(collectionId, dominoNucleusDatasetUiCreateSnapshotWithWorkspaceInput);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("createSnapshotWithWorkspace", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder createSnapshotWithWorkspaceRequestBuilder(String collectionId, DominoNucleusDatasetUiCreateSnapshotWithWorkspaceInput dominoNucleusDatasetUiCreateSnapshotWithWorkspaceInput) throws ApiException {
// verify the required parameter 'collectionId' is set
if (collectionId == null) {
throw new ApiException(400, "Missing the required parameter 'collectionId' when calling createSnapshotWithWorkspace");
}
// verify the required parameter 'dominoNucleusDatasetUiCreateSnapshotWithWorkspaceInput' is set
if (dominoNucleusDatasetUiCreateSnapshotWithWorkspaceInput == null) {
throw new ApiException(400, "Missing the required parameter 'dominoNucleusDatasetUiCreateSnapshotWithWorkspaceInput' when calling createSnapshotWithWorkspace");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/workspace/{collectionId}"
.replace("{collectionId}", ApiClient.urlEncode(collectionId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(dominoNucleusDatasetUiCreateSnapshotWithWorkspaceInput);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* deletes all marked for deletion snapshots for a dataset
*
* @return List<DominoDatasetApiDatasetSnapshotDto>
* @throws ApiException if fails to make API call
*/
public List deleteMarkedSnapshots() throws ApiException {
ApiResponse> localVarResponse = deleteMarkedSnapshotsWithHttpInfo();
return localVarResponse.getData();
}
/**
* deletes all marked for deletion snapshots for a dataset
*
* @return ApiResponse<List<DominoDatasetApiDatasetSnapshotDto>>
* @throws ApiException if fails to make API call
*/
public ApiResponse> deleteMarkedSnapshotsWithHttpInfo() throws ApiException {
HttpRequest.Builder localVarRequestBuilder = deleteMarkedSnapshotsRequestBuilder();
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("deleteMarkedSnapshots", localVarResponse);
}
return new ApiResponse>(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference>() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder deleteMarkedSnapshotsRequestBuilder() throws ApiException {
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi";
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("DELETE", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* delete project mount
*
* @param projectId the project id (required)
* @param mountPath the location at which the data set mounts (required)
* @return List<DominoNucleusDatasetUiDatasetProjectMountSummaryViewModel>
* @throws ApiException if fails to make API call
*/
public List deleteProjectMount(String projectId, String mountPath) throws ApiException {
ApiResponse> localVarResponse = deleteProjectMountWithHttpInfo(projectId, mountPath);
return localVarResponse.getData();
}
/**
* delete project mount
*
* @param projectId the project id (required)
* @param mountPath the location at which the data set mounts (required)
* @return ApiResponse<List<DominoNucleusDatasetUiDatasetProjectMountSummaryViewModel>>
* @throws ApiException if fails to make API call
*/
public ApiResponse> deleteProjectMountWithHttpInfo(String projectId, String mountPath) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = deleteProjectMountRequestBuilder(projectId, mountPath);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("deleteProjectMount", localVarResponse);
}
return new ApiResponse>(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference>() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder deleteProjectMountRequestBuilder(String projectId, String mountPath) throws ApiException {
// verify the required parameter 'projectId' is set
if (projectId == null) {
throw new ApiException(400, "Missing the required parameter 'projectId' when calling deleteProjectMount");
}
// verify the required parameter 'mountPath' is set
if (mountPath == null) {
throw new ApiException(400, "Missing the required parameter 'mountPath' when calling deleteProjectMount");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/mounts/{projectId}/imported/{mountPath}"
.replace("{projectId}", ApiClient.urlEncode(projectId.toString()))
.replace("{mountPath}", ApiClient.urlEncode(mountPath.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("DELETE", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Deletes a scratch space
*
* @param scratchSpaceId Id of the scratch space (required)
* @return DominoDatasetApiDatasetScratchSpaceDto
* @throws ApiException if fails to make API call
*/
public DominoDatasetApiDatasetScratchSpaceDto deleteScratchSpace(String scratchSpaceId) throws ApiException {
ApiResponse localVarResponse = deleteScratchSpaceWithHttpInfo(scratchSpaceId);
return localVarResponse.getData();
}
/**
* Deletes a scratch space
*
* @param scratchSpaceId Id of the scratch space (required)
* @return ApiResponse<DominoDatasetApiDatasetScratchSpaceDto>
* @throws ApiException if fails to make API call
*/
public ApiResponse deleteScratchSpaceWithHttpInfo(String scratchSpaceId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = deleteScratchSpaceRequestBuilder(scratchSpaceId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("deleteScratchSpace", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder deleteScratchSpaceRequestBuilder(String scratchSpaceId) throws ApiException {
// verify the required parameter 'scratchSpaceId' is set
if (scratchSpaceId == null) {
throw new ApiException(400, "Missing the required parameter 'scratchSpaceId' when calling deleteScratchSpace");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/scratchSpace/{scratchSpaceId}"
.replace("{scratchSpaceId}", ApiClient.urlEncode(scratchSpaceId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("DELETE", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* deletes the specified data set snapshot
*
* @param dataSetId Id of the dataset snapshot (required)
* @return DominoDatasetApiDatasetSnapshotDto
* @throws ApiException if fails to make API call
*/
public DominoDatasetApiDatasetSnapshotDto deleteSnapshot(String dataSetId) throws ApiException {
ApiResponse localVarResponse = deleteSnapshotWithHttpInfo(dataSetId);
return localVarResponse.getData();
}
/**
* deletes the specified data set snapshot
*
* @param dataSetId Id of the dataset snapshot (required)
* @return ApiResponse<DominoDatasetApiDatasetSnapshotDto>
* @throws ApiException if fails to make API call
*/
public ApiResponse deleteSnapshotWithHttpInfo(String dataSetId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = deleteSnapshotRequestBuilder(dataSetId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("deleteSnapshot", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder deleteSnapshotRequestBuilder(String dataSetId) throws ApiException {
// verify the required parameter 'dataSetId' is set
if (dataSetId == null) {
throw new ApiException(400, "Missing the required parameter 'dataSetId' when calling deleteSnapshot");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/{dataSetId}"
.replace("{dataSetId}", ApiClient.urlEncode(dataSetId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("DELETE", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Indicates the successful completion of the files upload in the UI
*
* @param datasetId (required)
* @param uploadKey (required)
* @return DominoDatasetApiDatasetSnapshotDto
* @throws ApiException if fails to make API call
*/
public DominoDatasetApiDatasetSnapshotDto endSnapshotUpload(String datasetId, String uploadKey) throws ApiException {
ApiResponse localVarResponse = endSnapshotUploadWithHttpInfo(datasetId, uploadKey);
return localVarResponse.getData();
}
/**
* Indicates the successful completion of the files upload in the UI
*
* @param datasetId (required)
* @param uploadKey (required)
* @return ApiResponse<DominoDatasetApiDatasetSnapshotDto>
* @throws ApiException if fails to make API call
*/
public ApiResponse endSnapshotUploadWithHttpInfo(String datasetId, String uploadKey) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = endSnapshotUploadRequestBuilder(datasetId, uploadKey);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("endSnapshotUpload", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder endSnapshotUploadRequestBuilder(String datasetId, String uploadKey) throws ApiException {
// verify the required parameter 'datasetId' is set
if (datasetId == null) {
throw new ApiException(400, "Missing the required parameter 'datasetId' when calling endSnapshotUpload");
}
// verify the required parameter 'uploadKey' is set
if (uploadKey == null) {
throw new ApiException(400, "Missing the required parameter 'uploadKey' when calling endSnapshotUpload");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/collections/{datasetId}/snapshot/file/end/{uploadKey}"
.replace("{datasetId}", ApiClient.urlEncode(datasetId.toString()))
.replace("{uploadKey}", ApiClient.urlEncode(uploadKey.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* retrieve the most recent consumption information for a snapshot
*
* @param snapshotId Id of the snapshot to get consumption data for (required)
* @return DominoDatasetApiConsumedSnapshotDto
* @throws ApiException if fails to make API call
*/
public DominoDatasetApiConsumedSnapshotDto getMostRecentConsumedSnapshotResource(String snapshotId) throws ApiException {
ApiResponse localVarResponse = getMostRecentConsumedSnapshotResourceWithHttpInfo(snapshotId);
return localVarResponse.getData();
}
/**
* retrieve the most recent consumption information for a snapshot
*
* @param snapshotId Id of the snapshot to get consumption data for (required)
* @return ApiResponse<DominoDatasetApiConsumedSnapshotDto>
* @throws ApiException if fails to make API call
*/
public ApiResponse getMostRecentConsumedSnapshotResourceWithHttpInfo(String snapshotId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getMostRecentConsumedSnapshotResourceRequestBuilder(snapshotId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getMostRecentConsumedSnapshotResource", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getMostRecentConsumedSnapshotResourceRequestBuilder(String snapshotId) throws ApiException {
// verify the required parameter 'snapshotId' is set
if (snapshotId == null) {
throw new ApiException(400, "Missing the required parameter 'snapshotId' when calling getMostRecentConsumedSnapshotResource");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/consumption/snapshot/{snapshotId}"
.replace("{snapshotId}", ApiClient.urlEncode(snapshotId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* gets mountable dataset collections
*
* @param ignoreProjects Ids of projects that should have their datasets excluded from being returned (optional
* @return List<DominoNucleusDatasetUiDatasetViewModel>
* @throws ApiException if fails to make API call
*/
public List getMountableDatasets(List ignoreProjects) throws ApiException {
ApiResponse> localVarResponse = getMountableDatasetsWithHttpInfo(ignoreProjects);
return localVarResponse.getData();
}
/**
* gets mountable dataset collections
*
* @param ignoreProjects Ids of projects that should have their datasets excluded from being returned (optional
* @return ApiResponse<List<DominoNucleusDatasetUiDatasetViewModel>>
* @throws ApiException if fails to make API call
*/
public ApiResponse> getMountableDatasetsWithHttpInfo(List ignoreProjects) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getMountableDatasetsRequestBuilder(ignoreProjects);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getMountableDatasets", localVarResponse);
}
return new ApiResponse>(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference>() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getMountableDatasetsRequestBuilder(List ignoreProjects) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/collections/mountable";
List localVarQueryParams = new ArrayList<>();
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
localVarQueryParameterBaseName = "ignoreProjects";
localVarQueryParams.addAll(ApiClient.parameterToPairs("multi", "ignoreProjects", ignoreProjects));
if (!localVarQueryParams.isEmpty() || localVarQueryStringJoiner.length() != 0) {
StringJoiner queryJoiner = new StringJoiner("&");
localVarQueryParams.forEach(p -> queryJoiner.add(p.getName() + '=' + p.getValue()));
if (localVarQueryStringJoiner.length() != 0) {
queryJoiner.add(localVarQueryStringJoiner.toString());
}
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath + '?' + queryJoiner.toString()));
} else {
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
}
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* gets imported project mounts
*
* @param projectId the project id (required)
* @return List<DominoNucleusDatasetUiDatasetProjectMountSummaryViewModel>
* @throws ApiException if fails to make API call
*/
public List getProjectImportedMountSummary(String projectId) throws ApiException {
ApiResponse> localVarResponse = getProjectImportedMountSummaryWithHttpInfo(projectId);
return localVarResponse.getData();
}
/**
* gets imported project mounts
*
* @param projectId the project id (required)
* @return ApiResponse<List<DominoNucleusDatasetUiDatasetProjectMountSummaryViewModel>>
* @throws ApiException if fails to make API call
*/
public ApiResponse> getProjectImportedMountSummaryWithHttpInfo(String projectId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getProjectImportedMountSummaryRequestBuilder(projectId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getProjectImportedMountSummary", localVarResponse);
}
return new ApiResponse>(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference>() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getProjectImportedMountSummaryRequestBuilder(String projectId) throws ApiException {
// verify the required parameter 'projectId' is set
if (projectId == null) {
throw new ApiException(400, "Missing the required parameter 'projectId' when calling getProjectImportedMountSummary");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/mounts/{projectId}/imported"
.replace("{projectId}", ApiClient.urlEncode(projectId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* gets local project mounts
*
* @param projectId the project id (required)
* @return List<DominoNucleusDatasetUiDatasetProjectMountSummaryViewModel>
* @throws ApiException if fails to make API call
*/
public List getProjectLocalMounts(String projectId) throws ApiException {
ApiResponse> localVarResponse = getProjectLocalMountsWithHttpInfo(projectId);
return localVarResponse.getData();
}
/**
* gets local project mounts
*
* @param projectId the project id (required)
* @return ApiResponse<List<DominoNucleusDatasetUiDatasetProjectMountSummaryViewModel>>
* @throws ApiException if fails to make API call
*/
public ApiResponse> getProjectLocalMountsWithHttpInfo(String projectId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getProjectLocalMountsRequestBuilder(projectId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getProjectLocalMounts", localVarResponse);
}
return new ApiResponse>(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference>() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getProjectLocalMountsRequestBuilder(String projectId) throws ApiException {
// verify the required parameter 'projectId' is set
if (projectId == null) {
throw new ApiException(400, "Missing the required parameter 'projectId' when calling getProjectLocalMounts");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/mounts/{projectId}/local"
.replace("{projectId}", ApiClient.urlEncode(projectId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Gets files in a subdirectory of a project's scratch space
*
* @param projectId (required)
* @param path Directory path (required)
* @return List<DominoNucleusDatasetUiDataSetFileBrowserRow>
* @throws ApiException if fails to make API call
*/
public List getScratchSpaceFiles(String projectId, String path) throws ApiException {
ApiResponse> localVarResponse = getScratchSpaceFilesWithHttpInfo(projectId, path);
return localVarResponse.getData();
}
/**
* Gets files in a subdirectory of a project's scratch space
*
* @param projectId (required)
* @param path Directory path (required)
* @return ApiResponse<List<DominoNucleusDatasetUiDataSetFileBrowserRow>>
* @throws ApiException if fails to make API call
*/
public ApiResponse> getScratchSpaceFilesWithHttpInfo(String projectId, String path) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getScratchSpaceFilesRequestBuilder(projectId, path);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getScratchSpaceFiles", localVarResponse);
}
return new ApiResponse>(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference>() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getScratchSpaceFilesRequestBuilder(String projectId, String path) throws ApiException {
// verify the required parameter 'projectId' is set
if (projectId == null) {
throw new ApiException(400, "Missing the required parameter 'projectId' when calling getScratchSpaceFiles");
}
// verify the required parameter 'path' is set
if (path == null) {
throw new ApiException(400, "Missing the required parameter 'path' when calling getScratchSpaceFiles");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/scratchspace/{projectId}/files/{path}"
.replace("{projectId}", ApiClient.urlEncode(projectId.toString()))
.replace("{path}", ApiClient.urlEncode(path.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Gets files in a subdirectory of a project's scratch space
*
* @param projectId (required)
* @return List<DominoNucleusDatasetUiDataSetFileBrowserRow>
* @throws ApiException if fails to make API call
*/
public List getScratchSpaceFilesAtRoot(String projectId) throws ApiException {
ApiResponse> localVarResponse = getScratchSpaceFilesAtRootWithHttpInfo(projectId);
return localVarResponse.getData();
}
/**
* Gets files in a subdirectory of a project's scratch space
*
* @param projectId (required)
* @return ApiResponse<List<DominoNucleusDatasetUiDataSetFileBrowserRow>>
* @throws ApiException if fails to make API call
*/
public ApiResponse> getScratchSpaceFilesAtRootWithHttpInfo(String projectId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getScratchSpaceFilesAtRootRequestBuilder(projectId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getScratchSpaceFilesAtRoot", localVarResponse);
}
return new ApiResponse>(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference>() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getScratchSpaceFilesAtRootRequestBuilder(String projectId) throws ApiException {
// verify the required parameter 'projectId' is set
if (projectId == null) {
throw new ApiException(400, "Missing the required parameter 'projectId' when calling getScratchSpaceFilesAtRoot");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/scratchspace/{projectId}/files"
.replace("{projectId}", ApiClient.urlEncode(projectId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Promotes a scratch space into a dataset snapshot
*
* @param projectId (required)
* @return DominoDatasetApiDatasetScratchSpaceDto
* @throws ApiException if fails to make API call
*/
public DominoDatasetApiDatasetScratchSpaceDto getScratchSpaceOrDefault(String projectId) throws ApiException {
ApiResponse localVarResponse = getScratchSpaceOrDefaultWithHttpInfo(projectId);
return localVarResponse.getData();
}
/**
* Promotes a scratch space into a dataset snapshot
*
* @param projectId (required)
* @return ApiResponse<DominoDatasetApiDatasetScratchSpaceDto>
* @throws ApiException if fails to make API call
*/
public ApiResponse getScratchSpaceOrDefaultWithHttpInfo(String projectId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getScratchSpaceOrDefaultRequestBuilder(projectId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getScratchSpaceOrDefault", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getScratchSpaceOrDefaultRequestBuilder(String projectId) throws ApiException {
// verify the required parameter 'projectId' is set
if (projectId == null) {
throw new ApiException(400, "Missing the required parameter 'projectId' when calling getScratchSpaceOrDefault");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/project/{projectId}/scratchSpace"
.replace("{projectId}", ApiClient.urlEncode(projectId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* retrieves the files in a specified data set at the specified directory
*
* @param snapshotId snapshot id (required)
* @param path Directory path (required)
* @return DominoNucleusDatasetUiDataSetFileBrowserViewModel
* @throws ApiException if fails to make API call
*/
public DominoNucleusDatasetUiDataSetFileBrowserViewModel getSnapshotFiles(String snapshotId, String path) throws ApiException {
ApiResponse localVarResponse = getSnapshotFilesWithHttpInfo(snapshotId, path);
return localVarResponse.getData();
}
/**
* retrieves the files in a specified data set at the specified directory
*
* @param snapshotId snapshot id (required)
* @param path Directory path (required)
* @return ApiResponse<DominoNucleusDatasetUiDataSetFileBrowserViewModel>
* @throws ApiException if fails to make API call
*/
public ApiResponse getSnapshotFilesWithHttpInfo(String snapshotId, String path) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getSnapshotFilesRequestBuilder(snapshotId, path);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getSnapshotFiles", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getSnapshotFilesRequestBuilder(String snapshotId, String path) throws ApiException {
// verify the required parameter 'snapshotId' is set
if (snapshotId == null) {
throw new ApiException(400, "Missing the required parameter 'snapshotId' when calling getSnapshotFiles");
}
// verify the required parameter 'path' is set
if (path == null) {
throw new ApiException(400, "Missing the required parameter 'path' when calling getSnapshotFiles");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/{snapshotId}/files/{path}"
.replace("{snapshotId}", ApiClient.urlEncode(snapshotId.toString()))
.replace("{path}", ApiClient.urlEncode(path.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* retrieves the files in a specified data set at the root directory
*
* @param dataSetId Id of the dataset (required)
* @return DominoNucleusDatasetUiDataSetFileBrowserViewModel
* @throws ApiException if fails to make API call
*/
public DominoNucleusDatasetUiDataSetFileBrowserViewModel getSnapshotFilesAtRoot(String dataSetId) throws ApiException {
ApiResponse localVarResponse = getSnapshotFilesAtRootWithHttpInfo(dataSetId);
return localVarResponse.getData();
}
/**
* retrieves the files in a specified data set at the root directory
*
* @param dataSetId Id of the dataset (required)
* @return ApiResponse<DominoNucleusDatasetUiDataSetFileBrowserViewModel>
* @throws ApiException if fails to make API call
*/
public ApiResponse getSnapshotFilesAtRootWithHttpInfo(String dataSetId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getSnapshotFilesAtRootRequestBuilder(dataSetId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getSnapshotFilesAtRoot", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getSnapshotFilesAtRootRequestBuilder(String dataSetId) throws ApiException {
// verify the required parameter 'dataSetId' is set
if (dataSetId == null) {
throw new ApiException(400, "Missing the required parameter 'dataSetId' when calling getSnapshotFilesAtRoot");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/{dataSetId}/files"
.replace("{dataSetId}", ApiClient.urlEncode(dataSetId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* retrieves data set summaries of all the data set verisions for a specified data set collection
*
* @param datasetId Id of the collection of datasets (required)
* @return List<DominoDatasetApiDatasetSnapshotSummaryDto>
* @throws ApiException if fails to make API call
*/
public List getSnapshotSummaries(String datasetId) throws ApiException {
ApiResponse> localVarResponse = getSnapshotSummariesWithHttpInfo(datasetId);
return localVarResponse.getData();
}
/**
* retrieves data set summaries of all the data set verisions for a specified data set collection
*
* @param datasetId Id of the collection of datasets (required)
* @return ApiResponse<List<DominoDatasetApiDatasetSnapshotSummaryDto>>
* @throws ApiException if fails to make API call
*/
public ApiResponse> getSnapshotSummariesWithHttpInfo(String datasetId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getSnapshotSummariesRequestBuilder(datasetId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getSnapshotSummaries", localVarResponse);
}
return new ApiResponse>(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference>() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getSnapshotSummariesRequestBuilder(String datasetId) throws ApiException {
// verify the required parameter 'datasetId' is set
if (datasetId == null) {
throw new ApiException(400, "Missing the required parameter 'datasetId' when calling getSnapshotSummaries");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/collections/{datasetId}/versionSummaries"
.replace("{datasetId}", ApiClient.urlEncode(datasetId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* retrieves the specified snapshot
*
* @param snapshotId Id of the snapshot (required)
* @return DominoDatasetApiDatasetSnapshotSummaryDto
* @throws ApiException if fails to make API call
*/
public DominoDatasetApiDatasetSnapshotSummaryDto getSnapshotUi(String snapshotId) throws ApiException {
ApiResponse localVarResponse = getSnapshotUiWithHttpInfo(snapshotId);
return localVarResponse.getData();
}
/**
* retrieves the specified snapshot
*
* @param snapshotId Id of the snapshot (required)
* @return ApiResponse<DominoDatasetApiDatasetSnapshotSummaryDto>
* @throws ApiException if fails to make API call
*/
public ApiResponse getSnapshotUiWithHttpInfo(String snapshotId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getSnapshotUiRequestBuilder(snapshotId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getSnapshotUi", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getSnapshotUiRequestBuilder(String snapshotId) throws ApiException {
// verify the required parameter 'snapshotId' is set
if (snapshotId == null) {
throw new ApiException(400, "Missing the required parameter 'snapshotId' when calling getSnapshotUi");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/{snapshotId}"
.replace("{snapshotId}", ApiClient.urlEncode(snapshotId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Tests whether or not an upload session exists
*
* @param datasetId (required)
* @param uploadKey (required)
* @param resumableChunkNumber (optional)
* @param resumableTotalChunks (optional)
* @param resumableIdentifier (optional)
* @param resumableRelativePath (optional)
* @throws ApiException if fails to make API call
*/
public void getSnapshotUploadSession(String datasetId, String uploadKey, Integer resumableChunkNumber, Integer resumableTotalChunks, String resumableIdentifier, String resumableRelativePath) throws ApiException {
getSnapshotUploadSessionWithHttpInfo(datasetId, uploadKey, resumableChunkNumber, resumableTotalChunks, resumableIdentifier, resumableRelativePath);
}
/**
* Tests whether or not an upload session exists
*
* @param datasetId (required)
* @param uploadKey (required)
* @param resumableChunkNumber (optional)
* @param resumableTotalChunks (optional)
* @param resumableIdentifier (optional)
* @param resumableRelativePath (optional)
* @return ApiResponse<Void>
* @throws ApiException if fails to make API call
*/
public ApiResponse getSnapshotUploadSessionWithHttpInfo(String datasetId, String uploadKey, Integer resumableChunkNumber, Integer resumableTotalChunks, String resumableIdentifier, String resumableRelativePath) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getSnapshotUploadSessionRequestBuilder(datasetId, uploadKey, resumableChunkNumber, resumableTotalChunks, resumableIdentifier, resumableRelativePath);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getSnapshotUploadSession", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
null
);
} finally {
// Drain the InputStream
while (localVarResponse.body().read() != -1) {
// Ignore
}
localVarResponse.body().close();
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getSnapshotUploadSessionRequestBuilder(String datasetId, String uploadKey, Integer resumableChunkNumber, Integer resumableTotalChunks, String resumableIdentifier, String resumableRelativePath) throws ApiException {
// verify the required parameter 'datasetId' is set
if (datasetId == null) {
throw new ApiException(400, "Missing the required parameter 'datasetId' when calling getSnapshotUploadSession");
}
// verify the required parameter 'uploadKey' is set
if (uploadKey == null) {
throw new ApiException(400, "Missing the required parameter 'uploadKey' when calling getSnapshotUploadSession");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/collections/{datasetId}/snapshot/file/session/{uploadKey}"
.replace("{datasetId}", ApiClient.urlEncode(datasetId.toString()))
.replace("{uploadKey}", ApiClient.urlEncode(uploadKey.toString()));
List localVarQueryParams = new ArrayList<>();
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
localVarQueryParameterBaseName = "resumableChunkNumber";
localVarQueryParams.addAll(ApiClient.parameterToPairs("resumableChunkNumber", resumableChunkNumber));
localVarQueryParameterBaseName = "resumableTotalChunks";
localVarQueryParams.addAll(ApiClient.parameterToPairs("resumableTotalChunks", resumableTotalChunks));
localVarQueryParameterBaseName = "resumableIdentifier";
localVarQueryParams.addAll(ApiClient.parameterToPairs("resumableIdentifier", resumableIdentifier));
localVarQueryParameterBaseName = "resumableRelativePath";
localVarQueryParams.addAll(ApiClient.parameterToPairs("resumableRelativePath", resumableRelativePath));
if (!localVarQueryParams.isEmpty() || localVarQueryStringJoiner.length() != 0) {
StringJoiner queryJoiner = new StringJoiner("&");
localVarQueryParams.forEach(p -> queryJoiner.add(p.getName() + '=' + p.getValue()));
if (localVarQueryStringJoiner.length() != 0) {
queryJoiner.add(localVarQueryStringJoiner.toString());
}
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath + '?' + queryJoiner.toString()));
} else {
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
}
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* retrieves all snapshots for a dataset
*
* @param datasetId Id of the collection of datasets (required)
* @return List<DominoDatasetApiDatasetSnapshotDto>
* @throws ApiException if fails to make API call
*/
public List getSnapshotsUi(String datasetId) throws ApiException {
ApiResponse> localVarResponse = getSnapshotsUiWithHttpInfo(datasetId);
return localVarResponse.getData();
}
/**
* retrieves all snapshots for a dataset
*
* @param datasetId Id of the collection of datasets (required)
* @return ApiResponse<List<DominoDatasetApiDatasetSnapshotDto>>
* @throws ApiException if fails to make API call
*/
public ApiResponse> getSnapshotsUiWithHttpInfo(String datasetId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getSnapshotsUiRequestBuilder(datasetId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getSnapshotsUi", localVarResponse);
}
return new ApiResponse>(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference>() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getSnapshotsUiRequestBuilder(String datasetId) throws ApiException {
// verify the required parameter 'datasetId' is set
if (datasetId == null) {
throw new ApiException(400, "Missing the required parameter 'datasetId' when calling getSnapshotsUi");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/collections/{datasetId}/snapshots"
.replace("{datasetId}", ApiClient.urlEncode(datasetId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* lookup dataset by project and name
*
* @param projectOwner project owner (required)
* @param projectName project name (required)
* @param collectionName dataset collection name (required)
* @return DominoDatasetApiDatasetDto
* @throws ApiException if fails to make API call
*/
public DominoDatasetApiDatasetDto lookupDatasetByName(String projectOwner, String projectName, String collectionName) throws ApiException {
ApiResponse localVarResponse = lookupDatasetByNameWithHttpInfo(projectOwner, projectName, collectionName);
return localVarResponse.getData();
}
/**
* lookup dataset by project and name
*
* @param projectOwner project owner (required)
* @param projectName project name (required)
* @param collectionName dataset collection name (required)
* @return ApiResponse<DominoDatasetApiDatasetDto>
* @throws ApiException if fails to make API call
*/
public ApiResponse lookupDatasetByNameWithHttpInfo(String projectOwner, String projectName, String collectionName) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = lookupDatasetByNameRequestBuilder(projectOwner, projectName, collectionName);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("lookupDatasetByName", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder lookupDatasetByNameRequestBuilder(String projectOwner, String projectName, String collectionName) throws ApiException {
// verify the required parameter 'projectOwner' is set
if (projectOwner == null) {
throw new ApiException(400, "Missing the required parameter 'projectOwner' when calling lookupDatasetByName");
}
// verify the required parameter 'projectName' is set
if (projectName == null) {
throw new ApiException(400, "Missing the required parameter 'projectName' when calling lookupDatasetByName");
}
// verify the required parameter 'collectionName' is set
if (collectionName == null) {
throw new ApiException(400, "Missing the required parameter 'collectionName' when calling lookupDatasetByName");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/collections/byProject";
List localVarQueryParams = new ArrayList<>();
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
localVarQueryParameterBaseName = "projectOwner";
localVarQueryParams.addAll(ApiClient.parameterToPairs("projectOwner", projectOwner));
localVarQueryParameterBaseName = "projectName";
localVarQueryParams.addAll(ApiClient.parameterToPairs("projectName", projectName));
localVarQueryParameterBaseName = "collectionName";
localVarQueryParams.addAll(ApiClient.parameterToPairs("collectionName", collectionName));
if (!localVarQueryParams.isEmpty() || localVarQueryStringJoiner.length() != 0) {
StringJoiner queryJoiner = new StringJoiner("&");
localVarQueryParams.forEach(p -> queryJoiner.add(p.getName() + '=' + p.getValue()));
if (localVarQueryStringJoiner.length() != 0) {
queryJoiner.add(localVarQueryStringJoiner.toString());
}
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath + '?' + queryJoiner.toString()));
} else {
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
}
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* removes a tag from a dataset by updating the dataset's collection tags map
*
* @param datasetId Id of the collection of datasets (required)
* @param tagName Name of the tag to be removed (required)
* @return DominoNucleusDatasetUiDatasetViewModel
* @throws ApiException if fails to make API call
*/
public DominoNucleusDatasetUiDatasetViewModel removeTag(String datasetId, String tagName) throws ApiException {
ApiResponse localVarResponse = removeTagWithHttpInfo(datasetId, tagName);
return localVarResponse.getData();
}
/**
* removes a tag from a dataset by updating the dataset's collection tags map
*
* @param datasetId Id of the collection of datasets (required)
* @param tagName Name of the tag to be removed (required)
* @return ApiResponse<DominoNucleusDatasetUiDatasetViewModel>
* @throws ApiException if fails to make API call
*/
public ApiResponse removeTagWithHttpInfo(String datasetId, String tagName) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = removeTagRequestBuilder(datasetId, tagName);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("removeTag", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder removeTagRequestBuilder(String datasetId, String tagName) throws ApiException {
// verify the required parameter 'datasetId' is set
if (datasetId == null) {
throw new ApiException(400, "Missing the required parameter 'datasetId' when calling removeTag");
}
// verify the required parameter 'tagName' is set
if (tagName == null) {
throw new ApiException(400, "Missing the required parameter 'tagName' when calling removeTag");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/collections/{datasetId}/tags/{tagName}"
.replace("{datasetId}", ApiClient.urlEncode(datasetId.toString()))
.replace("{tagName}", ApiClient.urlEncode(tagName.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("DELETE", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* add or update a dataset limit override for a project
*
* @param projectId project to override dataset limits for (required)
* @param dominoNucleusDatasetUiSetLimitOverrideInput JSON object with information describing the override (required)
* @return List<DominoDatasetApiDatasetSnapshotDto>
* @throws ApiException if fails to make API call
*/
public List setLimitOverride(String projectId, DominoNucleusDatasetUiSetLimitOverrideInput dominoNucleusDatasetUiSetLimitOverrideInput) throws ApiException {
ApiResponse> localVarResponse = setLimitOverrideWithHttpInfo(projectId, dominoNucleusDatasetUiSetLimitOverrideInput);
return localVarResponse.getData();
}
/**
* add or update a dataset limit override for a project
*
* @param projectId project to override dataset limits for (required)
* @param dominoNucleusDatasetUiSetLimitOverrideInput JSON object with information describing the override (required)
* @return ApiResponse<List<DominoDatasetApiDatasetSnapshotDto>>
* @throws ApiException if fails to make API call
*/
public ApiResponse> setLimitOverrideWithHttpInfo(String projectId, DominoNucleusDatasetUiSetLimitOverrideInput dominoNucleusDatasetUiSetLimitOverrideInput) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = setLimitOverrideRequestBuilder(projectId, dominoNucleusDatasetUiSetLimitOverrideInput);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("setLimitOverride", localVarResponse);
}
return new ApiResponse>(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference>() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder setLimitOverrideRequestBuilder(String projectId, DominoNucleusDatasetUiSetLimitOverrideInput dominoNucleusDatasetUiSetLimitOverrideInput) throws ApiException {
// verify the required parameter 'projectId' is set
if (projectId == null) {
throw new ApiException(400, "Missing the required parameter 'projectId' when calling setLimitOverride");
}
// verify the required parameter 'dominoNucleusDatasetUiSetLimitOverrideInput' is set
if (dominoNucleusDatasetUiSetLimitOverrideInput == null) {
throw new ApiException(400, "Missing the required parameter 'dominoNucleusDatasetUiSetLimitOverrideInput' when calling setLimitOverride");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/limits/{projectId}"
.replace("{projectId}", ApiClient.urlEncode(projectId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(dominoNucleusDatasetUiSetLimitOverrideInput);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* initializes chunked file uploads for a dataset collection snapshot
*
* @param datasetId Id of the collection of datasets (required)
* @return String
* @throws ApiException if fails to make API call
*/
public String startSnapshotUpload(String datasetId) throws ApiException {
ApiResponse localVarResponse = startSnapshotUploadWithHttpInfo(datasetId);
return localVarResponse.getData();
}
/**
* initializes chunked file uploads for a dataset collection snapshot
*
* @param datasetId Id of the collection of datasets (required)
* @return ApiResponse<String>
* @throws ApiException if fails to make API call
*/
public ApiResponse startSnapshotUploadWithHttpInfo(String datasetId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = startSnapshotUploadRequestBuilder(datasetId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("startSnapshotUpload", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder startSnapshotUploadRequestBuilder(String datasetId) throws ApiException {
// verify the required parameter 'datasetId' is set
if (datasetId == null) {
throw new ApiException(400, "Missing the required parameter 'datasetId' when calling startSnapshotUpload");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/collections/{datasetId}/snapshot/file/start"
.replace("{datasetId}", ApiClient.urlEncode(datasetId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* updates a dataset
*
* @param datasetId Id of the collection of datasets (required)
* @param dominoNucleusDatasetUiUpdateDatasetInput JSON object with information for new dataset tag (required)
* @return DominoDatasetApiDatasetDto
* @throws ApiException if fails to make API call
*/
public DominoDatasetApiDatasetDto updateDatasetUi(String datasetId, DominoNucleusDatasetUiUpdateDatasetInput dominoNucleusDatasetUiUpdateDatasetInput) throws ApiException {
ApiResponse localVarResponse = updateDatasetUiWithHttpInfo(datasetId, dominoNucleusDatasetUiUpdateDatasetInput);
return localVarResponse.getData();
}
/**
* updates a dataset
*
* @param datasetId Id of the collection of datasets (required)
* @param dominoNucleusDatasetUiUpdateDatasetInput JSON object with information for new dataset tag (required)
* @return ApiResponse<DominoDatasetApiDatasetDto>
* @throws ApiException if fails to make API call
*/
public ApiResponse updateDatasetUiWithHttpInfo(String datasetId, DominoNucleusDatasetUiUpdateDatasetInput dominoNucleusDatasetUiUpdateDatasetInput) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = updateDatasetUiRequestBuilder(datasetId, dominoNucleusDatasetUiUpdateDatasetInput);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("updateDatasetUi", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder updateDatasetUiRequestBuilder(String datasetId, DominoNucleusDatasetUiUpdateDatasetInput dominoNucleusDatasetUiUpdateDatasetInput) throws ApiException {
// verify the required parameter 'datasetId' is set
if (datasetId == null) {
throw new ApiException(400, "Missing the required parameter 'datasetId' when calling updateDatasetUi");
}
// verify the required parameter 'dominoNucleusDatasetUiUpdateDatasetInput' is set
if (dominoNucleusDatasetUiUpdateDatasetInput == null) {
throw new ApiException(400, "Missing the required parameter 'dominoNucleusDatasetUiUpdateDatasetInput' when calling updateDatasetUi");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/collections/{datasetId}"
.replace("{datasetId}", ApiClient.urlEncode(datasetId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(dominoNucleusDatasetUiUpdateDatasetInput);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* update a dataset snapshot's status
*
* @param snapshotId Id of the dataset snapshot to be updated (required)
* @param dominoNucleusDatasetUiUpdateSnapshotStatusInput JSON object with information describing the new status (required)
* @return DominoDatasetApiDatasetSnapshotDto
* @throws ApiException if fails to make API call
*/
public DominoDatasetApiDatasetSnapshotDto updateSnapshotStatus(String snapshotId, DominoNucleusDatasetUiUpdateSnapshotStatusInput dominoNucleusDatasetUiUpdateSnapshotStatusInput) throws ApiException {
ApiResponse localVarResponse = updateSnapshotStatusWithHttpInfo(snapshotId, dominoNucleusDatasetUiUpdateSnapshotStatusInput);
return localVarResponse.getData();
}
/**
* update a dataset snapshot's status
*
* @param snapshotId Id of the dataset snapshot to be updated (required)
* @param dominoNucleusDatasetUiUpdateSnapshotStatusInput JSON object with information describing the new status (required)
* @return ApiResponse<DominoDatasetApiDatasetSnapshotDto>
* @throws ApiException if fails to make API call
*/
public ApiResponse updateSnapshotStatusWithHttpInfo(String snapshotId, DominoNucleusDatasetUiUpdateSnapshotStatusInput dominoNucleusDatasetUiUpdateSnapshotStatusInput) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = updateSnapshotStatusRequestBuilder(snapshotId, dominoNucleusDatasetUiUpdateSnapshotStatusInput);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("updateSnapshotStatus", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder updateSnapshotStatusRequestBuilder(String snapshotId, DominoNucleusDatasetUiUpdateSnapshotStatusInput dominoNucleusDatasetUiUpdateSnapshotStatusInput) throws ApiException {
// verify the required parameter 'snapshotId' is set
if (snapshotId == null) {
throw new ApiException(400, "Missing the required parameter 'snapshotId' when calling updateSnapshotStatus");
}
// verify the required parameter 'dominoNucleusDatasetUiUpdateSnapshotStatusInput' is set
if (dominoNucleusDatasetUiUpdateSnapshotStatusInput == null) {
throw new ApiException(400, "Missing the required parameter 'dominoNucleusDatasetUiUpdateSnapshotStatusInput' when calling updateSnapshotStatus");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/{snapshotId}"
.replace("{snapshotId}", ApiClient.urlEncode(snapshotId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(dominoNucleusDatasetUiUpdateSnapshotStatusInput);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Tests whether or not this chunk has already been sent
*
* @param datasetId (required)
* @param resumableChunkNumber (optional)
* @param resumableTotalChunks (optional)
* @param resumableIdentifier (optional)
* @param resumableRelativePath (optional)
* @throws ApiException if fails to make API call
*/
public void uploadSnapshotFileTest(String datasetId, Integer resumableChunkNumber, Integer resumableTotalChunks, String resumableIdentifier, String resumableRelativePath) throws ApiException {
uploadSnapshotFileTestWithHttpInfo(datasetId, resumableChunkNumber, resumableTotalChunks, resumableIdentifier, resumableRelativePath);
}
/**
* Tests whether or not this chunk has already been sent
*
* @param datasetId (required)
* @param resumableChunkNumber (optional)
* @param resumableTotalChunks (optional)
* @param resumableIdentifier (optional)
* @param resumableRelativePath (optional)
* @return ApiResponse<Void>
* @throws ApiException if fails to make API call
*/
public ApiResponse uploadSnapshotFileTestWithHttpInfo(String datasetId, Integer resumableChunkNumber, Integer resumableTotalChunks, String resumableIdentifier, String resumableRelativePath) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = uploadSnapshotFileTestRequestBuilder(datasetId, resumableChunkNumber, resumableTotalChunks, resumableIdentifier, resumableRelativePath);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("uploadSnapshotFileTest", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
null
);
} finally {
// Drain the InputStream
while (localVarResponse.body().read() != -1) {
// Ignore
}
localVarResponse.body().close();
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder uploadSnapshotFileTestRequestBuilder(String datasetId, Integer resumableChunkNumber, Integer resumableTotalChunks, String resumableIdentifier, String resumableRelativePath) throws ApiException {
// verify the required parameter 'datasetId' is set
if (datasetId == null) {
throw new ApiException(400, "Missing the required parameter 'datasetId' when calling uploadSnapshotFileTest");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/collections/{datasetId}/snapshot/file/test"
.replace("{datasetId}", ApiClient.urlEncode(datasetId.toString()));
List localVarQueryParams = new ArrayList<>();
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
localVarQueryParameterBaseName = "resumableChunkNumber";
localVarQueryParams.addAll(ApiClient.parameterToPairs("resumableChunkNumber", resumableChunkNumber));
localVarQueryParameterBaseName = "resumableTotalChunks";
localVarQueryParams.addAll(ApiClient.parameterToPairs("resumableTotalChunks", resumableTotalChunks));
localVarQueryParameterBaseName = "resumableIdentifier";
localVarQueryParams.addAll(ApiClient.parameterToPairs("resumableIdentifier", resumableIdentifier));
localVarQueryParameterBaseName = "resumableRelativePath";
localVarQueryParams.addAll(ApiClient.parameterToPairs("resumableRelativePath", resumableRelativePath));
if (!localVarQueryParams.isEmpty() || localVarQueryStringJoiner.length() != 0) {
StringJoiner queryJoiner = new StringJoiner("&");
localVarQueryParams.forEach(p -> queryJoiner.add(p.getName() + '=' + p.getValue()));
if (localVarQueryStringJoiner.length() != 0) {
queryJoiner.add(localVarQueryStringJoiner.toString());
}
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath + '?' + queryJoiner.toString()));
} else {
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
}
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Sends file upload chunks
*
* @param datasetId (required)
* @param resumableChunkNumber (optional)
* @param resumableTotalChunks (optional)
* @param resumableIdentifier (optional)
* @param key unique identifier for this upload session (optional)
* @param resumableRelativePath (optional)
* @param resumableChunkSize (optional)
* @return String
* @throws ApiException if fails to make API call
*/
public String uploadSnapshotFileUi(String datasetId, Integer resumableChunkNumber, Integer resumableTotalChunks, String resumableIdentifier, String key, String resumableRelativePath, Integer resumableChunkSize) throws ApiException {
ApiResponse localVarResponse = uploadSnapshotFileUiWithHttpInfo(datasetId, resumableChunkNumber, resumableTotalChunks, resumableIdentifier, key, resumableRelativePath, resumableChunkSize);
return localVarResponse.getData();
}
/**
* Sends file upload chunks
*
* @param datasetId (required)
* @param resumableChunkNumber (optional)
* @param resumableTotalChunks (optional)
* @param resumableIdentifier (optional)
* @param key unique identifier for this upload session (optional)
* @param resumableRelativePath (optional)
* @param resumableChunkSize (optional)
* @return ApiResponse<String>
* @throws ApiException if fails to make API call
*/
public ApiResponse uploadSnapshotFileUiWithHttpInfo(String datasetId, Integer resumableChunkNumber, Integer resumableTotalChunks, String resumableIdentifier, String key, String resumableRelativePath, Integer resumableChunkSize) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = uploadSnapshotFileUiRequestBuilder(datasetId, resumableChunkNumber, resumableTotalChunks, resumableIdentifier, key, resumableRelativePath, resumableChunkSize);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("uploadSnapshotFileUi", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder uploadSnapshotFileUiRequestBuilder(String datasetId, Integer resumableChunkNumber, Integer resumableTotalChunks, String resumableIdentifier, String key, String resumableRelativePath, Integer resumableChunkSize) throws ApiException {
// verify the required parameter 'datasetId' is set
if (datasetId == null) {
throw new ApiException(400, "Missing the required parameter 'datasetId' when calling uploadSnapshotFileUi");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/datasetUi/collections/{datasetId}/snapshot/file"
.replace("{datasetId}", ApiClient.urlEncode(datasetId.toString()));
List localVarQueryParams = new ArrayList<>();
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
localVarQueryParameterBaseName = "resumableChunkNumber";
localVarQueryParams.addAll(ApiClient.parameterToPairs("resumableChunkNumber", resumableChunkNumber));
localVarQueryParameterBaseName = "resumableTotalChunks";
localVarQueryParams.addAll(ApiClient.parameterToPairs("resumableTotalChunks", resumableTotalChunks));
localVarQueryParameterBaseName = "resumableIdentifier";
localVarQueryParams.addAll(ApiClient.parameterToPairs("resumableIdentifier", resumableIdentifier));
localVarQueryParameterBaseName = "key";
localVarQueryParams.addAll(ApiClient.parameterToPairs("key", key));
localVarQueryParameterBaseName = "resumableRelativePath";
localVarQueryParams.addAll(ApiClient.parameterToPairs("resumableRelativePath", resumableRelativePath));
localVarQueryParameterBaseName = "resumableChunkSize";
localVarQueryParams.addAll(ApiClient.parameterToPairs("resumableChunkSize", resumableChunkSize));
if (!localVarQueryParams.isEmpty() || localVarQueryStringJoiner.length() != 0) {
StringJoiner queryJoiner = new StringJoiner("&");
localVarQueryParams.forEach(p -> queryJoiner.add(p.getName() + '=' + p.getValue()));
if (localVarQueryStringJoiner.length() != 0) {
queryJoiner.add(localVarQueryStringJoiner.toString());
}
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath + '?' + queryJoiner.toString()));
} else {
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
}
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy