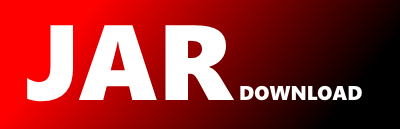
com.dominodatalab.api.rest.JobCommentsApi Maven / Gradle / Ivy
/*
* Domino Data Lab API v4
* This API is going to provide access to all the Domino functions available in the user interface. To authenticate your requests, include your API Key (which you can find on your account page) with the header X-Domino-Api-Key.
*
* The version of the OpenAPI document: 4.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.api.rest;
import com.dominodatalab.api.invoker.ApiClient;
import com.dominodatalab.api.invoker.ApiException;
import com.dominodatalab.api.invoker.ApiResponse;
import com.dominodatalab.api.invoker.Pair;
import com.dominodatalab.api.model.DominoApiErrorResponse;
import com.dominodatalab.api.model.DominoJobsInterfaceComment;
import com.dominodatalab.api.model.DominoJobsInterfaceCommentThread;
import com.dominodatalab.api.model.DominoJobsWebArchiveComment;
import com.dominodatalab.api.model.DominoJobsWebCreateComment;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import org.apache.http.HttpEntity;
import org.apache.http.NameValuePair;
import org.apache.http.entity.mime.MultipartEntityBuilder;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import java.io.InputStream;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.IOException;
import java.io.OutputStream;
import java.net.http.HttpRequest;
import java.nio.channels.Channels;
import java.nio.channels.Pipe;
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.time.Duration;
import java.util.ArrayList;
import java.util.StringJoiner;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.function.Consumer;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2023-10-17T15:20:46.682098100-04:00[America/New_York]")
public class JobCommentsApi {
private final HttpClient memberVarHttpClient;
private final ObjectMapper memberVarObjectMapper;
private final String memberVarBaseUri;
private final Consumer memberVarInterceptor;
private final Duration memberVarReadTimeout;
private final Consumer> memberVarResponseInterceptor;
private final Consumer> memberVarAsyncResponseInterceptor;
public JobCommentsApi() {
this(new ApiClient());
}
public JobCommentsApi(ApiClient apiClient) {
memberVarHttpClient = apiClient.getHttpClient();
memberVarObjectMapper = apiClient.getObjectMapper();
memberVarBaseUri = apiClient.getBaseUri();
memberVarInterceptor = apiClient.getRequestInterceptor();
memberVarReadTimeout = apiClient.getReadTimeout();
memberVarResponseInterceptor = apiClient.getResponseInterceptor();
memberVarAsyncResponseInterceptor = apiClient.getAsyncResponseInterceptor();
}
protected ApiException getApiException(String operationId, HttpResponse response) throws IOException {
String body = response.body() == null ? null : new String(response.body().readAllBytes());
String message = formatExceptionMessage(operationId, response.statusCode(), body);
return new ApiException(response.statusCode(), message, response.headers(), body);
}
private String formatExceptionMessage(String operationId, int statusCode, String body) {
if (body == null || body.isEmpty()) {
body = "[no body]";
}
return operationId + " call failed with: " + statusCode + " - " + body;
}
/**
* Archive the comment in a comment thread
*
* @param jobId (required)
* @param threadId (required)
* @param dominoJobsWebArchiveComment JSON object with information about the index of the comment to be archived (required)
* @return DominoJobsInterfaceComment
* @throws ApiException if fails to make API call
*/
public DominoJobsInterfaceComment archiveComment(String jobId, String threadId, DominoJobsWebArchiveComment dominoJobsWebArchiveComment) throws ApiException {
ApiResponse localVarResponse = archiveCommentWithHttpInfo(jobId, threadId, dominoJobsWebArchiveComment);
return localVarResponse.getData();
}
/**
* Archive the comment in a comment thread
*
* @param jobId (required)
* @param threadId (required)
* @param dominoJobsWebArchiveComment JSON object with information about the index of the comment to be archived (required)
* @return ApiResponse<DominoJobsInterfaceComment>
* @throws ApiException if fails to make API call
*/
public ApiResponse archiveCommentWithHttpInfo(String jobId, String threadId, DominoJobsWebArchiveComment dominoJobsWebArchiveComment) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = archiveCommentRequestBuilder(jobId, threadId, dominoJobsWebArchiveComment);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("archiveComment", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder archiveCommentRequestBuilder(String jobId, String threadId, DominoJobsWebArchiveComment dominoJobsWebArchiveComment) throws ApiException {
// verify the required parameter 'jobId' is set
if (jobId == null) {
throw new ApiException(400, "Missing the required parameter 'jobId' when calling archiveComment");
}
// verify the required parameter 'threadId' is set
if (threadId == null) {
throw new ApiException(400, "Missing the required parameter 'threadId' when calling archiveComment");
}
// verify the required parameter 'dominoJobsWebArchiveComment' is set
if (dominoJobsWebArchiveComment == null) {
throw new ApiException(400, "Missing the required parameter 'dominoJobsWebArchiveComment' when calling archiveComment");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/jobs/{jobId}/comment/{threadId}"
.replace("{jobId}", ApiClient.urlEncode(jobId.toString()))
.replace("{threadId}", ApiClient.urlEncode(threadId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(dominoJobsWebArchiveComment);
localVarRequestBuilder.method("DELETE", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Create a Job Comment
*
* @param jobId (required)
* @param dominoJobsWebCreateComment JSON object with information to comment on the job (required)
* @return DominoJobsInterfaceCommentThread
* @throws ApiException if fails to make API call
*/
public DominoJobsInterfaceCommentThread createJobComment(String jobId, DominoJobsWebCreateComment dominoJobsWebCreateComment) throws ApiException {
ApiResponse localVarResponse = createJobCommentWithHttpInfo(jobId, dominoJobsWebCreateComment);
return localVarResponse.getData();
}
/**
* Create a Job Comment
*
* @param jobId (required)
* @param dominoJobsWebCreateComment JSON object with information to comment on the job (required)
* @return ApiResponse<DominoJobsInterfaceCommentThread>
* @throws ApiException if fails to make API call
*/
public ApiResponse createJobCommentWithHttpInfo(String jobId, DominoJobsWebCreateComment dominoJobsWebCreateComment) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = createJobCommentRequestBuilder(jobId, dominoJobsWebCreateComment);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("createJobComment", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder createJobCommentRequestBuilder(String jobId, DominoJobsWebCreateComment dominoJobsWebCreateComment) throws ApiException {
// verify the required parameter 'jobId' is set
if (jobId == null) {
throw new ApiException(400, "Missing the required parameter 'jobId' when calling createJobComment");
}
// verify the required parameter 'dominoJobsWebCreateComment' is set
if (dominoJobsWebCreateComment == null) {
throw new ApiException(400, "Missing the required parameter 'dominoJobsWebCreateComment' when calling createJobComment");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/jobs/{jobId}/comment"
.replace("{jobId}", ApiClient.urlEncode(jobId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(dominoJobsWebCreateComment);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Create a Job Result File Comment
*
* @param jobId (required)
* @param fileName Name of the result file to comment to (required)
* @param commitId git commit identifier to pick the result file (required)
* @param dominoJobsWebCreateComment JSON object with information to comment on a result file (required)
* @return DominoJobsInterfaceCommentThread
* @throws ApiException if fails to make API call
*/
public DominoJobsInterfaceCommentThread createJobResultFileComment(String jobId, String fileName, String commitId, DominoJobsWebCreateComment dominoJobsWebCreateComment) throws ApiException {
ApiResponse localVarResponse = createJobResultFileCommentWithHttpInfo(jobId, fileName, commitId, dominoJobsWebCreateComment);
return localVarResponse.getData();
}
/**
* Create a Job Result File Comment
*
* @param jobId (required)
* @param fileName Name of the result file to comment to (required)
* @param commitId git commit identifier to pick the result file (required)
* @param dominoJobsWebCreateComment JSON object with information to comment on a result file (required)
* @return ApiResponse<DominoJobsInterfaceCommentThread>
* @throws ApiException if fails to make API call
*/
public ApiResponse createJobResultFileCommentWithHttpInfo(String jobId, String fileName, String commitId, DominoJobsWebCreateComment dominoJobsWebCreateComment) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = createJobResultFileCommentRequestBuilder(jobId, fileName, commitId, dominoJobsWebCreateComment);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("createJobResultFileComment", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder createJobResultFileCommentRequestBuilder(String jobId, String fileName, String commitId, DominoJobsWebCreateComment dominoJobsWebCreateComment) throws ApiException {
// verify the required parameter 'jobId' is set
if (jobId == null) {
throw new ApiException(400, "Missing the required parameter 'jobId' when calling createJobResultFileComment");
}
// verify the required parameter 'fileName' is set
if (fileName == null) {
throw new ApiException(400, "Missing the required parameter 'fileName' when calling createJobResultFileComment");
}
// verify the required parameter 'commitId' is set
if (commitId == null) {
throw new ApiException(400, "Missing the required parameter 'commitId' when calling createJobResultFileComment");
}
// verify the required parameter 'dominoJobsWebCreateComment' is set
if (dominoJobsWebCreateComment == null) {
throw new ApiException(400, "Missing the required parameter 'dominoJobsWebCreateComment' when calling createJobResultFileComment");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/jobs/{jobId}/file/comment"
.replace("{jobId}", ApiClient.urlEncode(jobId.toString()));
List localVarQueryParams = new ArrayList<>();
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
localVarQueryParameterBaseName = "fileName";
localVarQueryParams.addAll(ApiClient.parameterToPairs("fileName", fileName));
localVarQueryParameterBaseName = "commitId";
localVarQueryParams.addAll(ApiClient.parameterToPairs("commitId", commitId));
if (!localVarQueryParams.isEmpty() || localVarQueryStringJoiner.length() != 0) {
StringJoiner queryJoiner = new StringJoiner("&");
localVarQueryParams.forEach(p -> queryJoiner.add(p.getName() + '=' + p.getValue()));
if (localVarQueryStringJoiner.length() != 0) {
queryJoiner.add(localVarQueryStringJoiner.toString());
}
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath + '?' + queryJoiner.toString()));
} else {
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
}
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(dominoJobsWebCreateComment);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Get all aggregated comments
*
* @param jobId (required)
* @return List<DominoJobsInterfaceCommentThread>
* @throws ApiException if fails to make API call
*/
public List getAllComments(String jobId) throws ApiException {
ApiResponse> localVarResponse = getAllCommentsWithHttpInfo(jobId);
return localVarResponse.getData();
}
/**
* Get all aggregated comments
*
* @param jobId (required)
* @return ApiResponse<List<DominoJobsInterfaceCommentThread>>
* @throws ApiException if fails to make API call
*/
public ApiResponse> getAllCommentsWithHttpInfo(String jobId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getAllCommentsRequestBuilder(jobId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getAllComments", localVarResponse);
}
return new ApiResponse>(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference>() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getAllCommentsRequestBuilder(String jobId) throws ApiException {
// verify the required parameter 'jobId' is set
if (jobId == null) {
throw new ApiException(400, "Missing the required parameter 'jobId' when calling getAllComments");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/jobs/{jobId}/allComments"
.replace("{jobId}", ApiClient.urlEncode(jobId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Get all comments specific to job context
*
* @param jobId (required)
* @return DominoJobsInterfaceCommentThread
* @throws ApiException if fails to make API call
*/
public DominoJobsInterfaceCommentThread getComments(String jobId) throws ApiException {
ApiResponse localVarResponse = getCommentsWithHttpInfo(jobId);
return localVarResponse.getData();
}
/**
* Get all comments specific to job context
*
* @param jobId (required)
* @return ApiResponse<DominoJobsInterfaceCommentThread>
* @throws ApiException if fails to make API call
*/
public ApiResponse getCommentsWithHttpInfo(String jobId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getCommentsRequestBuilder(jobId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getComments", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getCommentsRequestBuilder(String jobId) throws ApiException {
// verify the required parameter 'jobId' is set
if (jobId == null) {
throw new ApiException(400, "Missing the required parameter 'jobId' when calling getComments");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/jobs/{jobId}/comments"
.replace("{jobId}", ApiClient.urlEncode(jobId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Get all comments specific to result files generated by running the job
*
* @param jobId (required)
* @param fileName Name of the result file (required)
* @param commitId git commit identifier to pick the result file (required)
* @return DominoJobsInterfaceCommentThread
* @throws ApiException if fails to make API call
*/
public DominoJobsInterfaceCommentThread getResultFileComments(String jobId, String fileName, String commitId) throws ApiException {
ApiResponse localVarResponse = getResultFileCommentsWithHttpInfo(jobId, fileName, commitId);
return localVarResponse.getData();
}
/**
* Get all comments specific to result files generated by running the job
*
* @param jobId (required)
* @param fileName Name of the result file (required)
* @param commitId git commit identifier to pick the result file (required)
* @return ApiResponse<DominoJobsInterfaceCommentThread>
* @throws ApiException if fails to make API call
*/
public ApiResponse getResultFileCommentsWithHttpInfo(String jobId, String fileName, String commitId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getResultFileCommentsRequestBuilder(jobId, fileName, commitId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getResultFileComments", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getResultFileCommentsRequestBuilder(String jobId, String fileName, String commitId) throws ApiException {
// verify the required parameter 'jobId' is set
if (jobId == null) {
throw new ApiException(400, "Missing the required parameter 'jobId' when calling getResultFileComments");
}
// verify the required parameter 'fileName' is set
if (fileName == null) {
throw new ApiException(400, "Missing the required parameter 'fileName' when calling getResultFileComments");
}
// verify the required parameter 'commitId' is set
if (commitId == null) {
throw new ApiException(400, "Missing the required parameter 'commitId' when calling getResultFileComments");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/jobs/{jobId}/resultFile/comments"
.replace("{jobId}", ApiClient.urlEncode(jobId.toString()));
List localVarQueryParams = new ArrayList<>();
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
localVarQueryParameterBaseName = "fileName";
localVarQueryParams.addAll(ApiClient.parameterToPairs("fileName", fileName));
localVarQueryParameterBaseName = "commitId";
localVarQueryParams.addAll(ApiClient.parameterToPairs("commitId", commitId));
if (!localVarQueryParams.isEmpty() || localVarQueryStringJoiner.length() != 0) {
StringJoiner queryJoiner = new StringJoiner("&");
localVarQueryParams.forEach(p -> queryJoiner.add(p.getName() + '=' + p.getValue()));
if (localVarQueryStringJoiner.length() != 0) {
queryJoiner.add(localVarQueryStringJoiner.toString());
}
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath + '?' + queryJoiner.toString()));
} else {
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
}
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy