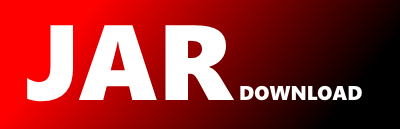
com.dominodatalab.api.rest.ModelProductsApi Maven / Gradle / Ivy
/*
* Domino Data Lab API v4
* This API is going to provide access to all the Domino functions available in the user interface. To authenticate your requests, include your API Key (which you can find on your account page) with the header X-Domino-Api-Key.
*
* The version of the OpenAPI document: 4.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.api.rest;
import com.dominodatalab.api.invoker.ApiClient;
import com.dominodatalab.api.invoker.ApiException;
import com.dominodatalab.api.invoker.ApiResponse;
import com.dominodatalab.api.invoker.Pair;
import java.math.BigDecimal;
import com.dominodatalab.api.model.DominoApiErrorResponse;
import com.dominodatalab.api.model.DominoCommonModelproductAppGoal;
import com.dominodatalab.api.model.DominoCommonModelproductAppVersionDetails;
import com.dominodatalab.api.model.DominoCommonModelproductAppVersionOverview;
import com.dominodatalab.api.model.DominoCommonModelproductLogSet;
import com.dominodatalab.api.model.DominoNucleusModelproductModelsAccessRequest;
import com.dominodatalab.api.model.DominoNucleusModelproductModelsConsumerModelProduct;
import com.dominodatalab.api.model.DominoNucleusModelproductModelsEmailInvitationResponse;
import com.dominodatalab.api.model.DominoNucleusModelproductModelsGrantAccessRequired;
import com.dominodatalab.api.model.DominoNucleusModelproductModelsGranteeList;
import com.dominodatalab.api.model.DominoNucleusModelproductModelsIsRunnable;
import com.dominodatalab.api.model.DominoNucleusModelproductModelsLinkAppToGoal;
import com.dominodatalab.api.model.DominoNucleusModelproductModelsLoginRequired;
import com.dominodatalab.api.model.DominoNucleusModelproductModelsModelProduct;
import com.dominodatalab.api.model.DominoNucleusModelproductModelsModelProductError;
import com.dominodatalab.api.model.DominoNucleusModelproductModelsStartParams;
import com.dominodatalab.api.model.DominoNucleusModelproductModelsUninviteRequest;
import com.dominodatalab.api.model.DominoNucleusModelproductModelsUnlinkAppFromGoal;
import com.dominodatalab.api.model.DominoNucleusModelproductModelsUsageStatisticsRecorded;
import com.dominodatalab.api.model.DominoNucleusModelproductModelsUsageStatisticsTimeseriesResponse;
import com.dominodatalab.api.model.DominoNucleusModelproductModelsUsageStatisticsTopNResponse;
import com.dominodatalab.api.model.DominoNucleusModelproductModelsUsageStatisticsTotalResponse;
import com.dominodatalab.api.model.DominoNucleusModelproductModelsVisibilityPatch;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import org.apache.http.HttpEntity;
import org.apache.http.NameValuePair;
import org.apache.http.entity.mime.MultipartEntityBuilder;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import java.io.InputStream;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.IOException;
import java.io.OutputStream;
import java.net.http.HttpRequest;
import java.nio.channels.Channels;
import java.nio.channels.Pipe;
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.time.Duration;
import java.util.ArrayList;
import java.util.StringJoiner;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.function.Consumer;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2023-10-17T15:20:46.682098100-04:00[America/New_York]")
public class ModelProductsApi {
private final HttpClient memberVarHttpClient;
private final ObjectMapper memberVarObjectMapper;
private final String memberVarBaseUri;
private final Consumer memberVarInterceptor;
private final Duration memberVarReadTimeout;
private final Consumer> memberVarResponseInterceptor;
private final Consumer> memberVarAsyncResponseInterceptor;
public ModelProductsApi() {
this(new ApiClient());
}
public ModelProductsApi(ApiClient apiClient) {
memberVarHttpClient = apiClient.getHttpClient();
memberVarObjectMapper = apiClient.getObjectMapper();
memberVarBaseUri = apiClient.getBaseUri();
memberVarInterceptor = apiClient.getRequestInterceptor();
memberVarReadTimeout = apiClient.getReadTimeout();
memberVarResponseInterceptor = apiClient.getResponseInterceptor();
memberVarAsyncResponseInterceptor = apiClient.getAsyncResponseInterceptor();
}
protected ApiException getApiException(String operationId, HttpResponse response) throws IOException {
String body = response.body() == null ? null : new String(response.body().readAllBytes());
String message = formatExceptionMessage(operationId, response.statusCode(), body);
return new ApiException(response.statusCode(), message, response.headers(), body);
}
private String formatExceptionMessage(String operationId, int statusCode, String body) {
if (body == null || body.isEmpty()) {
body = "[no body]";
}
return operationId + " call failed with: " + statusCode + " - " + body;
}
/**
* grants access to a ModelProduct to multiple users
*
* @param modelProductId (required)
* @param dominoNucleusModelproductModelsGranteeList (required)
* @return DominoNucleusModelproductModelsEmailInvitationResponse
* @throws ApiException if fails to make API call
*/
public DominoNucleusModelproductModelsEmailInvitationResponse bulkGrantAccess(String modelProductId, DominoNucleusModelproductModelsGranteeList dominoNucleusModelproductModelsGranteeList) throws ApiException {
ApiResponse localVarResponse = bulkGrantAccessWithHttpInfo(modelProductId, dominoNucleusModelproductModelsGranteeList);
return localVarResponse.getData();
}
/**
* grants access to a ModelProduct to multiple users
*
* @param modelProductId (required)
* @param dominoNucleusModelproductModelsGranteeList (required)
* @return ApiResponse<DominoNucleusModelproductModelsEmailInvitationResponse>
* @throws ApiException if fails to make API call
*/
public ApiResponse bulkGrantAccessWithHttpInfo(String modelProductId, DominoNucleusModelproductModelsGranteeList dominoNucleusModelproductModelsGranteeList) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = bulkGrantAccessRequestBuilder(modelProductId, dominoNucleusModelproductModelsGranteeList);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("bulkGrantAccess", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder bulkGrantAccessRequestBuilder(String modelProductId, DominoNucleusModelproductModelsGranteeList dominoNucleusModelproductModelsGranteeList) throws ApiException {
// verify the required parameter 'modelProductId' is set
if (modelProductId == null) {
throw new ApiException(400, "Missing the required parameter 'modelProductId' when calling bulkGrantAccess");
}
// verify the required parameter 'dominoNucleusModelproductModelsGranteeList' is set
if (dominoNucleusModelproductModelsGranteeList == null) {
throw new ApiException(400, "Missing the required parameter 'dominoNucleusModelproductModelsGranteeList' when calling bulkGrantAccess");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/modelProducts/{modelProductId}/invite"
.replace("{modelProductId}", ApiClient.urlEncode(modelProductId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(dominoNucleusModelproductModelsGranteeList);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* retrieves a Model Product by id
*
* @param modelProductId (required)
* @return DominoNucleusModelproductModelsConsumerModelProduct
* @throws ApiException if fails to make API call
*/
public DominoNucleusModelproductModelsConsumerModelProduct consumerSummary(String modelProductId) throws ApiException {
ApiResponse localVarResponse = consumerSummaryWithHttpInfo(modelProductId);
return localVarResponse.getData();
}
/**
* retrieves a Model Product by id
*
* @param modelProductId (required)
* @return ApiResponse<DominoNucleusModelproductModelsConsumerModelProduct>
* @throws ApiException if fails to make API call
*/
public ApiResponse consumerSummaryWithHttpInfo(String modelProductId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = consumerSummaryRequestBuilder(modelProductId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("consumerSummary", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder consumerSummaryRequestBuilder(String modelProductId) throws ApiException {
// verify the required parameter 'modelProductId' is set
if (modelProductId == null) {
throw new ApiException(400, "Missing the required parameter 'modelProductId' when calling consumerSummary");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/modelProducts/consumer/{modelProductId}"
.replace("{modelProductId}", ApiClient.urlEncode(modelProductId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* creates a new ModelProduct
*
* @param dominoNucleusModelproductModelsModelProduct (required)
* @return DominoNucleusModelproductModelsModelProduct
* @throws ApiException if fails to make API call
*/
public DominoNucleusModelproductModelsModelProduct createModelProduct(DominoNucleusModelproductModelsModelProduct dominoNucleusModelproductModelsModelProduct) throws ApiException {
ApiResponse localVarResponse = createModelProductWithHttpInfo(dominoNucleusModelproductModelsModelProduct);
return localVarResponse.getData();
}
/**
* creates a new ModelProduct
*
* @param dominoNucleusModelproductModelsModelProduct (required)
* @return ApiResponse<DominoNucleusModelproductModelsModelProduct>
* @throws ApiException if fails to make API call
*/
public ApiResponse createModelProductWithHttpInfo(DominoNucleusModelproductModelsModelProduct dominoNucleusModelproductModelsModelProduct) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = createModelProductRequestBuilder(dominoNucleusModelproductModelsModelProduct);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("createModelProduct", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder createModelProductRequestBuilder(DominoNucleusModelproductModelsModelProduct dominoNucleusModelproductModelsModelProduct) throws ApiException {
// verify the required parameter 'dominoNucleusModelproductModelsModelProduct' is set
if (dominoNucleusModelproductModelsModelProduct == null) {
throw new ApiException(400, "Missing the required parameter 'dominoNucleusModelproductModelsModelProduct' when calling createModelProduct");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/modelProducts";
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(dominoNucleusModelproductModelsModelProduct);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* denies access to a ModelProduct to a user
*
* @param modelProductId (required)
* @param dominoNucleusModelproductModelsAccessRequest (required)
* @return String
* @throws ApiException if fails to make API call
*/
public String denyAccess(String modelProductId, DominoNucleusModelproductModelsAccessRequest dominoNucleusModelproductModelsAccessRequest) throws ApiException {
ApiResponse localVarResponse = denyAccessWithHttpInfo(modelProductId, dominoNucleusModelproductModelsAccessRequest);
return localVarResponse.getData();
}
/**
* denies access to a ModelProduct to a user
*
* @param modelProductId (required)
* @param dominoNucleusModelproductModelsAccessRequest (required)
* @return ApiResponse<String>
* @throws ApiException if fails to make API call
*/
public ApiResponse denyAccessWithHttpInfo(String modelProductId, DominoNucleusModelproductModelsAccessRequest dominoNucleusModelproductModelsAccessRequest) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = denyAccessRequestBuilder(modelProductId, dominoNucleusModelproductModelsAccessRequest);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("denyAccess", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder denyAccessRequestBuilder(String modelProductId, DominoNucleusModelproductModelsAccessRequest dominoNucleusModelproductModelsAccessRequest) throws ApiException {
// verify the required parameter 'modelProductId' is set
if (modelProductId == null) {
throw new ApiException(400, "Missing the required parameter 'modelProductId' when calling denyAccess");
}
// verify the required parameter 'dominoNucleusModelproductModelsAccessRequest' is set
if (dominoNucleusModelproductModelsAccessRequest == null) {
throw new ApiException(400, "Missing the required parameter 'dominoNucleusModelproductModelsAccessRequest' when calling denyAccess");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/modelProducts/{modelProductId}/denyAccess"
.replace("{modelProductId}", ApiClient.urlEncode(modelProductId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(dominoNucleusModelproductModelsAccessRequest);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Get all Versions of an App
*
* @param modelProductId (required)
* @return List<DominoCommonModelproductAppVersionOverview>
* @throws ApiException if fails to make API call
*/
public List getAllAppVersions(String modelProductId) throws ApiException {
ApiResponse> localVarResponse = getAllAppVersionsWithHttpInfo(modelProductId);
return localVarResponse.getData();
}
/**
* Get all Versions of an App
*
* @param modelProductId (required)
* @return ApiResponse<List<DominoCommonModelproductAppVersionOverview>>
* @throws ApiException if fails to make API call
*/
public ApiResponse> getAllAppVersionsWithHttpInfo(String modelProductId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getAllAppVersionsRequestBuilder(modelProductId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getAllAppVersions", localVarResponse);
}
return new ApiResponse>(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference>() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getAllAppVersionsRequestBuilder(String modelProductId) throws ApiException {
// verify the required parameter 'modelProductId' is set
if (modelProductId == null) {
throw new ApiException(400, "Missing the required parameter 'modelProductId' when calling getAllAppVersions");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/modelProducts/{modelProductId}/versions"
.replace("{modelProductId}", ApiClient.urlEncode(modelProductId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Get the details of a particular App Version
*
* @param appVersionId (required)
* @return DominoCommonModelproductAppVersionDetails
* @throws ApiException if fails to make API call
*/
public DominoCommonModelproductAppVersionDetails getAppVersionDetails(String appVersionId) throws ApiException {
ApiResponse localVarResponse = getAppVersionDetailsWithHttpInfo(appVersionId);
return localVarResponse.getData();
}
/**
* Get the details of a particular App Version
*
* @param appVersionId (required)
* @return ApiResponse<DominoCommonModelproductAppVersionDetails>
* @throws ApiException if fails to make API call
*/
public ApiResponse getAppVersionDetailsWithHttpInfo(String appVersionId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getAppVersionDetailsRequestBuilder(appVersionId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getAppVersionDetails", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getAppVersionDetailsRequestBuilder(String appVersionId) throws ApiException {
// verify the required parameter 'appVersionId' is set
if (appVersionId == null) {
throw new ApiException(400, "Missing the required parameter 'appVersionId' when calling getAppVersionDetails");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/modelProducts/apps/versions/{appVersionId}"
.replace("{appVersionId}", ApiClient.urlEncode(appVersionId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Get the logs of an App Version
*
* @param appVersionId (required)
* @param logType Types of logs: * `console` - This is the default if the value is not provided. All logs lines displayed in the app instance's runtime environment. * `stdout` - Log lines displayed in the stdout of the app instance's runtime environment. * `stderr` - Log lines displayed in the stderr of the app instance's runtime environment. * `stdoutstderr` - Interleaved stdout and stderr. * `prepareoutput` - Log lines generated by the environment preparing the app instance. (optional, default to console)
* @param limit Max number of log lines to fetch. Will be overridden by the configuration's limit if this value exceeds the configuration's limit, or if this value is not provided. (optional, default to 10000)
* @param offset The index of the current body of logs to start fetching from. 0 by default and typically won't be used for a timestamp-based offset log fetching strategy. (optional, default to 0)
* @param latestTimeNano The epoch time in nanoseconds to start fetching from, such as \"1543538813745986325\". \"0\" by default and will typically be used for a timestamp-based offset log fetching strategy. (optional, default to 0)
* @return DominoCommonModelproductLogSet
* @throws ApiException if fails to make API call
*/
public DominoCommonModelproductLogSet getAppVersionLogs(String appVersionId, String logType, BigDecimal limit, BigDecimal offset, String latestTimeNano) throws ApiException {
ApiResponse localVarResponse = getAppVersionLogsWithHttpInfo(appVersionId, logType, limit, offset, latestTimeNano);
return localVarResponse.getData();
}
/**
* Get the logs of an App Version
*
* @param appVersionId (required)
* @param logType Types of logs: * `console` - This is the default if the value is not provided. All logs lines displayed in the app instance's runtime environment. * `stdout` - Log lines displayed in the stdout of the app instance's runtime environment. * `stderr` - Log lines displayed in the stderr of the app instance's runtime environment. * `stdoutstderr` - Interleaved stdout and stderr. * `prepareoutput` - Log lines generated by the environment preparing the app instance. (optional, default to console)
* @param limit Max number of log lines to fetch. Will be overridden by the configuration's limit if this value exceeds the configuration's limit, or if this value is not provided. (optional, default to 10000)
* @param offset The index of the current body of logs to start fetching from. 0 by default and typically won't be used for a timestamp-based offset log fetching strategy. (optional, default to 0)
* @param latestTimeNano The epoch time in nanoseconds to start fetching from, such as \"1543538813745986325\". \"0\" by default and will typically be used for a timestamp-based offset log fetching strategy. (optional, default to 0)
* @return ApiResponse<DominoCommonModelproductLogSet>
* @throws ApiException if fails to make API call
*/
public ApiResponse getAppVersionLogsWithHttpInfo(String appVersionId, String logType, BigDecimal limit, BigDecimal offset, String latestTimeNano) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getAppVersionLogsRequestBuilder(appVersionId, logType, limit, offset, latestTimeNano);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getAppVersionLogs", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getAppVersionLogsRequestBuilder(String appVersionId, String logType, BigDecimal limit, BigDecimal offset, String latestTimeNano) throws ApiException {
// verify the required parameter 'appVersionId' is set
if (appVersionId == null) {
throw new ApiException(400, "Missing the required parameter 'appVersionId' when calling getAppVersionLogs");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/modelProducts/apps/versions/{appVersionId}/logs"
.replace("{appVersionId}", ApiClient.urlEncode(appVersionId.toString()));
List localVarQueryParams = new ArrayList<>();
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
localVarQueryParameterBaseName = "logType";
localVarQueryParams.addAll(ApiClient.parameterToPairs("logType", logType));
localVarQueryParameterBaseName = "limit";
localVarQueryParams.addAll(ApiClient.parameterToPairs("limit", limit));
localVarQueryParameterBaseName = "offset";
localVarQueryParams.addAll(ApiClient.parameterToPairs("offset", offset));
localVarQueryParameterBaseName = "latestTimeNano";
localVarQueryParams.addAll(ApiClient.parameterToPairs("latestTimeNano", latestTimeNano));
if (!localVarQueryParams.isEmpty() || localVarQueryStringJoiner.length() != 0) {
StringJoiner queryJoiner = new StringJoiner("&");
localVarQueryParams.forEach(p -> queryJoiner.add(p.getName() + '=' + p.getValue()));
if (localVarQueryStringJoiner.length() != 0) {
queryJoiner.add(localVarQueryStringJoiner.toString());
}
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath + '?' + queryJoiner.toString()));
} else {
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
}
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* retrieves a Model Product by id
*
* @param modelProductId (required)
* @return DominoNucleusModelproductModelsModelProduct
* @throws ApiException if fails to make API call
*/
public DominoNucleusModelproductModelsModelProduct getModelProduct(String modelProductId) throws ApiException {
ApiResponse localVarResponse = getModelProductWithHttpInfo(modelProductId);
return localVarResponse.getData();
}
/**
* retrieves a Model Product by id
*
* @param modelProductId (required)
* @return ApiResponse<DominoNucleusModelproductModelsModelProduct>
* @throws ApiException if fails to make API call
*/
public ApiResponse getModelProductWithHttpInfo(String modelProductId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getModelProductRequestBuilder(modelProductId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getModelProduct", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getModelProductRequestBuilder(String modelProductId) throws ApiException {
// verify the required parameter 'modelProductId' is set
if (modelProductId == null) {
throw new ApiException(400, "Missing the required parameter 'modelProductId' when calling getModelProduct");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/modelProducts/{modelProductId}"
.replace("{modelProductId}", ApiClient.urlEncode(modelProductId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Get the logs of an App Version without going to s3
*
* @param appVersionId (required)
* @param logType Types of logs: * `console` - This is the default if the value is not provided. All logs lines displayed in the app instance's runtime environment. * `stdout` - Log lines displayed in the stdout of the app instance's runtime environment. * `stderr` - Log lines displayed in the stderr of the app instance's runtime environment. * `stdoutstderr` - Interleaved stdout and stderr. * `prepareoutput` - Log lines generated by the environment preparing the app instance. (optional, default to console)
* @param limit Max number of log lines to fetch. Will be overridden by the configuration's limit if this value exceeds the configuration's limit, or if this value is not provided. (optional, default to 10000)
* @param offset The index of the current body of logs to start fetching from. 0 by default and typically won't be used for a timestamp-based offset log fetching strategy. (optional, default to 0)
* @param latestTimeNano The epoch time in nanoseconds to start fetching from, such as \"1543538813745986325\". \"0\" by default and will typically be used for a timestamp-based offset log fetching strategy. (optional, default to 0)
* @return DominoCommonModelproductLogSet
* @throws ApiException if fails to make API call
*/
public DominoCommonModelproductLogSet getRealTimeAppVersionLogs(String appVersionId, String logType, BigDecimal limit, BigDecimal offset, String latestTimeNano) throws ApiException {
ApiResponse localVarResponse = getRealTimeAppVersionLogsWithHttpInfo(appVersionId, logType, limit, offset, latestTimeNano);
return localVarResponse.getData();
}
/**
* Get the logs of an App Version without going to s3
*
* @param appVersionId (required)
* @param logType Types of logs: * `console` - This is the default if the value is not provided. All logs lines displayed in the app instance's runtime environment. * `stdout` - Log lines displayed in the stdout of the app instance's runtime environment. * `stderr` - Log lines displayed in the stderr of the app instance's runtime environment. * `stdoutstderr` - Interleaved stdout and stderr. * `prepareoutput` - Log lines generated by the environment preparing the app instance. (optional, default to console)
* @param limit Max number of log lines to fetch. Will be overridden by the configuration's limit if this value exceeds the configuration's limit, or if this value is not provided. (optional, default to 10000)
* @param offset The index of the current body of logs to start fetching from. 0 by default and typically won't be used for a timestamp-based offset log fetching strategy. (optional, default to 0)
* @param latestTimeNano The epoch time in nanoseconds to start fetching from, such as \"1543538813745986325\". \"0\" by default and will typically be used for a timestamp-based offset log fetching strategy. (optional, default to 0)
* @return ApiResponse<DominoCommonModelproductLogSet>
* @throws ApiException if fails to make API call
*/
public ApiResponse getRealTimeAppVersionLogsWithHttpInfo(String appVersionId, String logType, BigDecimal limit, BigDecimal offset, String latestTimeNano) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getRealTimeAppVersionLogsRequestBuilder(appVersionId, logType, limit, offset, latestTimeNano);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getRealTimeAppVersionLogs", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getRealTimeAppVersionLogsRequestBuilder(String appVersionId, String logType, BigDecimal limit, BigDecimal offset, String latestTimeNano) throws ApiException {
// verify the required parameter 'appVersionId' is set
if (appVersionId == null) {
throw new ApiException(400, "Missing the required parameter 'appVersionId' when calling getRealTimeAppVersionLogs");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/modelProducts/apps/versions/{appVersionId}/realTimeLogs"
.replace("{appVersionId}", ApiClient.urlEncode(appVersionId.toString()));
List localVarQueryParams = new ArrayList<>();
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
localVarQueryParameterBaseName = "logType";
localVarQueryParams.addAll(ApiClient.parameterToPairs("logType", logType));
localVarQueryParameterBaseName = "limit";
localVarQueryParams.addAll(ApiClient.parameterToPairs("limit", limit));
localVarQueryParameterBaseName = "offset";
localVarQueryParams.addAll(ApiClient.parameterToPairs("offset", offset));
localVarQueryParameterBaseName = "latestTimeNano";
localVarQueryParams.addAll(ApiClient.parameterToPairs("latestTimeNano", latestTimeNano));
if (!localVarQueryParams.isEmpty() || localVarQueryStringJoiner.length() != 0) {
StringJoiner queryJoiner = new StringJoiner("&");
localVarQueryParams.forEach(p -> queryJoiner.add(p.getName() + '=' + p.getValue()));
if (localVarQueryStringJoiner.length() != 0) {
queryJoiner.add(localVarQueryStringJoiner.toString());
}
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath + '?' + queryJoiner.toString()));
} else {
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
}
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* get count of views on one model product for a given time range
*
* @param modelProductId (required)
* @param startMillis (required)
* @param endMillis (required)
* @param granularityMillis (optional, default to 3600000)
* @return DominoNucleusModelproductModelsUsageStatisticsTimeseriesResponse
* @throws ApiException if fails to make API call
*/
public DominoNucleusModelproductModelsUsageStatisticsTimeseriesResponse getTimeseries(String modelProductId, Long startMillis, Long endMillis, Long granularityMillis) throws ApiException {
ApiResponse localVarResponse = getTimeseriesWithHttpInfo(modelProductId, startMillis, endMillis, granularityMillis);
return localVarResponse.getData();
}
/**
* get count of views on one model product for a given time range
*
* @param modelProductId (required)
* @param startMillis (required)
* @param endMillis (required)
* @param granularityMillis (optional, default to 3600000)
* @return ApiResponse<DominoNucleusModelproductModelsUsageStatisticsTimeseriesResponse>
* @throws ApiException if fails to make API call
*/
public ApiResponse getTimeseriesWithHttpInfo(String modelProductId, Long startMillis, Long endMillis, Long granularityMillis) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getTimeseriesRequestBuilder(modelProductId, startMillis, endMillis, granularityMillis);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getTimeseries", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getTimeseriesRequestBuilder(String modelProductId, Long startMillis, Long endMillis, Long granularityMillis) throws ApiException {
// verify the required parameter 'modelProductId' is set
if (modelProductId == null) {
throw new ApiException(400, "Missing the required parameter 'modelProductId' when calling getTimeseries");
}
// verify the required parameter 'startMillis' is set
if (startMillis == null) {
throw new ApiException(400, "Missing the required parameter 'startMillis' when calling getTimeseries");
}
// verify the required parameter 'endMillis' is set
if (endMillis == null) {
throw new ApiException(400, "Missing the required parameter 'endMillis' when calling getTimeseries");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/modelProducts/{modelProductId}/timeseries"
.replace("{modelProductId}", ApiClient.urlEncode(modelProductId.toString()));
List localVarQueryParams = new ArrayList<>();
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
localVarQueryParameterBaseName = "startMillis";
localVarQueryParams.addAll(ApiClient.parameterToPairs("startMillis", startMillis));
localVarQueryParameterBaseName = "endMillis";
localVarQueryParams.addAll(ApiClient.parameterToPairs("endMillis", endMillis));
localVarQueryParameterBaseName = "granularityMillis";
localVarQueryParams.addAll(ApiClient.parameterToPairs("granularityMillis", granularityMillis));
if (!localVarQueryParams.isEmpty() || localVarQueryStringJoiner.length() != 0) {
StringJoiner queryJoiner = new StringJoiner("&");
localVarQueryParams.forEach(p -> queryJoiner.add(p.getName() + '=' + p.getValue()));
if (localVarQueryStringJoiner.length() != 0) {
queryJoiner.add(localVarQueryStringJoiner.toString());
}
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath + '?' + queryJoiner.toString()));
} else {
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
}
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* list top model product views for a given time range
*
* @param startMillis (required)
* @param endMillis (required)
* @param count (optional, default to 20)
* @return DominoNucleusModelproductModelsUsageStatisticsTopNResponse
* @throws ApiException if fails to make API call
*/
public DominoNucleusModelproductModelsUsageStatisticsTopNResponse getTopN(Long startMillis, Long endMillis, Integer count) throws ApiException {
ApiResponse localVarResponse = getTopNWithHttpInfo(startMillis, endMillis, count);
return localVarResponse.getData();
}
/**
* list top model product views for a given time range
*
* @param startMillis (required)
* @param endMillis (required)
* @param count (optional, default to 20)
* @return ApiResponse<DominoNucleusModelproductModelsUsageStatisticsTopNResponse>
* @throws ApiException if fails to make API call
*/
public ApiResponse getTopNWithHttpInfo(Long startMillis, Long endMillis, Integer count) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getTopNRequestBuilder(startMillis, endMillis, count);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getTopN", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getTopNRequestBuilder(Long startMillis, Long endMillis, Integer count) throws ApiException {
// verify the required parameter 'startMillis' is set
if (startMillis == null) {
throw new ApiException(400, "Missing the required parameter 'startMillis' when calling getTopN");
}
// verify the required parameter 'endMillis' is set
if (endMillis == null) {
throw new ApiException(400, "Missing the required parameter 'endMillis' when calling getTopN");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/modelProducts/top";
List localVarQueryParams = new ArrayList<>();
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
localVarQueryParameterBaseName = "startMillis";
localVarQueryParams.addAll(ApiClient.parameterToPairs("startMillis", startMillis));
localVarQueryParameterBaseName = "endMillis";
localVarQueryParams.addAll(ApiClient.parameterToPairs("endMillis", endMillis));
localVarQueryParameterBaseName = "count";
localVarQueryParams.addAll(ApiClient.parameterToPairs("count", count));
if (!localVarQueryParams.isEmpty() || localVarQueryStringJoiner.length() != 0) {
StringJoiner queryJoiner = new StringJoiner("&");
localVarQueryParams.forEach(p -> queryJoiner.add(p.getName() + '=' + p.getValue()));
if (localVarQueryStringJoiner.length() != 0) {
queryJoiner.add(localVarQueryStringJoiner.toString());
}
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath + '?' + queryJoiner.toString()));
} else {
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
}
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* get count of views on one model product for a given time range
*
* @param modelProductId (required)
* @param startMillis (required)
* @param endMillis (required)
* @return DominoNucleusModelproductModelsUsageStatisticsTotalResponse
* @throws ApiException if fails to make API call
*/
public DominoNucleusModelproductModelsUsageStatisticsTotalResponse getTotal(String modelProductId, Long startMillis, Long endMillis) throws ApiException {
ApiResponse localVarResponse = getTotalWithHttpInfo(modelProductId, startMillis, endMillis);
return localVarResponse.getData();
}
/**
* get count of views on one model product for a given time range
*
* @param modelProductId (required)
* @param startMillis (required)
* @param endMillis (required)
* @return ApiResponse<DominoNucleusModelproductModelsUsageStatisticsTotalResponse>
* @throws ApiException if fails to make API call
*/
public ApiResponse getTotalWithHttpInfo(String modelProductId, Long startMillis, Long endMillis) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getTotalRequestBuilder(modelProductId, startMillis, endMillis);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getTotal", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getTotalRequestBuilder(String modelProductId, Long startMillis, Long endMillis) throws ApiException {
// verify the required parameter 'modelProductId' is set
if (modelProductId == null) {
throw new ApiException(400, "Missing the required parameter 'modelProductId' when calling getTotal");
}
// verify the required parameter 'startMillis' is set
if (startMillis == null) {
throw new ApiException(400, "Missing the required parameter 'startMillis' when calling getTotal");
}
// verify the required parameter 'endMillis' is set
if (endMillis == null) {
throw new ApiException(400, "Missing the required parameter 'endMillis' when calling getTotal");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/modelProducts/{modelProductId}/totals"
.replace("{modelProductId}", ApiClient.urlEncode(modelProductId.toString()));
List localVarQueryParams = new ArrayList<>();
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
localVarQueryParameterBaseName = "startMillis";
localVarQueryParams.addAll(ApiClient.parameterToPairs("startMillis", startMillis));
localVarQueryParameterBaseName = "endMillis";
localVarQueryParams.addAll(ApiClient.parameterToPairs("endMillis", endMillis));
if (!localVarQueryParams.isEmpty() || localVarQueryStringJoiner.length() != 0) {
StringJoiner queryJoiner = new StringJoiner("&");
localVarQueryParams.forEach(p -> queryJoiner.add(p.getName() + '=' + p.getValue()));
if (localVarQueryStringJoiner.length() != 0) {
queryJoiner.add(localVarQueryStringJoiner.toString());
}
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath + '?' + queryJoiner.toString()));
} else {
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
}
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* grants access to a ModelProduct to a user
*
* @param modelProductId (required)
* @param dominoNucleusModelproductModelsAccessRequest (required)
* @return String
* @throws ApiException if fails to make API call
*/
public String grantAccess(String modelProductId, DominoNucleusModelproductModelsAccessRequest dominoNucleusModelproductModelsAccessRequest) throws ApiException {
ApiResponse localVarResponse = grantAccessWithHttpInfo(modelProductId, dominoNucleusModelproductModelsAccessRequest);
return localVarResponse.getData();
}
/**
* grants access to a ModelProduct to a user
*
* @param modelProductId (required)
* @param dominoNucleusModelproductModelsAccessRequest (required)
* @return ApiResponse<String>
* @throws ApiException if fails to make API call
*/
public ApiResponse grantAccessWithHttpInfo(String modelProductId, DominoNucleusModelproductModelsAccessRequest dominoNucleusModelproductModelsAccessRequest) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = grantAccessRequestBuilder(modelProductId, dominoNucleusModelproductModelsAccessRequest);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("grantAccess", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder grantAccessRequestBuilder(String modelProductId, DominoNucleusModelproductModelsAccessRequest dominoNucleusModelproductModelsAccessRequest) throws ApiException {
// verify the required parameter 'modelProductId' is set
if (modelProductId == null) {
throw new ApiException(400, "Missing the required parameter 'modelProductId' when calling grantAccess");
}
// verify the required parameter 'dominoNucleusModelproductModelsAccessRequest' is set
if (dominoNucleusModelproductModelsAccessRequest == null) {
throw new ApiException(400, "Missing the required parameter 'dominoNucleusModelproductModelsAccessRequest' when calling grantAccess");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/modelProducts/{modelProductId}/grantAccess"
.replace("{modelProductId}", ApiClient.urlEncode(modelProductId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(dominoNucleusModelproductModelsAccessRequest);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* retrieves whether this project can be run as the model product type
*
* @param projectId (required)
* @param type (required)
* @return DominoNucleusModelproductModelsIsRunnable
* @throws ApiException if fails to make API call
*/
public DominoNucleusModelproductModelsIsRunnable isRunnable(String projectId, String type) throws ApiException {
ApiResponse localVarResponse = isRunnableWithHttpInfo(projectId, type);
return localVarResponse.getData();
}
/**
* retrieves whether this project can be run as the model product type
*
* @param projectId (required)
* @param type (required)
* @return ApiResponse<DominoNucleusModelproductModelsIsRunnable>
* @throws ApiException if fails to make API call
*/
public ApiResponse isRunnableWithHttpInfo(String projectId, String type) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = isRunnableRequestBuilder(projectId, type);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("isRunnable", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder isRunnableRequestBuilder(String projectId, String type) throws ApiException {
// verify the required parameter 'projectId' is set
if (projectId == null) {
throw new ApiException(400, "Missing the required parameter 'projectId' when calling isRunnable");
}
// verify the required parameter 'type' is set
if (type == null) {
throw new ApiException(400, "Missing the required parameter 'type' when calling isRunnable");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/modelProducts/isRunnable";
List localVarQueryParams = new ArrayList<>();
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
localVarQueryParameterBaseName = "projectId";
localVarQueryParams.addAll(ApiClient.parameterToPairs("projectId", projectId));
localVarQueryParameterBaseName = "type";
localVarQueryParams.addAll(ApiClient.parameterToPairs("type", type));
if (!localVarQueryParams.isEmpty() || localVarQueryStringJoiner.length() != 0) {
StringJoiner queryJoiner = new StringJoiner("&");
localVarQueryParams.forEach(p -> queryJoiner.add(p.getName() + '=' + p.getValue()));
if (localVarQueryStringJoiner.length() != 0) {
queryJoiner.add(localVarQueryStringJoiner.toString());
}
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath + '?' + queryJoiner.toString()));
} else {
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
}
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Link a app to a goal
*
* @param dominoNucleusModelproductModelsLinkAppToGoal JSON object with information to link app to goal (required)
* @return DominoCommonModelproductAppGoal
* @throws ApiException if fails to make API call
*/
public DominoCommonModelproductAppGoal linkAppToGoal(DominoNucleusModelproductModelsLinkAppToGoal dominoNucleusModelproductModelsLinkAppToGoal) throws ApiException {
ApiResponse localVarResponse = linkAppToGoalWithHttpInfo(dominoNucleusModelproductModelsLinkAppToGoal);
return localVarResponse.getData();
}
/**
* Link a app to a goal
*
* @param dominoNucleusModelproductModelsLinkAppToGoal JSON object with information to link app to goal (required)
* @return ApiResponse<DominoCommonModelproductAppGoal>
* @throws ApiException if fails to make API call
*/
public ApiResponse linkAppToGoalWithHttpInfo(DominoNucleusModelproductModelsLinkAppToGoal dominoNucleusModelproductModelsLinkAppToGoal) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = linkAppToGoalRequestBuilder(dominoNucleusModelproductModelsLinkAppToGoal);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("linkAppToGoal", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder linkAppToGoalRequestBuilder(DominoNucleusModelproductModelsLinkAppToGoal dominoNucleusModelproductModelsLinkAppToGoal) throws ApiException {
// verify the required parameter 'dominoNucleusModelproductModelsLinkAppToGoal' is set
if (dominoNucleusModelproductModelsLinkAppToGoal == null) {
throw new ApiException(400, "Missing the required parameter 'dominoNucleusModelproductModelsLinkAppToGoal' when calling linkAppToGoal");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/modelProducts/linkToGoal";
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(dominoNucleusModelproductModelsLinkAppToGoal);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* retrieves Model Products the current consumer can access
*
* @param type the type of model product (optional)
* @return List<DominoNucleusModelproductModelsConsumerModelProduct>
* @throws ApiException if fails to make API call
*/
public List listModelProducts(String type) throws ApiException {
ApiResponse> localVarResponse = listModelProductsWithHttpInfo(type);
return localVarResponse.getData();
}
/**
* retrieves Model Products the current consumer can access
*
* @param type the type of model product (optional)
* @return ApiResponse<List<DominoNucleusModelproductModelsConsumerModelProduct>>
* @throws ApiException if fails to make API call
*/
public ApiResponse> listModelProductsWithHttpInfo(String type) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = listModelProductsRequestBuilder(type);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("listModelProducts", localVarResponse);
}
return new ApiResponse>(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference>() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder listModelProductsRequestBuilder(String type) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/modelProducts/consumer";
List localVarQueryParams = new ArrayList<>();
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
localVarQueryParameterBaseName = "type";
localVarQueryParams.addAll(ApiClient.parameterToPairs("type", type));
if (!localVarQueryParams.isEmpty() || localVarQueryStringJoiner.length() != 0) {
StringJoiner queryJoiner = new StringJoiner("&");
localVarQueryParams.forEach(p -> queryJoiner.add(p.getName() + '=' + p.getValue()));
if (localVarQueryStringJoiner.length() != 0) {
queryJoiner.add(localVarQueryStringJoiner.toString());
}
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath + '?' + queryJoiner.toString()));
} else {
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
}
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Retrieves Model Products the current publisher can access
*
* @param projectId (optional)
* @param type (optional)
* @return List<DominoNucleusModelproductModelsModelProduct>
* @throws ApiException if fails to make API call
*/
public List listProjectModelProducts(String projectId, String type) throws ApiException {
ApiResponse> localVarResponse = listProjectModelProductsWithHttpInfo(projectId, type);
return localVarResponse.getData();
}
/**
* Retrieves Model Products the current publisher can access
*
* @param projectId (optional)
* @param type (optional)
* @return ApiResponse<List<DominoNucleusModelproductModelsModelProduct>>
* @throws ApiException if fails to make API call
*/
public ApiResponse> listProjectModelProductsWithHttpInfo(String projectId, String type) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = listProjectModelProductsRequestBuilder(projectId, type);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("listProjectModelProducts", localVarResponse);
}
return new ApiResponse>(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference>() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder listProjectModelProductsRequestBuilder(String projectId, String type) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/modelProducts";
List localVarQueryParams = new ArrayList<>();
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
localVarQueryParameterBaseName = "projectId";
localVarQueryParams.addAll(ApiClient.parameterToPairs("projectId", projectId));
localVarQueryParameterBaseName = "type";
localVarQueryParams.addAll(ApiClient.parameterToPairs("type", type));
if (!localVarQueryParams.isEmpty() || localVarQueryStringJoiner.length() != 0) {
StringJoiner queryJoiner = new StringJoiner("&");
localVarQueryParams.forEach(p -> queryJoiner.add(p.getName() + '=' + p.getValue()));
if (localVarQueryStringJoiner.length() != 0) {
queryJoiner.add(localVarQueryStringJoiner.toString());
}
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath + '?' + queryJoiner.toString()));
} else {
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
}
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* record a view on a model product
*
* @param runId (required)
* @return DominoNucleusModelproductModelsUsageStatisticsRecorded
* @throws ApiException if fails to make API call
*/
public DominoNucleusModelproductModelsUsageStatisticsRecorded record(String runId) throws ApiException {
ApiResponse localVarResponse = recordWithHttpInfo(runId);
return localVarResponse.getData();
}
/**
* record a view on a model product
*
* @param runId (required)
* @return ApiResponse<DominoNucleusModelproductModelsUsageStatisticsRecorded>
* @throws ApiException if fails to make API call
*/
public ApiResponse recordWithHttpInfo(String runId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = recordRequestBuilder(runId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("record", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder recordRequestBuilder(String runId) throws ApiException {
// verify the required parameter 'runId' is set
if (runId == null) {
throw new ApiException(400, "Missing the required parameter 'runId' when calling record");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/modelProducts/{runId}/view"
.replace("{runId}", ApiClient.urlEncode(runId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* requests access to a ModelProduct
*
* @param modelProductId (required)
* @return String
* @throws ApiException if fails to make API call
*/
public String requestAccess(String modelProductId) throws ApiException {
ApiResponse localVarResponse = requestAccessWithHttpInfo(modelProductId);
return localVarResponse.getData();
}
/**
* requests access to a ModelProduct
*
* @param modelProductId (required)
* @return ApiResponse<String>
* @throws ApiException if fails to make API call
*/
public ApiResponse requestAccessWithHttpInfo(String modelProductId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = requestAccessRequestBuilder(modelProductId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("requestAccess", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder requestAccessRequestBuilder(String modelProductId) throws ApiException {
// verify the required parameter 'modelProductId' is set
if (modelProductId == null) {
throw new ApiException(400, "Missing the required parameter 'modelProductId' when calling requestAccess");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/modelProducts/{modelProductId}/requestAccess"
.replace("{modelProductId}", ApiClient.urlEncode(modelProductId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* restarts all active apps
*
* @throws ApiException if fails to make API call
*/
public void restartAll() throws ApiException {
restartAllWithHttpInfo();
}
/**
* restarts all active apps
*
* @return ApiResponse<Void>
* @throws ApiException if fails to make API call
*/
public ApiResponse restartAllWithHttpInfo() throws ApiException {
HttpRequest.Builder localVarRequestBuilder = restartAllRequestBuilder();
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("restartAll", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
null
);
} finally {
// Drain the InputStream
while (localVarResponse.body().read() != -1) {
// Ignore
}
localVarResponse.body().close();
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder restartAllRequestBuilder() throws ApiException {
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/modelProducts/restartAll";
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* starts a runnable ModelProduct
*
* @param modelProductId (required)
* @param dominoNucleusModelproductModelsStartParams (required)
* @return String
* @throws ApiException if fails to make API call
*/
public String startModelProduct(String modelProductId, DominoNucleusModelproductModelsStartParams dominoNucleusModelproductModelsStartParams) throws ApiException {
ApiResponse localVarResponse = startModelProductWithHttpInfo(modelProductId, dominoNucleusModelproductModelsStartParams);
return localVarResponse.getData();
}
/**
* starts a runnable ModelProduct
*
* @param modelProductId (required)
* @param dominoNucleusModelproductModelsStartParams (required)
* @return ApiResponse<String>
* @throws ApiException if fails to make API call
*/
public ApiResponse startModelProductWithHttpInfo(String modelProductId, DominoNucleusModelproductModelsStartParams dominoNucleusModelproductModelsStartParams) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = startModelProductRequestBuilder(modelProductId, dominoNucleusModelproductModelsStartParams);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("startModelProduct", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder startModelProductRequestBuilder(String modelProductId, DominoNucleusModelproductModelsStartParams dominoNucleusModelproductModelsStartParams) throws ApiException {
// verify the required parameter 'modelProductId' is set
if (modelProductId == null) {
throw new ApiException(400, "Missing the required parameter 'modelProductId' when calling startModelProduct");
}
// verify the required parameter 'dominoNucleusModelproductModelsStartParams' is set
if (dominoNucleusModelproductModelsStartParams == null) {
throw new ApiException(400, "Missing the required parameter 'dominoNucleusModelproductModelsStartParams' when calling startModelProduct");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/modelProducts/{modelProductId}/start"
.replace("{modelProductId}", ApiClient.urlEncode(modelProductId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(dominoNucleusModelproductModelsStartParams);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* stops a runnable ModelProduct
*
* @param modelProductId Domino id of the model product (app) (required)
* @param force Stop an app regardless of its status (optional)
* @return String
* @throws ApiException if fails to make API call
*/
public String stopModelProduct(String modelProductId, Boolean force) throws ApiException {
ApiResponse localVarResponse = stopModelProductWithHttpInfo(modelProductId, force);
return localVarResponse.getData();
}
/**
* stops a runnable ModelProduct
*
* @param modelProductId Domino id of the model product (app) (required)
* @param force Stop an app regardless of its status (optional)
* @return ApiResponse<String>
* @throws ApiException if fails to make API call
*/
public ApiResponse stopModelProductWithHttpInfo(String modelProductId, Boolean force) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = stopModelProductRequestBuilder(modelProductId, force);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("stopModelProduct", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder stopModelProductRequestBuilder(String modelProductId, Boolean force) throws ApiException {
// verify the required parameter 'modelProductId' is set
if (modelProductId == null) {
throw new ApiException(400, "Missing the required parameter 'modelProductId' when calling stopModelProduct");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/modelProducts/{modelProductId}/stop"
.replace("{modelProductId}", ApiClient.urlEncode(modelProductId.toString()));
List localVarQueryParams = new ArrayList<>();
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
localVarQueryParameterBaseName = "force";
localVarQueryParams.addAll(ApiClient.parameterToPairs("force", force));
if (!localVarQueryParams.isEmpty() || localVarQueryStringJoiner.length() != 0) {
StringJoiner queryJoiner = new StringJoiner("&");
localVarQueryParams.forEach(p -> queryJoiner.add(p.getName() + '=' + p.getValue()));
if (localVarQueryStringJoiner.length() != 0) {
queryJoiner.add(localVarQueryStringJoiner.toString());
}
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath + '?' + queryJoiner.toString()));
} else {
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
}
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* uninvite a user
*
* @param modelProductId (required)
* @param dominoNucleusModelproductModelsUninviteRequest (required)
* @return String
* @throws ApiException if fails to make API call
*/
public String uninvite(String modelProductId, DominoNucleusModelproductModelsUninviteRequest dominoNucleusModelproductModelsUninviteRequest) throws ApiException {
ApiResponse localVarResponse = uninviteWithHttpInfo(modelProductId, dominoNucleusModelproductModelsUninviteRequest);
return localVarResponse.getData();
}
/**
* uninvite a user
*
* @param modelProductId (required)
* @param dominoNucleusModelproductModelsUninviteRequest (required)
* @return ApiResponse<String>
* @throws ApiException if fails to make API call
*/
public ApiResponse uninviteWithHttpInfo(String modelProductId, DominoNucleusModelproductModelsUninviteRequest dominoNucleusModelproductModelsUninviteRequest) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = uninviteRequestBuilder(modelProductId, dominoNucleusModelproductModelsUninviteRequest);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("uninvite", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder uninviteRequestBuilder(String modelProductId, DominoNucleusModelproductModelsUninviteRequest dominoNucleusModelproductModelsUninviteRequest) throws ApiException {
// verify the required parameter 'modelProductId' is set
if (modelProductId == null) {
throw new ApiException(400, "Missing the required parameter 'modelProductId' when calling uninvite");
}
// verify the required parameter 'dominoNucleusModelproductModelsUninviteRequest' is set
if (dominoNucleusModelproductModelsUninviteRequest == null) {
throw new ApiException(400, "Missing the required parameter 'dominoNucleusModelproductModelsUninviteRequest' when calling uninvite");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/modelProducts/{modelProductId}/uninvite"
.replace("{modelProductId}", ApiClient.urlEncode(modelProductId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(dominoNucleusModelproductModelsUninviteRequest);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Unlink a app from a goal
*
* @param dominoNucleusModelproductModelsUnlinkAppFromGoal JSON object with information to unlink app from goal (required)
* @return DominoCommonModelproductAppGoal
* @throws ApiException if fails to make API call
*/
public DominoCommonModelproductAppGoal unlinkAppFromGoal(DominoNucleusModelproductModelsUnlinkAppFromGoal dominoNucleusModelproductModelsUnlinkAppFromGoal) throws ApiException {
ApiResponse localVarResponse = unlinkAppFromGoalWithHttpInfo(dominoNucleusModelproductModelsUnlinkAppFromGoal);
return localVarResponse.getData();
}
/**
* Unlink a app from a goal
*
* @param dominoNucleusModelproductModelsUnlinkAppFromGoal JSON object with information to unlink app from goal (required)
* @return ApiResponse<DominoCommonModelproductAppGoal>
* @throws ApiException if fails to make API call
*/
public ApiResponse unlinkAppFromGoalWithHttpInfo(DominoNucleusModelproductModelsUnlinkAppFromGoal dominoNucleusModelproductModelsUnlinkAppFromGoal) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = unlinkAppFromGoalRequestBuilder(dominoNucleusModelproductModelsUnlinkAppFromGoal);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("unlinkAppFromGoal", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder unlinkAppFromGoalRequestBuilder(DominoNucleusModelproductModelsUnlinkAppFromGoal dominoNucleusModelproductModelsUnlinkAppFromGoal) throws ApiException {
// verify the required parameter 'dominoNucleusModelproductModelsUnlinkAppFromGoal' is set
if (dominoNucleusModelproductModelsUnlinkAppFromGoal == null) {
throw new ApiException(400, "Missing the required parameter 'dominoNucleusModelproductModelsUnlinkAppFromGoal' when calling unlinkAppFromGoal");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/modelProducts/unlinkFromGoal";
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(dominoNucleusModelproductModelsUnlinkAppFromGoal);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* sets visibility for a ModelProduct
*
* @param modelProductId (required)
* @param dominoNucleusModelproductModelsVisibilityPatch (required)
* @return String
* @throws ApiException if fails to make API call
*/
public String updateVisibility(String modelProductId, DominoNucleusModelproductModelsVisibilityPatch dominoNucleusModelproductModelsVisibilityPatch) throws ApiException {
ApiResponse localVarResponse = updateVisibilityWithHttpInfo(modelProductId, dominoNucleusModelproductModelsVisibilityPatch);
return localVarResponse.getData();
}
/**
* sets visibility for a ModelProduct
*
* @param modelProductId (required)
* @param dominoNucleusModelproductModelsVisibilityPatch (required)
* @return ApiResponse<String>
* @throws ApiException if fails to make API call
*/
public ApiResponse updateVisibilityWithHttpInfo(String modelProductId, DominoNucleusModelproductModelsVisibilityPatch dominoNucleusModelproductModelsVisibilityPatch) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = updateVisibilityRequestBuilder(modelProductId, dominoNucleusModelproductModelsVisibilityPatch);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("updateVisibility", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder updateVisibilityRequestBuilder(String modelProductId, DominoNucleusModelproductModelsVisibilityPatch dominoNucleusModelproductModelsVisibilityPatch) throws ApiException {
// verify the required parameter 'modelProductId' is set
if (modelProductId == null) {
throw new ApiException(400, "Missing the required parameter 'modelProductId' when calling updateVisibility");
}
// verify the required parameter 'dominoNucleusModelproductModelsVisibilityPatch' is set
if (dominoNucleusModelproductModelsVisibilityPatch == null) {
throw new ApiException(400, "Missing the required parameter 'dominoNucleusModelproductModelsVisibilityPatch' when calling updateVisibility");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/modelProducts/{modelProductId}/visibility"
.replace("{modelProductId}", ApiClient.urlEncode(modelProductId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(dominoNucleusModelproductModelsVisibilityPatch);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy