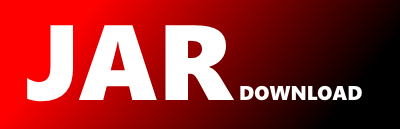
com.dominodatalab.api.rest.WorkspaceCommentsApi Maven / Gradle / Ivy
/*
* Domino Data Lab API v4
* This API is going to provide access to all the Domino functions available in the user interface. To authenticate your requests, include your API Key (which you can find on your account page) with the header X-Domino-Api-Key.
*
* The version of the OpenAPI document: 4.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.dominodatalab.api.rest;
import com.dominodatalab.api.invoker.ApiClient;
import com.dominodatalab.api.invoker.ApiException;
import com.dominodatalab.api.invoker.ApiResponse;
import com.dominodatalab.api.invoker.Pair;
import com.dominodatalab.api.model.DominoApiErrorResponse;
import com.dominodatalab.api.model.DominoWorkspacesApiComment;
import com.dominodatalab.api.model.DominoWorkspacesApiCommentThread;
import com.dominodatalab.api.model.DominoWorkspacesWebArchiveCommentInput;
import com.dominodatalab.api.model.DominoWorkspacesWebCreateCommentInput;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import org.apache.http.HttpEntity;
import org.apache.http.NameValuePair;
import org.apache.http.entity.mime.MultipartEntityBuilder;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import java.io.InputStream;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.IOException;
import java.io.OutputStream;
import java.net.http.HttpRequest;
import java.nio.channels.Channels;
import java.nio.channels.Pipe;
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.time.Duration;
import java.util.ArrayList;
import java.util.StringJoiner;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.function.Consumer;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2023-10-17T15:20:46.682098100-04:00[America/New_York]")
public class WorkspaceCommentsApi {
private final HttpClient memberVarHttpClient;
private final ObjectMapper memberVarObjectMapper;
private final String memberVarBaseUri;
private final Consumer memberVarInterceptor;
private final Duration memberVarReadTimeout;
private final Consumer> memberVarResponseInterceptor;
private final Consumer> memberVarAsyncResponseInterceptor;
public WorkspaceCommentsApi() {
this(new ApiClient());
}
public WorkspaceCommentsApi(ApiClient apiClient) {
memberVarHttpClient = apiClient.getHttpClient();
memberVarObjectMapper = apiClient.getObjectMapper();
memberVarBaseUri = apiClient.getBaseUri();
memberVarInterceptor = apiClient.getRequestInterceptor();
memberVarReadTimeout = apiClient.getReadTimeout();
memberVarResponseInterceptor = apiClient.getResponseInterceptor();
memberVarAsyncResponseInterceptor = apiClient.getAsyncResponseInterceptor();
}
protected ApiException getApiException(String operationId, HttpResponse response) throws IOException {
String body = response.body() == null ? null : new String(response.body().readAllBytes());
String message = formatExceptionMessage(operationId, response.statusCode(), body);
return new ApiException(response.statusCode(), message, response.headers(), body);
}
private String formatExceptionMessage(String operationId, int statusCode, String body) {
if (body == null || body.isEmpty()) {
body = "[no body]";
}
return operationId + " call failed with: " + statusCode + " - " + body;
}
/**
* Archive the comment in a comment thread
*
* @param workspaceId ID of the workspace (required)
* @param threadId (required)
* @param dominoWorkspacesWebArchiveCommentInput JSON object with information about the index of the comment to be archived (required)
* @return DominoWorkspacesApiComment
* @throws ApiException if fails to make API call
*/
public DominoWorkspacesApiComment archiveCommentFromThread(String workspaceId, String threadId, DominoWorkspacesWebArchiveCommentInput dominoWorkspacesWebArchiveCommentInput) throws ApiException {
ApiResponse localVarResponse = archiveCommentFromThreadWithHttpInfo(workspaceId, threadId, dominoWorkspacesWebArchiveCommentInput);
return localVarResponse.getData();
}
/**
* Archive the comment in a comment thread
*
* @param workspaceId ID of the workspace (required)
* @param threadId (required)
* @param dominoWorkspacesWebArchiveCommentInput JSON object with information about the index of the comment to be archived (required)
* @return ApiResponse<DominoWorkspacesApiComment>
* @throws ApiException if fails to make API call
*/
public ApiResponse archiveCommentFromThreadWithHttpInfo(String workspaceId, String threadId, DominoWorkspacesWebArchiveCommentInput dominoWorkspacesWebArchiveCommentInput) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = archiveCommentFromThreadRequestBuilder(workspaceId, threadId, dominoWorkspacesWebArchiveCommentInput);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("archiveCommentFromThread", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder archiveCommentFromThreadRequestBuilder(String workspaceId, String threadId, DominoWorkspacesWebArchiveCommentInput dominoWorkspacesWebArchiveCommentInput) throws ApiException {
// verify the required parameter 'workspaceId' is set
if (workspaceId == null) {
throw new ApiException(400, "Missing the required parameter 'workspaceId' when calling archiveCommentFromThread");
}
// verify the required parameter 'threadId' is set
if (threadId == null) {
throw new ApiException(400, "Missing the required parameter 'threadId' when calling archiveCommentFromThread");
}
// verify the required parameter 'dominoWorkspacesWebArchiveCommentInput' is set
if (dominoWorkspacesWebArchiveCommentInput == null) {
throw new ApiException(400, "Missing the required parameter 'dominoWorkspacesWebArchiveCommentInput' when calling archiveCommentFromThread");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/workspaces/{workspaceId}/comment/{threadId}"
.replace("{workspaceId}", ApiClient.urlEncode(workspaceId.toString()))
.replace("{threadId}", ApiClient.urlEncode(threadId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(dominoWorkspacesWebArchiveCommentInput);
localVarRequestBuilder.method("DELETE", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Create a Workspace Comment
*
* @param workspaceId ID of the workspace (required)
* @param dominoWorkspacesWebCreateCommentInput JSON object with information to comment on the workspace (required)
* @return DominoWorkspacesApiCommentThread
* @throws ApiException if fails to make API call
*/
public DominoWorkspacesApiCommentThread createWorkspaceComment(String workspaceId, DominoWorkspacesWebCreateCommentInput dominoWorkspacesWebCreateCommentInput) throws ApiException {
ApiResponse localVarResponse = createWorkspaceCommentWithHttpInfo(workspaceId, dominoWorkspacesWebCreateCommentInput);
return localVarResponse.getData();
}
/**
* Create a Workspace Comment
*
* @param workspaceId ID of the workspace (required)
* @param dominoWorkspacesWebCreateCommentInput JSON object with information to comment on the workspace (required)
* @return ApiResponse<DominoWorkspacesApiCommentThread>
* @throws ApiException if fails to make API call
*/
public ApiResponse createWorkspaceCommentWithHttpInfo(String workspaceId, DominoWorkspacesWebCreateCommentInput dominoWorkspacesWebCreateCommentInput) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = createWorkspaceCommentRequestBuilder(workspaceId, dominoWorkspacesWebCreateCommentInput);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("createWorkspaceComment", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder createWorkspaceCommentRequestBuilder(String workspaceId, DominoWorkspacesWebCreateCommentInput dominoWorkspacesWebCreateCommentInput) throws ApiException {
// verify the required parameter 'workspaceId' is set
if (workspaceId == null) {
throw new ApiException(400, "Missing the required parameter 'workspaceId' when calling createWorkspaceComment");
}
// verify the required parameter 'dominoWorkspacesWebCreateCommentInput' is set
if (dominoWorkspacesWebCreateCommentInput == null) {
throw new ApiException(400, "Missing the required parameter 'dominoWorkspacesWebCreateCommentInput' when calling createWorkspaceComment");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/workspaces/{workspaceId}/comment"
.replace("{workspaceId}", ApiClient.urlEncode(workspaceId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(dominoWorkspacesWebCreateCommentInput);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Create a Workspace Result File Comment
*
* @param workspaceId ID of the workspace (required)
* @param fileName Name of the result file to comment to (required)
* @param commitId git commit identifier to pick the result file (required)
* @param dominoWorkspacesWebCreateCommentInput JSON object with information to comment on a result file (required)
* @return DominoWorkspacesApiCommentThread
* @throws ApiException if fails to make API call
*/
public DominoWorkspacesApiCommentThread createWorkspaceResultFileComment(String workspaceId, String fileName, String commitId, DominoWorkspacesWebCreateCommentInput dominoWorkspacesWebCreateCommentInput) throws ApiException {
ApiResponse localVarResponse = createWorkspaceResultFileCommentWithHttpInfo(workspaceId, fileName, commitId, dominoWorkspacesWebCreateCommentInput);
return localVarResponse.getData();
}
/**
* Create a Workspace Result File Comment
*
* @param workspaceId ID of the workspace (required)
* @param fileName Name of the result file to comment to (required)
* @param commitId git commit identifier to pick the result file (required)
* @param dominoWorkspacesWebCreateCommentInput JSON object with information to comment on a result file (required)
* @return ApiResponse<DominoWorkspacesApiCommentThread>
* @throws ApiException if fails to make API call
*/
public ApiResponse createWorkspaceResultFileCommentWithHttpInfo(String workspaceId, String fileName, String commitId, DominoWorkspacesWebCreateCommentInput dominoWorkspacesWebCreateCommentInput) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = createWorkspaceResultFileCommentRequestBuilder(workspaceId, fileName, commitId, dominoWorkspacesWebCreateCommentInput);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("createWorkspaceResultFileComment", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder createWorkspaceResultFileCommentRequestBuilder(String workspaceId, String fileName, String commitId, DominoWorkspacesWebCreateCommentInput dominoWorkspacesWebCreateCommentInput) throws ApiException {
// verify the required parameter 'workspaceId' is set
if (workspaceId == null) {
throw new ApiException(400, "Missing the required parameter 'workspaceId' when calling createWorkspaceResultFileComment");
}
// verify the required parameter 'fileName' is set
if (fileName == null) {
throw new ApiException(400, "Missing the required parameter 'fileName' when calling createWorkspaceResultFileComment");
}
// verify the required parameter 'commitId' is set
if (commitId == null) {
throw new ApiException(400, "Missing the required parameter 'commitId' when calling createWorkspaceResultFileComment");
}
// verify the required parameter 'dominoWorkspacesWebCreateCommentInput' is set
if (dominoWorkspacesWebCreateCommentInput == null) {
throw new ApiException(400, "Missing the required parameter 'dominoWorkspacesWebCreateCommentInput' when calling createWorkspaceResultFileComment");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/workspaces/{workspaceId}/file/comment"
.replace("{workspaceId}", ApiClient.urlEncode(workspaceId.toString()));
List localVarQueryParams = new ArrayList<>();
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
localVarQueryParameterBaseName = "fileName";
localVarQueryParams.addAll(ApiClient.parameterToPairs("fileName", fileName));
localVarQueryParameterBaseName = "commitId";
localVarQueryParams.addAll(ApiClient.parameterToPairs("commitId", commitId));
if (!localVarQueryParams.isEmpty() || localVarQueryStringJoiner.length() != 0) {
StringJoiner queryJoiner = new StringJoiner("&");
localVarQueryParams.forEach(p -> queryJoiner.add(p.getName() + '=' + p.getValue()));
if (localVarQueryStringJoiner.length() != 0) {
queryJoiner.add(localVarQueryStringJoiner.toString());
}
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath + '?' + queryJoiner.toString()));
} else {
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
}
localVarRequestBuilder.header("Content-Type", "application/json");
localVarRequestBuilder.header("Accept", "application/json");
try {
byte[] localVarPostBody = memberVarObjectMapper.writeValueAsBytes(dominoWorkspacesWebCreateCommentInput);
localVarRequestBuilder.method("POST", HttpRequest.BodyPublishers.ofByteArray(localVarPostBody));
} catch (IOException e) {
throw new ApiException(e);
}
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Get all aggregated comments
*
* @param workspaceId ID of the workspace (required)
* @return List<DominoWorkspacesApiCommentThread>
* @throws ApiException if fails to make API call
*/
public List getAllWorkspaceComments(String workspaceId) throws ApiException {
ApiResponse> localVarResponse = getAllWorkspaceCommentsWithHttpInfo(workspaceId);
return localVarResponse.getData();
}
/**
* Get all aggregated comments
*
* @param workspaceId ID of the workspace (required)
* @return ApiResponse<List<DominoWorkspacesApiCommentThread>>
* @throws ApiException if fails to make API call
*/
public ApiResponse> getAllWorkspaceCommentsWithHttpInfo(String workspaceId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getAllWorkspaceCommentsRequestBuilder(workspaceId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getAllWorkspaceComments", localVarResponse);
}
return new ApiResponse>(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference>() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getAllWorkspaceCommentsRequestBuilder(String workspaceId) throws ApiException {
// verify the required parameter 'workspaceId' is set
if (workspaceId == null) {
throw new ApiException(400, "Missing the required parameter 'workspaceId' when calling getAllWorkspaceComments");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/workspaces/{workspaceId}/allComments"
.replace("{workspaceId}", ApiClient.urlEncode(workspaceId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Get all comments specific to workspace context
*
* @param workspaceId ID of the workspace (required)
* @return DominoWorkspacesApiCommentThread
* @throws ApiException if fails to make API call
*/
public DominoWorkspacesApiCommentThread getWorkspaceComments(String workspaceId) throws ApiException {
ApiResponse localVarResponse = getWorkspaceCommentsWithHttpInfo(workspaceId);
return localVarResponse.getData();
}
/**
* Get all comments specific to workspace context
*
* @param workspaceId ID of the workspace (required)
* @return ApiResponse<DominoWorkspacesApiCommentThread>
* @throws ApiException if fails to make API call
*/
public ApiResponse getWorkspaceCommentsWithHttpInfo(String workspaceId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getWorkspaceCommentsRequestBuilder(workspaceId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getWorkspaceComments", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getWorkspaceCommentsRequestBuilder(String workspaceId) throws ApiException {
// verify the required parameter 'workspaceId' is set
if (workspaceId == null) {
throw new ApiException(400, "Missing the required parameter 'workspaceId' when calling getWorkspaceComments");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/workspaces/{workspaceId}/comments"
.replace("{workspaceId}", ApiClient.urlEncode(workspaceId.toString()));
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
/**
* Get all comments specific to result files generated by running the workspace
*
* @param workspaceId ID of the workspace (required)
* @param fileName Name of the result file (required)
* @param commitId git commit identifier to pick the result file (required)
* @return DominoWorkspacesApiCommentThread
* @throws ApiException if fails to make API call
*/
public DominoWorkspacesApiCommentThread getWorkspaceResultFileComments(String workspaceId, String fileName, String commitId) throws ApiException {
ApiResponse localVarResponse = getWorkspaceResultFileCommentsWithHttpInfo(workspaceId, fileName, commitId);
return localVarResponse.getData();
}
/**
* Get all comments specific to result files generated by running the workspace
*
* @param workspaceId ID of the workspace (required)
* @param fileName Name of the result file (required)
* @param commitId git commit identifier to pick the result file (required)
* @return ApiResponse<DominoWorkspacesApiCommentThread>
* @throws ApiException if fails to make API call
*/
public ApiResponse getWorkspaceResultFileCommentsWithHttpInfo(String workspaceId, String fileName, String commitId) throws ApiException {
HttpRequest.Builder localVarRequestBuilder = getWorkspaceResultFileCommentsRequestBuilder(workspaceId, fileName, commitId);
try {
HttpResponse localVarResponse = memberVarHttpClient.send(
localVarRequestBuilder.build(),
HttpResponse.BodyHandlers.ofInputStream());
if (memberVarResponseInterceptor != null) {
memberVarResponseInterceptor.accept(localVarResponse);
}
try {
if (localVarResponse.statusCode()/ 100 != 2) {
throw getApiException("getWorkspaceResultFileComments", localVarResponse);
}
return new ApiResponse(
localVarResponse.statusCode(),
localVarResponse.headers().map(),
localVarResponse.body() == null ? null : memberVarObjectMapper.readValue(localVarResponse.body(), new TypeReference() {}) // closes the InputStream
);
} finally {
}
} catch (IOException e) {
throw new ApiException(e);
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new ApiException(e);
}
}
private HttpRequest.Builder getWorkspaceResultFileCommentsRequestBuilder(String workspaceId, String fileName, String commitId) throws ApiException {
// verify the required parameter 'workspaceId' is set
if (workspaceId == null) {
throw new ApiException(400, "Missing the required parameter 'workspaceId' when calling getWorkspaceResultFileComments");
}
// verify the required parameter 'fileName' is set
if (fileName == null) {
throw new ApiException(400, "Missing the required parameter 'fileName' when calling getWorkspaceResultFileComments");
}
// verify the required parameter 'commitId' is set
if (commitId == null) {
throw new ApiException(400, "Missing the required parameter 'commitId' when calling getWorkspaceResultFileComments");
}
HttpRequest.Builder localVarRequestBuilder = HttpRequest.newBuilder();
String localVarPath = "/workspaces/{workspaceId}/resultFile/comments"
.replace("{workspaceId}", ApiClient.urlEncode(workspaceId.toString()));
List localVarQueryParams = new ArrayList<>();
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
localVarQueryParameterBaseName = "fileName";
localVarQueryParams.addAll(ApiClient.parameterToPairs("fileName", fileName));
localVarQueryParameterBaseName = "commitId";
localVarQueryParams.addAll(ApiClient.parameterToPairs("commitId", commitId));
if (!localVarQueryParams.isEmpty() || localVarQueryStringJoiner.length() != 0) {
StringJoiner queryJoiner = new StringJoiner("&");
localVarQueryParams.forEach(p -> queryJoiner.add(p.getName() + '=' + p.getValue()));
if (localVarQueryStringJoiner.length() != 0) {
queryJoiner.add(localVarQueryStringJoiner.toString());
}
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath + '?' + queryJoiner.toString()));
} else {
localVarRequestBuilder.uri(URI.create(memberVarBaseUri + localVarPath));
}
localVarRequestBuilder.header("Accept", "application/json");
localVarRequestBuilder.method("GET", HttpRequest.BodyPublishers.noBody());
if (memberVarReadTimeout != null) {
localVarRequestBuilder.timeout(memberVarReadTimeout);
}
if (memberVarInterceptor != null) {
memberVarInterceptor.accept(localVarRequestBuilder);
}
return localVarRequestBuilder;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy