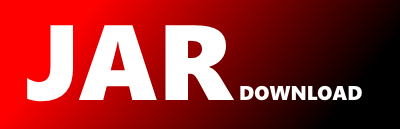
org.apache.iceberg.ksyun.ks3.Ks3LockManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iceberg-ksyun Show documentation
Show all versions of iceberg-ksyun Show documentation
apache iceberg support ks3
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy