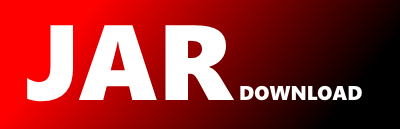
com.ksyun.pnp.sdk.domain.request.axb.AxbBindReq Maven / Gradle / Ivy
package com.ksyun.pnp.sdk.domain.request.axb;
import com.fasterxml.jackson.annotation.JsonProperty;
import org.apache.commons.lang3.StringUtils;
public class AxbBindReq {
/**
* 金山云控制台分配的号码池key
*/
@JsonProperty("PoolKey")
private String poolKey;
/**
* AXB中的A号码,目前仅支持手机号码
*/
@JsonProperty("PhoneNoA")
private String phoneNoA;
/**
* AXB中的B号码,A号码拨打X号码时会转接到B号码。
* B号码目前仅支持手机号码。
*/
@JsonProperty("PhoneNoB")
private String phoneNoB;
/**
* AXB中的X号码。用于转接电话。如果未指定X号码,
* 将根据参数ExpectCity从指定号码池中随机指定一个号码作为X号码
*/
@JsonProperty("PhoneNoX")
private String phoneNoX;
/**
* 过期时间, 日期格式 'yyyy-MM-dd HH:mm:ss'
* eg '2021-11-22 13:41:00'
*/
@JsonProperty("Expiration")
private String expiration;
/**
* 支持城市,具体支持的城市,与客户购买的号码资源有关系, eg. 北京
*/
@JsonProperty("ExpectCity")
private String expectCity;
/**
* 是否需要针对该绑定关系产生的所有通话录制通话录音
* true: 录音
* false: 不录音
*/
@JsonProperty("IsRecordingEnabled")
private boolean recordingEnabled;
public AxbBindReq(String poolKey, String phoneNoA, String phoneNoB, String expiration) {
this.poolKey = poolKey;
this.phoneNoA = phoneNoA;
this.phoneNoB = phoneNoB;
this.expiration = expiration;
}
public String getPoolKey() {
return poolKey;
}
public AxbBindReq setPoolKey(String poolKey) {
this.poolKey = poolKey == null ? StringUtils.EMPTY : poolKey.trim();
return this;
}
public String getPhoneNoA() {
return phoneNoA;
}
public AxbBindReq setPhoneNoA(String phoneNoA) {
this.phoneNoA = phoneNoA == null ? StringUtils.EMPTY : phoneNoA.trim();
return this;
}
public String getPhoneNoB() {
return phoneNoB;
}
public AxbBindReq setPhoneNoB(String phoneNoB) {
this.phoneNoB = phoneNoB == null ? StringUtils.EMPTY : phoneNoB.trim();
return this;
}
public String getPhoneNoX() {
return phoneNoX;
}
public AxbBindReq setPhoneNoX(String phoneNoX) {
this.phoneNoX = phoneNoX == null ? StringUtils.EMPTY : phoneNoX.trim();
return this;
}
public String getExpiration() {
return expiration;
}
public AxbBindReq setExpiration(String expiration) {
this.expiration = expiration == null ? StringUtils.EMPTY : expiration.trim();
return this;
}
public String getExpectCity() {
return expectCity;
}
public AxbBindReq setExpectCity(String expectCity) {
this.expectCity = expectCity == null ? StringUtils.EMPTY : expectCity.trim();
return this;
}
public boolean isRecordingEnabled() {
return recordingEnabled;
}
public AxbBindReq setRecordingEnabled(boolean recordingEnabled) {
this.recordingEnabled = recordingEnabled;
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy