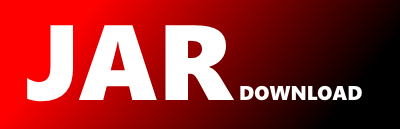
com.ksyun.pnp.sdk.util.http.SimpleHttpResponse Maven / Gradle / Ivy
package com.ksyun.pnp.sdk.util.http;
import com.fasterxml.jackson.core.type.TypeReference;
import com.ksyun.pnp.sdk.domain.response.OpenApiResponseResult;
import com.ksyun.pnp.sdk.util.sign.SignatureUtil;
import java.net.ProtocolException;
import java.util.List;
import java.util.Map;
/**
* httpResponse
*
* @author chenht
* @since 16/12/30
*/
public class SimpleHttpResponse {
private int statusCode;
private String body;
private Exception exception;
private Map> headers;
private SimpleHttpResponse(int statusCode, String body, Map> headers) {
this.statusCode = statusCode;
this.body = body;
this.exception = null;
this.headers = headers;
}
private SimpleHttpResponse(int statusCode, String body, Map> headers, Exception e) {
this.statusCode = statusCode;
this.body = body;
this.exception = e;
this.headers = headers;
}
static SimpleHttpResponse Error(Exception e) {
return new SimpleHttpResponse(500, null, null, e);
}
static SimpleHttpResponse newHttpResponse(okhttp3.Response theResponse) {
try {
if (theResponse.isSuccessful()) {
return new SimpleHttpResponse(theResponse.code(), theResponse.body().string(), theResponse.headers().toMultimap());
} else {
return new SimpleHttpResponse(theResponse.code(), theResponse.body().string(), theResponse.headers().toMultimap(),
new ProtocolException("Http StatusCode error: " + theResponse.code() + " (" + theResponse.message() + ")"));
}
} catch (Exception e) {
return Error(e);
} finally {
theResponse.close();
}
}
public Map> getHeaders() {
return headers;
}
public int getStatusCode() {
return statusCode;
}
public String getBody() {
return body;
}
public Exception getException() {
return exception;
}
public boolean isSuccess() {
return exception == null && getStatusCode() < 300;
}
@Override
public String toString() {
return "SimpleHttpResponse{" +
"statusCode=" + statusCode +
", body='" + body + '\'' +
", exception=" + exception +
'}';
}
public OpenApiResponseResult toResponse(TypeReference> t) {
return SignatureUtil.fromJson(this.getBody(), t);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy