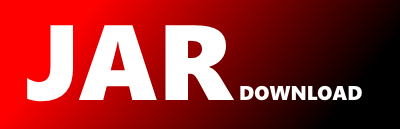
com.ksyun.ks3.service.request.ListObjectsV2Request Maven / Gradle / Ivy
package com.ksyun.ks3.service.request;
import com.ksyun.ks3.http.HttpMethod;
import com.ksyun.ks3.http.Request;
import com.ksyun.ks3.utils.StringUtils;
import static com.ksyun.ks3.exception.client.ClientIllegalArgumentExceptionGenerator.between;
import static com.ksyun.ks3.exception.client.ClientIllegalArgumentExceptionGenerator.notNull;
/**
* 9e71891758ca850feceaca3cbde4305f
* ListObjectsV2Request
*
* @author [email protected]
* @date 2022/6/1
*/
public class ListObjectsV2Request extends Ks3WebServiceRequest {
private String bucketName;
private String delimiter;
private String encodingType;
private Integer maxKeys;
private String prefix;
private String continuationToken;
private boolean fetchOwner;
private String startAfter;
public ListObjectsV2Request() {
}
public ListObjectsV2Request(String bucketName) {
this.bucketName = bucketName;
}
public ListObjectsV2Request(String bucketName, String prefix, String encodingType, Integer maxKeys, String delimiter, String continuationToken, boolean fetchOwner, String startAfter) {
this.bucketName = bucketName;
this.delimiter = delimiter;
this.encodingType = encodingType;
this.maxKeys = maxKeys;
this.prefix = prefix;
this.continuationToken = continuationToken;
this.fetchOwner = fetchOwner;
this.startAfter = startAfter;
}
public String getBucketName() {
return bucketName;
}
public void setBucketName(String bucketName) {
this.bucketName = bucketName;
}
public String getDelimiter() {
return delimiter;
}
public void setDelimiter(String delimiter) {
this.delimiter = delimiter;
}
public String getEncodingType() {
return encodingType;
}
public void setEncodingType(String encodingType) {
this.encodingType = encodingType;
}
public Integer getMaxKeys() {
return maxKeys;
}
public void setMaxKeys(Integer maxKeys) {
this.maxKeys = maxKeys;
}
public String getPrefix() {
return prefix;
}
public void setPrefix(String prefix) {
this.prefix = prefix;
}
public String getContinuationToken() {
return continuationToken;
}
public void setContinuationToken(String continuationToken) {
this.continuationToken = continuationToken;
}
public boolean isFetchOwner() {
return fetchOwner;
}
public void setFetchOwner(boolean fetchOwner) {
this.fetchOwner = fetchOwner;
}
public String getStartAfter() {
return startAfter;
}
public void setStartAfter(String startAfter) {
this.startAfter = startAfter;
}
@Override
public void buildRequest(Request request) {
request.setMethod(HttpMethod.GET);
request.setBucket(bucketName);
request.addQueryParam("list-type","2");
request.addQueryParamIfNotNull("prefix", prefix);
request.addQueryParamIfNotNull("continuation-token", continuationToken);
request.addQueryParamIfNotNull("fetch-owner", String.valueOf(fetchOwner));
request.addQueryParamIfNotNull("start-after", startAfter);
request.addQueryParamIfNotNull("delimiter", delimiter);
if(maxKeys!=null)
request.addQueryParamIfNotNull("max-keys", String.valueOf(maxKeys));
request.addQueryParamIfNotNull("encoding-type", this.encodingType);
}
@Override
public void validateParams() {
if (StringUtils.isBlank(this.bucketName))
throw notNull(
"bucketName");
if (this.maxKeys != null && (this.maxKeys > 1000 || this.maxKeys < 1))
throw between(
"maxKeys",String.valueOf(maxKeys),"1","1000");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy