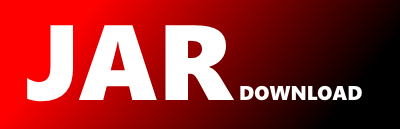
com.ksyun.ks3.utils.Md5Utils Maven / Gradle / Ivy
package com.ksyun.ks3.utils;
import org.apache.commons.logging.LogFactory;
import java.io.*;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
/**
* Utility methods for computing MD5 sums.
*/
public class Md5Utils {
private static final int SIXTEEN_K = 1 << 14;
/**
* Computes the MD5 hash of the data in the given input stream and returns
* it as an array of bytes.
* Note this method closes the given input stream upon completion.
*/
public static byte[] computeMD5Hash(InputStream is) throws IOException {
BufferedInputStream bis = new BufferedInputStream(is);
try {
MessageDigest messageDigest = MessageDigest.getInstance("MD5");
byte[] buffer = new byte[SIXTEEN_K];
int bytesRead;
while ((bytesRead = bis.read(buffer, 0, buffer.length)) != -1) {
messageDigest.update(buffer, 0, bytesRead);
}
return messageDigest.digest();
} catch (NoSuchAlgorithmException e) {
// should never get here
throw new IllegalStateException(e);
} finally {
try {
bis.close();
} catch (Exception e) {
LogFactory.getLog(Md5Utils.class).debug(
"Unable to close input stream of hash candidate: " + e);
}
}
}
/**
* Returns the MD5 in base64 for the data from the given input stream.
* Note this method closes the given input stream upon completion.
*/
public static String md5AsBase64(InputStream is) throws IOException {
return Base64.encodeAsString(computeMD5Hash(is));
}
/**
* Computes the MD5 hash of the given data and returns it as an array of
* bytes.
*/
public static byte[] computeMD5Hash(byte[] input) {
try {
MessageDigest md = MessageDigest.getInstance("MD5");
return md.digest(input);
} catch (NoSuchAlgorithmException e) {
// should never get here
throw new IllegalStateException(e);
}
}
/**
* Returns the MD5 in base64 for the given byte array.
*/
public static String md5AsBase64(byte[] input) {
return Base64.encodeAsString(computeMD5Hash(input));
}
/**
* Computes the MD5 of the given file.
*/
public static byte[] computeMD5Hash(File file) throws FileNotFoundException, IOException {
return computeMD5Hash(new FileInputStream(file));
}
/**
* Returns the MD5 in base64 for the given file.
*/
public static String md5AsBase64(File file) throws FileNotFoundException, IOException {
return Base64.encodeAsString(computeMD5Hash(file));
}
/**
* @Title:md5
* @Description: 加密
* @param str
* @return String
* @author kyj
*/
public static String md5(String str) {
String key = md5(str, "MD5", "UTF-8");
return key;
}
/**
* @method_name md5
* @desc
* @param str
* @param signType
* @param charset
* @return
* @date 2014-9-16
* @return String
* @throws
* @author kyj
*/
public static String md5(String str, String signType, String charset) {
if (str == null) {
return null;
}
MessageDigest messageDigest = null;
try {
messageDigest = MessageDigest.getInstance(signType);
messageDigest.reset();
messageDigest.update(str.getBytes(charset));
} catch (NoSuchAlgorithmException e) {
return str;
} catch (UnsupportedEncodingException e) {
return str;
}
byte[] byteArray = messageDigest.digest();
StringBuffer md5StrBuff = new StringBuffer();
for (int i = 0; i < byteArray.length; ++i)
if (Integer.toHexString(0xFF & byteArray[i]).length() == 1)
md5StrBuff.append("0").append(
Integer.toHexString(0xFF & byteArray[i]));
else
md5StrBuff.append(Integer.toHexString(0xFF & byteArray[i]));
return md5StrBuff.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy