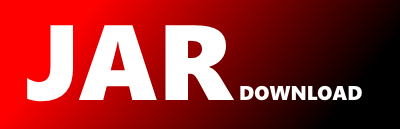
com.ktanx.autocoder.db.AbstractDatabaseProcessor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ktanx-autocoder Show documentation
Show all versions of ktanx-autocoder Show documentation
ktanx-autocoder is a java code generate kit.
The newest version!
package com.ktanx.autocoder.db;
import com.ktanx.autocoder.config.ColumnMetaData;
import com.ktanx.autocoder.config.JdbcConfig;
import com.ktanx.autocoder.config.TableMetaData;
import com.ktanx.common.exception.KtanxException;
import org.apache.commons.lang3.StringUtils;
import java.sql.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
public abstract class AbstractDatabaseProcessor implements DatabaseProcessor {
protected Connection connection;
private void initConnection(JdbcConfig jdbcConfig) {
try {
if (connection != null && !connection.isClosed()) {
return;
}
Class.forName(jdbcConfig.getDriverClassName());
connection = DriverManager.getConnection(jdbcConfig.getUrl(), jdbcConfig.getUsername(), jdbcConfig.getPassword());
} catch (ClassNotFoundException e) {
throw new KtanxException("Did not find the database driver class,whether to add the database driver jar ?");
} catch (SQLException e) {
throw new KtanxException("Create database connection error");
}
}
/**
* 获取数据库表的信息
*
* @param tableName 表名
* @return meta data
*/
public TableMetaData getTableMetaData(String tableName, JdbcConfig jdbcConfig) {
try {
initConnection(jdbcConfig);
TableMetaData.TableMetaDataBuilder builder = TableMetaData.builder();
String pkName = this.getPkName(tableName,connection);
Map columnComments = this.getColumnComments(tableName,connection);
tableName = this.getSelectTableName(tableName);
List columnList = new ArrayList<>();
//查询时没有数据,只返回表头信息
PreparedStatement pst = connection.prepareStatement("select * from " + tableName + " where 1=2");
ResultSetMetaData rsd = pst.executeQuery().getMetaData();
for (int i = 1; i <= rsd.getColumnCount(); i++) {
String name = rsd.getColumnName(i);
String dbType = rsd.getColumnTypeName(i);
String javaClass = rsd.getColumnClassName(i);
int nullable = rsd.isNullable(i);
int columnDisplaySize = rsd.getColumnDisplaySize(i);
int scale = rsd.getScale(i);
boolean autoIncrement = rsd.isAutoIncrement(i);
boolean isPk = StringUtils.equalsIgnoreCase(pkName, name) ? true : false;
ColumnMetaData columnMetaData = ColumnMetaData.builder()
.name(name)
.dbType(dbType)
.jdbcType(dbType)
.javaType(javaClass)
.isNullable(nullable == 1 ? true : false)
.displaySize(columnDisplaySize)
.scale(scale)
.isAutoIncrement(autoIncrement)
.comment(columnComments == null ? "" : columnComments.get(name))
.isPk(isPk)
.build();
columnList.add(columnMetaData);
}
pst.close();
TableMetaData tableMetaData = builder.name(tableName)
.columns(columnList)
.comment(this.getTableComment(tableName,connection))
.build();
return tableMetaData;
} catch (Exception e) {
throw new KtanxException(e);
}
}
/**
* 获取select查询sql使用的表名,一般情况下无需处理,必要时重写
* 只有当表名跟系统表名或关键字一样时,需要加工下
* 例如postgresql中USER表,必须是select * from "USER" —> 和系统表名重合需要加双引号
*
* @param tableName the table name
* @return select table name
*/
protected String getSelectTableName(String tableName) {
return tableName;
}
/**
* 获取表备注
*
* @param tableName the table name
* @param connection the connection
* @return table comment
* @throws SQLException the sQL exception
*/
protected String getTableComment(String tableName, Connection connection) throws SQLException {
return "";
}
/**
* 获取主键名
*
* @param tableName the table name
* @param connection the connection
* @return pk name
* @throws SQLException the sQL exception
*/
protected abstract String getPkName(String tableName, Connection connection) throws SQLException;
/**
* 获取列注释
*
* @param tableName the table name
* @param connection the connection
* @return column comments
* @throws SQLException the sQL exception
*/
protected abstract Map getColumnComments(String tableName, Connection connection) throws SQLException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy