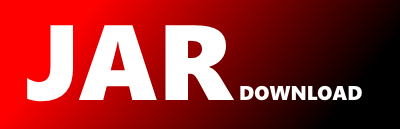
com.ktanx.autocoder.parse.xml.XmlConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ktanx-autocoder Show documentation
Show all versions of ktanx-autocoder Show documentation
ktanx-autocoder is a java code generate kit.
The newest version!
package com.ktanx.autocoder.parse.xml;
import com.ktanx.autocoder.config.*;
import com.ktanx.common.xml.XmlNode;
import java.util.*;
public class XmlConfiguration implements Configuration {
private XmlNode xmlNode;
private JdbcConfig jdbcConfig;
private Map constantMap;
private Map typeMappings;
private Map codeTaskMap;
private Map> tableCodeTaskMap;
public XmlConfiguration(XmlNode xmlNode) {
this.xmlNode = xmlNode;
constantMap = new HashMap<>();
typeMappings = new HashMap<>();
codeTaskMap = new HashMap<>();
tableCodeTaskMap = new HashMap<>();
List nodes = this.getRecursionNode(xmlNode, "include");
nodes.add(xmlNode);
for (XmlNode node : nodes) {
this.readJdbcConfig();
this.readConstants(node);
this.readTypeMappings(node);
this.readCodeTasks(node);
this.readTableCodeTasks(node);
}
}
@Override
public boolean isOverwrite() {
String overwrite = this.getConstant(Constants.OVERWRITE);
return Boolean.valueOf(overwrite);
}
@Override
public boolean isChildMode() {
String runOnChildModule = this.getConstant(Constants.RUN_ON_CHILD_MODULE);
return Boolean.valueOf(runOnChildModule);
}
@Override
public JdbcConfig getJdbcConfig() {
return this.jdbcConfig;
}
@Override
public String getConstant(String name) {
return constantMap.get(name);
}
@Override
public Map getConstants() {
return new HashMap<>(constantMap);
}
@Override
public TypeMapping getTypeMapping(String dbType) {
return typeMappings.get(dbType);
}
@Override
public Collection getCodeTasks() {
return codeTaskMap.values();
}
@Override
public CodeTask getCodeTask(String taskName) {
return codeTaskMap.get(taskName);
}
@Override
public Map> getTableCodeTasks() {
return new HashMap<>(tableCodeTaskMap);
}
private List getRecursionNode(XmlNode xmlNode, String nodeName) {
List result = new ArrayList<>();
List childNodes = xmlNode.getChildNodes(nodeName);
result.addAll(childNodes);
for (XmlNode childNode : childNodes) {
List nodes = this.getRecursionNode(childNode, nodeName);
result.addAll(nodes);
}
return result;
}
private void readJdbcConfig() {
XmlNode jdbcNode = xmlNode.getSingleChildNode("jdbc");
List childNodes = jdbcNode.getChildNodes();
JdbcConfig.JdbcConfigBuilder builder = JdbcConfig.builder();
for (XmlNode childNode : childNodes) {
String name = childNode.getAttribute("name");
String value = childNode.getAttribute("value");
switch (name) {
case "dialect":
builder.dialect(value);
break;
case "driverClassName":
builder.driverClassName(value);
break;
case "url":
builder.url(value);
break;
case "username":
builder.username(value);
break;
case "password":
builder.password(value);
break;
}
}
this.jdbcConfig = builder.build();
}
private void readConstants(XmlNode node) {
XmlNode constantsNode = node.getSingleChildNode("constants");
if (constantsNode == null) {
return;
}
List childNodes = constantsNode.getChildNodes();
for (XmlNode childNode : childNodes) {
String n = childNode.getAttribute("name");
String v = childNode.getAttribute("value");
constantMap.put(n, v);
}
}
private void readTypeMappings(XmlNode node) {
XmlNode mappingNode = node.getSingleChildNode("typeMapping");
if (mappingNode == null) {
return;
}
List mappingChildNodes = mappingNode.getChildNodes();
for (XmlNode childNode : mappingChildNodes) {
TypeMapping typeMapping = TypeMapping.builder()
.dbType(childNode.getAttribute("dbType").toUpperCase())
.javaType(childNode.getAttribute("javaType"))
.build();
typeMappings.put(typeMapping.getDbType(), typeMapping);
}
}
private void readCodeTasks(XmlNode node) {
XmlNode tasks = node.getSingleChildNode("tasks");
if (tasks == null) {
return;
}
List taskNodes = tasks.getChildNodes();
for (XmlNode childNode : taskNodes) {
String name = childNode.getAttribute("name");
List taskChildNodes = childNode.getChildNodes();
Map properties = new HashMap<>();
Map plugins = new HashMap<>();
for (XmlNode taskChildNode : taskChildNodes) {
switch (taskChildNode.getName()) {
case "property":
properties.put(taskChildNode.getAttribute("name"), taskChildNode.getAttribute("value"));
break;
case "plugin":
plugins.put(taskChildNode.getAttribute("name"), taskChildNode.getAttribute("value"));
break;
}
}
CodeTask codeTask = CodeTask.builder()
.name(name)
.properties(properties)
.plugins(plugins)
.build();
codeTaskMap.put(name, codeTask);
}
}
private void readTableCodeTasks(XmlNode node) {
XmlNode tableNode = node.getSingleChildNode("table");
if (tableNode == null) {
return;
}
String tableName = tableNode.getAttribute("name");
List childNodes = tableNode.getChildNodes();
List list = new ArrayList<>();
for (XmlNode childNode : childNodes) {
list.add(childNode.getAttribute("name"));
}
tableCodeTaskMap.put(tableName, list);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy